EventHandler in C#: What it is and How to Use it?
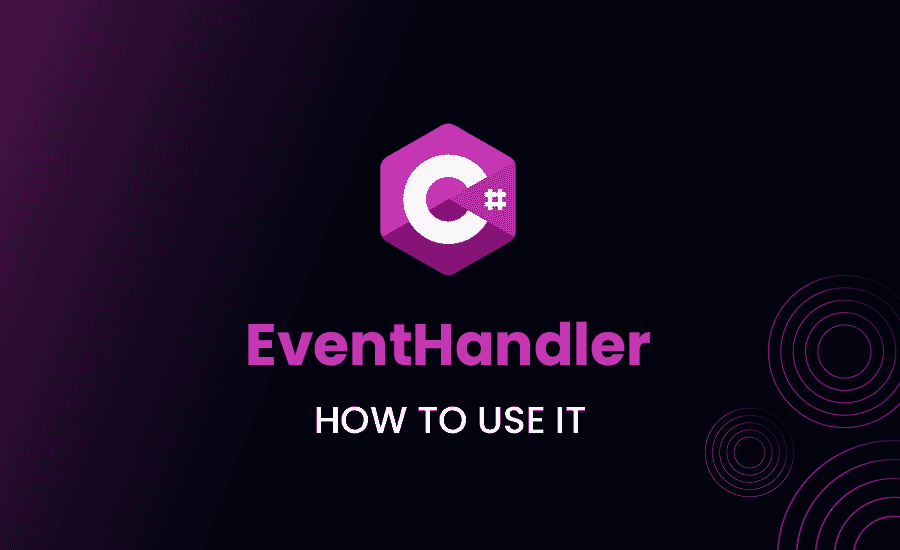
The EventHandler
in C# is a fundamental piece in the vast puzzle of C# programming. So, why is it so integral? Because it’s the cornerstone in event-driven programming, ushering the way for responsive and dynamic applications.
Right now, you may find it a bit fuzzy, but trust me, by the end of this article, it will all make sense. Here, we’ll take an enlightening journey from the basic stuffs – what, why and how of EventHandler
, to the advanced – custom EventHandler
, async, and more!
You are going to break all barriers and discover the full potential of EventHandler
in C#. So, are you ready to board this train? Then let’s get started!
Understanding the Concept of EventHandler in C#
Before diving straight into the pool of codes and functions, let’s first grasp the concept of EventHandler
in C#. Remember when your application automatically updated with real-time data, or how a button color changed when you clicked on it? This is all made possible with the help of Event Handling!
An Introduction to EventHandler C#
Every responsive application contains interactions, and hence, events – mouse clicks, key-presses, or something as simple as loading a page. But for every action to create a reaction, we need something in-between. Enter EventHandler
– the mediator between the event (action) and its corresponding function (reaction).
Loading code snippet...
In this simple application, when you click on Button1
, it will call the function Button1_Click
, where you can define what happens next.
Core Components and Functions of an EventHandler
The mechanics of an EventHandler
rotate around two core elements: the event
and the delegate
. Think of a scenario when you click a button, a message pops up. Here, the click is the event and the message pop-up is the function controlled by the delegate.
Now you may wonder, why do we need a delegate here? Well, delegate is the postman who carries the address (function pointer) of your reaction to your action.
There’s a lot more to explore about EventHandler
; how to create one, customize it, and much more. Ready to dive deeper?
How to Create an EventHandler in C#
Creating an EventHandler
in C# can seem like rocket science at first, but guess what? It’s not! Let’s crack the code now.
Step by Step Guide to c# create EventHandler
All you need to create an EventHandler are: an event keyword, a delegate and an Event Handler method, that’s all to create a magic interaction in your application.
Loading code snippet...
Here, when the event SomeEvent
is raised, it triggers MyEventHandler
method with the string “Hello, EventHandler!”
Importance of Custom EventHandler and How to Develop It
Adding a touch of customization to your EventHandler
can completely revamp the interaction of your application. But how do we do that?
C# Custom EventHandler
Creating a Custom EventHandler involves defining your own delegate unlike the typical EventHandler. Structurally it’s the same, all you need is to define your custom delegate.
Loading code snippet...
Here, we’ve created a custom delegate MyEventHandler
to handle CustomEvent
. The delegate, when trigged by the event, passes the string “Custom Event Handler!” – a custom reaction to your action!
EventHandler vs Delegate in C#
Just as you need both a guitarist and drummer to have a perfect jam, EventHandler
and delegate
together create the symphony of a responsive application.
Understanding c# delegate vs eventhandler
A delegate
is a type-safe function pointer, acting as a catalyst in initializing an event
. On the other hand, EventHandler
leverages this pointer to control the function of an event.
But wait…what if you want more control over adding or removing the EventHandler
? And can your EventHandler
process asynchronous events? Let’s take a deeper plunge into these concepts.
A Comparative Study: C# Event vs EventHandler
Just like five fingers of your hand aren’t the same, event
and EventHandler
too have their differences, even though they are part of the same hand of Event-Driven Programming.
Distinguishing between C# event vs eventhandler
An event
is a message sent by an object, announcing an action. EventHandler
is the code that responds to that announcement.
Practical Applications and Use-cases
Let’s say, you have a button on your website that, when clicked, displays a pop-up message. Here, the click is the event. The resulting pop-up message is the reaction controlled by the EventHandler.
Now, you may wonder how to add or remove these EventHandlers effectively without cluttering your code. That brings us to our next topic.
EventHandler Add Remove: A Detailed Overview
Adding and removing EventHandlers gives you precise control over your events. So tighten your seatbelts, as we’re about to glide into the world of c# eventhandler add remove
.
Understanding The Concept: C# eventhandler add remove
Imagine throwing a party. You send invites (add eventhandlers) and some guests RSVP, affirming their presence (subscribe to the event). Some might retract their RSVP (unsubscribe, hence remove eventhandler). This add-remove is a crucial feature to manage events effectively.
Loading code snippet...
Here, myObject.MyEvent
adds MyFunction
when an event is triggered and removes when it’s not needed anymore.
Async EventHandler in C#
In an era where time is everything, async EventHandler
makes your application more efficient, as it doesn’t block its code while processing an event. But how?
Essentials of C# async eventhandler
In regular event handling, your application suspends until an event processing completes, making it synchronous. But with async
, your event runs in the background, allowing your application code to run simultaneously.
Loading code snippet...
With async EventHandler, Button_Click
event triggers SomeFunction()
, which runs in the background, leaving your application undisrupted. Magic, isn’t it?
Dive Deep Into EventHandler BeginInvoke
BeginInvoke
is a hidden gem, allowing asynchronous call of your delegates. But what does it bring to EventHandler
?
Essentials of c# eventhandler begininvoke
Imagine multi-tasking, doing your laundry while cooking dinner. Just like how you accomplish two tasks simultaneously, BeginInvoke
helps EventHandler
call multiple events at the same time. Now that’s efficient!
Loading code snippet...
Here, MyEvent
is called asynchronously without disrupting the rest of your code.
A Brief on eventhandler eventargs in C#
Every EventHandler function carries an EventArgs
parameter, encapsulating event-specific data. So are you ready to unwrap the package?
Understanding c# eventhandler eventargs
Similar to how a pillbox contains different pills for different purposes, eventargs
provides diverse data for various events.
Loading code snippet...
Here, OnSomeEvent
handles SomeEvent
, using EventArgs
(e) to pass event data.
Mastering the Public Event EventHandler in C#
Remember how a public town-hall meeting allows everyone in town to participate? Public Event EventHandler
does the same! It allows other classes to access these events. Let’s see it in action!
Core Concepts and Functions of c# public event eventhandler
A public event EventHandler
is an accessible interaction point in your code from other classes, amplifying its versatility.
Loading code snippet...
RaiseCustomEvent
is an event accessible to other classes throughout your code.
Remember, mastering the EventHandler
puts you on a pedestal in the world of event-driven programming. So get onboard this journey of transforming your applications with EventHandler
. Now, when you encounter EventHandler
, won’t you smile saying, “EventHandler? Easy peasy!”