Enable CORS in ASP.NET Core in the Easiest Way
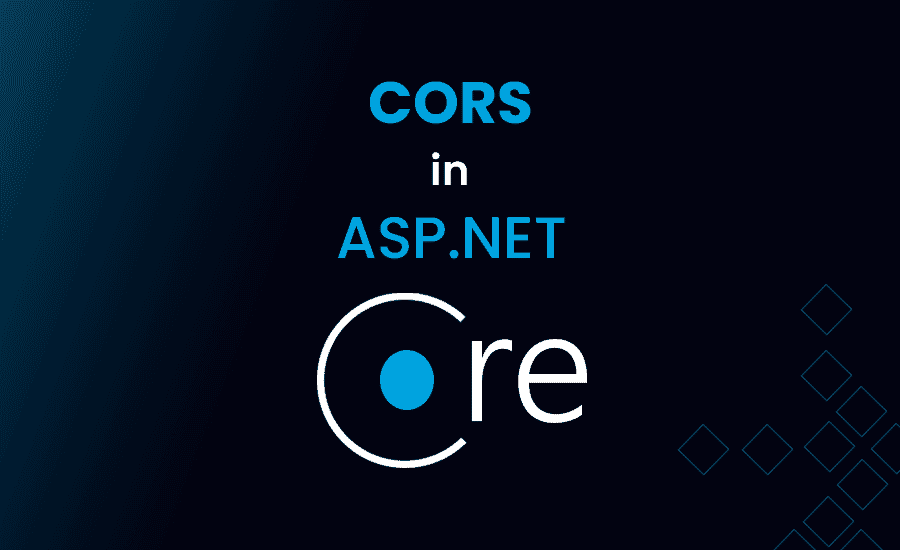
In this guide, we’ll unravel the core principles of Cross-Origin Resource Sharing (CORS) in ASP.NET Core. We will cover its role in web applications, provide practical examples and highlight some common mistakes to avoid.
Let’s dive in!
Introduction to CORS
Ever wondered about the invisible rules that keep your website interactions safe and secure? That’s where CORS, or Cross-Origin Resource Sharing, comes in. This primer will help us understand its importance in our web applications.
Understanding CORS and Its Importance
CORS or Cross-Origin Resource Sharing is the guardian angel that allows or restricts external domains to access resources from your domain. Imagine you’re hosting a party (your website), and CORS is the bouncer deciding who gets in (requests) based on the guest list (rules you’ve set). Oh, and that party? It’s one thrilling and secure online experience!
Loading code snippet...
How Does CORS Work
Once you send a request from a client to a server in the dance of web communication, the server responds with access control headers. These headers decide who gets to dance along to the beat (the resources). But hey, let’s not only talk in riddles, let’s dive deeper into CORS’s working mechanism in ASP.NET Core context in the next sections.
Loading code snippet...
Setting Up the Development Environment for ASP.NET Core
Software development is a bit like cooking: the right ingredients and proper tools can make all the difference. In our case, those tools are mainly software and your willpower to code.
Required Tools and Software
To cook up an irresistible ASP.NET Core application, you’ll need:
- .NET Core SDK
- Visual Studio or any other preferred code editor
- A burning desire to code
Loading code snippet...
Creating Your First ASP.NET Core Application
Like a chef preparing a new recipe, let’s create our first ASP.NET Core web application. By doing so, we’ll lay the groundwork necessary to explore CORS in ASP.NET Core in the upcoming sections.
Loading code snippet...
Voila! You have created your first ASP.NET Core application.
The Role of CORS in ASP.NET Core
Are you ready to untangle the spaghetti of CORS in the context of ASP.NET Core? Fear not, for we are armed with the shining armor of knowledge and a sprinkle of good humor.
Basic Concepts of CORS in NET Core
Let’s refresh our basics; every master coder was once a beginner, right? CORS is central to ASP.NET Core, especially when developing web applications that interact with resources from different domains.
Loading code snippet...
ASP.NET Core and CORS: A Detailed Overview
Let’s cover some common scenarios in which CORS is pivotal in developing sophisticated features in ASP.NET Core. Incoming, real-life examples and mind-blowing explanations!
How to Enable CORS in ASP.NET Core
Hey friend, ready to enable your first CORS in ASP.NET Core? Well, buckle up, because you’re in for an exhilarating ride!
Step-by-step Guide to Enabling CORS in Web API NET Core
Firstly, we need to install the Microsoft.AspNetCore.Cors package. Then, add the CORS service in ConfigureServices method and apply the policy in the Configure method. I know, it sounds tricky, but let’s break it down step-by-step with code examples.
Loading code snippet...
Understanding NET Core Allow CORS Methods
Are you tired of the legends and myths surrounding NET Core Allow CORS methods? Time to bust those myths with some concrete knowledge and non-negotiable facts! And yep, you guessed it – we’ll see how we can apply these CORS methods to our ASP.NET Core application.
Loading code snippet...
That concludes our fascinating tour into the world of CORS in ASP.NET Core. We’ve demystified CORS, danced around with its implementation in ASP.NET Core, and even seen how we can enable it. Remember, the ASP.NET Core world is your oyster, and CORS is your pearl of secure web communication.
Practical Examples of Using CORS in ASP.NET Core
Our journey into the realm of CORS and ASP.NET Core just got more interesting. Ready to roll up your sleeves and dive head-first into some real-world examples? Let’s do it!
A Practical Example of CORS ASP.NET Core
CORS and ASP.NET Core go together like bread and butter, cookies and milk, or… well, you get the idea. To make this partnership crystal clear, let’s look at a practical example where we configure an ASP.NET Core application to allow requests from specific origins.
Loading code snippet...
Bazinga! We have set a CORS policy “AllowSpecificOrigin” that allows requests from the domains http://example.com and http://example2.com.
ASP.NET Core CORS Allow All: What Does It Mean and When to Use It
Sometimes, you simply want to open all the doors and have a grand open house. Or in tech terms, you want to accept requests from all origins. Let’s see how we can “allow all” using ASP.NET Core CORS.
Loading code snippet...
And there you have it, friend! The ASP.NET Core CORS Allow All policy in its full glory.
Applying CORS policies on a granular level (e.g., to individual controllers or actions) can give you more control over your application. But remember, with great power comes great responsibility, so make sure you handle your CORS powers wisely.
Deep Dive into CORS Policies in ASP.NET Core
As we continue our journey through the core of CORS policies within ASP.NET Core, we’re about to dive even deeper. Brace yourself, as we ‘go under the hood’ and explore how to tailor CORS policies to suit specific use cases. Notice what I did there? Brace? Dive? Alright, let’s move on…
Adding CORS Policies in ASP.NET Core: An In-Depth Guide
Crafting CORS policies is like sculpting a piece of art – it gives you control over who can admire (access) your masterpiece (web application) and how they can interact with it. With that spirited analogy, let’s go on a crafty expedition to add and configure CORS policies in ASP.NET Core.
Loading code snippet...
That was exhilarating, right? But wait, there’s more!
Enable CORS NET Core: Understanding CORS Policy Options
Battles are won by understanding your weapons! Knowing CORS policy options comes handy when tailor-making your own policies. Let’s dive into the ocean of policy options, so we can emerge as triumphant warriors safeguarding our apps from unnecessary cross-origin requests.
Here is a snapshot of CORS policy options:
WithOrigins
: To allow requests only from specific domains.AllowAnyOrigin
: To accept requests from any domain (use with caution).AllowAnyMethod
: To allow any HTTP method (GET, POST, PUT etc.) in the request.WithMethods
: To specify which HTTP methods are allowed in the request.AllowAnyHeader
: To accept any HTTP request header.WithHeaders
: To specify which HTTP headers are allowed in the request.WithExposedHeaders
: To specify which headers are safe to expose to the API of a CORS API specification.AllowCredentials
: To allow requests with credentials.
Clearly, these options are your sword and shield while configuring CORS policies in ASP.NET Core.
Now, let’s apply this freshly-acquired knowledge to use the ‘AllowSpecificOrigins’ policy.
ASP.NET Core Allow CORS: Utilizing the ‘AllowSpecificOrigins’ Policy
The ‘AllowSpecificOrigins’ policy is like the gatekeeper of your ASP.NET Core application. It allows entry only to the domains on your ‘guest list’. Let’s see how this policy can be used to manage cross-origin requests. Remember our lovely analogy about a party? Yeah, we’re hanging on to it!
Loading code snippet...
Remember how we learned to ride a bike? Start slow, understand how it works, fall (maybe a little), get up and then ride like a champ? Well, that’s pretty much how you master CORS policies in ASP.NET Core. You start by understanding what CORS is, dive into how CORS works in ASP.NET Core, understand how to enable it, and finally, nail it by adding intricately designed policies.
CORS Middleware in .NET 6
Up next, we’ll unfold the mystery surrounding the pivotal role of middleware in .NET 6 while managing CORS. It’s about to get even more technical and exciting, so hold on to your hats!
Understanding the Role of Middleware in Net Core 6 CORS Handling
In the grand orchestra of .NET 6, middleware is like a conductor, managing how different components interact with each other. It’s a pivotal cog in the machine, especially when handling CORS. Let’s examine this conductor’s role and see how well it plays the symphony of the cross-origin resource sharing.
Loading code snippet...
How to AddCORS Middleware in NET Core 6
Alrighty, ready to play the role of conductor and add CORS middleware to your .NET 6 application? Let’s create a harmonious symphony that revolves around allowing cross-origin requests from specific domains. In the process, you’ll understand how middleware can make your life easier!
Loading code snippet...
Now your application will accept cross-origin requests from http://example.com.
That’s pretty much everything you need to get started with CORS in ASP.NET Core and .NET 6. At this point, you should be familiar with how CORS works, why it’s crucial for your applications, and how to implement it using middleware in .NET 6. Congratulations, you’re now a CORS ninja!
But wait, that’s not it. All ninjas should be ready to avoid traps during their mission. So let’s discuss some common pitfalls and best practices when implementing CORS in ASP.NET Core.
Avoid Common Mistakes with CORS in ASP.NET Core
CORS in NET Core opens up a world of connectivity, but the road to success is riddled with pitfalls. Let’s be prepare ourselves to step wisely on this path.
Pitfalls in CORS NET Core: What to Watch Out For
In this enlightening quest of CORS in NET Core, one cannot ignore the potential pitfalls threatening to throw us off track. However, with our guide to common mistakes and solutions, these won’t seem menacing anymore.
Firstly, always be vigilant about the middleware order. The positioning of app.UseCors()
is paramount in your configuration.
Loading code snippet...
Loading code snippet...
Secondly, never pair AllowAnyOrigin()
and AllowCredentials()
due to potential security risks. It’s akin to leaving your front door open!
Loading code snippet...
Let’s not forget, CORS is not a substitute for user authorizations and permissions. CORS merely adds an additional layer of security.
Loading code snippet...
Remember, a keen eye, cautious mind, and double-checking your code can save you from these pitfalls in the journey of CORS in .NET Core!
Best Practices for Implementing CORS C#
The path to becoming an exemplary C# developer is not just steering clear of blunders but embracing best practices. So let’s stroll through essential practices in the realm of CORS C#.
Firstly, do not use AllowAnyOrigin()
and AllowAnyMethod()
in production – an open door is an invitation to trouble!
Loading code snippet...
Implement vigorous CORS policies by regulating the origins, headers, and methods.
Loading code snippet...
Lastly, never underestimate the vitality of updates. Imagine always carrying the latest toolkit – it’s a lifesaver!
- Keep an eye on the official ASP.NET Core documentation for updates
- Stay proactive in updating your CORS middleware to the latest version
Following these practices could be your first steps towards becoming a trusted and respected C# developer. Aim high, friend!
Conclusion: The Power of CORS in ASP.NET
Quite a journey, wasn’t it? We’ve journeyed through the realm of CORS, danced with the intricacies of ASP.NET Core, and navigated the twists and turns of middleware in .NET 6.
Recap of Enabling CORS in Net Core
We broke down the fundamentals of CORS, explored how to set up the development environment for ASP.NET Core, examined the roles and methods of enabling CORS, and gave you the practical examples you needed. We then took a thrilling plunge into CORS policies, saw how middleware fits into the picture, and even debunked some CORS related myths.
Further Steps for Mastering CORS in ASP.NET Core.
However, we are standing on the shoulders of giants, and there’s more window to peek from. So explore, dive deeper and don’t hesitate to get your hands dirty. Leverage CORS policies to ensure your applications are top-notch when it comes to managing cross-origin requests securely. The world of ASP.NET Core is vast and fascinating, so we encourage you to continue tinkering, creating, and innovating. Happy coding!