C# Interview Questions and Answers
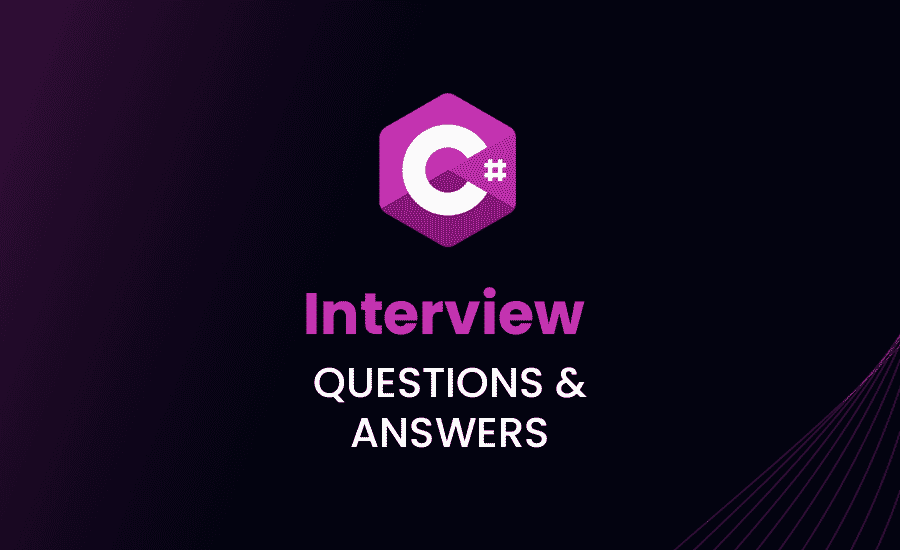
Welcome to the ultimate guide to C# Interview Questions and Answers! Whether you’re a fresh graduate or a seasoned developer, this comprehensive guide is designed to provide you with the knowledge and confidence needed to ace your next C# interview. With a total of 100 questions spanning easy, medium, and hard difficulties, this article covers a wide range of C# concepts and scenarios that you’re likely to encounter.
In this guide, we’ll be discussing:
- C# fundamentals
- Object-oriented programming (OOP) principles in C#
- Collections, data structures, and algorithms
- C# best practices and design patterns
- Working with APIs, libraries, and frameworks
- Real-world problem-solving using C#
So, sharpen your skills and be prepared for your next C# interview by diving deep into these Top 100 C# Interview Questions and Answers!
What does C# stand for?
Answer
C# (pronounced as “C-sharp”) is named after a musical notation, where the “sharp” symbol indicates that a note should be played one semitone higher. It is an analogy from the programming language C++, implying that C# is an enhanced and more advanced version of the C++ language.
Which company developed C#?
Answer
Microsoft is the company that developed the C# programming language.
What type of language is C#?
Answer
C# is a high-level, multi-paradigm programming language, which means it incorporates various programming paradigms such as procedural, object-oriented, and functional programming. It is a statically-typed and managed language, meaning that variable data types are checked at compile-time and C# uses a garbage collector to manage memory.
In which year was C# released?
Answer
C# was released in the year 2000.
Can you name the creator of the C# language?
Answer
Anders Hejlsberg is the creator of the C# language. He is a prominent software engineer from Denmark who has also contributed to the development of languages like Delphi and TypeScript.
What is the keyword for adding comments in C# code?
Answer
There are two types of comments in C#:
- Single-line comments: To create a single-line comment, use the double forward slashes (//) followed by your comment.
Loading code snippet...
- Multi-line comments: To create a multi-line comment, use the forward slash and asterisk(//) at the end of the comment.
Loading code snippet...
What type of loop does the ‘foreach’ statement create in C#?
Answer
The ‘foreach’ statement in C# creates a loop that iterates over a collection or an array. It allows you to work with each element in the collection or array without using an index variable.
Loading code snippet...
In this example, the foreach loop iterates through the “names” array and prints each name to the console.
Can you name a widely used C# Integrated Development Environment (IDE)?
Answer
Visual Studio is a widely used IDE for C# development. It is developed by Microsoft and provides numerous features and tools for efficiently developing, testing, and debugging C# programs. Some alternatives to Visual Studio include Visual Studio Code and JetBrains Rider.
What is the file extension for C# source code files?
Answer
C# source code files have the file extension “.cs”.
How do you declare a variable in C#?
Answer
To declare a variable in C#, you need to specify the datatype followed by the variable name and the assignment operator (=) if you want to initialize the variable with a value at the declaration. Here’s an example:
Loading code snippet...
In this example, we declare and initialize three variables with different data types: int (integer), string (text), and bool (boolean).
What is the syntax for defining a class in C#?
Answer
A class can be defined in C# using the class
keyword followed by the class name and a pair of curly braces that enclose the class definition. Here’s the general syntax for defining a class in C#:
Loading code snippet...
For example, to create a simple Person
class, the following syntax can be used:
Loading code snippet...
How do you instantiate an object of a class in C#?
Answer
To instantiate an object of a class in C#, use the new
keyword followed by the class name and a pair of parentheses for invoking the constructor. The general syntax for creating an object is:
Loading code snippet...
For example, to create an object of the Person
class:
Loading code snippet...
What is the print() method used for in C#?
Answer
There is no print()
method in C#. Instead, we use the Console.WriteLine()
or Console.Write()
methods to output text to the console window.
Console.WriteLine()
: Writes the specified text or variable value followed by a new line.Console.Write()
: Writes the specified text or variable value without appending a new line.
Example:
Loading code snippet...
What is the purpose of the Main() method in C#?
Answer
The Main()
method in C# serves as the entry point of the application. When an application starts executing, the Main()
method is the first method that gets called. It typically contains the code that starts the application and initializes its behavior.
The Main()
method can be defined with different signatures, including:
Loading code snippet...
- The method must be
static
and have a return type of eithervoid
orint
. - The optional
string[] args
parameter is used for passing command-line arguments to the application.
Example of a basic Main()
method:
Loading code snippet...
How do you create a single-line comment in C#?
Answer
To create a single-line comment in C#, use the double forward-slash //
. The text following the double forward-slash on the same line will be treated as a comment and ignored by the compiler.
Example:
Loading code snippet...
Which keyword is used to create a function in C#?
Answer
In C#, the keyword void
or a data type (like int
, float
, string
, etc.) is used to create a function. A function is a named block of code that performs a specific task and can return a value.
Here is the general syntax for declaring a function in C#:
Loading code snippet...
access_modifier
: Optional. Determines the visibility of the function (e.g.,public
,private
,protected
,internal
).return_type
: The data type of the value that the function returns. Usevoid
if the function doesn’t return any value.FunctionName
: The name of the function.parameters
: Optional. A list of input values (arguments) passed to the function.
Example:
Loading code snippet...
How do you create an array in C#?
Answer
In C#, arrays are created using the data type followed by square brackets []
. To create an array, you need to specify its data type, size, and (optionally) its elements during initialization.
There are several ways to create an array in C#:
- Declare an array and then initialize its elements:
Loading code snippet...
- Directly initialize an array with elements:
Loading code snippet...
- A shorter syntax for initializing an array with elements:
Loading code snippet...
How do you initialize the value of a variable?
Answer
To initialize the value of a variable in C#, first declare it using the data type followed by the variable name. Then, assign a value using the assignment operator =
.
Here’s the general syntax for initializing a variable:
Loading code snippet...
Examples:
Loading code snippet...
What is the base class for all C# classes?
Answer
The base class for all C# classes is the System.Object
class, which is also referred to as object
. Every class in C#, either directly or indirectly, inherits from the object
class. When you create a new class, it implicitly inherits from object
if no other base class is specified.
Example:
Loading code snippet...
In this example, the Person
class implicitly inherits from the object
class.
What is the format specifier for an Integer in C#?
Answer
The format specifier for an integer in C# is {index_number:D}
or {index_number:Dn}
where D
represents the decimal format and n
represents the minimum size of the integer field if you want to add leading zeroes.
The index_number
indicates the position of the argument to be formatted in the list of arguments provided. To use format specifiers, include them inside the string to be formatted and pass the integer(s) to the string.Format()
method or inside the interpolation brackets in an interpolated string.
Examples:
Loading code snippet...
Congratulations on successfully tackling the first set of easy C# interview questions! You’re now ready to advance to the next round, which consists of medium-easy questions. These questions delve a bit deeper into the concepts of object-oriented programming, exception handling, and basic data structures in C#. Remember, practice makes perfect, so keep honing your skills with these questions and reinforce your understanding of C#.
What is a namespace in C#?
Answer
A namespace in C# is a way to group related types and improve the organization of your code. It is used to avoid naming conflicts between different classes or types that may have the same identifier. Namespaces work as a hierarchy, where you can have nested namespaces, and they can span multiple files or even libraries.
For example:
Loading code snippet...
In this example, the MyClass
class is declared inside the Company.Project
namespace.
What is the purpose of the ‘using’ directive in C#?
Answer
The using
directive in C# has two primary purposes:
- Namespace importing:
using
allows you to import a namespace so that you can use the types and members defined in that namespace without specifying their fully qualified name. It makes your code more readable and reduces code complexity. For example:
Loading code snippet...
- Resource management: The
using
statement is used to help you manage resources like file streams, network connections, or database connections that implement the IDisposable interface. Theusing
statement ensures that theDispose()
method is called on the resource when the block of code is exited, releasing the resources properly. For example:
Loading code snippet...
What are reference types in C#?
Answer
Reference types in C# are types that store a reference to the memory location where the data is stored, rather than the actual data itself. When you create objects from classes or use arrays, delegates, interfaces, or most built-in data structures from the .NET Framework, you are working with reference types. Unlike value types, reference types are allocated on the heap, and the garbage collector manages their memory.
Key aspects of reference types:
- When you pass a reference type as a method parameter or assign it to another variable, both variables will refer to the same memory location. Thus, modifications in one variable will affect the other one.
- Reference types can have a
null
value, which means they don’t reference any memory location or object.
Loading code snippet...
How do you declare a method in C#?
Answer
A method in C# is a block of code that performs a specific task and can be called as many times as needed. To declare a method, you need to specify its access level, return type, method name, and a parameter list (if applicable) within the class. Here’s an example:
Loading code snippet...
In this example, we declare a method named Add
with the public access modifier, an int return type, and two parameters of type int. The method calculates the sum of the two numbers and returns the result.
What are the basic access modifiers available in C#?
Answer
Access modifiers in C# control the visibility and accessibility of class members (methods, properties, fields, etc.) and classes themselves. The basic access modifiers available in C# are:
- public: Members and classes with the
public
access modifier are accessible from any code inside the same assembly or another assembly that references it. - private: Members declared as
private
can only be accessed within the same class. They are not visible to other classes, even within the same assembly. By default, class members areprivate
if no access modifier is specified. - protected: Members declared with the
protected
access modifier can be accessed within the same class, as well as within derived classes. They are not visible to other non-derived classes in the same assembly. - internal: Members and classes with the
internal
access modifier can be accessed anywhere within the same assembly but are not visible to other assemblies. - protected internal: Members declared with the
protected internal
access modifier are accessible within the same assembly and by derived classes in another assembly.
Loading code snippet...
What is a constructor in C#?
Answer
A constructor is a special method in a class that is called when an object of that class is created. Constructors have the same name as the class and don’t have an explicit return type. Constructors can be overloaded, which means you can have multiple constructors within a class with different parameter lists.
Constructors are used to:
- Initialize the object’s state (set default values for properties, fields, etc.).
- Allocate resources, such as memory or network connections.
- Verify input parameters or ensure that the object is in a consistent state.
Example:
Loading code snippet...
What are indexers in C#?
Answer
Indexers in C# are class members that allow you to access an object’s elements using index notation, similar to how you access elements in an array or a collection. Indexers provide a more intuitive way to interact with the elements stored in a class, especially when it contains a collection or an array.
To define an indexer, you need to use the this
keyword, the type of the elements you want to access, and implement the get
and set
accessors to access and modify the underlying elements.
Here’s an example of an indexer implementation for a simple custom collection class:
Loading code snippet...
How do you create custom exception handling in C#?
Answer
To create custom exception handling in C#, you can create a custom exception class that inherits from the built-in System.Exception
class or one of its derived classes. Custom exceptions are useful when the built-in exception classes don’t cover specific error situations in your application.
Here’s an example of a custom exception class:
Loading code snippet...
Now you can throw and catch the CustomException
in your code:
Loading code snippet...
How can you prevent class inheritance in C#?
Answer
To prevent class inheritance in C#, you can use the sealed
keyword when declaring the class. A sealed
class cannot be inherited by any other class, and it is a compile-time error to try to inherit from a sealed class.
Loading code snippet...
What is the difference between ‘const’ and ‘readonly’ in C#?
Answer
const
and readonly
are both used in C# to create variables that cannot be changed after they are assigned a value, but they have some differences:
const
:- Can only be applied to fields, not properties.
- Must be assigned a value at declaration.
- The value assigned must be a constant expression that can be resolved at compile-time.
const
fields are implicitly static, and you cannot use instance members to initialize them.
Loading code snippet...
readonly
:- Can be applied to fields and properties.
- Can be assigned a value at declaration or inside a constructor.
- The value assigned can be a constant expression or a non-constant expression that is resolved at runtime.
readonly
fields can be either static or instance members, depending on your needs.
Loading code snippet...
Summary of differences:
const
is a compile-time constant, and its value cannot depend on runtime conditions.readonly
is a runtime constant, and its value can be determined at runtime.const
fields are implicitly static, whilereadonly
fields can be either static or instance members.
What is garbage collection in C#?
Answer
Garbage collection (GC) in C# is an automatic memory management system provided by the .NET Framework. It is responsible for freeing up memory that is no longer being used by the application. The garbage collector keeps track of objects allocated on the heap, determines which objects are no longer accessible, and reclaims the corresponding memory space.
The main benefits of garbage collection are:
- Improved developer productivity by reducing boilerplate code for manual memory management.
- Prevention of memory leaks, as unused memory gets automatically reclaimed.
- Protection against certain programming bugs, like dangling pointers or double-free issues.
C#’s garbage collection is non-deterministic, meaning that you cannot predict when exactly the garbage collector will run. It uses a generational approach, classifying objects into generations to reduce the time and resources spent on garbage collection. The garbage collector will typically run when available memory is getting low or when the system is idle.
While garbage collection can bring benefits, care should still be taken when dealing with heavy resource-consuming objects, like file handlers or database connections. Classes that deal with such resource management should implement the IDisposable
interface to allow deterministic resource release.
Describe object-oriented programming (OOP) in C#. Name the main OOP concepts.
Answer
Object-Oriented Programming (OOP) is a programming paradigm that represents concepts as objects with attributes (fields or properties) and behaviors (methods). The goal of OOP is to produce reusable, maintainable, and modular code by emphasizing the separation of concerns and adhering to OOP principles.
C# is an object-oriented programming language, and it fully supports OOP concepts. The main OOP concepts in C# are:
- Encapsulation: The process of bundling data (attributes) and methods (functions) that operate on the data into a single unit (class). Encapsulation helps to protect the internal state of an object and control access to its attributes.
- Inheritance: The ability of a class to inherit properties, methods, and behaviors from another class (
base
class) to enable code reuse and represent a hierarchy of related objects. In C#, a class can only inherit from a single class but can implement multiple interfaces. - Polymorphism: Polymorphism is the ability of a single interface to represent different types or the ability of a method to have multiple implementations based on derived types. In C#, polymorphism can be achieved through overloading (using the same method name with different parameters in the same type) and overriding (redefining a base class method in a derived class).
- Abstraction: Abstraction is the process of simplifying complex real-world objects and concepts by focusing on their essential features. Abstraction can be achieved in C# by using abstract classes and interfaces to define the common properties and methods that a group of related types must implement.
How can you create a generic class or method in C#?
Answer
A generic class or method in C# is a class or method that can work with any type without the need for explicit type casting or type checking at runtime. Generic types increase the reusability, flexibility, and type safety of your code.
To create a generic class or method in C#, you need to use the angle brackets <T>
to define a type parameter. Here’s an example of a generic class and a generic method:
Loading code snippet...
In this example, T
is the type parameter for both the generic class GenericList<T>
and the generic method Max<T>(T, T)
. The method also uses a constraint (where T : IComparable<T>
) to ensure that the type parameter T
implements the IComparable<T>
interface.
What is the difference between an abstract class and an interface in C#?
Answer
Both abstract classes and interfaces are used to define abstract entities in C#, but they have some differences:
Abstract Class:
- Can have both abstract and non-abstract (implemented) methods.
- Can have fields (variables), properties, and events.
- Supports constructors and destructors.
- Can have access modifiers for members (public, protected, internal, etc.).
- Supports inheritance; a class can inherit from only one abstract class.
- Can have complete implementations for some methods or properties, but cannot be instantiated directly.
Interface:
- Can only have method, property, and event signatures (no implementation).
- Cannot have fields or constructors.
- No member (methods, properties, events) can have an access modifier (all are implicitly public).
- Supports multiple inheritance; a class can implement multiple interfaces.
- Cannot have any implementation details, just the signatures of the members.
In summary, abstract classes provide a base implementation that can be shared among multiple derived classes, while interfaces define a contract that any implementing class must adhere to. Use an abstract class when you want to provide some default behavior and an interface when you need multiple inheritance or want to strictly define a common functionality contract.
What is the difference between ‘out’ and ‘ref’ parameters in C#?
Answer
Both out
and ref
parameters in C# are used to pass arguments by reference, allowing a method to modify the values of the passed variables outside the method. However, there are some differences between the two:
ref:
- The variable passed as a
ref
parameter must be initialized before passing it to the method. - The method called doesn’t have to assign a value to the
ref
parameter, as it already has a value. Example:
Loading code snippet...
out:
- The variable passed as an
out
parameter doesn’t need to be initialized before passing it to the method. - The method called must assign a value to the
out
parameter before the method returns. Example:
Loading code snippet...
In summary, use ref
parameters when you want the called method to modify an already initialized variable, and use out
parameters when you want the called method to provide a value that doesn’t depend on the initial value of the passed variable.
Describe the concept of delegates in C#.
Answer
A delegate in C# is a reference type that represents a method with a specific signature. Delegates allow you to treat methods as objects and pass them as arguments, return them from methods, or store them as properties. Delegates provide a way to create function pointers or callbacks, making it possible to develop more flexible and extensible applications.
Delegates are particularly useful when designing event-driven systems, where components must react to other component events or signals without tight coupling. Here’s an example of a delegate declaration, instantiation, and invocation:
Loading code snippet...
C# also supports generic delegates, multicast delegates, and built-in Func<T, TResult>
and Action<T>
delegates (introduced in .NET Framework 3.5) for increased flexibility and usability.
What is the difference between the ‘==’, ‘Equals’, and ‘ReferenceEquals’ methods?
Answer
In C#, ‘==’, ‘Equals’, and ‘ReferenceEquals’ are used to compare objects or values for equality, but with some differences:
- == operator: The ‘==’ operator compares two objects or values for equality. For value types, it checks if the values are equal. For reference types, it checks if the object references are equal, which means they’re pointing to the same object in memory. This behavior can be overridden for custom reference types by overloading the ‘==’ operator.
Loading code snippet...
- Equals: The ‘Equals’ method compares two objects for equality by checking their values, not their references. The default implementation of ‘Equals’ for reference types checks reference equality, but it can be overridden in your custom class to provide value-based comparison.
Loading code snippet...
- ReferenceEquals: The ‘ReferenceEquals’ method checks if two object references are equal, meaning they point to the same object in memory. It does not compare values. This method cannot be overridden and is useful when you need to perform a strict reference comparison.
Loading code snippet...
In summary, use the ‘==’ operator for simple value comparisons and reference comparisons, ‘Equals’ when you need to perform a value-based comparison for reference types, and ‘ReferenceEquals’ when you want to specifically compare object references.
Explain the concept of dependency injection in C#.
Answer
Dependency Injection (DI) is a design pattern used in C# and other object-oriented languages that helps to separate the creation and management of object dependencies, increasing the modularity, maintainability, and testability of an application.
In the context of C#, dependency injection is a technique in which an object receives its dependencies (objects, services, or values it relies on) from an external source instead of directly creating, managing, or looking up these dependencies. Dependency injection can be achieved using constructor injection, property injection, or method injection:
- Constructor Injection: The dependencies are passed to the object through its constructor. This is the most common and recommended method of dependency injection because it enforces that the object is created with all required dependencies and in a valid state.
Loading code snippet...
- Property Injection: The dependencies are set through public properties or setters of an object. This technique is used when the dependency is optional or can be changed during object lifetime.
Loading code snippet...
- Method Injection: The dependencies are passed to an object through a method. This technique is suitable when an object requires a specific dependency for just one method and not for the entire lifecycle of the object.
Loading code snippet...
In C#, popular dependency injection frameworks like Autofac, Unity, or built-in .NET Core Dependency Injection can be used to manage and resolve object dependencies.
How does C# support multiple inheritances?
Answer
C# does not directly support multiple inheritance for classes. A class in C# can inherit from only one base class. However, C# allows multiple inheritance through interfaces, as a class can implement multiple interfaces.
When a class implements multiple interfaces, it essentially inherits the behavior of each interface, and each interface can be considered as a contract that the class must adhere to. This approach to multiple inheritance enables flexibility while avoiding the complexity and ambiguity that can arise from multiple class inheritance.
Example:
Loading code snippet...
In this example, Car
implements the IEngine
and IDriver
interfaces to provide multiple inheritance-like functionality.
What is method overloading in C#?
Answer
Method overloading is a feature in C# that allows a class to have multiple methods with the same name, but different parameter lists. The correct method is called at compile time based on the arguments provided. Method overloading is a way to achieve compile-time polymorphism. The return type of the methods doesn’t factor into overloading. The method signature, i.e., the method name and parameter list, must be unique.
Here’s an example:
Loading code snippet...
In the example above, we’ve overloaded the Add
method with different parameter lists.
Great job completing the medium-easy questions! As you’ve noticed, the complexity has gradually increased, and you’ve now reached the medium-level questions. In this section, we’ll be focusing on more advanced features of C#, such as generics, delegates, events, and design patterns. Don’t worry if some of these topics are unfamiliar; this guide is here to help you expand your knowledge and master these essential C# concepts.
How do you create a property in C#?
Answer
In C#, properties are members of a class that provide a flexible mechanism to read, write, or compute the value of private fields. Properties can be created using get
and set
accessors in the property definition. A property can have a get
accessor, a set
accessor, or both, depending on whether you want the property to be read-only, write-only, or read-write.
Here’s an example of a property with both get
and set
accessors in C#:
Loading code snippet...
In C# 3.0 and later, you can use auto-implemented properties, which enables you to create properties without having to define the backing field explicitly:
Loading code snippet...
What is the purpose of the ‘params’ keyword in C#?
Answer
The params
keyword in C# allows a method to accept a variable number of arguments of a specified type. Using the params
keyword, you can pass an array of values or separate comma-separated values to the method. Inside the method, the params
parameter is treated like an array.
Here’s an example of using the params
keyword:
Loading code snippet...
You can call the Sum
method with any number of arguments, like this:
Loading code snippet...
What are the characteristics of a static class in C#?
Answer
In C#, a static class is a class that cannot be instantiated or inherited. It can only have static members, and it cannot have instance members (like instance properties, methods, or fields). The main characteristics of a static class are:
- It is declared with the
static
keyword. - It cannot have a constructor (except the static constructor).
- It cannot be instantiated or inherited.
- All members of a static class must also be static.
- It can be accessed directly using the class name, without creating an instance.
A common use of static classes is for utility functions and extension methods. Here’s an example of a static class in C#:
Loading code snippet...
To use this static class, you simply call its methods like this:
Loading code snippet...
How do you pass data between two forms in C#?
Answer
In C#, there are several ways to pass data between two forms in a Windows Forms application. One common approach is to use public properties or methods to expose the data that needs to be passed. Here’s an example:
- Create a second form with a public property that will hold the data to be passed.
Loading code snippet...
- In the first form, instantiate the second form and set the
Data
property with the data you want to pass.
Loading code snippet...
In this example, when the user clicks the OpenSecondFormButton
, the second form opens, and the Data
property of the second form contains the data passed from the first form.
What is the difference between a class and an object in C#?
Answer
In C#, a class is a blueprint for creating objects. It defines the structure, properties, and methods that objects of that class will have. Classes are reference types, and they can inherit from other classes to extend or override functionality.
An object, on the other hand, is an instance of a class. It is created from the class definition and occupies memory when instantiated. Objects are instances of classes, and they contain the data (fields) and behaviors (methods) that are defined in the class.
For example, consider a Car
class:
Loading code snippet...
In this example, Car
is the class, and it defines the structure and behavior of car objects. To create objects (instances) of the class, you use the new
keyword, like this:
Loading code snippet...
In this example, car1
and car2
are objects (instances) of the Car
class, and they have their own individual sets of properties and methods.
What is a delegate in C#?
Answer
A delegate in C# is a type that defines a method signature and can hold a reference to a method with a matching signature. Delegates are similar to function pointers in C++, but they are type-safe and secure. They are commonly used for implementing events and callbacks.
Delegates can be used to pass methods as arguments, assign them to class properties, or store them in collections. Once a delegate is defined, it can be used to create delegate instances that point to methods with the same signature.
Here’s an example of a delegate in C#:
Loading code snippet...
In this example, MyDelegate
is a delegate that defines the method signature for methods that take a string
argument and return void
. The Main
method creates a delegate instance, assigns the MyClass.DisplayMessage
method to it, and invokes the method through the delegate.
How do you implement polymorphism in C#?
Answer
Polymorphism is one of the fundamental principles of object-oriented programming. It allows objects of different classes to be treated as objects of a common superclass. In C#, there are two types of polymorphism: compile-time (method overloading) and runtime (method overriding and interfaces).
Method overriding and interfaces are the common ways to implement runtime polymorphism. Here’s an example using method overriding:
Loading code snippet...
In this example, we define a base class Animal
and a derived class Dog
. The Speak
method in the Animal
class is marked as virtual
, allowing its behavior to be overridden by derived classes. The Dog
class overrides the Speak
method with its own implementation. When we create a Dog
object and assign it to an Animal
variable, the overridden method in the Dog
class is called at runtime, exhibiting polymorphism.
To implement polymorphism via interfaces, you can define an interface and then have classes implement the interface methods. For example:
Loading code snippet...
In this example, we define an ISpeak
interface with a Speak
method. The Dog
and Cat
classes implement this interface and provide their own implementation of the Speak
method. We can create and assign objects of these classes to an ISpeak
variable, and the appropriate implementation of the Speak
method is called at runtime, demonstrating polymorphism.
What is a struct in C#?
Answer
A struct (short for “structure”) in C# is a value type that can contain fields, properties, methods, and other members like a class. However, unlike classes, which are reference types, structs are value types and are stored on the stack. Structs do not support inheritance (except for implicitly inheriting from System.ValueType), and they cannot be used as a base for other classes or structs.
Structs are useful for representing small, lightweight objects that have a small memory footprint and do not require garbage collection overhead. They are commonly used to represent simple data structures, such as points in a 2D space, dates, time intervals, and colors.
Here’s an example of a struct in C#:
Loading code snippet...
In this example, we define a Point
struct with properties X
and Y
, a constructor, and a method DistanceToOrigin
. We can create Point
instances and use their methods like we would with class instances.
What is the difference between ‘throw’ and ‘throw ex’ in exception handling?
Answer
In C#, when handling exceptions, it’s important to understand the difference between throw
and throw ex
:
throw
: When you catch an exception and want to rethrow the original exception, you use thethrow
statement without specifying any exception object. This preserves the original exception’s stack trace and allows the upstream exception handler to access the full stack trace.throw ex
: When you catch an exception and want to throw a new or modified exception object, you use thethrow ex
statement, whereex
is an instance of an exception. This replaces the original exception’s stack trace, and the upstream exception handler will only see the stack trace starting from the point wherethrow ex
was called.
Here’s an example:
Loading code snippet...
In Method1
, we catch an exception and rethrow it using throw
. The upstream exception handler can access the original stack trace of the exception. In Method2
, we catch an exception and throw a new ApplicationException
with the original exception as its inner exception using throw ex
. The upstream exception handler will see the stack trace starting from the point where throw ex
was called.
What is a nullable type and how is it used in C#?
Answer
Nullable types in C# are value types that can have a ‘null’ value. By default, value types cannot have a ‘null’ value, as they have a default value (like 0 for int, false for bool, etc.). To create a nullable type, you can use the built-in Nullable<T>
structure or the syntactic sugar ?
modifier.
Nullable types are useful when you need to indicate the absence of a value or when working with data sources (like databases) that might contain missing values.
Creating nullable types:
Loading code snippet...
Nullable types can be checked for having a value using the HasValue
property and retrieved using the Value
property. Alternatively, you can use the GetValueOrDefault
method to get the value if it exists or a default value:
Loading code snippet...
C# also supports nullable value type conversions and null coalescing using the ??
operator to provide a default value when a nullable type is ‘null’:
Loading code snippet...
Describe the concept of LINQ in C#.
Answer
Language Integrated Query (LINQ) is a feature introduced in C# 3.0 (.NET Framework 3.5) that provides a set of query operators and a unified query syntax for querying, filtering, and transforming data from various types of data sources like collections, XML, databases, or even custom sources.
LINQ allows you to write type-safe, powerful, and expressive queries directly in C# with the benefits of full support from the compiler, static typing, and IntelliSense.
LINQ offers two types of syntax for writing queries:
- Query Syntax: This is a more SQL-like, declarative syntax that provides a high level of abstraction and readability. Query syntax requires the
from
keyword (retrieves the data),select
keyword (specifies the output), and optional keywords (such aswhere
,group
, ororderby
).
Loading code snippet...
- Method Syntax: This is based on LINQ extension methods of the
System.Linq.Enumerable
class and uses lambda expressions to manipulate the data. Method syntax provides a more functional approach but may be less readable if the query is complex.
Loading code snippet...
LINQ supports a variety of providers like LINQ to Objects (for in-memory collections), LINQ to XML (for XML documents), and LINQ to Entities (for entity framework), enabling developers to work with different data sources using a similar query syntax.
What is a lambda expression in C#?
Answer
A lambda expression in C# is an anonymous function that is used to create inline delegates, expression trees, and expressions for LINQ queries or other functional programming constructs. Lambda expressions rely on the =>
operator (called the lambda operator) to separate the input parameters and the expression or statement block.
Lambda expressions can be used for both expression and statement lambdas:
- Expression Lambda: This consists of an input parameter list, followed by the lambda operator, and a single expression. An expression lambda automatically returns the result of the expression without the need for an explicit
return
statement.
Loading code snippet...
- Statement Lambda: This is similar to an expression lambda but contains a statement block enclosed in braces
{}
instead of a single expression. A statement lambda allows for multiple statements and requires an explicitreturn
statement if a value must be returned.
Loading code snippet...
Lambda expressions are a concise and efficient way of representing delegates or expressions, and are commonly used with LINQ queries, event handlers, or higher-order functions that expect a function as an argument.
Describe the async and await keywords in C#. How can they be used together?
Answer
In C#, the async
and await
keywords are used to write asynchronous code that can run concurrently without blocking the main execution thread. This allows for more efficient and responsive applications, particularly in cases of I/O-bound operations (e.g., calling web services, reading files, or working with databases) or CPU-bound operations that can be parallelized.
- async: The
async
keyword is added to a method to indicate that it’s an asynchronous method. An async method usually returns aTask
for void-returning methods orTask<TResult>
for methods that return a value of type TResult. An async method can contain one or moreawait
expressions.
Loading code snippet...
- await: The
await
keyword is used to pause the execution of an async method until an awaited task completes. It asynchronously waits for the task to complete and returns the result (if any) without blocking the main execution thread. Theawait
keyword can only be used inside an async method.
Loading code snippet...
When using async
and await
together, ensure that you propagate the asynchronous behavior up the call stack by marking every calling method with the async
keyword and awaiting async methods in the method body. This “async all the way” approach ensures that the benefits of asynchronous programming are fully utilized throughout the application.
What is the Task Parallel Library (TPL) and how does it relate to C#?
Answer
The Task Parallel Library (TPL) is a set of APIs introduced in the .NET Framework 4.0 to simplify parallelism, concurrency, and asynchronous programming. It is part of the System.Threading.Tasks
namespace and provides a higher-level, more abstract model for working with multithreading, asynchrony, and parallelism than the traditional lower-level threading constructs (e.g., ThreadPool or Thread class).
TPL’s main components include Task
, Task<TResult>
, and Parallel
classes, which serve the following purposes:
- Task: Represents an asynchronous operation that doesn’t return a value. Tasks can be awaited using the
await
keyword, or you can attach continuations to them using theContinueWith
method.
Loading code snippet...
- Task: Represents an asynchronous operation that returns a value of type TResult. It derives from the
Task
class and has aResult
property that holds the result once the task is complete.
Loading code snippet...
- Parallel: Provides parallel versions of
for
andforeach
loops, as well as anInvoke
method to execute multiple actions concurrently. TheParallel
class can automatically utilize multiple CPU cores or threads, allowing you to parallelize CPU-bound operations easily.
Loading code snippet...
The TPL allows developers to write efficient and scalable parallel, concurrent, and asynchronous code in C# without having to deal directly with low-level threading and synchronization primitives.
Can you explain covariance and contravariance in C#?
Answer
Covariance and contravariance are terms related to the type relationships in C# when dealing with generics, delegates, or arrays. They describe how a derived type can be used in place of a base type (or vice versa) when assigning variables, parameters, or return values.
- Covariance (from less derived type to more derived type): Covariance allows you to use a more derived type instead of the original generic type parameter. In C#, covariance is supported for arrays, interfaces with generic parameters, and delegates with matching return types.
Loading code snippet...
- Contravariance (from more derived type to less derived type): Contravariance allows you to use a less derived type instead of the original generic type parameter. In C#, contravariance is supported for interfaces with generic parameters marked with the
in
keyword and delegates with matching input parameters.
Loading code snippet...
Covariance and contravariance can help increase the flexibility and reusability of your code by allowing assignments and conversions between different type hierarchies without explicit type casting.
Describe the differences between early binding and late binding in C#.
Answer
Early binding and late binding are two mechanisms in C# related to how the compiler and runtime resolve types, methods, or properties during program execution.
- Early Binding: Also known as static or compile-time binding, early binding refers to the process of resolving types, methods, or properties at compile time. With early binding, the compiler validates that the members being called exist and that they’re being called correctly regarding their accessibility, parameters, and return values. Early binding provides benefits like better performance (due to reduced runtime overhead) and type safety, as the compiler can catch and report errors during compilation. Example:
Loading code snippet...
- Late Binding: Also known as dynamic or runtime binding, late binding refers to the process of resolving types, methods, or properties at runtime. With late binding, the actual binding of members occurs only when the code is executed, which can lead to runtime errors if the members don’t exist or are not accessible. Late binding is often used when the exact types or members aren’t known at compile time or when working with COM objects, reflection, or dynamic types. Late binding has certain advantages, such as allowing for more flexibility, extensibility, and the ability to work with types that aren’t known at compile time. However, it also comes with disadvantages such as increased runtime overhead (due to additional type checks, method lookups, or dynamic dispatch) and a loss of type safety (due to the possibility of runtime errors). Example:
Loading code snippet...
Understanding the differences between early and late binding can help you make informed decisions about when to use each mechanism, based on your requirements for type safety, performance, and flexibility.
What is the Global Assembly Cache (GAC) in C#?
Answer
The Global Assembly Cache (GAC) is a centralized repository or cache for storing and sharing .NET assemblies (DLLs) on a machine. The GAC is part of the .NET Framework and is used to avoid DLL conflicts and enable side-by-side execution of different versions of the same assembly.
Assemblies stored in the GAC must have a strong name, which consists of the assembly name, version number, culture information (if applicable), and a public key token (generated from the developer’s private key). This strong name allows the GAC to uniquely identify and manage each assembly and its versions.
To install an assembly in the GAC, you can use the gacutil
utility, or you can drag and drop the assembly into the GAC folder using Windows Explorer.
Loading code snippet...
As a developer, you typically don’t need to explicitly reference assemblies from the GAC, as the .NET runtime automatically looks for them in the GAC before it searches other locations. However, it’s essential to be aware of the GAC and understand how it impacts assembly sharing, versioning, and deployment scenarios.
Explain how the ‘var’ keyword works in C#, and give a practical example of its use.
Answer
In C#, the var
keyword is used to implicitly type a local variable when you don’t need or want to explicitly specify the type. When you use the var
keyword, the C# compiler infers the type of the variable based on the value assigned to it during initialization. The variable is still strongly-typed at compile-time, just like any other typed variable.
It’s important to note that the var
keyword can only be used with local variables, and the variable must be initialized with a value during its declaration (otherwise, the compiler cannot infer the type).
Using the var
keyword can provide benefits like increased readability and ease of refactoring, particularly when dealing with complex or verbose types (e.g., generics or anonymous types).
Example:
Loading code snippet...
In the example above, the compiler infers the types of the variables based on their assigned values. Using var
here can make the code more concise and readable.
However, it’s important to strike the right balance between readability and maintainability. In cases where using var
might decrease the readability or understandability of the code, it’s better to use an explicit type.
What is a thread-safe collection, and can you give an example of one in C#?
Answer
A thread-safe collection is a data structure designed to be safely and correctly accessed or modified by multiple threads concurrently. In general, most built-in .NET collection classes (e.g., List<T>
, Dictionary<TKey, TValue>
, etc.) are not thread-safe, which means that concurrent access or modifications can lead to unexpected results or data corruption.
To provide thread-safe collections, the .NET Framework offers the System.Collections.Concurrent
namespace, which includes several thread-safe collection classes, such as:
ConcurrentBag<T>
: An unordered collection of items that allows duplicates and has fastAdd
andTake
operations.ConcurrentQueue<T>
: A first-in, first-out (FIFO) queue that supports concurrentEnqueue
andTryDequeue
operations.ConcurrentStack<T>
: A last-in, first-out (LIFO) stack that supports concurrentPush
andTryPop
operations.ConcurrentDictionary<TKey, TValue>
: A dictionary that supports thread-safeAdd
,Remove
, and other dictionary operations.
Example:
Loading code snippet...
In the example above, the ConcurrentQueue<int>
instance safely handles concurrent Enqueue
operations without the need for manual locking or synchronization.
Keep in mind that using thread-safe collections can come with some performance overhead, so you should carefully evaluate the trade-off between thread-safety and performance in your specific use case.
Explain how exception handling works in C# using try-catch-finally blocks.
Answer
Exception handling in C# is a mechanism for dealing with unexpected or exceptional conditions that may occur during program execution. It helps to maintain the normal flow of the program and ensure that resources are properly released even when an exception is encountered.
In C#, exception handling is primarily done using the try
, catch
, and finally
blocks:
- try: The
try
block contains the code that might throw an exception. You enclose the code that may raise exceptions within this block. - catch: The
catch
block is used to handle the specific exception that is thrown within thetry
block. You can have multiplecatch
blocks for different exception types. The first matchingcatch
block that can handle the exception type will be executed. - finally: The
finally
block is used to execute code regardless of whether an exception was thrown or not. This block is optional, and it’s typically used for resource cleanup (e.g., closing files or database connections).
Example:
Loading code snippet...
In the example above, the try
block attempts to execute a division operation that throws a DivideByZeroException
. The catch
block handling DivideByZeroException
is executed, and the exception message is written to the console. Regardless of the exception, the finally
block will always execute, ensuring that any necessary cleanup is performed.
Understanding and properly utilizing exception handling in C# allows you to write more robust, resilient, and fault-tolerant applications.
Superb work in completing the medium questions! You’ve shown great proficiency in C#, and now you’re ready for the next challenge: medium-hard questions. In this section, we explore advanced topics such as LINQ, multithreading and parallel programming, and C# 8.0 features. These questions will truly put your C# skills to the test, but don’t be discouraged. Keep pushing through and learning as you go!
What are the differences between IEnumerable, ICollection, and IList interfaces in C#?
Answer
IEnumerable, ICollection, and IList are interfaces provided by the .NET framework libraries for working with collections of data. They have the following differences:
IEnumerable:
- Provides the basic functionality to iterate through a collection using a foreach loop.
- It supports only-read access.
- Ideal for situations where you only need to iterate through a collection.
- Defines only one method: GetEnumerator(), which returns an IEnumerator.
ICollection:
- Inherits from IEnumerable.
- Represents a collective group of elements with the addition of functionalities like size, enumeration, and synchronization.
- Adds basic collection manipulation like Add, Remove, and Clear.
- Supports both read and write access.
- Provides properties like Count and IsReadOnly.
- Provides methods like Add, Remove, Clear, and Contains.
IList:
- Inherits from ICollection.
- Represents a list of items that are accessible by index.
- Provides advanced collection manipulation like insert and remove by index.
- Supports random access to elements.
- Contains properties like Item (property to access elements by index) and methods like Insert and RemoveAt.
Explain the concept of partial classes in C#.
Answer
A partial class, denoted with the partial
keyword in C#, is a class whose definition can be split into multiple separate class definitions in the same namespace. All the separate class definitions with the partial
keyword are combined by the compiler into a single class at the compile-time. Partial classes can be used to logically organize a large class or to enhance classes generated by code generators (e.g., for UI or web services).
Advantages of partial classes:
- Provides better maintainability by dividing a large class into multiple logical files.
- Enhances code generation in situations when you need to add your own code to a generated class.
- Allows multiple developers to work on the same class simultaneously without conflicts.
Syntax:
Loading code snippet...
At compile-time, these two files are combined into a single class.
What is boxing and unboxing in C#? Provide examples.
Answer
Boxing and unboxing are operations in C# related to type conversions between value types and reference types.
Boxing is the process of converting a value type to a reference type (System.Object) by wrapping the value type inside a new object and storing it on the heap. The conversion is implicit.Example:
Loading code snippet...
Unboxing is the process of converting a reference type back to a value type by extracting the value from the object and storing it on the stack, after checking its type is compatible. Unboxing requires an explicit type cast.Example:
Loading code snippet...
Note that these operations come with a performance cost as they involve memory allocation and deallocation, particularly when dealing with large data structures or frequent conversions.
How can you implement multithreading in C#?
Answer
Multithreading is a technique for executing multiple threads concurrently to improve performance and responsiveness in applications. In C#, there are several ways to implement multithreading, including:
- Using the
Thread
class from theSystem.Threading
namespace. Creating a new Thread requires passing a method (delegate) as a parameter to its constructor that will be executed when the thread starts.
Loading code snippet...
- Using
Task
andTask<TResult>
classes fromSystem.Threading.Tasks
. Tasks provide better abstraction and integration with the ThreadPool, eliminating the need to manually manage threads and their resources.
Loading code snippet...
- Using the
async
andawait
keywords for asynchronous programming. This approach simplifies working with long-running methods without blocking the calling thread.
Loading code snippet...
Additionally, you can use Parallel
class for parallel operations on collections (e.g., Parallel.ForEach
or Parallel.For
), and ThreadPool
for managing a pool of threads.
What is the concept of anonymous methods in C#?
Answer
Anonymous methods in C# are a way to define inline unnamed methods that can be assigned to delegate instances. They provide a concise way to create small functions without declaring an entire named method. Anonymous methods use the delegate
keyword and do not require a separate method declaration.
Example:
Loading code snippet...
In C# 3.0 and later, anonymous methods can be replaced by lambda expressions, which provide a more concise syntax. The previous example can be rewritten as:
DisplayMessage display = message => Console.WriteLine(message);
What are the different types of delegates in C#?
Answer
Delegates in C# can be divided into three main types:
- Singlecast Delegates: These delegates reference a single method with a matching signature. When the delegate is invoked, it calls the referenced method.
Loading code snippet...
- Multicast Delegates: These delegates can reference multiple methods with a matching signature. When the delegate is invoked, it calls all the referenced methods in the order they were added. Multicast delegates are created using the
+=
or-=
operators.
Loading code snippet...
- Generic Delegates: These delegates use generic type parameters, allowing them to work with multiple types without casting or boxing/unboxing. C# provides three built-in generic delegates:
Func
,Action
, andPredicate
.
Func<TResult>
: A delegate that represents a function with a return type.Action
: A delegate that represents a void-returning method with no parameters.Predicate<T>
: A delegate that represents a method that takes an input parameter and returns a boolean.
Example using Func
and Action
:
Loading code snippet...
Explain the principle of encapsulation in C#.
Answer
Encapsulation is a fundamental object-oriented programming principle that refers to the bundling of data (properties) and behavior (methods) within a single class or object. This concept aims to:
- Hide internal implementation details from other parts of the application (encapsulating inner workings).
- Expose a well-defined public interface for communication between objects.
- Easily update or modify the implementation without affecting the external callers.
In C#, encapsulation can be achieved using access modifiers (public, private, protected, internal, protected internal) to restrict access to the class members and by combining fields, properties, and methods inside a class.
Example:
Loading code snippet...
What is the benefit of using an extension method in C#?
Answer
Extension methods in C# are a way to add new methods to an existing type without modifying the original type, inheriting from it, or using any other type of customization. Extension methods can be useful in situations where you do not have the source code of the type, or you want to extend a sealed (non-inheritable) class. The benefits of using extension methods are:
- Improved code readability and maintainability.
- The ability to extend a class without modifying its code.
- Adding functionality to a class without inheriting from it or using composition.
An extension method is defined as a static method in a static class and uses the this
keyword before the first parameter to specify the type it is extending. Example:
Loading code snippet...
Here, the Reverse()
extension method is added to the string
type and can be called as if it was a native method of the string
class.
How does C# support operator overloading?
Answer
Operator overloading in C# allows any custom class or struct to redefine the behavior of certain operators (like +
, -
, *
, ==
, etc.) for its instances. This can provide a more intuitive and natural syntax when working with objects of the custom type.
To overload an operator, you need to define a public static
method in your class with the operator
keyword followed by the operator symbol being overloaded. The method must have the required parameters and return type as specified by the operator being overloaded.
Example:
Loading code snippet...
What is the use of the ‘base’ keyword in C#?
Answer
The base
keyword in C# is a reference to a class’s immediate parent class (base class) and is used to access its members, including properties, methods, and constructors. The base
keyword is often used in the following scenarios:
- Calling base class constructors: When creating an instance of a derived class, you can call a base class constructor from the derived class constructor using the
base
keyword followed by the constructor parameters.
Loading code snippet...
- Accessing base class methods: In a derived class, you may want to access the original implementation of an overridden or shadowed method from the base class. In this case, you can use the
base
keyword followed by the method name.
Loading code snippet...
By using the base
keyword, you can extend or reuse functionality from the base class while maintaining the inheritance relationship between the classes.
Explain how LINQ queries work in C# and give an example.
Answer
Language Integrated Query (LINQ) is a powerful feature in C# that allows you to query data from various data sources directly from within the C# language.
In LINQ, query operations are performed using familiar language syntax. This makes the query quite readable and easy to understand. LINQ queries can work with anything that implements the IEnumerable interface, such as arrays and collections. Plus, they are statically typed and use IntelliSense, making writing queries error-free.
Here is an example.
Loading code snippet...
In the above example, evenNumbers
will hold 2, 4, 6, and 8. The query determines the numbers in the input sequence that fulfill the condition (num % 2 == 0), which are even numbers.
What is a Thread Pool in C#? How can it be beneficial than creating a new thread?
Answer
A Thread Pool in C# is a collection of threads that are made available for use by various tasks. Instead of each task creating, starting, and stopping a new thread, tasks can use a thread from the thread pool, greatly improving efficiency.
There are several benefits to using a thread pool:
- Threads in a thread pool are reused, avoiding the overhead of creating a new thread every time.
- The number of threads in the application does not spike up, avoiding the issue of too many threads which can slow down the system.
- The system automatically manages the details of how and when tasks are assigned to threads in the thread pool.
Here is how you can use the ThreadPool:
Loading code snippet...
In the above line, SomeMethod
is a method that is executed on a separate thread.
What is a Nullable type in C# and how do you use it?
Answer
In C#, Nullable types are value types which can take null values. This capability is crucial because the value types don’t normally take null values. A Nullable type is declared using the ?
symbol after the value type.
For instance, int?
is a Nullable type based on the int
type. You can assign any value which an int
can take, plus an additional null value.
Here is how you can use it:
Loading code snippet...
In the code above, because int?
is a nullable type, we’re able to assign a null
value to number
.
How does C# manage memory automatically?
Answer
Memory management in C# is handled through the Garbage Collector (GC), an automatically enabled feature in the .NET framework. Here is how GC works:
- When an object is created in .NET, it is stored in the heap memory.
- The GC automatically reclaims the memory taken up by the unused objects, or the ones that are no longer being used by the application.
- The GC does this automatically without the programmer having to write any specific code for it. This automatic feature saves the developer from various potential errors like memory leaks and invalid memory references.
- The GC also compacts the space, thus enhancing memory locality, which in turn increases performance since objects that are referenced frequently end up being located close each other.
How do you use reflection in C# to inspect an object’s properties and methods?
Answer
Reflection in C# is a feature that enables you to obtain type information at runtime, inspect the metadata of types, create and manipulate instances of types, and invoke methods.
Here’s an example of using reflection to get an object’s properties:
Loading code snippet...
In the example above, GetType()
gets the type of the person object, GetProperties()
gets all the properties of that type, then we loop through the properties and for each property name and its value, we write it out to the console.
Can you explain what a Lambda expression is and give an example of its use?
Answer
A lambda expression in C# is an anonymous function or method that can be used wherever delegate types are required. They provide a simple and concise way of defining methods. Lambda expressions use the =>
operator, which can be read as “goes to”.
For example, here is a lambda expression that squares a number:
Loading code snippet...
Here x
is the input parameter (can be more than one, comma separated) and x * x
is the method body that is returned from the function.
Lambda expressions can be used in a lot of cases, but they are specially useful in LINQ queries, event handling and creating expression trees.
How do you enforce thread safety in C#?
Answer
Thread safety ensures that data shared between threads are shielded from corruption due to the threads being executed in an overlapping manner. In C#, you can enforce thread safety by using various techniques:
- Locks: The
lock
keyword can be used to ensure that a block of code is not executed by more than one thread at the same time.
Loading code snippet...
- Monitor:
Monitor
class provides exclusive lock similar tolock
keyword.
Loading code snippet...
- Mutexes and Semaphores: Mutex and Semaphore classes can be used to control access over a pool of resources.
- Thread-Safe Collections: .NET provides inherently thread-safe collections like
ConcurrentBag
,ConcurrentQueue
,ConcurrentStack
,ConcurrentDictionary
, etc.
What are the differences between a struct and a class in C#?
Answer
While structs and classes in C# are quite similar, there are many key differences between them:
- Value Type vs Reference Type: The most significant difference is that struct is a value type and class is a reference type. When a struct is assigned to a new variable, a completely new copy of the value is created and changes made to one will not affect the other. However, with classes, assigning an instance of a class to a new variable doesn’t create a new object but creates a new reference to the existing object.
- Inheritance: Structs in C# cannot inherit from a class or struct, but it can implement an interface. But classes in C# can inherit from a class or implement an interface.
- Default Constructor: Structs do not contain a default (parameterless) constructor, and the compiler does not add one. Classes, however, have a default constructor if no constructors are specified.
- Nullability: Structs cannot be null as they are a value type, whereas classes being a reference type can have a null value.
What are Attribute classes and how are they beneficial?
Answer
Attribute classes in C# are a way for adding declarative information to code. It is a kind of metadata that can be added to the assemblies, types, methods, fields etc. at compile time and can be queried at runtime using reflection.
Benefits of Attribute Classes:
- Code readability and maintainability: With attribute classes, you can control behavior of methods or classes without affecting their source code.
- Metadata Access at Runtime: You can access metadata at runtime for affecting the behavior or else for diagnostics / inspection purpose.
- Controlling behavior: Custom attributes can be queried at runtime and accordingly certain behaviors can be enabled or disabled, without hard-coding them.
For example, Conditional attribute in C# is used for conditional compilation:
Loading code snippet...
In this example, DebugMethod
will only be called if “DEBUG” symbol is defined.
You’ve made it through the medium-hard questions, proving you have a solid C# foundation. Now, you’re ready to face the hardest questions in the list. This section contains questions on performance optimizations, architectural patterns, and interop with other languages and technologies. The hard questions are meant to stretch your limits and expose you to real-world programming challenges. Keep pushing forward, and remember that the more you practice, the more confident you’ll be in your C# programming abilities.
Can you describe just-in-time compilation (JIT) in the context of C#?
Answer
Just-In-Time (JIT) Compilation is a technique used by the .NET runtime to provide significant performance improvements by compiling the Intermediate Language (IL) bytecode to native machine code at runtime. Instead of pre-compiling the entire code to native code before executing, the JIT compiler optimizes and compiles only the required methods at runtime, greatly reducing load times and memory usage.
The main benefits of JIT compilation in the context of C# are:
- Faster application startup: Because only the necessary parts of the code are compiled, the application starts up more quickly.
- Better memory usage: Unused IL bytecode is never converted to native code, leading to lower memory usage.
- Platform-specific optimization: Native code is generated specifically for the runtime platform, allowing better optimization and performance.
The process followed by the JIT compiler in the context of C# consists of three stages:
- Loading the IL bytecodes: The CLR loads the required IL bytecode of the method to be executed.
- Compiling IL bytecodes to native code: The JIT compiler compiles the IL bytecodes to native machine code.
- Executing the native code: The generated native code is executed.
What is the difference between a normal class property and a computed class property in C#?
Answer
A normal class property is a simple property that holds a value and includes a getter and/or a setter method. These properties can be used to store and retrieve data for an object. The setter is responsible for setting the property value, and the getter is responsible for returning the property value.
A computed class property, also known as a calculated property, is a property that does not store any data but rather computes its value based on other property values within the class. Computed properties only have a getter method, which returns the calculated result, and do not have a setter method.
Normal property:
Loading code snippet...
Computed property:
Loading code snippet...
In this example, FullName
is a computed property that returns the concatenated first name and last name.
How can you implement a custom awaitable type in C#?
Answer
To implement a custom awaitable type in C#, you need to follow these steps:
- Create a class that represents the awaitable type.
- Implement the
INotifyCompletion
interface in the class for notifying when the operation is complete. - Add a method named
GetAwaiter
that returns an instance of the class itself. - Implement the
IsCompleted
property in the class as part of the awaitable pattern. - Add a method named
OnCompleted(Action continuation)
to the class which takes an action that will be executed when the operation is complete. - Implement the
GetResult
method, which will return the result of the asynchronous operation.
Here’s an example of a custom awaitable type:
Loading code snippet...
Why would you use the System.Reflection namespace, and how does it relate to C#?
Answer
System.Reflection is a namespace in .NET that provides functionality to obtain type information (metadata) about classes, objects, and assemblies at runtime. It allows developers to inspect and interact with the code in a dynamic manner, providing the ability to:
- Examine type information such as properties, fields, events, and methods.
- Create and manipulate instances of objects.
- Invoke methods and access fields/properties on instances.
- Discover and examine attributes applied to types and members.
- Load and interact with assemblies.
The following example demonstrates using System.Reflection to get information about a class’s type and its methods:
Loading code snippet...
What are expression trees in C# and how can they be used?
Answer
Expression trees in C# are a data structure that represents code – specifically, Expressions – in a tree-like format, where each node is an object that represents a part of the expression. Expression trees enable developers to inspect, manipulate, or interpret code in a structured way at runtime. They allow operations such as modification, compilation, and execution of the expressed code.
Expression trees are mainly used in scenarios such as:
- Building dynamic LINQ queries for data manipulation.
- Dynamic code generation for performance-critical code paths.
- Serialization and deserialization of expression trees.
- Analyzing lambda expressions for parallelism or converting them to another form.
Here’s an example of creating an expression tree for a simple lambda expression:
Loading code snippet...
What is the real-world use case for the ‘yield’ keyword in C#?
Answer
The yield
keyword in C# is used in iterator methods to create a stateful iterator and return a sequence of values on-the-fly, without storing the entire sequence in memory. It generates a custom implementation of the IEnumerator<T>
interface based on the code in the iterator method and remembers the current execution state between MoveNext()
calls. This lazy evaluation of the iterator improves memory usage and performance, especially for large or infinite sequences.
Real-world use cases for the yield
keyword include:
- Implementing custom collections or sequences that need to support
foreach
iteration. - Generating an infinite sequence of values or a sequence that should not be stored in memory.
- Processing large data sets or streaming data incrementally without consuming a lot of memory.
Here’s an example demonstrating the use of the yield
keyword for generating an infinite sequence of even numbers:
Loading code snippet...
Explain the role of the ‘volatile’ keyword in C#.
Answer
The volatile
keyword in C# is applied to fields to indicate that they can be accessed by multiple threads and that the field’s value may change unexpectedly, due to optimizations performed by the .NET runtime or underlying hardware.
When a field is marked as volatile
, the compiler and the runtime will not reorder or cache its read and write operations, ensuring that the most recent value is always read and that writes are immediately visible to other threads. This provides a memory barrier, forcing atomic read and write operations and preventing unexpected side effects due to optimizations.
The volatile
keyword should be used in scenarios where multiple threads must access a shared field, and proper synchronization is required to maintain the consistency of data.
Here’s an example demonstrating the use of a volatile
variable:
Loading code snippet...
In this example, the _shouldTerminate
field is used to signal the worker thread when to terminate. Marking it as volatile
ensures that the worker thread will see the updated value immediately.
What are weak references, and when would you use them in C#?
Answer
In C#, weak references are references to objects that aren’t strong enough to prevent these objects from being garbage collected. They allow you to maintain a reference to an object as long as the object is alive in memory, but without preventing garbage collection (GC) from reclaiming the object when memory pressure increases. With weak references, you can access the object as long as it’s still in memory, but it will not prevent the GC from collecting the object if required.
Weak references are useful in scenarios where you want to hold a reference to a large object for caching purposes but do not want to prevent the object from being garbage collected if the memory pressure increases. This allows for more efficient memory management, especially when working with large data sets or in-memory caches.
To use a weak reference in C#, you create an instance of the WeakReference
or WeakReference<T>
class.
Here’s an example demonstrating the usage of a weak reference:
Loading code snippet...
In this example, if the GC decides to reclaim the memory used by the MyLargeObject
instance, the weakReference.TryGetTarget
call returns false
. Otherwise, the largeObject
will remain accessible through the weak reference.
Describe how to implement a custom attribute in C#.
Answer
To implement a custom attribute in C#, you follow these steps:
- Create a class that derives from the
System.Attribute
class. - Add properties, fields, or methods to the class as required to store metadata.
- Apply the attribute to elements in your code (such as classes, methods, or properties) by using the attribute syntax.
Here’s an example of creating and using a custom attribute in C#:
Loading code snippet...
In this example, we created a custom attribute called CustomAttribute
with a description
property. We applied it to the ExampleClass
and its ExampleMethod()
. The AttributeUsage
attribute is used to limit the targets to which the custom attribute can be applied.
Explain the concept of memory-efficient array handling in C# using ArraySegment.
Answer
The ArraySegment<T>
structure in C# provides a memory-efficient way of handling arrays by allowing you to work with a segment of an existing array. This is useful in scenarios where you need to process a portion of a large array and want to avoid memory overhead caused by creating new subarrays.
The ArraySegment<T>
structure represents a contiguous range of elements within an array and provides properties, such as Array
, Offset
, and Count
, to access the underlying array and the segment boundaries.
Here’s an example that demonstrates the use of ArraySegment<T>
for memory-efficient array processing:
Loading code snippet...
In this example, an ArraySegment<int>
object is created to represent a portion of the largeArray
. The PrintArraySegment
method processes the array segment without creating a new subarray, thus reducing memory overhead.
What is the Roslyn Compiler Platform, and how does it relate to C#?
Answer
The Roslyn Compiler Platform is a set of open-source compilers, code analysis APIs, and code refactoring tools developed by Microsoft for C# and Visual Basic .NET (VB.NET) languages. Roslyn exposes a powerful code analysis and transformation API, allowing developers to create more advanced static code analyzers, code fixers, and refactoring tools.
Roslyn’s relation to C#:
- It provides the default C# compiler which transforms C# code into Microsoft Intermediate Language (MSIL) code.
- It offers a modern C# language service implementation for Visual Studio.
- It lets developers take advantage of the code analysis and manipulation APIs for deeper code insights and generation.
- It supports advanced features of modern C# versions like pattern matching, expression-bodied members, and async-await constructs.
Explain the concept of duck typing and how it can be achieved in C#.
Answer
Duck typing refers to a programming concept in which the type of an object is determined by its behavior (methods and properties) rather than its explicit class or inheritance hierarchy. In other words, duck typing is based on the principle: “If it looks like a duck, swims like a duck, and quacks like a duck, then it probably is a duck.”
C# is a statically typed language, which means duck typing is not directly supported by the language. However, you can achieve a form of duck typing in C# using dynamic
keyword or reflection.
Using dynamic
keyword:
Loading code snippet...
But it’s important to note that using dynamic
may bring performance overhead, and you lose some compile-time safety. Errors will occur at runtime if the method or property doesn’t exist on the object.
What is the difference between GetHashCode and Equals methods in C#?
Answer
GetHashCode
and Equals
methods are members of the System.Object
class, the base class for all objects in C#. They are used to compare objects for equality and serve different purposes:
GetHashCode
: This method returns an integer (hash code) representation of the object. It is primarily used by hashing-based collections likeDictionary
,HashSet
, etc., to optimize the object’s lookup and storage. When implementing this method, you should make sure objects considered equal have the same hash code value.Equals
: This method checks whether two objects are equal in their content. It uses their data members to determine equality. By default, theEquals
method will compare object references, but it can be overridden to provide custom comparison logic based on the actual object content (like comparing properties).
When you override the Equals
method, you should also override the GetHashCode
method to maintain consistency between the two methods, ensuring objects that are considered equal have the same hash code.
Explain the concept of unmanaged resources in C# and how they can be managed.
Answer
Unmanaged resources are those not directly controlled by the .NET runtime, such as file handles, network connections, database connections, and other system resources. Because the .NET runtime’s garbage collector does not manage them, developers must handle such resources explicitly to avoid resource leaks or potential application instability.
To manage unmanaged resources properly, you can:
- Implement the
IDisposable
interface in your class which uses unmanaged resources. TheIDisposable
interface provides aDispose
method for cleaning up unmanaged resources.
Loading code snippet...
- Use the
using
statement to ensure theDispose
method is called automatically when the object goes out of scope.
Loading code snippet...
In this example, the Dispose
method will be called automatically when the using
block is exited, ensuring proper cleanup of the unmanaged resources.
Describe parallel programming support in C# and its benefits.
Answer
Parallel programming support in C# is provided by the System.Threading
, System.Threading.Tasks
, and System.Collections.Concurrent
namespaces. These parallel execution features allow developers to write applications that leverage modern multi-core and multi-processor hardware for better performance and responsiveness.
Key parallel programming features in C#:
- Task Parallel Library (TPL): Provides a high-level abstraction for executing tasks concurrently, simplifying the parallelism work like managing threads, synchronization, and exception handling.
- Parallel LINQ (PLINQ): A parallel execution version of standard LINQ, enabling developers to easily parallelize data-intensive query operations efficiently.
- Concurrent Collections: Collections like
ConcurrentDictionary
,ConcurrentQueue
,ConcurrentBag
, andConcurrentStack
provide thread-safe data structures to help manage shared state in parallel applications.
Benefits of parallel programming in C#:
- Improved performance by taking advantage of multi-core and multi-processor systems.
- Increased responsiveness by executing time-consuming tasks concurrently without blocking the UI thread.
- Simplified parallel programming through high-level abstractions provided by TPL and PLINQ.
- Better utilization of system resources leading to more efficient, scalable applications.
How do you perform compile-time code generation in C#, and what are the benefits?
Answer
Compile-time code generation in C# can be achieved using Source Generators, which are introduced in C# 9.0 and .NET 5. Source Generators are components that run during compilation and can inspect, analyze, and generate additional C# source code to be compiled alongside the original code.
A Source Generator is a separate assembly containing one or more classes implementing the ISourceGenerator
interface. Visual Studio and the .NET build system will discover Source Generators with the appropriate project references and will run them during the compilation process.
Benefits of compile-time code generation:
- Improved performance: Code generation at compile-time reduces runtime overhead.
- Code reduction: Automatically generating repetitive or boilerplate code reduces the code a developer needs to write and maintain.
- Security: Generated code is verified and secured before runtime, preventing security vulnerabilities arising from runtime code generation.
- Extensibility: Source Generators enable the creation of advanced libraries and frameworks, which can further enhance and optimize code generation and manipulation.
What is stackalloc in C#, and when should it be used?
Answer
In C#, stackalloc
is a keyword that allows you to allocate a block of memory on the stack.
Loading code snippet...
Normally, when we work with arrays in C#, they are created on the heap. This introduces a level of overhead, as memory must be allocated and then garbage collected when the object is no longer in use. When dealing with large arrays or high performance code, the impact of this can sometimes be significant.
The stackalloc
keyword bypasses this by creating the array directly on the stack. This has 3 major implications:
- Performance: Generally, allocating memory on the stack is faster than allocating it on the heap. There’s no need to worry about garbage collection, as the memory will be automatically reclaimed when the method exits. This makes it highly efficient for small arrays.
- Memory Limit: The stack is a far more limited resource compared to the heap. The exact size depends on settings and other factors, but it’s typically in the region of 1MB. This makes
stackalloc
unsuitable for larger arrays. - Lifespan: Memory allocated on the heap can exist as long as your application does, whereas memory allocated on the stack only exists until the end of the current method. Any attempt to work with stack-allocated memory outside of this will lead to issues.
The typical use cases for stackalloc
are high performance scenarios that involve relatively small arrays, such as graphical or mathematical operations. Be aware that improper use can easily lead to stack overflows, causing your application to crash.
Consider using stackalloc
if the following cases are true:
- You have a small array (typically few hundred elements max).
- The array is local to your method and doesn’t need to be returned or passed elsewhere.
- The overhead of garbage collection has a significant impact on performance in your use case.
Here is an example of using stackalloc
:
Loading code snippet...
Please note that stackalloc
uses unsafe code, so you must compile with the /unsafe switch.
Explain the Tuple class and its use case in C#.
Answer
The Tuple
class in C# represents a fixed-size, immutable collection of heterogeneously-typed elements. It’s part of the System
namespace and provides a simple way to group objects without defining custom domain-specific classes. Tuples are useful in scenarios where you need to return multiple values from a method or store or pass around data without creating a specific data structure.
Example of using Tuple:
Loading code snippet...
In C# 7.0, tuples were improved with the introduction of ValueTuple. ValueTuples are struct-based (instead of class-based) tuples that allow named elements and other enhancements:
Loading code snippet...
What are local functions in C# 7.0, and how can they be used?
Answer
Local functions, introduced in C# 7.0, are functions defined within the scope of another method. They enable you to declare a helper function inside the method that needs it, keeping the helper function private and avoiding clutter in the class level namespace.
Local functions offer advantages like:
- Better organization and encapsulation of functionality.
- Access to the containing method’s variables, allowing them to share state easily.
- Limited accessibility, as local functions are not visible outside their containing method.
Example of a local function:
Loading code snippet...
In this example, the Add
local function is only visible within the CalculateSum
method and can access the local variables (e.g., sum
) of the containing method.
Explain the concept of marshaling in .NET and its application in C#.
Answer
Marshaling is the process of converting the types, objects, and data structures of one runtime environment to another, especially in the context of communication between managed (.NET) code and unmanaged (native) code. Marshaling is widely used in .NET when you want to interact with components developed in other programming languages, operating systems, or hardware and software platforms (e.g., COM, Win32 API, or other third-party libraries).
In C#, marshaling is facilitated by the System.Runtime.InteropServices
namespace, providing required attributes, methods, and structures to:
- Define the layout of structures and unions in memory.
- Map managed types to unmanaged types.
- Specify calling conventions for unmanaged functions.
- Allocate and deallocate unmanaged memory.
Example of using marshaling in C#:
Loading code snippet...
In this example, we import the MessageBox
function from the user32.dll
native library using the DllImport
attribute. This enables the managed C# code to call the unmanaged Win32 MessageBox function. The marshaling process will handle the conversion of string values and other necessary data types when calling the unmanaged function.
You’ve made it! Congratulations on completing this comprehensive set of C# interview questions and answers. Through this journey, you’ve gained valuable insights into C#, from the basics to the most advanced programming concepts. By mastering these questions, you’ve built the knowledge and confidence needed to excel in your next C# interview.
Remember that the key to success lies in continuous learning and staying updated on the latest developments in C# and the .NET ecosystem. Keep sharpening your skills and networking with fellow developers to stay informed and be more competitive in the job market. We wish you the best of luck in your upcoming interviews, and we’re confident that you’ll shine as a C# developer!