What is Lock Keyword in C#? Main Usages
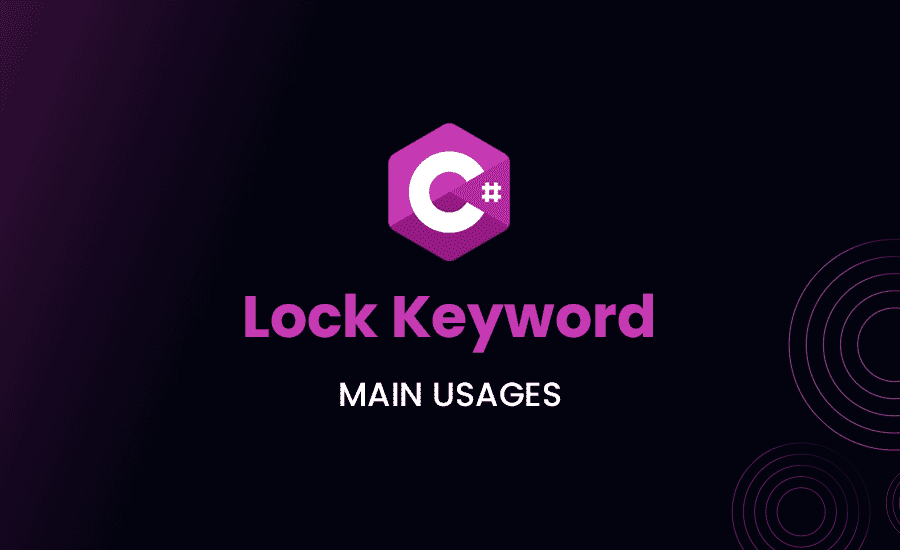
Understanding the C# Lock Keyword
Can’t tell a lock from a block in C#? Reading all this without a clue is exhausting. Let’s turn confusion into mastery, shoving the fear away!
Imagine you’re in a house full of curious kids who want to access a room full of treasures (say, video games!). To protect the room, what would you do? You’d put a lock on the door, right? The lock
keyword in C# is that security guard, that keeps the data safe from simultaneous update attempts. Let’s get to know this guardian better!
Loading code snippet...
In this code snippet, lock(_lock)
ensures only one thread can access and update the inventory at a time. Neat, isn’t it?
Understanding the C# Lock Keyword
Confused about the lock keyword in C#? No worries. Let’s bring some clarity to this topic!
In C#, the ‘lock’ keyword is used to manage access to a block of code that can be executed by multiple threads simultaneously. It’s the gatekeeper, protecting access to a shared resource and making sure only one thread is executing the code at a time, avoiding the chaos of a race condition.
In the C# world, the lock keyword is used to create a critical section around your code. But what’s a ‘critical section’? Simply put, it’s a piece of code where you coordinate access to shared resources. The rule is: Only one thread at a time can execute in a critical section.
Think of it this way: The lock
keyword role is like having a single toilet in a small restaurant. You need to coordinate usage, because let’s be frank, we don’t want two people in there at the same time!
Here’s a simple example of using ‘lock’ in C#:
Loading code snippet...
In the above example, myLock
is a gatekeeper, guarding the door to the critical section (the code inside the lock statement block). When one thread is executing the guarded code, if another thread arrives, it must wait outside until the first one is finished. See how the lock statement helps us maintain order in a potentially chaotic world of concurrent execution?
Delving Deeper: Lock Keyword in C# with Example
Better understanding always comes with examples, right? Let’s break down how ‘lock’ really works with a more concrete example.
Imagine an online ticket booking system for a popular concert. There are limited tickets and a lot of users trying to book at the same time – that’s a lot like threads trying to access a shared resource. Problems like overbooking can occur if the booking process is not atomic. Without coordination (locking), we could accidentally sell more tickets than we have – quite chaos, isn’t it?
In C#, ‘lock’ is a hero that ensures that the booking process remains orderly and only one thread can book at a time. So even if there’s a mad rush, with ‘lock’ in enforcement, there’s no overbooking.
Loading code snippet...
Best Practices Associated with C# Lock Keyword
Just like in other aspects of life, there are preferable ways of doing things in programming. Here are some pro tips about ‘lock’:
- It’s not wise to lock on ‘this’ or public types. It can lead to issues with race conditions if the same object is locked by other parts of your application. It’s akin to sharing your bedroom key with all your neighbors!
- Use a private, readonly object to lock on. This reduces the risk of another thread locking on the same object causing deadlocks – it’s like having your personal locker, safe and reliable.
- Remember that the smaller the scope of the lock, the better. Being locked out isn’t fun, so keep the duration of your lock as short as possible. It’s more like waiting outside a pub for a friend – shorter waiting times are more liked!
Investigating C# Lock Keyword Performance
Thinking about the performance implications of ‘lock’? Inspired by great musicians, let’s tune in our chords to the rhythm of best performance!
‘Lock’ adds a slight overhead to your application. Like adding security in real life, it slows things a bit. But the cost is usually worth paying for the reduced risk of data corruption due to race conditions.
Keep the amount of code inside the lock block as minimal as possible since the lock blocks the execution of other threads, similar to how congested traffic can slow down movement. Be aware that too much use of ‘lock’ can lead to problems like deadlocks and thread starvations.
Loading code snippet...
In the above code, only one line of code is within the lock
block, which will limit the period of time other threads are blocked and thus enhance the performance.
Conclusions: The Power and Efficacy of C# Lock Keyword
After journeying through the world of C#’s ‘lock’ keyword, you’ve learned how invaluable it is to smoothly navigate the realm of multicore processing. You’ve met the effective gatekeeper called ‘lock’, designed to deal with the challenges offered by parallel processing.
You’ve also discovered the recommendations and cautions to leverage it without getting caught up in any deadlock. It’s all about keeping things orderly and efficient in the bustling world of concurrent threads!
Can we use the lock keyword with non-bool types?
Yes, absolutely! A common misunderstanding is that the lock
keyword in C# can be used with only boolean types. Here’s the plot twist – lock
keyword can be used with any reference type, not just boolean. Essentially, the lock
keyword is looking for an object to lock on.
Loading code snippet...
Here, we’re using lock
with the _lock
object to ensure only one thread can add to the list at a time. Simple, right?
What will happen if another thread hits the ‘lock’ when one is still inside?
Picture ten people trying to get through a narrow door at the same time. Chaos, right? Same with threads! When a lock
is hit by another thread while it’s still locked, that thread waits until the first one completes. It’s like forming an orderly line to get through the door – orderly and civil!
Loading code snippet...
If CriticalFunction()
is hit by two threads simultaneously, the first thread will take the lock
and begin executing the critical section. The second thread will wait at the lock
until the first one exits. It’s like waiting patiently for your turn at the grocery store checkout!
How does ‘Monitor’ relate to the ‘lock’ keyword in C#?
Are lock
and Monitor
siblings, you ask? You’re right, kind of! lock
keyword in C# is essentially syntactic sugar provided by C# over some methods provided in the Monitor
class. When you use lock
, you’re using Monitor
under the hood. It’s like driving a car where lock
is the steering wheel, and Monitor
is the engine.
Loading code snippet...
The above code does the exact same thing as lock(_lock)
. The try/finally
block ensures Monitor.Exit()
is always hit even if an exception is thrown, similar to how lock
would behave. So lock
and Monitor
are two sides of the same coin!
In the world of C#, lock
keyword is a key player. It’s one of those indispensable friends like cookies with milk or chips with salsa. So, get comfy and make ‘lock’ your pal. Happy coding, folks!