C# Nagarro Interview Questions and Answers
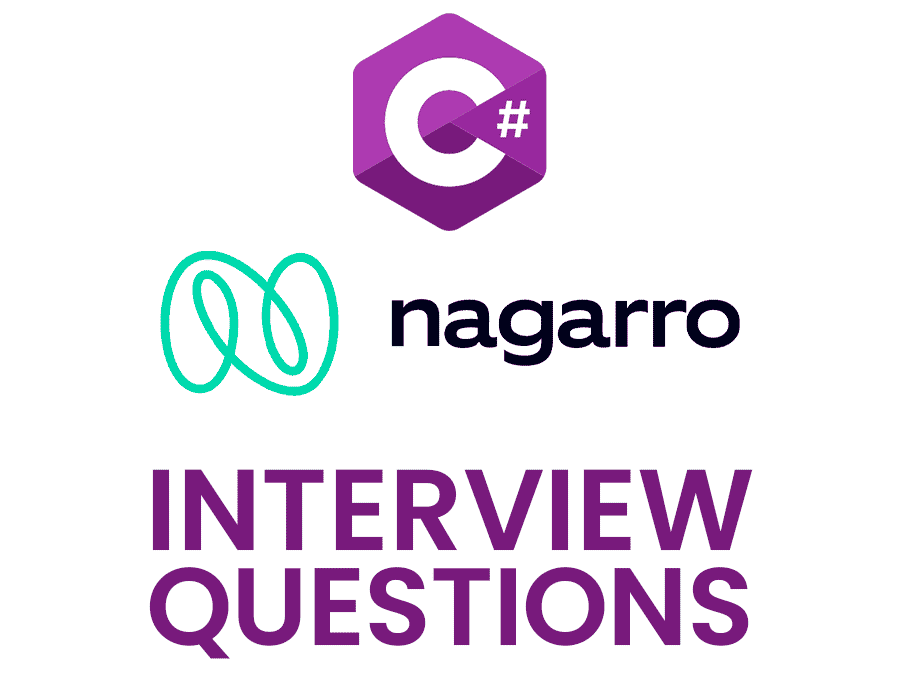
Welcome to our comprehensive guide on Nagarro C# interview questions and answers, designed to help you ace your next coding interview. With the increasing demand for C# developers in the industry, Nagarro, a leading software development company, has been known for its rigorous coding tests and interview process.
This article covers a wide range of topics, including the essential concepts of C#, Entity Framework, SignalR, Dependency Injection, and much more. Whether you are preparing for a Nagarro C# coding test, brushing up on nagarro coding questions, or simply looking to expand your knowledge, this article is tailored to meet your needs.
So, let’s dive in and take your C# skills to the next level!
Describe the modifications required in the Entity Framework to implement the repository pattern in a Nagarro C# project. What are the benefits of this approach?
Answer
To implement the repository pattern in a Nagarro C# project using Entity Framework, follow these steps:
- Create domain models: Start by defining your domain models/entities that represent tables in your database.
- Create IRepository interface: Define a generic IRepository interface that outlines the basic CRUD operations, such as Add, Update, Delete, GetById, and GetAll.
Loading code snippet...
- Create RepositoryBase class: Implement a RepositoryBase class that inherits from IRepository and provides the basic CRUD operations using the context of Entity Framework.
Loading code snippet...
- Create specific repositories: For specific domain models, create their corresponding repository classes that inherit from the RepositoryBase class.
Loading code snippet...
- Register repositories in DI container: Register the repositories in the dependency injection container to make them available throughout the application.
Benefits of using the repository pattern with Entity Framework include:
- Abstraction: The repository pattern provides an abstraction layer between data access and business logic, making it easier to modify and maintain the application.
- Testability: It improves testability by isolating data access components and allowing you to create mock repositories for testing.
- Centralized data access: By centralizing data access logic in repositories, you reduce code duplication and improve consistency and maintainability.
Discuss the best practices for handling real-time updates in a Nagarro C# project using SignalR along with its appropriate use cases.
Answer
Here are some best practices and guidelines for handling real-time updates using SignalR in Nagarro C# projects:
- Choose the right transport: SignalR automatically selects the best transport based on client capabilities, but you can specify a preferred transport by configuring it when setting up the connection. WebSockets are generally preferred as they offer low latency communication.
- Keep message payloads small: Ensure that the data sent over SignalR connections is lightweight and minimal. Smaller payloads reduce bandwidth utilization and improve performance.
- Manage client connections carefully: When a client is disconnected, make sure to handle reconnection logic gracefully. You may need to manage groups, subscriptions, or other metadata associated with clients as they connect and disconnect.
- Optimize server performance: Ensure that the server-side code is lightweight and doesn’t block the execution. Use async/await to handle asynchronous operations and prevent blocking the calling threads.
- Scale-out the backplane: Consider using a scale-out backplane, such as Redis or Azure Service Bus, to distribute messages across multiple servers. This helps handle high traffic loads and maintain real-time connections.
- Implement appropriate authorization and authentication: Ensure that your SignalR hubs and methods are protected with the necessary authentication and authorization mechanisms. Validate client connections and enforce access control.
- Monitor and log: Instrument your SignalR applications to collect performance and diagnostic data and monitor logs to identify issues and potential bottlenecks.
Appropriate use cases for SignalR include:
- Real-time dashboards or monitoring systems
- Chat applications
- Notification systems
- Collaborative editing or drawing applications
- Online gaming
Explain the critical aspects of using a DbContext within a using block in C#. What precautions should be taken when working with DbContext instances in a Nagarro project to ensure proper resource management?
Answer
DbContext represents a session with the database, and it holds the connection, transactions, and change tracking. When using a DbContext within a using block in C#, it gets automatically disposed at the end of the block, ensuring proper resource management. However, there are some critical aspects and precautions to consider when working with DbContext instances:
- Short-lived usage: DbContext is designed to be short-lived. Ideally, you should instantiate it, perform the operations, and dispose it as soon as possible. Using blocks help with this by disposing of the DbContext at the end of the block.
- Concurrency management: Executing multiple operations concurrently using the same DbContext instance may lead to inconsistent data states or other concurrency-related issues. Ensure that each thread uses a separate DbContext instance, or synchronize access to the shared instance to prevent concurrent modifications.
- Lazily loading and disposing: If you have navigation properties that are set to be lazy-loaded, accessing them after disposal of the DbContext will result in exceptions. To avoid this, either fetch the necessary data before disposing of the DbContext or switch to eager or explicit loading.
- Avoiding leaking connections: Dispose of the DbContext properly to avoid leaking database connections and exhausting the connection pool. When not using a using block, call the
Dispose
method explicitly, or implement theIDisposable
interface in the class that holds the DbContext instance. - Dependency Injection and lifetime: When using dependency injection (DI) to manage your DbContext instances, ensure that the DI container handles the lifetime of the DbContext correctly. For example, using a scoped DbContext in a web application helps to create a new instance per request, ensuring short-lived usage and proper disposal.
By following these precautions, you can ensure proper resource management and avoid issues related to DbContext instances in a Nagarro C# project.
Describe the use of NLog for logging purposes in a Nagarro C# application. Explain how you would implement custom logging targets and filters, ensuring maximum performance.
Answer
NLog is a flexible and high-performance logging library for .NET applications. To use NLog for logging purposes in a Nagarro C# application, follow these steps:
- Installation: Install the NLog package using NuGet.
Loading code snippet...
- Configuration: Create an NLog configuration file (NLog.config) in your project to define the logging rules, targets, and layout.
Loading code snippet...
- Logging: Use the Nlog LogManager to create a logger instance and log messages.
Loading code snippet...
To implement custom logging targets, follow these steps:
- Inherit from Target: Create a new class that inherits from the
NLog.Targets.Target
(orNLog.Targets.TargetWithLayout
, if you want to use a custom layout) and overrides theWrite
(orWriteAsyncLogEvent
, for async version) method.
Loading code snippet...
- Register custom target: Register the custom target using the
NLog.Config.ConfigurationItemFactory
in the NLog configuration.
Loading code snippet...
For performance, you can consider the following:
- Use asynchronous and buffered targets for slower targets (e.g., writing to a database or sending over the network).
- Choose the logging levels carefully to avoid unnecessary overhead.
- Use filtering and conditional layouts to output only relevant information.
How would you implement Dependency Injection (DI) using the Autofac library in a Nagarro C# project? Describe the steps and benefits of using Autofac as your DI container.
Answer
Here are the steps to implement Dependency Injection (DI) using the Autofac library in a Nagarro C# project:
- Add Autofac to the project: First, install the Autofac package using NuGet.
Loading code snippet...
- Create an Autofac ContainerBuilder: Initialize a new instance of the
Autofac.ContainerBuilder
class.
Loading code snippet...
- Register services and types: Register your types, services, and their configurations (e.g., interfaces, classes, lifetimes) using the
ContainerBuilder
.
Loading code snippet...
- Build the container: Call the
Build
method on theContainerBuilder
to create a newIContainer
instance.
Loading code snippet...
- Resolve dependencies: Use the
IContainer
instance to resolve your dependencies in the application.
Loading code snippet...
- Integrate with application frameworks: Autofac provides integration packages for popular application frameworks (e.g., ASP.NET Core, ASP.NET MVC, WCF, etc.). Use the appropriate package to configure Autofac as the default DI container for the respective framework.
Benefits of using Autofac as your DI container include:
- Flexibility: Autofac offers a high level of flexibility with advanced features such as open generics, property injection, and assembly scanning.
- Performance: Autofac is known for its performance and optimization.
- Ease of configuration: Autofac allows easy registration of components, factories, and instances with various lifetime scopes (e.g., single instance, per scope, etc.).
- Integration: Autofac provides seamless integration with popular application frameworks, making it a preferred choice for various types of projects.
- Testability: Dependency Injection using Autofac enables better testability of your application, as you can easily substitute dependencies with mock implementations during testing.
As we move further into the intricacies of Nagarro C# projects, let’s shift our focus from dependency management to design patterns. Understanding how to implement clean and maintainable code is crucial in modern software development.
In the following questions, we will discuss strategies that can make your code easier to test, manage, and update.
Explain the process of implementing Unit of Work design pattern in a Nagarro C# project to handle transactions. How does this pattern help improve maintainability and testability of the code?
Answer
To implement the Unit of Work design pattern in a Nagarro C# project, follow these steps:
- Create IUnitOfWork interface: Define an interface (e.g.,
IUnitOfWork
) for the Unit of Work, which outlines the key methods likeCommit
and, if necessary,Rollback
.
Loading code snippet...
- Create UnitOfWork class: Implement a
UnitOfWork
class that inherits from theIUnitOfWork
interface and wraps the DbContext.
Loading code snippet...
- Use UnitOfWork with repositories: Modify your repositories to work with the
IUnitOfWork
implementation.
Loading code snippet...
Implementing the Unit of Work design pattern helps improve maintainability and testability of the code in the following ways:
- Consolidates transaction management: Unit of Work centralizes transaction management, making it easier to enforce consistency and handle transaction-related tasks.
- Decouples repositories: By handling transactions at the Unit of Work level instead of inside individual repositories, the codebase becomes more modular and maintainable.
- Simplifies testing: The Unit of Work pattern simplifies testing, as you can mock or stub the transaction process, isolating the repositories and their associated operations. This makes it easier to test individual repository methods without worrying about external transactions or database concerns.
- Increases flexibility: The Unit of Work pattern enables flexibility in handling transactions across different repositories, which improves overall application design and management.
What is non-blocking I/O in the context of a Nagarro C# project? Discuss the key aspects of implementing asynchronous operations using async/await and potential pitfalls to watch for while coding.
Answer
In the context of a Nagarro C# project, non-blocking I/O refers to performing input/output operations, such as file or network access, without blocking the execution of the calling thread. Non-blocking I/O is essential for improving application performance and responsiveness, especially when dealing with long-running or resource-intensive operations.
Key aspects of implementing asynchronous operations using async/await in C# include:
- Async methods: Declare methods that perform asynchronous operations with the
async
keyword. These methods typically return aTask
orTask<T>
object.
Loading code snippet...
- Await keyword: Use the
await
keyword to call asynchronous methods, which suspends the execution of the current method until the awaitedTask
orTask<T>
is completed. This allows the calling thread to continue processing other work while waiting for the asynchronous operation.
Loading code snippet...
- ConfigureAwait: When using async/await in a library or component that may be consumed by other applications (e.g., UI applications), use
ConfigureAwait(false)
to avoid capturing the original synchronization context. This helps prevent potential deadlocks.
Loading code snippet...
Potential pitfalls to watch for while coding with async/await include:
- Deadlocks: Ensure that you properly handle synchronization contexts and avoid blocking on async code (e.g.,
Task.Result
orTask.Wait()
) to prevent deadlocks. - Exception handling: Asynchronous methods require special attention to exception handling. Use try-catch blocks around awaited tasks to handle exceptions correctly.
- Memory consumption: When using async/await in loops or recursive calls, be cautious about inadvertently creating large numbers of Task objects, which may lead to increased memory consumption.
- UI responsiveness: Ensure that you use async/await correctly in UI applications to keep the main thread free for processing user interactions. Avoid running CPU-bound operations on the main thread.
- Nested async calls: Be aware of nested async calls and handle them properly to prevent unobserved exceptions or unexpected behavior.
Describe the essential considerations while using Task Parallel Library (TPL) for parallelization in a Nagarro C# project. How would you ensure thread safety and optimum performance?
Answer
Essential considerations while using Task Parallel Library (TPL) for parallelization in a Nagarro C# project include:
- Selecting the right parallel construct: Based on your use case, choose the appropriate TPL construct, such as
Task
,Parallel.For
,Parallel.ForEach
, orParallel.Invoke
, that best fits the scenario. - CancellationToken: Use
CancellationToken
to enable cancellation of parallel operations, like tasks or loops, to gracefully terminate a parallel operation when required. - Exception handling: Handle exceptions in parallel operations using the AggregateException class if using TPL constructs like
Parallel.For
orParallel.ForEach
, and use try-catch blocks in the delegated tasks. - Limit degree of parallelism: For better performance and resource utilization, limit the degree of parallelism using
MaxDegreeOfParallelism
, especially in environments with many concurrent tasks or limited resources.
Loading code snippet...
- TaskSchedulers: When required, use custom
TaskScheduler
implementations to manage task scheduling and allocate resources efficiently. - Partitioning data: Using the right partitioning strategy for working with large datasets can significantly impact performance. Consider partitioning data appropriately, such as using
Partitioner.Create
.
To ensure thread safety and optimum performance:
- Identify critical sections in the code that must be protected from concurrent access. Use synchronization constructs, such as
lock
,SemaphoreSlim
,Mutex
, orMonitor
, to synchronize access to shared resources. - Use the
Concurrent
namespace collections instead of standard collections while working with parallel operations to avoid the overhead of manual synchronization and ensure thread safety. - Avoid using thread-static members, as they do not guarantee thread safety in parallel scenarios.
- Balance between parallelism and potential overhead of task creation and context switching, as excessive parallelism may lead to diminished performance. Adjust parallelism levels based on available system resources.
By following these considerations, you can ensure thread safety and optimum performance while using the Task Parallel Library (TPL) in a Nagarro C# project.
Compare and contrast the use of AutoMapper and manual mapping in Nagarro C# projects. What are the potential risks and challenges associated with AutoMapper, and how can they be mitigated?
Answer
- AutoMapper is a popular open-source library used to map properties between different object types.
- It allows developers to reduce the amount of manual mapping code, making the codebase more maintainable and less error-prone.
- AutoMapper supports features like nested mapping, custom value resolvers, and projection.
Manual Mapping:
- Manual mapping requires writing custom code to map properties between source and destination objects.
- It gives developers full control over the mapping process, which can be useful for complex scenarios.
- Manual mapping can lead to a more significant amount of boilerplate code, which can make the codebase harder to maintain.
Risks and Challenges with AutoMapper:
- Performance: AutoMapper can be slower than manual mapping, primarily due to using reflection to discover properties to map. However, in most scenarios, the performance difference will not be noticeable.
- Hidden Errors: AutoMapper, by default, will silently ignore unmappable properties. This can lead to hidden errors in the mapping if a developer forgets to map a property or makes a spelling mistake.
- Complex Mappings: AutoMapper may not always handle complex mappings without additional configuration, which can result in less-readable code compared to manual mapping.
Mitigation strategies:
- Use
AssertConfigurationIsValid
to validate the mapping configuration during application startup or integration tests, ensuring that all properties are correctly mapped. - Use custom value resolvers or type converters when dealing with complex mappings to maintain clean and readable code.
- Use the
ExplicitExpansion()
option for mapping properties that require expensive calculations or database queries, allowing for more control over performance. - Familiarize yourself with the various features and configuration options in AutoMapper to maximize the benefits while avoiding pitfalls.
Discuss the pros and cons of using Dapper as an ORM in a Nagarro C# project. How does its performance compare to Entity Framework in various use cases?
Answer
Pros of using Dapper:
- Performance: Dapper is a lightweight, fast, and efficient micro-ORM, offering better performance in most scenarios compared to Entity Framework.
- Close to SQL: Dapper works very close to raw SQL, giving developers full control over the queries and SQL features.
- Flexibility: Dapper allows for a minimalistic and clean coding approach, making it easier to adapt to various use cases or integrate with other libraries.
- Easy to learn: As Dapper has a lighter surface area and less magic, it’s easier to understand its concepts and quickly incorporate it into a project.
Cons of using Dapper:
- Lack of advanced ORM features: Dapper does not offer features such as change tracking, lazy loading, or LINQ support, like Entity Framework.
- More manual coding: Using Dapper may require more manual coding compared to Entity Framework’s more automated nature, potentially resulting in more boilerplate code.
- No built-in Unit of Work pattern: Unlike Entity Framework, Dapper does not have built-in support for the Unit of Work pattern, requiring developers to implement their transaction handling.
Performance Comparison:While Dapper often outperforms Entity Framework in terms of raw query and object-mapping performance, the difference might not be significant for smaller projects or simple queries. However, when dealing with complex scenarios or demanding use cases, the superior performance of Dapper can provide a vital advantage.
It’s essential to consider each project’s specific requirements and weigh the benefits of Dapper’s performance and flexibility against EntityManager’s advanced functionality and ease of use when making a decision.
the data is divided among parallel tasks.
- Task Composition: Use
Task.WaitAll()
,Task.WhenAll()
,Task.WaitAny()
, orTask.WhenAny()
to control the execution flow when working with multiple tasks. Leverageasync/await
when dealing with I/O bound operations or a mix of I/O and CPU-bound tasks. - Throttling: Limit parallelism when necessary, using tools like
SemaphoreSlim
or customTaskScheduler
implementations, to avoid overwhelming shared resources or saturating the system.
Thread safety and optimum performance:
- Locking: Use C#
lock
statement or other synchronization primitives likeMonitor
,Mutex
,Semaphore
,ReaderWriterLockSlim
when accessing shared resources that need to be protected from concurrent access. - Concurrent Collections: Utilize thread-safe collections provided by .NET, like
ConcurrentDictionary
,ConcurrentQueue
,ConcurrentBag
, orBlockingCollection
to safely manage shared data structures. - Immutable Data Structures: Use immutable data structures and encourage immutability, reducing the need for synchronization and making the code safer for concurrency.
- Parallelism Degree: Be aware of the appropriate level of parallelism for your specific use case. You can control the parallelism degree using the
MaxDegreeOfParallelism
property of theParallelOptions
class when working with theParallel
class. - Performance Profiling: Regularly profile your parallel code to identify any bottlenecks, contention or other issues that might affect performance. Use tools like Visual Studio Diagnostics Tools or other profiling utilities to gain insights and optimize your code accordingly.
By following these guidelines and best practices, you can ensure thread safety and achieve optimal performance in your Nagarro C# projects using the Task Parallel Library.
Now that we’ve explored some fundamental aspects of Nagarro C# coding tests, it’s time to delve into more advanced topics related to data management and caching.
Efficient data handling and caching techniques can significantly improve your application’s performance and user experience. Let’s examine how to implement these strategies in a Nagarro C# project.
Walk us through the process of implementing data caching using Redis in a Nagarro C# project. Explain the essential configuration settings and strategies to cache data effectively.
Answer
Implementing Redis cache in a Nagarro C# project involves the following steps:
- Install Redis server: To use Redis in your C# project, you first need to have a Redis server installed and running. You can either set up a local Redis server for development or use a cloud provider like Azure Redis Cache.
- Install StackExchange.Redis NuGet package: In your C# project, install the
StackExchange.Redis
NuGet package, which is a popular library to interact with Redis from C# applications. - Create ConnectionMultiplexer: This will handle the connections to the Redis server. ConnectionMultiplexer is designed to be shared, and it’s recommended to use a single instance throughout your application:
Loading code snippet...
Replace "localhost"
with the connection string to your Redis server.
- Interact with Redis: To cache data in Redis, obtain a reference to the database and store/retrieve the data using the
StringSet
andStringGet
methods. Here’s an example:
Loading code snippet...
- Configuration Settings: Some essential configuration settings include:
connectTimeout
: The timeout in milliseconds to establish a connection to Redis.keepAlive
: To maintain the connection and avoid continuous reconnections, set the keepAlive parameter to a greater value.ssl
: If using encrypted communication, enabling SSL is crucial.
- Caching Strategies: Select an appropriate caching strategy based on your application requirements. Some standard strategies include:
- Cache-Aside: Load data into the cache when requested, and check the cache first before querying the primary data store.
- Read-through: Data is read from the primary data store and written to the cache automatically.
- Write-through: Data is written to both cache and primary data store simultaneously.
Explain the strategy to implement various Authentication and Authorization mechanisms in a Nagarro C# application, including OAuth2, OpenID Connect, and JWT. How would you secure different services and APIs?
Answer
To implement various authentication and authorization mechanisms in a Nagarro C# application, follow these steps:
- Use ASP.NET Core Identity: Start by setting up ASP.NET Core Identity, which provides essential building blocks for managing users and securing your application.
- OAuth2: To implement OAuth2, use the built-in
OAuthAuthorizationServerMiddleware
. Register the middleware inStartup.cs
, configure the endpoints, and provide implementations forIAuthorizationCodeProvider
andIAccessTokenProvider
. Ensure to use a secure token generation and storage mechanism. - OpenID Connect: For implementing OpenID Connect, add the
Microsoft.AspNetCore.Authentication.OpenIdConnect
NuGet package. Then, configure the middleware inStartup.cs
to use your identity provider (e.g., Azure AD, Google, or custom provider) and specify the Authority, Client ID, and other necessary metadata. - JSON Web Tokens (JWT): Use the
Microsoft.AspNetCore.Authentication.JwtBearer
NuGet package to implement JWT-based authentication. InStartup.cs
, configure the middleware with a valid audience, issuer, and signing key. Ensure to use a strong signing key and secure storage. - Securing Services and APIs: To secure different services and APIs, use the following approaches:
- Use Role-based Authorization and Policy-based Authorization as needed.
- Use the
[Authorize]
attribute to secure controllers and action methods. - Enforce HTTPS and enable HSTS.
- Take advantage of CORS to limit cross-origin requests.
- Validate incoming tokens using middleware and custom authentication handlers.
- Secure sensitive data using Data Protection APIs or encryption techniques.
Describe the critical aspects of handling errors and exceptions in a Nagarro C# project. How do you implement centralized exception handling and reporting to ensure consistent error responses across different applications?
Answer
Critical aspects of handling errors and exceptions in a Nagarro C# project include:
- Catching specific exceptions: Catch only the exceptions that you can handle, and let the others propagate up the call stack to be handled by a more appropriate catch block or global handler.
- Throwing meaningful custom exceptions: Create and throw custom exceptions that provide more context and detail about the issues encountered, making it easier to diagnose and resolve problems.
- Using try-catch-finally blocks judiciously: Ensure to use try-catch-finally blocks to handle exceptions and clean up resources. Avoid using catch blocks without any specific handling logic.
- Logging exceptions: Log exception details to identify and diagnose the issues quickly. Ensure to include the necessary information while logging, such as time, user, and system information.
- Graceful degradation: Design your application to degrade gracefully when an error occurs. Show a user-friendly error page or message instead of the generic error message or stack trace.
To implement centralized exception handling and reporting:
- Use exception filters: Implement custom exception filters by deriving from the
ExceptionFilterAttribute
class. In theOnException
method, you can handle exceptions, log them, and generate a consistent and meaningful error response.
Loading code snippet...
- Register the exception filter globally: In
Startup.cs
, register the custom exception filter globally to handle exceptions across all controllers and actions.
Loading code snippet...
By following these steps, you can implement centralized exception handling and reporting to ensure consistent error responses across different applications.
In a Nagarro C# project, how would you implement Continuous Deployment using Azure DevOps? What are the key aspects and components in the pipeline that need to be considered?
Answer
To implement Continuous Deployment using Azure DevOps in a Nagarro C# project, follow these steps:
- Create a new Azure DevOps project: Sign in to your Azure DevOps account and create a new project.
- Setup source control: Use Azure Repos or an external Git repository. Configure the build triggers, such as branch filters, and setup the required branches and policies.
- Create a build pipeline: Configure the build pipeline by selecting the appropriate project type (e.g., .NET Core for a C# project). Add tasks and configure them according to the project requirements, such as building the solution, running tests, and creating artifacts. Save and run the build pipeline to ensure it works correctly.
- Create a release pipeline: The release pipeline deploys the artifacts generated by the build pipeline to target environments. Configure the deployment stages and environments, such as development, staging, and production. In each stage, specify additional tasks for deploying the application, like executing scripts, deploying resources using ARM templates or Terraform, and setting up monitoring.
- Configure Environment approvers: Configure the required approvals for each environment, such as having the project manager approve the deployment to production.
- Configure Continuous Deployment: Enable the Continuous Deployment trigger in the release pipeline to trigger a deployment whenever new artifacts are created.
Key aspects and components in the pipeline to be considered:
- Build triggers: Determine appropriate build triggers to ensure your pipeline runs when needed, such as on a new pull request or push to a specific branch.
- Test coverage and quality: Include automated tests in the build pipeline to ensure code quality and minimize the risk of regressions.
- Deployment strategies: Choose a deployment strategy that meets your organization’s requirements, such as rolling deployments or blue-green deployments.
- Deploying to multiple environments: Configure your release pipeline to deploy the application to multiple environments, such as test, staging, and production.
- Configuration management: Manage configurations separately for each environment in the release pipeline.
- Monitoring and logging: Ensure that monitoring and logging are set up correctly in each environment, so potential issues are quickly identified and addressed.
What strategies would you implement to improve the performance and scalability of a Nagarro C# project? Discuss caching, parallelism, and code optimization techniques you would apply.
Answer
To improve the performance and scalability of a Nagarro C# project, employ the following strategies:
Caching: Implement caching to reduce the load on processing and data retrieval components. Possible caching techniques include:
- In-memory caching using
IMemoryCache
. - Distributed caching using Redis or other caching providers.
- Output caching for static resources.
- ASP.NET Core Response Caching Middleware.
Parallelism: Utilize parallelism to improve performance by executing tasks concurrently. Methods to implement parallelism include:
Parallel.ForEach
orParallel.For
.- Task Parallel Library (TPL) with
Task.Run
andTask.WhenAll
. - Async/await for asynchronous, non-blocking IO.
Code optimization: Optimize your code by identifying bottlenecks and making improvements such as:
- Using profiling tools to find performance issues.
- Reducing excessive object allocations.
- Optimizing object serialization/de-serialization.
- Minimizing blocking calls.
- Utilizing lazy loading for data retrieval.
Database optimizations: Optimize your database by:
- Using indexing and paging for queries.
- Denormalizing data, when applicable.
- Utilizing stored procedures.
- Optimizing Entity Framework performance with techniques such as eager/lazy loading,
As NoTracking()
, and batching queries.
Architectural Patterns: Apply architectural patterns to scale your application, such as microservices or CQRS (Command Query Responsibility Segregation), which separates read and write operations to improve scalability.
Load balancing: Implement load balancing using reverse proxies, Azure Load Balancer, or Azure Application Gateway to distribute incoming traffic across multiple servers effectively.
Auto-scaling: Employ auto-scaling solutions like Azure Auto-Scaling or Kubernetes to automatically adapt your infrastructure based on the current workload and demand, ensuring optimal resource utilization.
Optimizing front-end performance: Optimize your front-end performance by leveraging techniques like:
- Minifying and bundling JavaScript and CSS files.
- Employing efficient image compression.
- Profiling and optimizing rendering performance.
By applying these strategies, you can significantly improve the performance and scalability of a Nagarro C# project.
With numerous techniques for optimizing performance and scalability behind us, it’s time to turn our attention to some cutting-edge features and tools in C# development.
By stepping beyond the basics, you can further advance your mastery of Nagarro coding questions in C# and bring your project to the next level in terms of capabilities and functionality.
Explain the process of creating custom Attributes in C# and their usage in a Nagarro project. Provide an example scenario.
Answer
Creating custom attributes in C# involves the following steps:
- Derive from System.Attribute: Create a new class that inherits from
System.Attribute
.
Loading code snippet...
- Define optional properties: Add properties to the custom attribute to store additional information.
Loading code snippet...
- Add constructor parameters: Define a constructor for the attribute to provide values for the properties during initialization.
Loading code snippet...
- Apply the attribute: Apply the custom attribute to classes, methods, or properties as needed.
Loading code snippet...
- Read the attributes: Use reflection to read the attributes and perform any required operations based on the attribute properties.
Loading code snippet...
Example scenario:
Consider a Nagarro project where APIs must undergo a specific validation process. In such a case, you can create a custom attribute to denote the validation requirements for each API. While generating API documentation, the attributes can be read using reflection to describe the necessary validation details for each API in the documentation.
By following this process, you can create custom attributes in C# and apply them to a Nagarro project for various purposes, such as documentation, validation, or logging.
How would you implement a real-time communication between a C# backend and a front-end application in a Nagarro project? Discuss the approach and challenges.
Answer
To implement real-time communication between a C# backend and a front-end application in a Nagarro project, you can use SignalR, a library that simplifies adding real-time web functionality to your applications. Follow these steps to implement SignalR:
- Install packages: Add the
Microsoft.AspNetCore.SignalR
NuGet package to your ASP.NET Core project and the@microsoft/signalr
package to your front-end project using npm or yarn. - Create a SignalR Hub: In the C# backend, define a SignalR Hub by creating a class that inherits from
Hub
. This class defines the server-side methods that clients will invoke.
Loading code snippet...
- Configure SignalR: In
Startup.cs
, configure SignalR by adding it to the dependency injection container and defining a route for the hub.
Loading code snippet...
- Connect to the hub from the front-end: In your front-end application, establish a connection to the hub and invoke server-side methods or listen to events from the server.
Loading code snippet...
Challenges in implementing real-time communication:
- Scaling: Scaling real-time applications built with SignalR can be challenging, as each instance of server must share the same information with others. Implementing a backplane solution like Redis or Azure SignalR Service can help to scale out the application.
- Network limitations: Some clients might have network limitations, like firewalls or proxies, preventing them from connecting to the hub. SignalR automatically falls back to other supported transport protocols, such as Long Polling, to provide the best possible connection experience.
- Error handling and retries: Handling connection errors, reconnecting, and retrying failed operations requires proper and careful implementation. The new version of SignalR offers automatic reconnection, making this process smoother.
- Security: Ensuring proper authentication and authorization for your SignalR hub and controlling access to various messages and methods is crucial to prevent unauthorized access.
In Nagarro C#, how do you implement Validation using Data Annotations or FluentValidation? Explain their uses and advantages.
Answer
Data Annotations
To implement validation using Data Annotations, follow these steps:
- Add annotation attributes to model properties: Apply the appropriate validation annotation attributes to the properties in your model class. For example:
Loading code snippet...
- Validate the model: Inside your controller, use the
ModelState.IsValid
property to check if the validation has passed.
Loading code snippet...
Advantages of Data Annotations:
- Easy to use and widely supported by ASP.NET libraries.
- Integrates with model binding, so validation occurs automatically.
- Can be used for both server-side and client-side validation.
FluentValidation
To implement validation using FluentValidation, follow these steps:
- Install the FluentValidation package: Install the
FluentValidation.AspNetCore
NuGet package in your project. - Create a validator class: Create a new class derived from
AbstractValidator<T>
(whereT
is the model type) and define the validation rules using the provided fluent syntax.
Loading code snippet...
- Register the validator: In the
Startup.cs
, register the validator with the dependency injection container.
Loading code snippet...
- Validate the model: Inside your controller, use the
ModelState.IsValid
property to check if the FluentValidation rules have passed.
Loading code snippet...
Advantages of FluentValidation:
- Highly flexible and powerful with a rich set of built-in validators.
- Allows complex validation logic and conditional validation.
- Integrates easily with ASP.NET Core and can coexist with Data Annotations.
- Reusable validators can be shared across multiple types.
- Can be used for both server-side and client-side validation, with somewhat more setup required for the client side.
Both Data Annotations and FluentValidation serve the purpose of ensuring your data conforms to the desired structure and constraints. While Data Annotations are more straightforward and simple, FluentValidation provides more flexibility and power, allowing you to handle more complex validation scenarios. Choose the best option based on your project requirements and complexity.
How would you apply Aspect-Oriented Programming (AOP) concepts in a Nagarro C# project using PostSharp? Provide an example scenario and its benefits.
Answer
Aspect-Oriented Programming (AOP) helps separate cross-cutting concerns in your code, such as logging, caching, and error-handling, from the core business logic. PostSharp is a powerful AOP framework for .NET that enables you to implement AOP concepts in a Nagarro C# project. Here are the steps to apply AOP using PostSharp:
- Install PostSharp: Install the
PostSharp
NuGet package in your project. - Create an aspect class: Create a new class that inherits from one of PostSharp’s aspect base classes, such as
OnMethodBoundaryAspect
for method-level aspects orLocationInterceptionAspect
for property-level aspects.
Loading code snippet...
- Apply the aspect: Apply the aspect to the desired classes or methods using attributes.
Loading code snippet...
- Aspect configuration: Configure the aspect as needed, such as changing the behavior based on input parameters or controlling the order in which multiple aspects are applied.
Example scenario:
Consider a Nagarro C# project where you need to log the execution time of particular methods in your application. Instead of adding repetitive logging code to each method, you can use AOP with PostSharp to create a reusable PerformanceLoggingAspect
that automatically logs the execution time for the methods decorated with the attribute.
Loading code snippet...
Benefits of using AOP with PostSharp:
- Improved code maintainability: AOP helps you separate cross-cutting concerns from the main business logic, resulting in cleaner and more maintainable code.
- Code reusability: Aspects can be shared across projects, preventing code duplication.
- Easier to extend and modify: By isolating cross-cutting concerns in aspects, it becomes easier to extend or modify the behavior without affecting the core business logic.
What are the main differences between .NET Framework, .NET Core, and .NET 5 in the context of developing a Nagarro C# project? Discuss their advantages and disadvantages, as well as how they affect project development and deployment.
Answer
The main differences between .NET Framework, .NET Core, and .NET 5 in the context of developing a Nagarro C# project are:
.NET Framework: A mature, feature-rich, and extensive framework used for building applications on Windows platforms. It includes various libraries, languages, runtimes, and tools for developing a wide range of applications, such as web, desktop, mobile, and cloud-based applications. The .NET Framework has a large ecosystem and extensive community support. Advantages:
- Mature and feature-rich.
- Large ecosystem and extensive community support.
- Supports various application types, including desktop and web. Disadvantages:
- Windows-only platform.
- Not being actively developed; new features and improvements are focused on .NET Core and .NET 5.
.NET Core: An open-source, cross-platform runtime for developing modern, cloud-based, connected applications. It’s a modular and lightweight framework that can be deployed alongside your application, allowing for multiple versions to coexist on the same machine. Advantages:
- Cross-platform and support for containerization.
- Better performance and optimization.
- Flexible deployment options, including side-by-side deployment.
- Actively developed and maintained. Disadvantages:
- Some features and libraries from .NET Framework are not available or have limited support.
- Smaller ecosystem compared to .NET Framework.
.NET 5: The next evolution of .NET Core, .NET 5 is designed as a unified platform for developing applications with one runtime and framework. It combines the best of .NET Framework, .NET Core, and Xamarin, offering a single platform for any application. Advantages:
- Unified platform with a single runtime and framework.
- New features, performance improvements, and optimizations.
- Cross-platform and support for containerization.
- Actively developed and maintained. Disadvantages:
- Some features and libraries from .NET Framework are not available or have limited support.
- Relatively new, so the ecosystem is still growing.
In the context of a Nagarro C# project, the choice between .NET Framework, .NET Core, and .NET 5 depends on your requirements, existing infrastructure, and desired application architecture. .NET 5 is the recommended choice moving forward, as it offers the best of both the .NET Framework and .NET Core and is being actively developed and maintained. However, for legacy projects that depend on specific .NET Framework features or libraries, migrating to .NET Core or .NET 5 may require significant efforts and planning.
We hope this comprehensive guide to Nagarro C# interview questions and answers has provided valuable insights into the essential concepts and best practices of C# development. By understanding and applying the knowledge shared in this article, you’ll be well-prepared for your next Nagarro coding test in C# and any challenges you may face during your C# development journey.
Remember, continuous learning and fine-tuning your skills are key aspects of personal and professional growth as a software developer. Keep exploring, practicing, and refining your C# expertise to excel in your future C# projects and interviews!