Mastering Await in C#: From Zero to Hero

Welcome to this comprehensive guide on mastering the await
keyword in C#. By the end of this article, you’ll have a strong understanding of asynchronous programming and how to leverage the power of await
in your C# applications. Buckle up and let’s dive right in!
Introduction to Asynchronous Programming in C#
Before we dive into the await
keyword, it’s essential to understand the concept of asynchronous programming and its importance in modern software development.
What is Asynchronous Programming?
Asynchronous programming is a programming paradigm that allows the execution of multiple tasks concurrently without blocking the main thread. This enables your application to remain responsive, even when performing time-consuming operations, such as file I/O, network requests, or database queries.
Example
Imagine you are a chef in a kitchen. You have to cook multiple dishes at the same time. Asynchronous programming is like cooking different dishes side by side without waiting for one to finish before you start the next one.
This way, you can keep working on other dishes even if one takes a long time to cook. Just like the chef, your computer program can work on many tasks at the same time without waiting for one task to finish. This makes your program faster and able to do more things at once.
Why is Asynchronous Programming Important?
Asynchronous programming is vital for several reasons:
- It improves application responsiveness by allowing time-consuming tasks to run in the background.
- It can increase performance by making better use of available resources.
- It helps prevent common issues like deadlocks and race conditions.
With a clear understanding of asynchronous programming, let’s explore the await
keyword in C#.
Understanding Await in C#
The await
keyword is a core aspect of asynchronous programming in C#. Let’s break down its functionality, how it works, and when to use it.
The Await Keyword
The await
keyword is used to pause the execution of an async method until a specific task has completed. It allows your application to continue processing other tasks while waiting for the awaited task to finish.
How Does Await Work?
When you use await
in an async method, the compiler transforms your code into a state machine that manages the asynchronous operation’s lifecycle. This state machine keeps track of the method’s current state and automatically resumes execution when the awaited task completes.
When to Use Await
You should use await
whenever you need to perform a potentially time-consuming operation without blocking the main thread. Some common scenarios include:
- Reading or writing files
- Making network requests
- Querying databases
Now that we have a basic understanding of await
, let’s explore how to use it with the async
keyword.
Working with Async and Await in C#
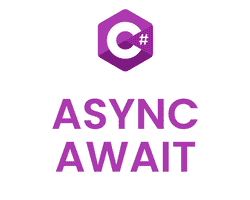
Async and Await in C#
May 10, 2023It’s crucial to understand how to create and call async methods using await
. We’ll also discuss handling exceptions and utilizing Task.Run
.
Creating Async Methods
To create an async method, you need to use the in the method signature and return a Task
or Task<T>
:
Loading code snippet...
Calling Async Methods with Await
To call an async method and wait for its result, use the await
keyword:
Loading code snippet...
Handling Exceptions with Async and Await
When using async and await, handle exceptions using the standard try-catch
block:
Loading code snippet...
Using Async and Await with Task.Run
To offload CPU-bound work to a separate thread, use Task.Run
with async and await:
Loading code snippet...
Now that we have a solid grasp on the basics, let’s dive into some advanced await usage techniques.
Advanced Await Usage in C#
In this section, we’ll delve deeper into advanced await usage techniques, such as ConfigureAwait
, ValueTask
, combining tasks, and using CancellationToken
with async and await.
Using ConfigureAwait
By default, an awaited task will resume on the original synchronization context (such as the UI thread). With ConfigureAwait
, you can control this behavior. This is particularly useful in library code, as it helps prevent potential deadlocks:
Loading code snippet...
Await with ValueTask
ValueTask
is a more efficient alternative to Task
for short-lived asynchronous operations. It can help reduce heap allocations, thereby improving performance. You can use await
with ValueTask
just like with Task
:
Loading code snippet...
Combining Tasks with Task.WhenAll and Task.WhenAny
Task.WhenAll
and Task.WhenAny
allow you to wait for multiple tasks to complete, enabling efficient parallelism:
Loading code snippet...
CancellationToken with Async and Await
Use CancellationToken
to cancel an ongoing async operation gracefully. This is especially useful in scenarios where the user may want to cancel time-consuming tasks:
Loading code snippet...
Using IAsyncEnumerable with Async and Await
IAsyncEnumerable
enables asynchronous iteration over a collection of items, allowing you to process items one at a time as they become available:
Loading code snippet...
With a robust understanding of advanced await usage, you’re now better equipped to handle more complex async scenarios in your C# applications.
Real-World Examples of Await in C#
In this section, we will explore real-world examples of await
in action, including file I/O, web requests, database operations, and UI responsiveness.
File I/O with Async and Await
Loading code snippet...
Web Requests with Async and Await
Loading code snippet...
Database Operations with Async and Await
Loading code snippet...
UI Responsiveness with Async and Await
Loading code snippet...
Finally, let’s discuss best practices when using await in C#.
Best Practices for Using Await in C#
To make the most of async and await in C# and ensure your code is efficient, maintainable, and reliable, follow these best practices:
Avoid Deadlocks
Deadlocks can occur when tasks wait for each other to finish and inadvertently block each other. To avoid deadlocks:
- Use
ConfigureAwait(false)
when appropriate, especially in library code. - Refrain from using
Task.Result
orTask.Wait()
—instead, useawait
to wait for tasks to complete.
Consistent Method Signatures
Maintain consistency in your method signatures by:
- Including the
async
keyword in async method declarations. - Returning
Task
for async methods with no return value orTask<T>
for methods returning a value of typeT
.
Proper Error Handling and Logging
Implement robust error handling and logging for asynchronous methods:
- Use
try-catch
blocks to catch exceptions thrown by awaited tasks. - Ensure proper logging of errors and exceptions to simplify debugging and maintenance.
Performance Considerations
Consider performance when choosing between Task
and ValueTask
, as well as when managing resources:
- Opt for
ValueTask
overTask
for short-lived operations to minimize heap allocations and improve performance. - Avoid unnecessary allocations or resource usage by carefully managing objects and data during asynchronous operations.
Conclusion
Congratulations! You’ve mastered the await
keyword in C# and are now equipped to create more responsive and scalable applications. Remember the key takeaways and best practices discussed in this article, and don’t forget to explore further resources to continue your learning journey. Happy coding!