String Interpolation in C# for Efficient Code
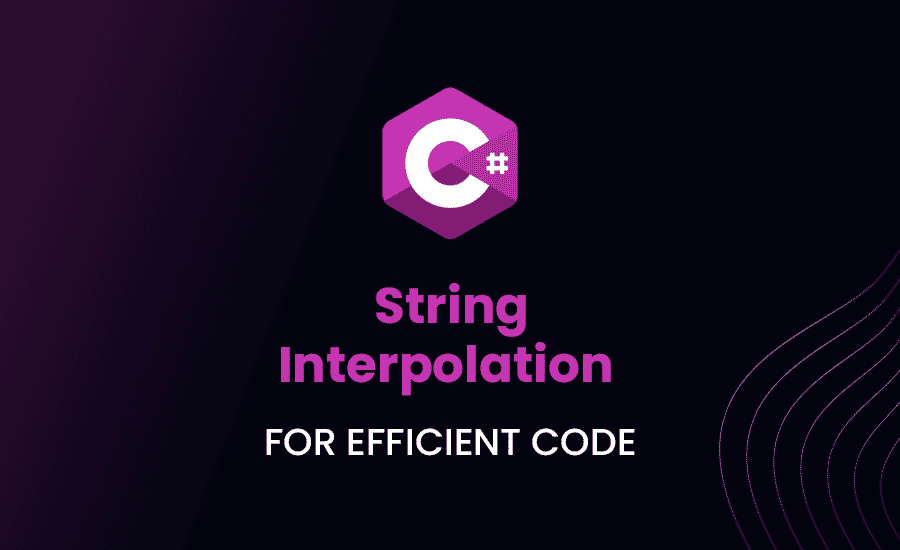
Have you ever been lost in a jungle of string concatenations, forgetting where one string starts and another ends? If your answer is yes, then you’re just in the right place. In this article, we’re all set to discuss how C# string interpolation can be your savior, simplifying that jungle and making your code clearer, cleaner, and more efficient.
Introduction to C# String Interpolation
Our code journey begins with a deep dive into C# String Interpolation. Why do the work when C# can do it for you?
C# Interpolate String: Basics and Syntax
In the C# universe, string interpolation is a method to define and format string values. It was introduced in C# version 6 to help programmers like us to embed variables directly inside of strings. No need for plus signs or complicated concatenations anymore!
Let’s take a look at a basic example:
Loading code snippet...
This code would output “I have 5 apples”. Neat, right? No concatenations, just pure, simple embedded code.
The Power of String Interpolation in C#
Now you may wonder: What’s the big deal? Well, imagine a program with tens, hundreds, maybe even thousands of strings. Handling them one by one is not only tedious but also an unnecessary reinvention of the wheel. Besides, interpolation handles complex expressions, not only simple variables. That, my dear developer, is the power of string interpolation.
Delving Deep into String Interpolation
We have seen a glimpse of string interpolation, but how does it work in real, complex coding scenarios?
C# String Interpolation Format: Cleaning Your Code
String interpolation also lets you format your values. Want to output the price as a decimal with 2 decimal places? You got it. Let’s see another example:
Loading code snippet...
This code would output “The price is $4.99”. C2 formats the price as currency with 2 decimal places. Saving time, and enhancing code readability is one stroke, that’s string interpolation for you.
Advanced Formatting with String Interpolation in C#
But wait, there’s more! String interpolation’s power extends beyond basic formatting. What if you want to add a conditional statement inside your string? Or process a function? Well, string interpolation has got you covered.
Conditional Statements and String Interpolation
Handling conditional statements is as easy as pie with string interpolation.
C# String Interpolation Conditional: Combining Logic with Interpolation
You can easily add simple conditions right within your interpolated string. No more concatenating chunks of string based on conditions. You can now do this:
Loading code snippet...
This code outputs “Today is Monday”. If day was not 1, it would output “Today is not Monday”. No mess, no confusion.
String Interpolation vs Concatenation
Now, we all love string concatenation a tiny bit. But let’s compare it to string interpolation and see who’s the real champ.
C# String Interpolation vs Concatenation Performance: A Comparative Insight
On the surface, string interpolation and concatenation could deliver the same results. But when you look under the hood, you’ll see the difference. String interpolation can handle more complex scenarios cleanly which really gives it an edge in terms of code readability and maintenance. Moreover, in terms of performance, string interpolation has the upper hand overall.
String Interpolation vs Concatenation C#: Choosing Wisely
In conclusion, while concatenation has its uses, string interpolation offers better and cleaner solutions. So, think wisely and choose for the long term.
Implementing String Interpolation in Console.WriteLine
Now, let’s put our acquired knowledge to use. Specifically, let’s see how string interpolation can simplify writing to the Console.
C# Console WriteLine String Interpolation: Simplifying Console Outputs
Remember how we usually concatenate variables and strings in a Console.WriteLine statement? With string interpolation, that’s history. Let’s take this example:
Loading code snippet...
And boom! The console displays “The current year is 2022”. No concatenations, no distractions.
Broadening the Scope: Dynamic String Interpolation
Now, let’s take our understanding up a notch. Time to dive into dynamic string interpolation!
Dynamic String Interpolation: Beyond Static Strings
Imagine you want your string to adapt to changing values and conditions. That’s when dynamic string interpolation comes into play. It allows you to generate strings dynamically, which can be pretty useful in many scenarios.
Real-world Scenarios of Using Dynamic String Interpolation in C#
Enough of the theory now, let’s have a look at a practical, real-world scenario where dynamic string interpolation can save us from unnecessary work.
Loading code snippet...
Managing Multiline Strings with Interpolation
Embedding expressions in multiline strings is a common need. But many developers find it challenging. Fear not, string interpolation is here to help!
C# Multiline String with Interpolation: A guide for edge cases
With C#, handling multiline strings becomes stress-free. Here’s an example:
Loading code snippet...
This code will print all sentences line by line. No extra effort, right?
Conclusion: Smart Coding with C# String Interpolation
In conclusion, every one of us aims to write clean and efficient code. And guess what? C# string interpolation is a game-changer in that quest. It’s powerful, versatile, and easy to use.
That’s it, folks. I hope you’ve gained some new insights and are all set to try out C# string interpolation. So what are you waiting for? Let’s dive into that code!