C# IDisposable Method: Full Guide
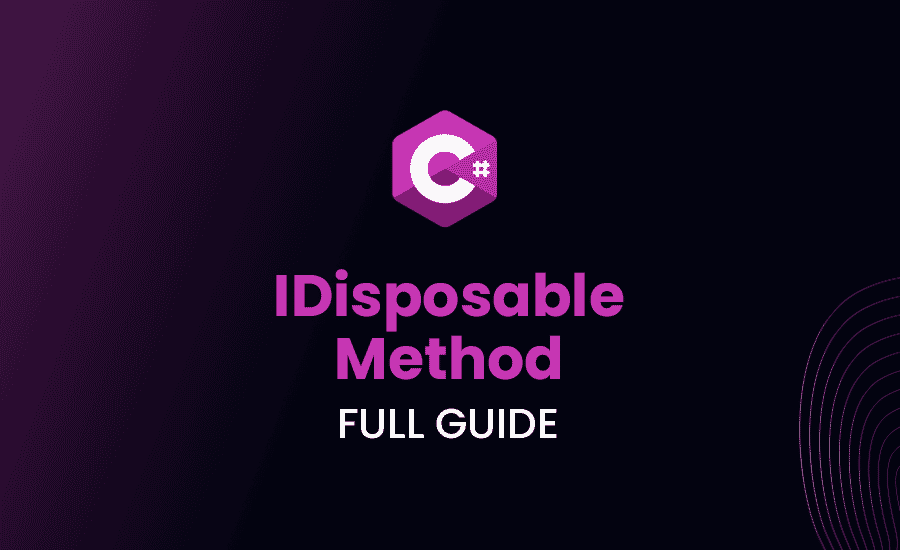
Unraveling the mysteries and complexities of the programming world is rarely a straightforward journey. Yet, with passion, patience and the right guidance, it can certainly be rewarding! Think about it – the joy you derive upon solving a complex problem using innovative programming solutions…
It’s incomparable, isn’t it? Now, imagine being able to handle system resources efficiently in your applications. And what if we told you this is possible using C# IDisposable? Intriguing, huh? Then buckle up as we embark on an enriching ride on the landscape of one of C#’s seamless interfaces – IDisposable!
Grasping the fundamentals of programming languages like C# can sometimes be like piecing together a jigsaw puzzle. It’s all about finding the right pieces – or, in our case, the right practices and principles. And one such principle is the use of the C# IDisposable, a hidden gem that can overhaul your resource management strategy.
IDisposable is like your program’s personal housekeeper, ensuring things are tidied up and kept in order, leaving more space for the core parts of your application to shine. Let’s delve deeper into this.
C# IDisposable Demystified
So, what is C# IDisposable? Essentially, IDisposable is an interface that helps manage system resources by disposing of them when they’re no longer needed. It makes certain there are no memory and resource leaks for your program to deal with long-term. It’s like your dedicated docent when you don’t need your used objects and resources.
Implementing IDisposable in your C# code is simple. It revolves around the Dispose() method – a unique method to clean up your resources.
Loading code snippet...
Here, FileHandler
is a class that has implemented IDisposable
. Why? To ensure that any resources (like file streams or handles) acquired by a FileHandler
object are properly released when the object has served its purpose.
This strategy becomes invaluable when dealing with a lot of resource-heavy operations. Without it, your application could start behaving like a snail with a heavy shell, barely making progress!
But don’t just take our word for it. Let’s explore a more hands-on example of IDisposable in action.
Code Example: Using IDisposable with Database Connections
Consider a scenario where you’re dealing with database connections. Unmanaged resources like these, if not handled properly, can cause performance issues or even crashes!
In such a situation, the IDisposable
interface and its Dispose ()
method acts as a superstar.
Loading code snippet...
What’s going on here? Quite simply, a DatabaseConnectionHandler
object opens a database connection using the provided connection string. Once the operations involving this connection have been completed, calling the Dispose
method closes the connection and frees up the allocated resources.
You can also use Dispose
in similar ways when dealing with file systems, image processing, and other areas involving heavy resource usage such as streams, handles, network connections, etc.
C# IDisposable Example: A Practical Overview
Let’s roll up our sleeves and dive into some practical aspects of C# IDisposable. Don’t worry, it’s more like playing with Lego blocks than rocket science!
Implement IDisposable C#
Imagine you’ve got a class, say TextFileWriter
, that’s responsible for writing text to files. The crux of this class is a StreamWriter
object that handles the actual file writing process. Just like a writer uses a pen to write, our class utilises this StreamWriter
to write text to files. Let’s take a look at how this class might look with IDisposable
implementation:
Loading code snippet...
In the TextFileWriter
class, we declare a private StreamWriter
object named _streamWriter
. In our WriteTextToFile(string text)
method, we initialize _streamWriter
and use it to write some text to a file. Simple enough, right?
Sure! But what happens when we’re done writing the text? Just like we put the cap back onto a pen after writing, we also need to properly close and dispose of our _streamWriter
when we’re finished with it. The Dispose()
method is our virtual pen cap! When called, it checks if _streamWriter
is null
, if not, it safely closes and disposes the StreamWriter
and sets _streamWriter
to null
.
Now let’s take a step further. Think about a scenario where you’d want to append text multiple times to the same file during the lifecycle of a TextFileWriter
object. How would you handle it?
Loading code snippet...
In this extended version of TextFileWriter
, we introduce SaveAndReopenStreamWriter()
. Using this method, we can safely close and reopen the _streamWriter
any time we like, all while ensuring no resources are leaked with proper disposal and re-initialization of _streamWriter
. This way, we can append text as many times as we want without having to worry about wasted resources or file locks.
Having an in-depth understanding of IDisposable Pattern is a must in your programmer arsenal. This pattern offers us a well-defined hook for cleaning up resources and ties in with .NET’s garbage collection process. Sounds intriguing? Wait until you see it in action!
Unlocking the Mystery of C# IDisposable Pattern: A Thorough Guide
Let’s begin with our DisposableClass
. It’s a class used as a blueprint for creating objects that hold unmanaged resources. In this class, we implement the standard dispose pattern of C#. This pattern is used whenever a class is supposed to manage resources that are not automatically managed by .NET’s garbage collector, like file handles or database connections.
Loading code snippet...
You notice how the Dispose(bool disposing)
method is where all the cleaning occurs, and it does so depending on the parameter passed. When disposing
equals true
, you clean the managed and unmanaged resources. If disposing
equals false
, you only clean the unmanaged resources.
You might wonder why? Well, when disposing
equals false
, it signifies that the method is being called by the Finalizer where the managed resources may have already been garbage collected. Interesting, right?
Let’s move forward to a more specific example, let’s say, a class that holds a database connection (unmanaged resource) and a list of data (managed resource).
Loading code snippet...
In this case, _dataList
represents managed resource that would normally be cleaned up by the garbage collector. However, you can and should clean up these resources in the Dispose(bool disposing)
method when disposing
equals true
. The SqlConnection _connection
represents an unmanaged resource that needs manual cleanup.
At this point, you might be thinking, “IDisposable and I are cool. We’re friends!”. You’ve got the basics, a hint of disposals, and clean-up logic behind IDisposable down pat. You even understand the essence of Dispose()
. But hey, in the world of C#, things never stop at “just enough”. To truly champion this game, we need to delve deeper and peel back the extra layers of complexity around IDisposable. So, are you ready to go beyond the surface and unravel the next level? Let’s make a deep dive into Windows internals!
An Insight into the Disposable Pattern C#
The world of C# programming is akin to a multifaceted gemstone, every turn uncovers new depth and richness. Disposable Pattern is one of these. Enticing, isn’t it?
Consider Disposable Pattern to be the friendly sibling of IDisposable – a two-faced component that ensures clean-ups are done accurately, whether you remember to call Dispose()
or not. One face is the Dispose Pattern we talked about earlier, and the other face? Enter the Finalizer!
In C#, Finalizers act as a final safety net, cleaning up if you ever forget to call Dispose(). Remember, though, only implement a Finalizer if your class directly uses unmanaged resources.
To get how this works, let’s unveil the magic with a code example:
Loading code snippet...
This code showcases a class ManagedWrapper
that uses both managed and unmanaged resources, represented by the AnotherIDisposable
and nativeResource
, correspondingly. When you’re done using an instance of ManagedWrapper
, call Dispose()
. Also, the Finalizer ensures that unmanaged resources are released if Dispose()
is not called.
Loading code snippet...
Here, we’ve utilized using
statement, which makes your Dispose()
calling much cleaner. As soon as the execution leaves the using
block, Dispose()
gets called – simple, clean, and efficient!
So, by exploring the deeper layers of IDisposable, you are essentially turbocharging your C# coding process. With Finalizers at your disposal, you can rest easy even if Dispose()
method slips your mind.
IDisposable C# Implementation
As we advance in this fascinating journey through the intricacies of IDisposable, we’re now ready to get our hands dirty with some practical stuff. Nervous? Don’t be! Even the best C# developers started somewhere.
C# IDisposable Implementation: Digging Deeper
Implementing IDisposable correctly isn’t just a fancy trick to impress your fellow devs, it can drastically improve the performance and robustness of your applications. There’s not an ounce of doubt that understanding when and how to use the Dispose method as well as the using
statement will pay off in the long run.
- Always Call Dispose(): This golden rule is critical to grasping the essence of IDisposable. The
Dispose()
method is like your tidy roommate, it cleans up after itself.
Let’s walk through an example. If you’ve ever worked with a StreamReader
to read data from a file, you know that the file remains locked until the StreamReader is closed. Want to modify or delete the file while it’s locked? Good luck with that!
Loading code snippet...
In this case, the using
statement automatically calls the Dispose()
method, ensuring the file gets unlocked, even if an exception occurs inside the using block.
- Implement Dispose Pattern: You might be thinking, “Hold on a second, haven’t we already covered this?” True, but as we delve deeper into the IDisposable rabbit hole, it’s essential to underline the impact of correctly implementing the Dispose Pattern.
The main idea behind implementing the Dispose Pattern lies in separating the cleanup logic for managed and unmanaged resources.
Loading code snippet...
In this Dispose Pattern, we can dispose managed resources when disposing
is true (typically when directly called by the developer), while unmanaged resources can be disposed whether disposing
is true or not (i.e., during finalization).
- Finalizers – The Last Line of Defense: Like a diligent superhero, Finalizers are there to save the day when all else fails. They are the final safety net ensuring your unmanaged resources are correctly disposed of if Dispose() was not called manually. The good part? .NET automatically calls the finalizer at some point after an object becomes eligible for finalization.
It’s important to remember that finalizers should be implemented judiciously as they come with performance overheads.
Loading code snippet...
- Embrace the statement: The
using
statement is a life-saver which calls theDispose()
method automatically. To clarify, anything insideusing
parentheses should implementIDisposable
. TheDispose()
method is then automatically called once the code inside theusing
block is completed.
Loading code snippet...
How to Implement IDisposable in C#
With programming, there are factors and concepts that should never be overlooked or underestimated. Take again the analogy of driving a car. If using IDisposable is akin to using power steering for a smoother drive, neglecting its implementation is like disregarding the importance of brakes.
And obviously, that’s a disaster waiting to happen! Would you like to ensure your C# programming adventure is smooth and far from disastrous? Then it’s crucial you understand how to implement IDisposable effectively.
Mastering the IDisposable Interface in C#
The initial steps towards understanding any concept involve a slight climb, IDisposable is no different. However, rest assured, once you conquer the initial gradient, the rest is a comfortable cruise along an open freeway.
Let’s revisit the DatabaseConnection
class that ideally uses the IDisposable for managing the database connection.
Loading code snippet...
In the DatabaseConnection
class, the SqlConnection
is opened during the object instantiation. This is your entry point into the world of data manipulation through queries with the help of the ExecuteQuery()
method.
However, you got to remember that while the door is open, your resources are engaged. And you wouldn’t want to exit leaving the door wide open, would you? This is where the Dispose()
method comes in. It exists solely to ensure that when you’re finished, your resources aren’t left open or hanging.
To illustrate this, here’s how you could use it in real programming:
Loading code snippet...
Just as you wouldn’t leave a room without switching the lights off, the using
statement ensures that Dispose()
automatically gets called when you’re finished with the DatabaseConnection
object, effectively closing the connection.
Let’s dive a bit deeper with another example. This time, consider a scenario where you need to work with multiple files simultaneously – opening each file, performing some operations (say reading data), and then safely closing it.
Loading code snippet...
In MultiFileReader
, multiple files are opened upon object instantiation and each file is read line. Once we are done reading, each StreamReader is disposed of safely, ensuring that we don’t end up with a memory leak or choked-up resources.
Traversing the IDisposable Interface .NET
After going through this wide spectrum, you must have realized how significant the role of IDisposable is. Manipulating unmanaged resources inside .NET can sometimes feel like navigating a maze, don’t you think? But, IDisposable just hands you the magical map!