Attributes in C#: All You Need to Know
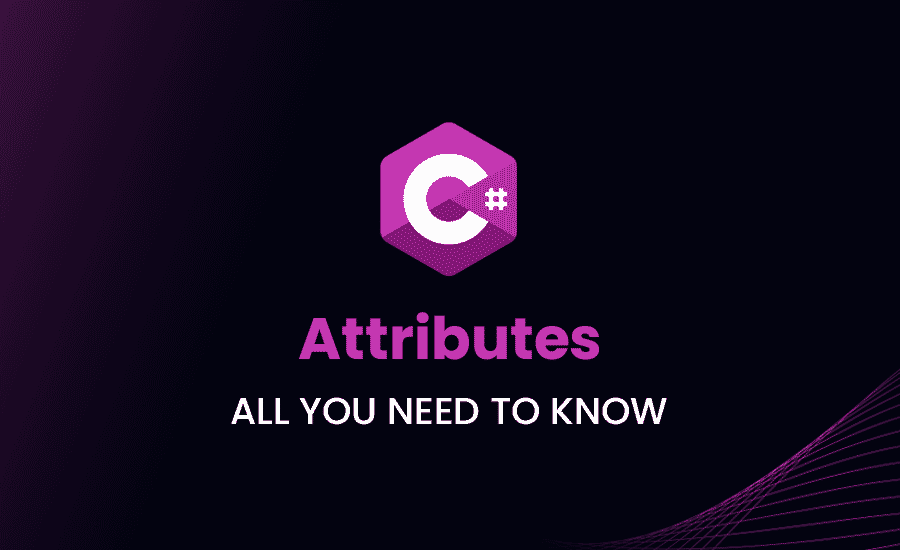
In this comprehensive yet straightforward guide, we will unravel the intriguing world of C# attributes. Yes, things are about to get a bit geeky, but worry not, we will break it down to bite-sized chunks that are easy to digest. In other words, there is enough coffee to make this nocturnal programming venture an enlightening experience.
Ready? Allons-y! (That’s Let’s go! in French. Sometimes we just got to sound cool, don’t we?)
Understanding C# Attributes
Before we dive in, wouldn’t it be helpful if we grasp the basics? Great! So sit tight, grab a warm cup of your favourite drink, and let’s lay a solid foundation on C# attributes.
Definition of C# Attributes
Attributes in C# are classes that provide metadata for your code.
Metadata? Yep, data about data. It is like the back story of your favourite superhero.
Loading code snippet...
In this small block of code, [Serializable]
is an attribute that gives some additional insights about MyClass
.
Purpose and Use of Attributes in C#
Why use attributes? Well, if you want to add extra identities, behaviors or features to elements in your code without changing their functionality, attributes are your best bet. It’s like adding a hat to a duck. It’s still a duck, just a cooler duck.
Elementary Attributes in C#
Having understood the basics, it’s time we move into some commonly used C# attributes. Promise, you won’t be left in the loop.
C# Deprecated and Obsolete Attribute
Imagine you have made some changes in your code and you don’t want others to use the old code. This is where you can use the Obsolete attribute to warn the users.
Loading code snippet...
So, anyone who tries to use OldMethod()
will get a warning. A pretty nifty way to redirect, isn’t it?
C# JSON Datetime Format Attribute
If you’ve worked with JSON in C#, you know it’s like trying to herd cats when it comes to formatting DateTime. But thanks to JSON datetime format attribute, managing it is as easy as pie.
With [JsonConverter(typeof(MyDateTimeConverter))]
, you can predefine the format you want.
C# Custom Attribute
Now, here comes the real code kung-fu part – creating Custom Attributes. Yes, you can create your custom attributes to use in your code. It is not rocket science, just follow along.
Creating and Implementing Custom Attributes in C#
Custom attributes are one of those features in C# that may seem advanced and intimidating at first, but they can become your new secret weapon once you understand how they work. They push the frontier of what’s possible with your code.
Creating and Implementing Personalized Custom Attributes
An easy-to-envision example is crossing off tasks from a to-do list. If you have a bunch of functions in your code that need testing, you can create a custom attribute, called IsTested
, to represent this status.
Loading code snippet...
Here, IsTested
has now become an attribute that you can stick onto any function to let everyone know it’s been tested.
Implementing a custom attribute is as stress-free as calling your best friend and saying “hey, I’m using you” (only, with code, it’s much more appreciated).
Loading code snippet...
Now your function MyFunction
is adorned with the IsTested
attribute. It stands out, and it communicates more clearly to anyone interacting with the code. It’s like giving your function its own unique fashion statement!
But wait, going a step further, what if you want to store more information in your attribute, like the date it was tested?
Loading code snippet...
Here, we’ve just added a property dateTested
that gets initialized when you add the IsTested
attribute to a function.
Let’s see it in action:
Loading code snippet...
This means that not only do we know the function has been tested, we also know it’s been tested on June 15, 2022. Pretty sweet, right?
Unleashing the Power of C# Enum Attributes
Enumerations, aka Enums, closely resemble superheroes in the world of programming. They group together constants, making our lives easier. And like every superhero, they become even better with C# attributes.
Our Enum attribute could look like this:
Loading code snippet...
However, we can’t tell why a user would be active or inactive. Bing, bing, bing! That sounds like a job for C# attributes!
Loading code snippet...
With the [Description]
attribute, we provide meaningful context about Active
and Inactive
states. Now that’s what I call crime fighting… I mean, code fighting, hero style!
Simplifying Life with C# Model Validation Attributes
Ensuring correct data in models is often as torturous as stepping on Lego bricks. Fortunately, C# model validation attributes are the shoes that protect our code from these pesky Lego bricks.
For instance, when we implement model classes to create forms, we normally have to fire off multiple lines of code to validate the form data. But with validation attributes, our lives become easier.
Behold the simplicity:
Loading code snippet...
In this example, the Name
field must contain at least 1 character but no more than 100. If these constraints are not met, the model is not valid, and thus, the form is not submitted.
The best part is, with just two lines of attributes, we have made our code more robust and avoided an potential nightmare of invalid data.
File Operations: C# Get File Attributes
Working with file attributes in C# doesn’t have to be tedious. C# provides a simple method: GetFileAttributes()
which does exactly what it sounds like.
Example of Using C# Get File Attributes
Below is an example of using GetFileAttributes()
method.
Loading code snippet...
With the above code, you pulled off the magician’s trick. You just fetched attributes of a file.
Checking for Attribute Existence in C#
Finally, before we wrap up, let’s explore how we can check if an attribute exists on an element. Why? Well, it’s like checking a list before going shopping. It’s just good practice.
C# Check If Attribute Exists: Coding Tips
Let’s say we want to check if our custom attribute IsTested
is present on MyFunction
.
Loading code snippet...
If IsTested
is defined on MyFunction
, isTestedPresent
will be true
.
And that’s it, folks. You’ve braved through the wonderful world of Attributes in C#. Remember, like any tool, the power of attributes depends on how skillfully you wield it. So keep coding, keep exploring, and keep adding feathers to your developer’s hat.
Wait, didn’t we agree on adding hats to ducks instead?