Dynamic Type in C# – Full Guide
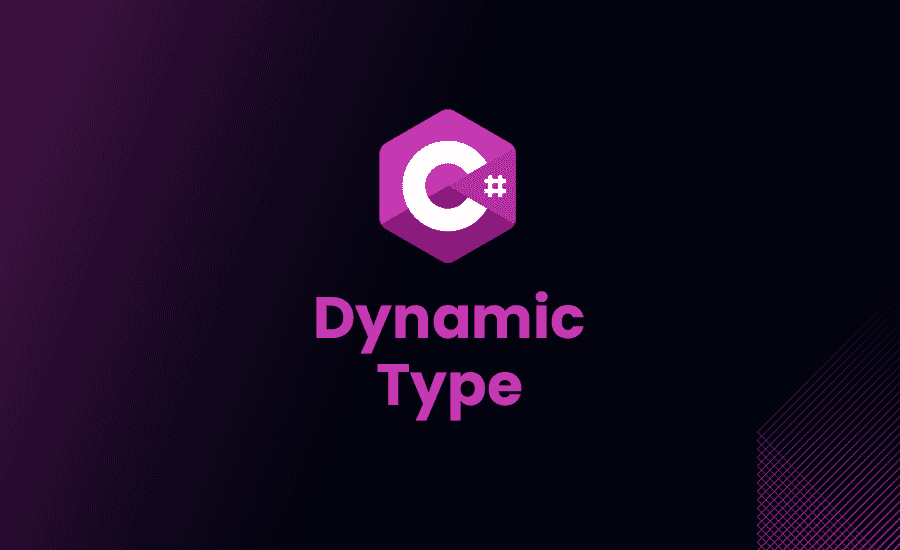
You’ll agree with me that the dynamic type in C# is no less than a cherry on the cake for programmers, granting them unprecedented flexibility. However, it’s a feature that necessitates a strong grasp of its functionality and purpose. That’s where we step in! In this article, we will journey into the heart of dynamic types in C#, stirring in some dynamic JSON objects, dynamic classes, and vital keywords along the way. All set? Let’s dive in!
Introducing Dynamic Type in C#
In static programming languages like C#, the data type of a variable is known at compile time. However, this context takes a different turn with the advent of dynamic type. With dynamic type, C# introduces a paradigm shift enabling you to declare variables, the type of which is not known until they are used during program execution.
This feature comes in particularly useful when the type of data can be different in different scenarios.
It’s important to note that dynamic type bypasses static type checking. While this feature can help you write more flexible code, it means that type errors won’t be caught until runtime, potentially leading to runtime errors if not handled properly.
To put it simply, dynamic type arms you with great programming power, but you must wield it thoughtfully and with care.
Loading code snippet...
In the code above, various variables of dynamic type are declared and initialized with various types of values such as integer, string, and boolean.
Dynamic Keyword in C#
The “dynamic” keyword is your primary conduit to leverage the usage of dynamic type in C#. It signals to the compiler that the type checking needs to be done at runtime rather than compile time.
This functionality allows objects to be used in ways that cannot be known at compile-time, giving you a greater degree of flexibility especially while working with external libraries, COM objects, reflection, or with serialized data.
Let’s explore more about its practical implementations:
The Dynamic Keyword in Variable Declaration
The dynamic keyword can be brilliantly utilized for variable declaration when you do not know what type of data the variable will store. The compiler trusts that you’ve done your homework and leaves the type checking for runtime.
Loading code snippet...
In this code snippet, the same dynamic variable ‘price’ initially holds a double, and subsequently, it’s amended to a string without any compilation error. This roller coaster ride is perfectly smooth and legal!
The Dynamic Keyword with Object Operations
Dynamic operations involve the execution of certain operations with the dynamically-typed objects which the compiler cannot verify. Such instances are best dealt with using the dynamic keyword.
For instance, let’s create an anonymous type, and assign an instance of this type to a dynamic variable:
Loading code snippet...
Even though the anonymous type does not explicitly declare these properties at compile-time, no error is thrown as they are dynamically bound at run-time.
Handling Dynamic JSON Objects in C#
JSON objects are the lingua franca of data in modern programming. They’re versatile, language-agnostic, and exceedingly handy for storing and transporting data. In the realm of C#, dynamic JSON objects provide us with the capability to handle this data in a flexible and efficient manner.
This segment will delve into the nuances of working with dynamic JSON objects in C#, guiding you through parsing dynamic JSON and creating dynamic JSON arrays.
Parsing Dynamic JSON Objects in C#
In C#, handling dynamic JSON objects is a breeze, thanks to libraries such as Newtonsoft.Json. This library allows us to parse JSON strings into dynamic objects which we can then use in our code.
Loading code snippet...
In the above piece of code, we use JObject.Parse()
method to parse a JSON string into a dynamic object named data.
This dynamic object behaves in a such way that you can access its properties directly, just as you’d do with a regular object:
Loading code snippet...
This will print the Name
and Age
properties from the parsed JSON.
Building and Manipulating Dynamic JSON Arrays in C#
In addition to handling dynamic JSON objects, C# also allows us to create and manipulate dynamic JSON arrays. This ability is extremely useful when you need to construct or alter JSON arrays on the fly, without a predefined structure.
You start by creating an empty JArray
:
Loading code snippet...
Next, you can add objects to this array. Note that you can add any JSON-compatible data: strings, numbers, booleans, null, other arrays or objects, and so on:
Loading code snippet...
The jsonArray
now contains four elements of different types. When serialized to a string, it will look like this:
Loading code snippet...
What’s truly beautiful about dynamic JSON arrays in C# is the flexibility they provide, you can modify existing or add new elements at any time without concern for static typing restrictions. Furthermore, using dynamic JSON arrays with libraries like Newtonsoft.Json makes it easy to parse, manipulate, and validate JSON data in C#.
Defining Dynamic Classes in C#
Dynamic classes in C# are a powerful tool for developers. They provide unparalleled flexibility in managing and manipulating data, opening doors to versatile applications and solutions. Let’s delve deeper into this concept and further explore how you can dynamically create classes in C#.
How to Tailor Classes in C#: Dynamic Approach
Creating a class dynamically in C# involves majorly two steps: defining a dynamic class and then using it in your code. The power of this approach lies in defining properties and methods dynamically, allowing for versatile usage as per specific requirements in real-time applications.
Let’s start with defining a dynamic class:
Loading code snippet...
Here, we create a class DynamicClass
that extends the DynamicObject
class. The DynamicObject
class is a base class for specifying dynamic behavior at runtime. We use it to specify how the DynamicClass
should handle dynamic operations like setting and getting values of properties.
Let’s see how we can utilize this dynamic class:
Loading code snippet...
In the above code, we create an instance of DynamicClass
and assign it to a dynamic
type variable demoClass
. We then dynamically add two properties FirstName
and LastName
to the demoClass
object and set their values. Finally, we print these properties to the console. The dynamic nature allows us to define only the properties we need, when we need them.
Real-life Applications of Dynamically Created Classes
In real-life scenarios, there could be many use cases for dynamically created classes, especially in areas where fixed data structures are not desirable or practical.
- Data Mappings: If you are dealing with API responses or database records where data structure is not consistent, you can use dynamic classes to map received data as per your requirement.
- Prototyping: Dynamic classes can be handy for prototyping implementations where you want to quickly test a concept without having to define a rigid structure upfront.
Loading code snippet...
While it’s powerful, it’s also important to bear in mind that dynamically created classes should be used judiciously and can potentially introduce debugging difficulties if not handled properly. They are a potent tool in the C# dynamic arsenal but require a careful and considered approach.
In conclusion, it’s safe to say that the dynamic type in C# is a game-changer, pushing the boundaries and providing potent solutions to modern development challenges.
However, remember to tread lightly. There’s a reason great chefs measure their ingredients meticulously — maintaining balance in their creation. Do the same with the dynamic type.
Now, I won’t hold back from asking you — What’s holding you back from embracing dynamic types? What’s stopping you from seizing the power of flexibility in C# programming?
Whether it’s creating interactive web applications or reshaping how you handle data, the dynamic type is your secret weapon! Explore, experiment and excel.