ASP.NET Interview Questions and Answers
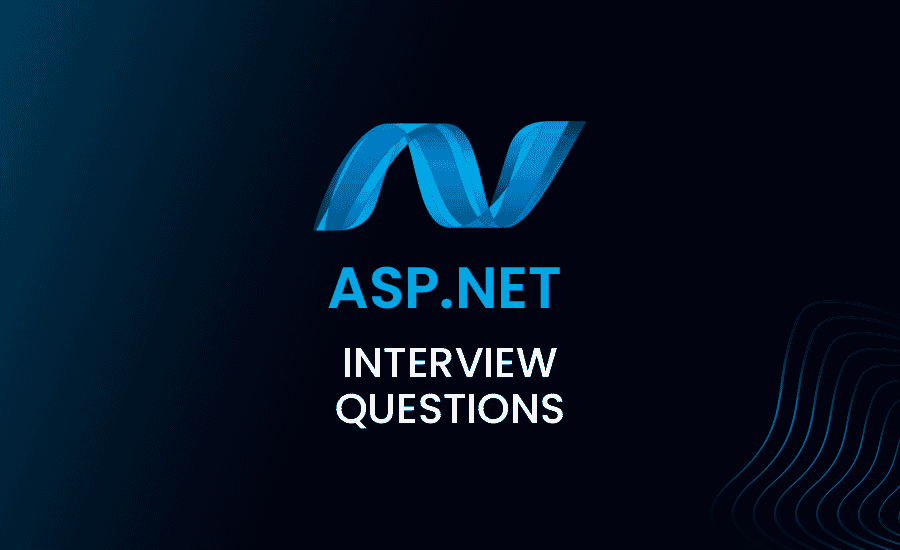
Welcome to a comprehensive guide on ASP.NET interview questions and answers that will help you ace your next developer job interview.
Through this article, we cover a wide range of topics – from basic to advanced, and even scenario-based questions – so you can be thoroughly prepared.
Whether you are a beginner or an experienced professional, this collection of asp net core interview questions and asp net interview questions will provide you with valuable insights and a deeper understanding of the ASP.NET framework.
So, let’s dive right in and explore what it takes to stand out during your upcoming asp net interview.
In ASP.NET, how does the ViewState maintain state across postbacks for a given control? Explain the role of hidden fields, encryption, and serialization in this process.
Answer
ASP.NET’s ViewState is a mechanism to preserve the state of a control across postbacks, without using server resources like session state. It is implemented with various techniques such as hidden fields, encryption, and serialization. Here’s a brief overview of ViewState and how these techniques come into play:
- Hidden fields: ViewState information is stored in a hidden field on the web page. This field is named
__VIEWSTATE
and is located within the HTML source code of the page. ViewState data is converted into base-64 encoded strings and stored in this hidden field. When a postback occurs, ViewState information is sent back to the server, which is then used to repopulate the control state. - Serialization: ViewState data needs to be converted into strings so that they can be stored in the hidden field. This is accomplished through serialization. The ViewState data is serialized into a stream of bytes, which is then converted into a base-64 encoded string to be stored in hidden fields.
- Encryption: ViewState data can be sensitive, and to maintain security, it is possible to encrypt the serialized data before storing it in the hidden field. ASP.NET provides the
LosFormatter
class to perform this encryption. By enabling ViewState encryption in the web.config file, the ViewState data is encrypted before it is stored in the hidden field and decrypted when retrieved during a postback.
In summary, ViewState works by serializing, and optionally encrypting, the control state data and storing it in a hidden field on the webpage. When a postback occurs, the ViewState data is sent back to the server, decrypted if necessary, and deserialized to restore the state of the control.
What are the differences between a custom control and a user control in ASP.NET? Provide examples of when you would choose to use each.
Answer
Custom controls and user controls are two methods of creating reusable components in ASP.NET. They have distinct differences and usage scenarios:
Custom Control:
- Developed using a compiled language, such as C# or VB.NET
- Derives from the
System.Web.UI.WebControls.WebControl
class - Renders its own HTML output
- Can be reused in multiple applications by referencing the assembly
- Has better performance due to pre-compilation
- Generally more flexible and customizable
Usage example: Create a custom calendar control that displays events and supports custom theming or dynamic interactions, such as dragging an event to a new date.
User Control:
- Developed using an ASCX file, which is a mix of HTML markup and server-side code (C# or VB.NET)
- Derives from the
System.Web.UI.UserControl
base class - Easier to develop because it uses a visual designer
- Can be reused only within the same application (unless packaged and distributed)
- Usually serves as a container for multiple existing controls with predefined layout
Usage example: Create a reusable header that includes navigation links, a logo, and a search box to be used across an entire web application.
How would you implement lazy loading for large data sets in an ASP.NET application, optimizing performance while maintaining responsiveness?
Answer
Lazy loading is a technique that loads data on-demand when it’s needed, instead of loading all the data upfront. This approach can improve performance when dealing with large data sets, especially when dealing with lists, grids, or images.
Here’s a general approach for implementing lazy loading in an ASP.NET application:
- Paginate data: Divide the data set into smaller chunks called pages. Determine the appropriate page size based on the data and user experience considerations.
- Client-side implementation:
- Implement an event listener on scrolling or button click to detect when the user requires more data.
- Send an AJAX request to the server with the required page number and page size as parameters when loading more data is needed.
- Server-side implementation:
- Receive the AJAX request and extract the page number and page size.
- Retrieve the required data page-wise from the data source (such as a database or API).
- Serialize the response data and send it back to the client as JSON or HTML.
- Update UI: Once the client receives the data from the server, update the UI with the new data, either by appending new elements to the existing list or updating specific parts of the UI.
By using this approach, the application will load a smaller amount of data initially and only request more data when necessary, thus optimizing performance and maintaining responsiveness for the end user.
In what situations would you choose to use an IHttpModule instead of an HttpHandler?
Answer
Both IHttpModule and IHttpHandler are used for handling and processing HTTP requests in an ASP.NET application, but they serve different purposes:
IHttpModule:
- Responds to global application events such as
BeginRequest
,Error
, andEndRequest
. - Can perform tasks that are unrelated to the specific request URL.
- Can be used to implement cross-cutting concerns (e.g., authentication, logging, rewriting URLs, etc.)
- Multiple modules can execute code for a single request as part of the ASP.NET request pipeline.
Usage Example: Implement a custom logging IHttpModule that logs the details of every request and response, no matter which URL is accessed.
HttpHandler:
- Processes specific types of requests based on the URL or file extension.
- The HttpHandler directly generates the output for the request, such as rendering an image or generating an XML file.
- A single HttpHandler processes a request and finalizes the response output.
Usage Example: Implement a custom HttpHandler to serve dynamic images or handle file downloads with specific access restrictions based on request parameters.
To decide between IHttpModule and HttpHandler, consider the scope of the functionality. If the functionality is related to the processing of a specific request (e.g., rendering output), use an HttpHandler. If the functionality applies to many or all requests, such as logging or authentication, use an IHttpModule.
Explain the benefits and drawbacks of using a single-instance Singleton pattern in an ASP.NET application.
Answer
The Singleton pattern is a design pattern that ensures a class has only one instance and provides a global point of access to it. It can be useful in certain situations but also has its drawbacks.
Benefits:
- Resource efficiency: By ensuring only one instance, Singletons can reduce memory usage and resource consumption, which is useful for expensive shared resources (e.g., database connections or configuration objects).
- Global access: Singleton objects can be accessed from anywhere within the application without the need to pass them around, simplifying code access to shared resources.
- Consistency: Singleton objects maintain their state throughout the application lifecycle, which can ensure data consistency when managing shared resources.
Drawbacks:
- Potential scalability issues: Since Singleton objects are accessed globally, they can become a bottleneck, leading to concurrency issues and affecting scalability in high-traffic multi-threaded environments.
- Testing difficulty: Singletons can make unit testing difficult due to their global state, which can lead to tests affecting each other and producing false results.
- Increased coupling: The Singleton pattern increases coupling between application components, as it leads to a higher dependency on the Singleton object.
While Singletons can be beneficial for resource management and access to shared resources, they should be used with caution, considering the potential drawbacks in terms of scalability, testing, and increased coupling. Alternatives, such as dependency injection, should be considered when appropriate to mitigate these drawbacks.
Now that we’ve discussed the benefits and drawbacks of using a single-instance Singleton pattern in ASP.NET, let’s move on to more asp net practical interview questions and answers, specifically related to synchronous and asynchronous programming.
Describe the differences between synchronous and asynchronous programming in ASP.NET. How would you determine which approach to use in a specific scenario?
Answer
Synchronous and asynchronous programming are two different ways to handle tasks and their execution within an application. In the context of ASP.NET:
Synchronous programming:
- Tasks are executed sequentially, one at a time
- The next task only starts when the previous task is complete
- The application may become unresponsive or slow during long-running tasks
- Easier to understand and write code
- Increases the risk of blocking, which can lead to poor application performance
Asynchronous programming:
- Allows multiple tasks to run concurrently
- Enables the execution of other tasks while waiting for one task to complete
- Frees up resources, such as threads, and improves application responsiveness
- Can be more complex to write and understand the code
- Less risk of blocking, leading to better performance in many scenarios
To decide which approach to use in a specific situation, you would evaluate the following factors:
- Long-running tasks: If your application includes long-running tasks, such as network calls, file I/O operations, or complex calculations, using asynchronous programming can help avoid blocking resources and maintain application responsiveness.
- Concurrency requirements: If the application requires high levels of concurrency or parallelism, asynchronous programming is a better fit, as it optimizes the usage of available resources.
- Code complexity: While asynchronous programming can improve performance, it can also make the code more challenging to understand and maintain. If performance is not a critical factor and the code is easier to manage using synchronous programming, it may be an acceptable trade-off.
- Framework support: Recent versions of ASP.NET (e.g., ASP.NET Core) have built-in support for asynchronous programming, making it easier to implement.
In general, asynchronous programming is recommended when improving responsiveness and performance is a priority, particularly in applications that involve long-running tasks or high concurrency.
How can you implement custom authentication and authorization in an ASP.NET application?
Answer
Custom authentication and authorization can be implemented in an ASP.NET application by following these general steps:
- Create a custom authentication provider: Implement a custom authentication provider that inherits from the
IAuthenticationFilter
interface (in ASP.NET Web API) orIAuthorizationFilter
interface (in ASP.NET MVC). In this custom provider, you can define your authentication logic based on your requirements, such as validating credentials against a custom user store, a database, or an external service. The authentication process might involve checking a token, a username/password combination, or other means of validating a user’s identity.
Loading code snippet...
- Create a custom authorization provider: Implement a custom authorization provider that inherits from the
AuthorizeAttribute
class. In this custom provider, you can define your authorization logic based on your application’s requirements, such as checking for specific roles, permissions, or claims associated with the authenticated user.
Loading code snippet...
- Register the custom providers: Register your custom authentication and authorization providers within the ASP.NET application. This can be done by adding the custom provider as a global filter in the
FilterConfig
class for an MVC application or theHttpConfiguration
object for a Web API application.
Loading code snippet...
- Apply the custom providers: Apply your custom authentication and authorization providers to specific controllers or actions within your application by using the
[CustomAuthorize]
and[CustomAuthenticationFilter]
attributes as needed.
By following these steps, you can implement custom authentication and authorization logic in your ASP.NET application, tailored to your specific requirements and external systems.
Describe how to create and use a custom HttpHandler to process requests in an ASP.NET application.
Answer
An HttpHandler is a custom class in ASP.NET that processes HTTP requests based on URL patterns or file extensions. Creating a custom HttpHandler involves the following steps:
- Implement IHttpHandler: Create a class that implements the
IHttpHandler
interface. Implement theProcessRequest
method to define the logic for processing the request, such as generating an image or generating a dynamic response based on query parameters.
Loading code snippet...
- Register the custom HttpHandler: Register your custom HttpHandler in the
web.config
file of your application. Specify the URL pattern or the file extension that should be handled by the custom HttpHandler.
Loading code snippet...
- Use the custom HttpHandler: When a request matches the specified URL pattern or file extension, the custom HttpHandler will process the request and generate the appropriate output.
By using a custom HttpHandler, you can handle specific types of requests in your ASP.NET application and generate dynamic output tailored to your application’s requirements.
What is memory management like in ASP.NET? Discuss garbage collection and memory leak prevention strategies.
Answer
Memory management in ASP.NET, as in the .NET ecosystem in general, is based on automatic memory management and garbage collection. Here’s an overview of ASP.NET memory management and some strategies for preventing memory leaks:
- Managed memory: ASP.NET applications use managed memory, which means that the .NET runtime is responsible for allocating and deallocating memory for objects.
- Garbage collection: The .NET runtime uses a garbage collector (GC) to automatically release memory occupied by objects that are no longer needed. The GC determines whether an object is still in use by checking if there are any active references to the object. If an object has no active references, it is considered garbage and the memory is freed.
- Generations: The .NET garbage collector uses a generational approach, organizing objects into three generations (Gen 0, Gen 1, and Gen 2). Newly created objects are initially placed in Gen 0. As objects survive garbage collection cycles, they are promoted to higher generations. Higher generations are collected less frequently, improving GC performance.
Memory leak prevention strategies:
- of objects: Always dispose of objects that implement the
IDisposable
interface to release unmanaged resources, such as file handles, network connections, and database connections. Use theusing
statement to ensure proper disposal.
Loading code snippet...
- Remove event handlers: Unregister event handlers when they are no longer needed to prevent objects from being held in memory due to active event handler references.
- Avoid static references: Use static references sparingly as they persist throughout the application’s lifetime and can hold on to objects, preventing garbage collection.
- Monitor memory usage: Use performance counters and diagnostic tools (e.g., Visual Studio’s performance profiler) to monitor memory usage and identify potential memory leaks in your application.
By understanding ASP.NET memory management and implementing memory leak prevention strategies, you can optimize the memory usage and performance of your application.
Explain the differences between Serialization and Deserialization in ASP.NET. How can they be used in data handling within a web application?
Answer
Serialization and deserialization are processes used to convert data between different formats, often for the purpose of transmitting or storing data.
Serialization: The process of converting an object’s state, including its properties and field values, into a format that can be transmitted (e.g., JSON, XML) or stored (e.g., in a file or database). In ASP.NET, the DataContractJsonSerializer
, JsonConvert
(from Newtonsoft.JSON), or System.Text.Json.JsonSerializer
classes can be used for JSON serialization, while the XmlSerializer
class can be used for XML serialization.
Deserialization: The reverse process of serialization, converting the data in a serialized format back into an object’s state within an application. The DataContractJsonSerializer
, JsonConvert
, System.Text.Json.JsonSerializer
, and XmlSerializer
classes can also be used for deserialization in ASP.NET.
Serialization and deserialization can play a crucial role in handling data within a web application, such as:
- Data transmission: Serializing data before sending it to the client or another service allows for efficient data transmission in a standard format, such as JSON or XML. Deserialize the received data at the destination to convert it back into objects that can be used within the application.
- Data storage: Serialize objects before storing them in a file, database, or cache to efficiently store object states that can later be retrieved and deserialized back into objects.
- State management: Serialize objects to maintain their state across postbacks in ViewState or session state in ASP.NET Web Forms applications.
By understanding serialization and deserialization and utilizing appropriate classes in ASP.NET, you can efficiently handle data transmission, storage, and state management within your web application.
With a solid understanding of custom authentication and authorization in ASP.NET, let’s advance to more complex asp interview questions and delve into the process of creating and using custom HttpHandlers to process requests in an ASP.NET application.
How can you implement caching in an ASP.NET application, to enhance performance?
Answer
Caching in ASP.NET applications can help improve performance by reducing the workload on the server, decreasing response times, and enabling quicker access to frequently requested data. There are different types of caching available in ASP.NET:
- Output Caching: Stores the output of a page or a user control so that it can be served faster when requested again. You can enable output caching using the
OutputCache
attribute at the page or controller level.
Loading code snippet...
- Data Caching: Stores data objects, such as the results of a database query, in memory for faster access. You can use the
MemoryCache
class to store and retrieve data in memory.
Loading code snippet...
- Distributed Caching: Stores cached data in an external cache server or a cache cluster (e.g., Redis or Memcached) for distributed and scalable caching. You can use the
IDistributedCache
interface with various providers in ASP.NET Core or theCache
object in ASP.NET with custom providers.
Loading code snippet...
- Cache Profiles: Define reusable cache settings (e.g., duration, location) in one place (
web.config
for ASP.NET orappsettings.json
for ASP.NET Core) and apply them to your controllers or pages using cache profile names.
To enhance performance in your ASP.NET application, choose the appropriate type of caching based on your requirements and implement caching mechanisms using the available attributes, classes, or third-party libraries. Remember to consider cache expiration and data consistency when implementing caching scenarios.
What is the difference between WebForms and MVC in ASP.NET? Compare their components and advantages.
Answer
WebForms and MVC are two different programming models for building web applications in ASP.NET. They have different approaches, components, and advantages:
ASP.NET WebForms:
- Event-driven programming model
- Rich server-side control library with built-in state management
- ViewState for maintaining control state across postbacks
- Uses a code-behind model for separating markup and logic
- Relies on server-side form processing and lifecycle events
- Generally easier to learn for developers with a background in desktop applications
Advantages:
- Rapid application development with built-in server controls
- Abstraction of complex client-side scripting and state management
- Familiar programming model for developers transitioning from desktop applications
ASP.NET MVC:
- Model-View-Controller (MVC) programming model
- Clear separation of concerns (SoC) between data (Model), presentation (View), and control (Controller)
- Supports routing and friendly URLs
- Encourages test-driven development (TDD) with easy-to-test components
- Better control over HTML output and client-side scripting
Advantages:
- Improved testability due to clear separation of concerns
- Greater control over markup and output, which can help with SEO and client-side scripting
- Encourages best practices, such as modularity, design patterns, and dependency injection
In summary, WebForms is better suited for rapid application development and developers familiar with desktop development, while MVC is more suited for web developers who prioritize testability, separation of concerns, and greater control over HTML and client-side scripting.
How would you handle cross-site scripting (XSS) and SQL injection attacks in an ASP.NET application?
Answer
Cross-site scripting (XSS) and SQL injection are common security threats in web applications. To handle these threats in an ASP.NET application:
Preventing XSS attacks:
- Encode output: Encode user-generated content that is displayed on the web page, effectively neutralizing potential XSS attacks. Use the
Html.Encode()
method in Razor views or theServer.HtmlEncode()
in WebForms to encode output. - Validate input: Validate and sanitize user input on both the client and server sides, ensuring that potentially malicious content is filtered or rejected.
- Use anti-forgery tokens: In form submissions, use anti-forgery tokens (
AntiForgeryToken
in MVC orViewStateUserKey
in WebForms) to prevent malicious users from tricking users into submitting forged requests.
Preventing SQL injection attacks:
- Use parameterized queries: Always use parameterized queries, prepared statements, or the Entity Framework (ORM) when dealing with dynamic SQL queries to prevent attackers from injecting malicious SQL code.
- Validate and sanitize input: Validate user input against a predefined set of rules (e.g., length, characters allowed) and sanitize the input by removing or escaping potentially harmful characters.
- Limit database privileges: Use the principle of least privilege when setting up database access for your application, granting only the necessary permissions required to perform specific tasks.
By implementing these best practices in your ASP.NET application, you can significantly reduce the risk of XSS and SQL injection attacks.
Explain the concept of connection pooling in ASP.NET. How can you configure and manage it to optimize database access performance?
Answer
Connection pooling is a technique used in ASP.NET to optimize database access performance by reducing the overhead of opening and closing connections. A connection pool is a collection of database connections that are created and maintained for reuse by the application.
When an application requests a connection, it can use an existing (idle) connection from the pool if available, or create a new one if the pool hasn’t reached its maximum size. When the application releases the connection, it is returned to the pool, becoming available for other requests.
To manage and configure connection pooling in ASP.NET, you can follow these steps:
- Enable connection pooling: Connection pooling is enabled by default in ADO.NET when using SqlConnection or other managed providers. You don’t need to enable it explicitly.
- Configure connection string: Edit the connection string in your
web.config
orappsettings.json
to specify connection pool settings, such asMinPoolSize
,MaxPoolSize
, andConnectionLifetime
.
Loading code snippet...
- Best practices: Follow best practices for using connection pooling, such as:
- Open and close connections as late and as soon as possible, ensuring they are returned to the pool for reuse.
- Use the
using
statement to ensure that connections are properly closed and disposed of, even in case of an exception.
Loading code snippet...
By managing connection pooling efficiently in your ASP.NET application, you can optimize database access performance and resource utilization.
How to implement role-based security in ASP.NET using the Role Manager API.
Answer
Role-based security in ASP.NET allows you to restrict access to specific parts of your application based on user roles. Using the Role Manager API, you can create and manage roles and associate them with users:
- Enable Role Manager: Enable Role Manager in your web application by adding the following configuration to the
web.config
file:
Loading code snippet...
- Create and manage roles: Use the Role Manager API to create, delete, and manage roles within your application:
Loading code snippet...
- Authorize access based on roles: Use the
Authorize
attribute to restrict access to specific actions or controllers in an MVC application, or use theasp:LoginView
control in a WebForms application.
For MVC:
Loading code snippet...
For WebForms:
Loading code snippet...
- Check roles programmatically: Use the
User.IsInRole()
method to check if the current user is a member of a specific role and display content or execute logic accordingly:
Loading code snippet...
By using the Role Manager API, you can implement role-based security in your ASP.NET application, effectively managing access to resources and functionality based on user roles.
Memory management is a crucial aspect to consider in any ASP.NET application. As we discuss garbage collection and memory leak prevention strategies, we also pave the way for more intriguing asp net developer interview questions, focusing on serialization and deserialization.
Describe the concepts of master pages and themes in ASP.NET. What benefits do they provide for designing and maintaining a website’s layout and appearance?
Answer
Master pages and themes are two concepts in ASP.NET that help you design and maintain your website’s layout and appearance more efficiently.
Master Pages:
- Provide a consistent layout for multiple pages in your web application.
- Allow for the definition of placeholders (called content placeholders) where individual content pages can inject their specific content.
- Help in maintaining the overall website layout in one place, making updates or changes to the layout much easier and faster.
- Can be nested, allowing different levels of layout inheritance.
Example of a master page with a content placeholder:
Loading code snippet...
Example of a content page using the master page:
Loading code snippet...
Themes:
- Define a set of CSS styles, images, and controls that can be applied to multiple pages or the entire web application.
- Help you separate the styling from the content and create a consistent look and feel across your web application.
- Can be easily switched to quickly change the appearance of your website.
- Consist of multiple skin files for different controls, CSS files, and other resources (such as images).
To use themes, store them in the App_Themes
folder in your web application and apply the theme in your web.config
or page directives:
Loading code snippet...
By using master pages and themes in your ASP.NET application, you can create a consistent layout and appearance for your website, helping you maintain and update your web application more efficiently.
Which dependency injection frameworks are available for use with ASP.NET, and what are the advantages and disadvantages of each?
Answer
Dependency injection (DI) frameworks help you manage dependencies between objects in your web application, making it more modular, testable, and maintainable. There are several DI frameworks available for use with ASP.NET:
Unity Container (by Microsoft)
- Advantages:
- Disadvantages:
Autofac:
- Advantages:
- Disadvantages:
Ninject:
- Advantages:
- Disadvantages:
Castle Windsor Container:
- Advantages:
- Disadvantages:
Built-in Dependency Injection (ASP.NET Core):
- Advantages:
- Disadvantages:
Each DI framework has its own advantages and disadvantages, and the choice depends on your specific requirements, such as feature set, performance, and ease of use. If you are using ASP.NET Core, the built-in DI system is often a good starting point.
Describe the differences between the DataSet and DataReader objects in ADO.NET.
Answer
ADO.NET provides two primary objects for working with data from a database: DataSet
and DataReader
. They have different characteristics, use cases, and performance implications:
DataSet:
- Disconnected, in-memory representation of data (tables, relationships, and constraints)
- Provides a full set of CRUD (create, read, update, delete) operations on the data
- Can query, filter, and sort data without interacting with the database
- Can store multiple tables and establish relationships between them
- Consumes more memory, as it holds the entire data set in memory
- Useful in situations where you need to work with data offline or manipulate data in complex ways
DataReader:
- Connected, forward-only, read-only stream of data from the database
- Provides high performance and low memory consumption for reading large amounts of data
- Requires an open database connection while reading data
- Suitable for scenarios where you need to access and display data quickly, without the need for complex data manipulation or offline caching
In summary, use DataSet
when you need a disconnected, in-memory representation of data with support for complex manipulations (e.g., filtering, sorting, relationships). Use DataReader
when you prioritize performance and need to read large amounts of data quickly, without the need for complex operations or offline caching.
How would you implement localization and globalization in an ASP.NET application? Include an explanation of using resource files.
Answer
Localization and globalization are concepts used to tailor a web application’s content, formatting, and functionality to different cultures, languages, and regions. In ASP.NET applications, you can implement localization and globalization using resource files, culture settings, and formatting.
Resource Files:
- Store localized content, such as strings and images, for different languages and cultures in
.resx
files. - Create a default resource file (e.g.,
Resources.resx
) and additional resource files for each culture you want to support (e.g.,Resources.fr-FR.resx
). - Access localized resources using the
ResourceManager
class or the.GetGlobalResourceObject()
and.GetLocalResourceObject()
methods in ASP.NET.
Example of using resources in an ASP.NET MVC view:
Loading code snippet...
Culture Settings:
- Define and apply culture settings for number formatting, date and time formatting, and other culture-specific settings.
- Set the culture for your web application in the
web.config
file or programmatically using theCultureInfo
class and theThread.CurrentThread.CurrentCulture
andThread.CurrentThread.CurrentUICulture
properties.
Example of setting culture programmatically:
Loading code snippet...
Formatting:
- Use the
CultureInfo
class and .NET formatting methods (such asToString()
) to display numbers, dates, and other values in a culture-specific format.
Example of formatting a date using the current culture:
Loading code snippet...
By using resource files, culture settings, and formatting, you can implement localization and globalization in your ASP.NET application to support different languages, cultures, and regions.
Compare and contrast ASP.NET Web API and ASP.NET MVC. Which would be more appropriate for developing a RESTful API?
Answer
ASP.NET Web API and ASP.NET MVC are both web application frameworks in the ASP.NET ecosystem. They have similarities and differences, and their appropriateness for developing a RESTful API depends on your requirements and priorities.
ASP.NET Web API:
- Designed specifically for building RESTful services and APIs
- Supports content negotiation and various data formats (e.g., JSON, XML) out of the box
- Optimized for HTTP services and follows the HTTP protocol more closely (e.g., more granular control over HTTP methods, response codes, and headers)
- Provides better support for versioning and routing
- Offers IHttpActionResult for better flexibility in generating HTTP responses
ASP.NET MVC:
- Designed for building web applications with an emphasis on the Model-View-Controller (MVC) pattern
- Can create RESTful services and APIs using controller actions, but not specifically designed for that purpose
- Returns ActionResult from controller actions, which can be less flexible for generating HTTP responses compared to IHttpActionResult
- Primarily focused on rendering HTML views, but can also return JSON, XML, or other data formats using ActionResult
Considering the above points, ASP.NET Web API is the more appropriate choice for developing a RESTful API, as it is specifically designed for that purpose and provides better support for HTTP services, content negotiation, and versioning when compared to ASP.NET MVC. However, if you have an existing MVC application or want to develop a web application with both UI and API functionality, you can still build a RESTful API using ASP.NET MVC.
It’s time to explore the world of caching! In the next few asp net questions, we’ll discuss the importance of caching in enhancing website performance, followed by an in-depth analysis of the differences between WebForms and MVC in ASP.NET.
What is the significance of HttpHandlers and HttpModules in an ASP.NET application? Explain with examples.
Answer
HttpHandlers and HttpModules are essential components in ASP.NET applications that allow you to handle and process incoming HTTP requests and provide extensible mechanisms for customizing how your application responds to requests.
HttpHandlers:
- Implement the
IHttpHandler
interface - Process incoming HTTP requests and generate appropriate HTTP responses
- Handle specific file types and URL patterns, providing a way to customize your application’s behavior for different resource types
- Examples include
.aspx
pages (a built-in HttpHandler), image manipulation handlers, and download handlers
Example of a custom HttpHandler for processing image requests:
Loading code snippet...
HttpModules:
- Implement the
IHttpModule
interface - Provide a way to intercept and modify an HTTP request at different points in the request lifecycle (e.g., authentication, authorization, caching)
- Can be chained together, allowing you to build a pipeline of custom features and processing
- Examples include URL rewriting modules, custom logging modules, and error handling modules
Example of a custom HttpModule for logging incoming requests:
Loading code snippet...
In summary, HttpHandlers and HttpModules give you the flexibility to customize how your ASP.NET application processes and generates HTTP responses for different resources and at various points in the request lifecycle.
Describe the differences between early binding and late binding in ASP.NET, and which one is more efficient.
Answer
Early Binding:
- Type information is known at compile-time
- Directly creates instances of objects, calls methods, and accesses properties using their exact data types
- Provides better performance, type checking, and IntelliSense support in development environments
- Requires referenced assemblies to be available at compile time
Example of early binding:
Loading code snippet...
Late Binding:
- Type information is determined at runtime
- Creates instances of objects, calls methods, and accesses properties using reflection
- Gives more flexibility in working with types that are not known or available at compile time, but may come from external sources (e.g., plugins)
- Has a slower performance compared to early binding, as it requires additional runtime type checks and casting
Example of late binding:
Loading code snippet...
Regarding efficiency, early binding is generally more efficient in terms of performance, type safety, and development experience. However, there are situations where late binding is necessary or useful, such as when working with dynamic types, plugins, or COM objects that are not available at compile time.
Explain how to create custom error pages for specific HTTP status codes in an ASP.NET application.
Answer
Creating custom error pages in an ASP.NET application helps you provide a better user experience and display useful information to users when an error occurs. You can create custom error pages for specific HTTP status codes using the following approach:
- Create custom error page(s): Design your error pages with helpful information based on the HTTP status code (e.g., a 404 Not Found error page should indicate that the requested resource was not found).
Example of a custom 404 error page (in Custom404.aspx
):
Loading code snippet...
- Configure error page handling: Update the
web.config
file to specify the custom error pages for the desired HTTP status codes.
Loading code snippet...
- Handle errors programmatically (optional): In some cases, you may want to handle specific errors at different application levels (
Page
,Controller
, orGlobal
), using theOnError
event,HandleError
attribute, orApplication_Error
method.
Example of handling a 403 (Forbidden) error in the Global.asax
file and redirecting to a custom error page:
Loading code snippet...
By following these steps, you can create and configure custom error pages in your ASP.NET application for specific HTTP status codes, providing a user-friendly experience when errors occur.
How can you optimize an ASP.NET application to run efficiently in a mobile environment?
Answer
Optimizing your ASP.NET application to run efficiently in a mobile environment involves improving performance, reducing network latency, and providing a responsive user interface. Some techniques for achieving this include:
- Responsive design: Use responsive web design (RWD) practices to ensure that your web application looks and works well across various devices, screen sizes and resolutions. Use CSS media queries, mobile-first design, and a fluid grid layout to adapt your UI to different screen sizes.
- Adaptive rendering: Use built-in ASP.NET features like device filtering and view switching to serve different content, layouts, or views based on the user’s device and capabilities.
- Optimize images: Compress and resize images to reduce file size and load times. Use techniques like lazy loading and progressive image loading to improve perceived performance.
- Minify and bundle resources: Minify and bundle CSS, JavaScript, and other static files to reduce their size and the number of HTTP requests.
- Enable caching: Cache static resources, partial views, and data to reduce the load on your server and improve response times.
- Optimize server-side processing: Improve the performance of your server-side code using efficient data retrieval and processing, asynchronous programming, and output caching.
- Use Content Delivery Network (CDN): Utilize a CDN to serve static resources (like images, CSS and JavaScript files) to users from servers that are geographically closer to them, reducing latency.
- Analyze and monitor performance: Use tools like Google PageSpeed Insights, YSlow, and browser developer tools to analyze and monitor your application’s performance, identify bottlenecks and areas for improvement.
By implementing these optimization techniques, you can improve your ASP.NET application’s performance and user experience in a mobile environment.
What is a sessionless controller in ASP.NET MVC, and when would you use it?
Answer
A sessionless controller in ASP.NET MVC is a controller that explicitly disables session state functionality for all of its actions. This is useful in scenarios where you don’t need to access session data and want to improve performance by avoiding the overhead associated with session state management.
To create a sessionless controller, apply the SessionStateAttribute
to the controller class and set its behavior property to SessionStateBehavior.Disabled
:
Loading code snippet...
Use a sessionless controller when:
- You don’t need to access or modify session data in the controller’s actions
- You want to improve performance, particularly in scenarios with high concurrent user load, since disabling session state allows for better request handling and prevents session state-related bottlenecks
- You are developing a RESTful API where session state is generally not required, and should be avoided, to maintain stateless communication
By using a sessionless controller in these situations, you can improve your ASP.NET MVC application’s performance and resource utilization while maintaining the stateless nature of your web services or APIs.
Security is paramount in any web application development process. As we learn to handle cross-site scripting (XSS) and SQL injection attacks in ASP.NET, we’ll further enrich our asp net interview questions and answers pdf by tackling connection pooling concepts to optimize database access performance.
Explain the process of implementing optimistic concurrency in an ASP.NET web application using entity framework.
Answer
Optimistic concurrency is a strategy used to handle conflicts when multiple users update the same data simultaneously. In this approach, the system allows concurrent data modifications but checks whether any conflicts have occurred during updates. If a conflict is detected, the system presents an error message and requires the user to resolve the conflict.
The following steps outline the process of implementing optimistic concurrency using Entity Framework in an ASP.NET web application:
- Add a concurrency token: Add a property to your entity class that will be used to track changes for concurrency control. This is typically a timestamp or a row version column. Enable the property as a concurrency token by adding the
[ConcurrencyCheck]
or[Timestamp]
attribute.
Loading code snippet...
- Enable optimistic concurrency for updates: When updating the data, use the
DbContext.SaveChanges()
method wrapped in a try-catch block to handleDbUpdateConcurrencyException
exceptions. This will allow you to detect the concurrency conflicts and take appropriate action.
Loading code snippet...
- Handle concurrency exceptions: In the catch block for
DbUpdateConcurrencyException
, retrieve the current data from the database and compare it with the data submitted by the user. If any differences are found, present them to the user and require a decision to overwrite the current data or refresh the form with the updated data.
Loading code snippet...
By following these steps, you can implement optimistic concurrency in your ASP.NET web application using Entity Framework, allowing for better handling of concurrent data updates and conflict resolution.
Describe the differences between server-side, client-side, and AJAX-based validation in an ASP.NET application.
Answer
Validation is a crucial part of any web application to ensure that the data entered by users is valid and conforms to the required format. In ASP.NET applications, validation can be performed at different levels, including server-side, client-side, and AJAX-based validation:
Server-side validation:
- Occurs on the server after the form data has been submitted
- Involves validating the input data in the server-side code (e.g., controller actions or business logic layer)
- Is mandatory, as it is the last line of defense to prevent invalid or malicious data from being processed
- Provides more control and flexibility, but increases server load and may lead to a slower user experience due to full postbacks
Client-side validation:
- Occurs in the user’s browser using JavaScript before the form data is submitted
- In ASP.NET, it can be enabled using the built-in validation controls (e.g.,
RequiredFieldValidator
,RegularExpressionValidator
) and unobtrusive JavaScript - Provides a better user experience as it prevents unnecessary server requests for invalid data and gives real-time feedback
- Is not a substitute for server-side validation, as client-side scripts can be bypassed or manipulated by malicious users
AJAX-based validation:
- Combines the advantages of both server-side and client-side validation
- Involves making asynchronous requests to the server without a full postback, allowing the execution of server-side validation rules while providing a seamless and responsive user experience
- Can be implemented using jQuery AJAX, partial views, or built-in AJAX controls (e.g.,
UpdatePanel
,ValidationSummary
) - Offers the reliability of server-side validation combined with the responsiveness of client-side validation
In summary, server-side validation is essential for data integrity and security, while client-side validation aims to improve the user experience by providing real-time feedback. AJAX-based validation combines the best of both worlds, offering a responsive user experience with reliable server-side validation. It is recommended to use a combination of server-side and client-side (or AJAX-based) validation to provide a seamless and secure user experience in ASP.NET applications.
Explain the benefits and limitations of using ASP.NET Web Forms for developing web applications.
Answer
ASP.NET Web Forms is a framework for building web applications using a design pattern similar to traditional desktop applications, with components like controls and events. It has been part of the ASP.NET framework since its inception.
Benefits:
- Rapid development: The drag-and-drop visual designer and rich set of built-in controls make it easy to create complex web applications quickly, even for developers without extensive web development experience.
- State management: Web Forms includes various state management features like ViewState, session state, and application state, which simplify maintaining state between postbacks.
- Event-driven programming: Web Forms uses an event-driven programming model that makes it easy to handle user interactions and respond to events triggered by server controls.
- Large community: ASP.NET Web Forms has been around for quite some time, which has led to a large community, extensive documentation, and numerous third-party libraries and tools that can enrich the development process.
Limitations:
- Limited control over HTML and JavaScript: Web Forms often generate bloated and non-semantic HTML, and the resulting JavaScript can be difficult to maintain. Moreover, it may be challenging to implement custom client-side scripts or more modern web development techniques, such as AJAX calls.
- Performance issues: ViewState can grow large and result in slow page load times and increased server load. The postback model also generates additional server roundtrips and can lead to a less responsive user experience.
- Less separation of concerns: Web Forms tightly couples business logic to the UI, making it more challenging to separate concerns, test, and maintain the application.
- Less flexibility: As Web Forms follow a more restrictive development pattern with less control over the generated HTML and scripts, it can be harder to implement custom solutions or adopt modern web development best practices.
Despite its limitations, ASP.NET Web Forms remains a popular choice for developers coming from a desktop application background or preferring a more rapid development process. However, the introduction of alternative frameworks like ASP.NET MVC and ASP.NET Core has provided modern architectures and additional flexibility that has made Web Forms increasingly less popular for new projects.
What are the core components of the ASP.NET Web API pipeline?
Answer
ASP.NET Web API is a framework for creating HTTP services that can be consumed by a broad range of clients, including browsers and mobile devices. The Web API pipeline is a series of components that process incoming HTTP requests and generate corresponding HTTP responses. The core components of the pipeline include:
- Routing: Routing is responsible for matching incoming HTTP requests to the appropriate action methods in the Web API controllers. It uses route templates defined in the configuration to determine the controller, action, and parameters.
- Controllers: Controllers are the Web API classes that implement the logic for processing HTTP requests. They inherit from the ApiController class and contain action methods to handle HTTP verbs like GET, POST, PUT, and DELETE.
- Model Binding: Model binding is responsible for extracting data from the HTTP request and creating model objects that can be used as action method parameters. It supports a variety of sources, including route data, query strings, and request bodies.
- Action Filters: Action filters are custom attributes that can be applied to action methods or entire controllers to perform additional processing or modify the execution pipeline. Examples include authentication and authorization, action logging, and caching.
- Content Negotiation: Content negotiation is the process of determining the best response format (e.g., JSON, XML) based on the client’s Accept header and the server’s capabilities. Web API uses media type formatters (e.g., JsonMediaTypeFormatter, XmlMediaTypeFormatter) to serialize and deserialize data in the appropriate format.
- Exception Handling: Exception handling allows you to catch and handle errors that may occur during request processing, providing a consistent way to return error information to the client. You can use built-in exception filters or create custom exception handlers to handle and log exceptions.
- Message Handlers: Message handlers are components derived from the
DelegatingHandler
class that can intercept and process HTTP messages (i.e., requests and responses) at the lowest level of the pipeline. They can be used for cross-cutting concerns like request/response logging, authentication, or custom HTTP headers.
By understanding and utilizing these core components, you can create a flexible and extensible HTTP service using the ASP.NET Web API framework.
In an ASP.NET application, how can you minimize the impact of cross-origin resource sharing (CORS) security risks?
Answer
Cross-Origin Resource Sharing (CORS) is a browser security mechanism that restricts web pages from making requests to a domain different from the one that served the web page. While it is an essential security feature, it can cause issues for legitimate cross-origin requests in web applications.
In an ASP.NET application, you can minimize the impact of CORS security risks by following these steps:
- Enable CORS: To allow cross-origin requests in an ASP.NET application, enable CORS by adding a
Cors
package via NuGet (Microsoft.AspNet.WebApi.Cors for Web API, or Microsoft.AspNet.Cors for ASP.NET Core). - Configure CORS policy: Define a CORS policy in the
WebApiConfig.cs
(for Web API) orStartup.cs
(for ASP.NET Core) file to specify which origins, headers, and methods are allowed in cross-origin requests.
Example of configuring a CORS policy in a Web API application:
Loading code snippet...
Example of configuring a CORS policy in an ASP.NET Core application:
Loading code snippet...
- Limit access: Avoid using wildcard
*
for allowed origins. Instead, specify a list of trusted origins that are allowed to make cross-origin requests to your application. - Restrict allowed methods: Limit the allowed HTTP methods to only those required by your application. This reduces the attack surface and helps protect against potential CORS exploits.
- Implement additional security measures: Use other security practices like CSRF tokens, OAuth2, and HTTPS to provide additional layers of protection for your application.
By following these steps, you can minimize the impact of CORS security risks in your ASP.NET application while enabling legitimate cross-origin requests.
Master pages and themes offer a multitude of benefits for designing and maintaining a website’s layout and appearance. As we explore these concepts, we’ll also begin to dive into the intriguing world of dependency injection frameworks for ASP.NET, making it a valuable addition to our asp net c# interview questions collection.
Describe the differences between TempData, ViewData, and ViewBag in ASP.NET MVC.
Answer
In ASP.NET MVC, TempData, ViewData, and ViewBag are used to store and pass data between controllers, actions, and views. They offer different ways to achieve the same goal but have some differences in terms of their purpose, lifecycle, and usage.
TempData:
- It is a dictionary that allows data to be stored and passed between consecutive requests during a single user session.
- TempData uses session state to store data.
- Its primary and intended use is to pass data between different controller actions or between multiple requests for the same action, known as “PRG Pattern” (Post-Redirect-Get).
- TempData persists data only for the current and subsequent request, after which the data is removed automatically.
ViewData:
- It is a dictionary that allows data to be stored and passed from the controller to the view within a single request.
- ViewData uses the
ViewDataDictionary
class for storing data. - It has a short lifecycle, as the data is removed once it is passed to the view and the view is rendered.
- Strongly typed views cannot use ViewData directly.
ViewBag:
- ViewBag is a dynamic object that allows data to be stored and passed from the controller to the view within a single request.
- It is, in fact, a wrapper around ViewData. ViewBag internally uses ViewData, but it allows developers to use dynamic properties instead of the dictionary structure.
- Similar to ViewData, it has a short lifecycle.
- ViewBag provides more flexibility and simplified syntax compared to ViewData, but it doesn’t support type checking or intellisense in Visual Studio.
In summary, TempData is used for passing data between requests or actions, whereas ViewData and ViewBag are used for passing data from the controller to the view within a single request. While TempData is a dictionary and relies on session state, ViewData is a dictionary of objects and ViewBag is a dynamic wrapper around ViewData.
Explain the process of implementing a three-tier architecture using ASP.NET.
Answer
A three-tier architecture is a software design pattern that separates an application into three logical layers, making the application more modular, scalable, and maintainable. The three layers are the presentation layer, business logic layer (BLL), and data access layer (DAL). In an ASP.NET application, the implementation of a three-tier architecture typically follows these steps:
- Presentation layer: The presentation layer corresponds to the ASP.NET web application, which can be an MVC application, Web Forms application, or Razor Pages application. This layer is responsible for displaying the user interface, handling user input, and communicating with the business logic layer. The user interface is implemented using HTML, CSS, JavaScript, and other client-side technologies.
- Business logic layer (BLL): The business logic layer is implemented using a separate class library project in the solution. This layer contains the core domain models, services, and business rules for the application. The presentation layer communicates with the BLL using dependency injection or service locator pattern, and the BLL handles the application’s workflows and decision-making processes.
- Data access layer (DAL): The data access layer is also implemented using a separate class library project. The DAL is responsible for interacting with the underlying data stores (e.g., databases, file systems, or web services). The data access logic is decoupled from the business logic layer so that data sources can be changed or extended without affecting the BLL or presentation layer. The DAL can be implemented using technologies like ADO.NET, Entity Framework, or custom data access components.
To implement a three-tier architecture in an ASP.NET application, follow these steps:
- Create a solution with three separate projects – one for the presentation layer (ASP.NET web application), one for the business logic layer (class library), and one for the data access layer (class library).
- Define the interfaces and classes for the business logic layer and data access layer.
- Implement the business logic and data access methods in their respective projects.
- In the presentation layer, instantiate and utilize the services from the business logic layer to perform the required actions or retrieve data based on user input.
- Configure the dependency injection mechanism (e.g., using built-in DI in ASP.NET Core or third-party libraries like Autofac or Ninject) to resolve the dependencies between the layers.
By following this process, you can successfully implement a three-tier architecture in your ASP.NET application, resulting in a more maintainable, scalable, and modular application.
How can you ensure that sensitive information, such as passwords and encryption keys, is securely stored in an ASP.NET application?
Answer
Storing sensitive information like passwords, encryption keys, or connection strings securely is vital for protecting your ASP.NET application and user’s data. To ensure the secure storage of sensitive information, you can follow these best practices:
- Use encryption: Encrypt sensitive data before storing it to prevent unauthorized access or tampering. You can use built-in encryption libraries like AesManaged, DataProtection, or other third-party encryption libraries.
- Secure configuration: Store sensitive information, such as connection strings and API keys, in encrypted format within configuration files (e.g.,
appsettings.json
,web.config
) and use the built-inConfiguration
API to access them. In ASP.NET Core, you can use theIConfiguration
interface or create strongly typed configuration objects using theIOptions
pattern. - User secrets: When working with local development environments, ensure that sensitive information is not accidentally committed to the source code repository. In ASP.NET Core, you can store sensitive data in the “user secrets” storage, a secure key-value store specifically designed for local development.
- Hash passwords: For storing user passwords, don’t store plain-text passwords; instead, use one-way hash functions (e.g., BCrypt, SCrypt, or Argon2) combined with a unique salt value per password to ensure their security even if the database is compromised.
- Use secure key management practices: Follow best practices for managing encryption keys, which include using strong keys, periodic key rotation, and secure storage of keys (e.g., Azure Key Vault, AWS Key Management Service).
- Implement proper access controls: Ensure that only authorized personnel have access to the sensitive data, limit access to a need-to-know basis, and regularly review and update permissions.
By following these best practices, you can ensure that sensitive information is securely stored in your ASP.NET application and minimize the risks associated with data breaches or other security incidents.
What is the role of a virtual path provider in an ASP.NET application, and when would you use it?
Answer
A virtual path provider is a feature in ASP.NET that enables you to serve content (e.g., web pages, images, and stylesheets) to the application from custom locations, other than the default file system. The virtual path provider acts as an abstraction layer between the web application and the content storage, allowing you to serve content from alternative sources such as databases, remote servers, or embedded resources.
Some common scenarios when using a virtual path provider can be beneficial are:
- Content management systems: A CMS might store content in a database, allowing editors to modify the content without modifying the application’s file system. A virtual path provider can be used to serve content directly from the database to the web application.
- Distributed applications: In a distributed application with multiple instances, storing and serving shared content becomes tricky. A virtual path provider can allow instances to access and serve common content from a centralized location, ensuring consistency across multiple instances.
- Embedded resources: When deploying a self-contained application, embedding resources (e.g., images, stylesheets) directly within the application’s assembly can simplify the deployment process. A virtual path provider can be used to serve these embedded resources as if they were files in the file system.
To implement a custom virtual path provider in an ASP.NET application, follow these steps:
- Create a class derived from
VirtualPathProvider
or one of its derived classes (e.g.,VirtualFile
orVirtualDirectory
). - Override the methods required for your custom storage mechanism, such as
GetFile
,GetDirectory
,FileExists
, orDirectoryExists
. - Register the custom virtual path provider in the
Global.asax
file orStartup.cs
file, using theHostingEnvironment.RegisterVirtualPathProvider
method.
By implementing a virtual path provider, you can serve content from custom locations and storage mechanisms, providing more flexibility and opportunities for unique application scenarios.
How do you implement a Custom Model Binder and Value Provider in an ASP.NET MVC application?
Answer
A custom model binder and value provider can be used in an ASP.NET MVC application to customize the process of binding incoming HTTP request data to action method parameters or model properties.
To implement a custom model binder, follow these steps:
- Create a class that implements the
IModelBinder
interface:
Loading code snippet...
- Implement the
BindModel
method within the custom model binder class to define the custom binding process, which may involve retrieving and converting values from the request using a custom value provider or other sources:
Loading code snippet...
- Register the custom model binder in the
Global.asax
file orStartup.cs
file:
Loading code snippet...
To implement a custom value provider, follow these steps:
Optional but generally good practice when implementing a custom model binder.When you only need to read data from a custom source and still use built-in model binding process.
1. Create a class that implements the IValueProvider
interface:
Loading code snippet...
2. Implement the ContainsPrefix
and GetValue
methods within the custom value provider class to define the process of retrieving values from the custom data source:
Loading code snippet...
3. Create a class that implements the `ValueProviderFactory` interface and includes the logic for instantiating the custom value provider:
Loading code snippet...
4. Register the custom value provider factory in the `Global.asax` file or `Startup.cs` file:
Loading code snippet...
By implementing a custom model binder and value provider, you can customize the process of binding request data to action method parameters or model properties, allowing for more flexibility and control over the data binding process in an ASP.NET MVC application.
Dataset and DataReader objects play critical roles in ADO.NET. Let’s take the time to understand their fundamental differences and continue enriching our asp dot net interview questions with an insightful discussion on localization, globalization, and resource files.
Describe the use of asynchronous controllers in ASP.NET MVC and the benefits they provide.
Answer
Asynchronous controllers in ASP.NET MVC are designed to handle long-running requests or requests that involve time-consuming I/O operations (e.g., database access, web service calls, or file I/O). By performing these operations asynchronously, you can prevent the application from blocking or waiting, which can significantly improve the application’s responsiveness, scalability, and resource utilization.
To create an asynchronous controller in ASP.NET MVC, follow these steps:
- Inherit your controller class from
AsyncController
instead ofController
.
Loading code snippet...
- Create asynchronous action methods by using
async
andTask<ActionResult>
as the return type and theawait
keyword for invoking asynchronous operations. Use theHttpClient
,Task.Run
, or other asynchronous APIs to perform I/O-bound or time-consuming operations.
Loading code snippet...
The benefits of using asynchronous controllers in an ASP.NET MVC application include:
- Improved responsiveness: As asynchronous actions do not block the main thread, the application remains responsive and can handle other incoming requests even when an asynchronous operation is in progress.
- Better resource utilization: Asynchronous controllers use fewer threads and release the server resources back to the thread pool when they are waiting for I/O-bound or time-consuming operations. This allows the application to handle more concurrent requests with the same resources.
- Scalability: The efficient use of resources and improved responsiveness result in better overall scalability of the application, particularly when handling long-running or I/O-bound request processing.
In summary, asynchronous controllers in ASP.NET MVC provide a convenient way to handle long-running requests or I/O-bound operations without blocking the application, resulting in improved responsiveness, better resource utilization, and increased scalability.
How can you implement a real-time notification system in an ASP.NET application using SignalR?
Answer
SignalR is a library for ASP.NET that enables real-time, bidirectional communication between clients (web browsers) and servers using WebSockets or other fallback transport mechanisms. SignalR can be used to implement a real-time notification system for an ASP.NET application when you need to push notifications to connected clients in response to server-side events or changes.
To implement a real-time notification system using SignalR, follow these steps:
- Install the SignalR package for your ASP.NET application using NuGet:
Loading code snippet...
- Create a SignalR Hub class, which defines the methods and events that clients can call or subscribe to:
Loading code snippet...
- Add a mapping to the
RouteTable
to configure the URL routing for the SignalR hubs:
Loading code snippet...
- Add the necessary JavaScript references to the SignalR library and hub scripts in your HTML/View files:
Loading code snippet...
- Create a JavaScript client that connects to the SignalR Hub and subscribes to the
ReceiveNotification
event:
Loading code snippet...
- Invoke the
SendNotification
method on the server-side when you need to broadcast notifications to connected clients:
Loading code snippet...
By following these steps, you can implement a real-time notification system in your ASP.NET application using SignalR, allowing you to push notifications to connected clients in response to server-side events or changes.
What are the different types of caching available in ASP.NET? Compare their advantages and disadvantages.
Answer
Caching is an essential technique for optimizing application performance in ASP.NET by temporarily storing frequently accessed or computationally expensive data to reduce database or service calls and improve response times. The main types of caching available in ASP.NET are:
- Output Caching: Output caching stores the whole rendered HTML output of a page or partial page (User control) to the server’s memory, reducing the need for the server to recreate the same content repeatedly for multiple requests. Output caching can be implemented using the
OutputCache
attribute on a Controller’s action method (or at the Controller level) in MVC applications or using theOutputCache
directive on a Web Forms page.
- Advantages: Easy to implement, reduces server processing overhead, and suitable for static or infrequently changing content.
- Disadvantages: Not suitable for personalized or frequently changing content, consumes server memory, and lacks more granular control over cached data.
- Data Caching: Data caching refers to storing application-specific data in memory on the server for quick access. It can be implemented using the
MemoryCache
class in ASP.NET Core or theHttpRuntime.Cache
property in older versions of ASP.NET.
- Advantages: Provides greater control over what to cache and cache eviction policies, suitable for caching data that is expensive to compute or fetch, such as database query results or API response data.
- Disadvantages: Requires manual management of the cache (e.g. insertion, retrieval, and eviction), and consumes server memory.
- Distributed Caching: Distributed caching stores the cached data across multiple servers or in separate cache storage. This allows the cache to be shared across multiple instances of an application, ideal for load-balanced or distributed environments. Distributed caching can be implemented using cache providers like Redis, Memcached, or NCache.
- Advantages: Scalable, supports cache sharing across multiple instances, reduces the risk of data loss due to server failures, and does not consume server memory.
- Disadvantages: Additional complexity and configuration required, network latency when accessing the cache, and potential additional costs for using external caching services.
In summary, each caching type has its advantages and disadvantages. Output caching is the best choice for static or infrequently changing content, data caching is suited for application-specific data that is expensive to compute or fetch, and distributed caching is ideal for load-balanced or distributed environments where cache sharing is needed.
Explain the intricacies of embedding resources, like images and JavaScript, within an assembly for an ASP.NET application.
Answer
Embedding resources such as images, JavaScript files, or CSS files within an assembly can be useful in ASP.NET applications for various reasons:
- To simplify deployment by packaging all resources within a single assembly, making it easier to distribute or reference as a library.
- To protect resources from unauthorized access or tampering by obfuscating their location and access methods.
- To reuse embedded resources across multiple projects without duplicating files or managing separate resource files.
To embed resources within an assembly for an ASP.NET application, follow these steps:
- Add the resource files (e.g., images, JavaScript, CSS) to your project, usually in a folder named “Resources” or similar.
- Set the “Build Action” property for each resource file to “Embedded Resource”:
Right-click on the resource file -> Properties -> Build Action -> Embedded Resource
- In the
Global.asax
file orStartup.cs
file, register a customHttpHandler
that intercepts requests for embedded resources and serves them from the assembly:
Loading code snippet...
- Implement the custom
HttpHandler
class that extracts the embedded resources from the assembly and serves them in the response:
Loading code snippet...
By following these steps, you can embed resources like images, JavaScript, and CSS within an assembly for an ASP.NET application, simplifying deployment, protecting resources, and enabling resource reuse across multiple projects or libraries.
How do you handle communications between multiple web apps in an ASP.net application, ensuring data consistency and transaction safety?
Answer
Handling communications between multiple web apps in an ASP.NET application, while ensuring data consistency and transaction safety, can be achieved using several approaches, depending on the specific requirements and constraints of the application. Some of the common approaches are:
- Web Services (SOAP or RESTful APIs): Exchange data between web apps using web services, either based on SOAP (e.g., ASMX or WCF) or RESTful APIs (e.g., Web API). Web services provide a standardized and platform-agnostic communication mechanism to exchange data between applications. To ensure transaction safety, the web apps can implement a two-phase commit protocol, coordinating the transactions between them using a transaction coordinator.
- Message Queues: Use message queues (e.g., Azure Service Bus, RabbitMQ, or Apache Kafka) to achieve asynchronous and reliable communication between web apps. Message queues can provide better fault tolerance, load balancing, and scalability compared to direct web service calls. Use message-based patterns like publish-subscribe, competing consumers, or request-reply to ensure data consistency and transaction safety between multiple web apps.
- Distributed caching: Use distributed caching systems (e.g., Redis, Memcached) or data storage (e.g., Azure Cosmos DB, Amazon DynamoDB) to store and share data between multiple web apps. Implement optimistic or pessimistic concurrency control techniques to ensure data consistency when multiple web apps access or modify shared data.
- Distributed transactions: Use distributed transactions management systems (e.g., Microsoft Distributed Transaction Coordinator – MSDTC) to coordinate and manage transactions across multiple web apps. A transaction coordinator can support various transaction models like two-phase commit, three-phase commit, or compensation-based transactions to ensure the consistency and safety of transactions between web apps.
- Data synchronization: If data consistency between multiple web apps can be eventually consistent, implement data synchronization techniques (e.g., replication, change data capture, or event sourcing) to replicate and synchronize data between web apps’ data storage.
Depending on your application’s specific requirements, you can use one or a combination of these approaches to handle communications between multiple web apps in an ASP.NET application, ensuring data consistency and transaction safety.
Our journey through asp net viva questions now guides us through ApiController and ControllerBase in ASP.NET Core. By contrasting these concepts with their counterparts in ASP.NET, we prepare for a deeper dive into the HttpHandlers and HttpModules we touched on earlier in our asp net scenario based interview questions and answers.
Explain the differences between a content management system (CMS) built using ASP.NET and a traditional ASP.NET web application.
Answer
A Content Management System (CMS) is a software application that allows users to create, manage, and modify digital content easily without any technical expertise. A CMS built using ASP.NET and a traditional ASP.NET web application have several key differences:
- Purpose: A traditional ASP.NET web application is a custom-built web application focusing on specific business requirements and user needs. A CMS built using ASP.NET, on the other hand, is a generic, reusable web application that provides content management functionality for various types of websites and applications.
- Features: A CMS built using ASP.NET provides pre-built functionality and features for content management, such as content creation, editing, organization, publishing, workflow management, version control, and access control. A traditional ASP.NET web application typically needs to have these features custom-built, if required.
- Customization: A CMS built using ASP.NET usually provides flexibility and extensibility in terms of adding custom modules, plugins, or themes for specific functionality or appearance. In contrast, a traditional ASP.NET web application requires custom coding and design to implement any additional features or appearance.
- Coding Effort: Traditional ASP.NET web applications require starting from scratch or using templates, whereas a CMS built using ASP.NET already includes a complete codebase, APIs, and UI components. The initial coding effort for a CMS is significantly reduced, allowing developers to focus on customizations or specific business logic.
Some examples of popular ASP.NET-based CMS include Umbraco, Kentico, and Sitefinity.
In summary, a CMS built using ASP.NET is a generic, reusable web application providing pre-built content management functionality and features, whereas a traditional ASP.NET web application is a custom-built application focused on specific business requirements. The choice between the two depends on your project’s needs, available resources, and desired flexibility.
How do you create and consume custom server controls in an ASP.NET Web Forms application?
Answer
Custom server controls, also known as composite controls, are custom ASP.NET Web Forms components that encapsulate complex functionality or combinations of existing controls in a reusable manner. Creating and consuming custom server controls in an ASP.NET Web Forms application usually involves the following steps:
- Create a custom server control: Inherit from the
System.Web.UI.WebControls.WebControl
,System.Web.UI.Control
, or any existing server control class. Override the necessary methods, properties, or events to define the control’s functionality, appearance, and behavior.
Loading code snippet...
- Register the custom server control: Register the custom server control using the
Register
directive on individual Web Form pages that use the control or in theweb.config
file for automatic registration across the entire application.
Loading code snippet...
- Consume the custom server control: Use the custom server control on Web Form pages by including it as an element with the registered tag prefix.
Loading code snippet...
By following these steps, you can create and consume custom server controls in an ASP.NET Web Forms application. Custom server controls allow you to encapsulate complex functionality, reuse code, and simplify the development process for Web Form pages.
What are some common ways to optimize the performance of an ASP.NET application that relies heavily on databases?
Answer
Optimizing the performance of an ASP.NET application that relies heavily on databases can be accomplished using several strategies and techniques:
- Caching: Implement different types of caching mechanisms, such as data caching, output caching, or distributed caching, to store and reuse frequently accessed or computationally expensive data, reducing the need for multiple database calls.
- Connection Pooling: Use connection pooling to manage and reuse database connections, reducing the overhead of creating and closing connections for each database query.
- Lazy Loading: Implement lazy loading to load related data or objects only when they are explicitly accessed, instead of pre-loading all data at once. Use Entity Framework or other ORMs that support lazy loading functionality.
- Pagination: Use pagination to load and display large data sets in smaller chunks, reducing the database load and improving response times.
- Indexing: Create appropriate indexes on database tables to speed up query performance. Analyze query performance and optimize the table schema using indexes according to the application’s data access patterns.
- Query Optimization: Optimize database queries and minimize the number of round trips to the database by using stored procedures, views, or batch operations.
- Eager Loading: Use eager loading when fetching related data to reduce the number of database round trips and minimize the impact of the “N+1” problem. Eager loading can be used with Entity Framework or other ORMs that support the functionality.
- Asynchronous Data Access: Use asynchronous data access techniques like async/await in combination with async-enabled ORMs like Entity Framework to execute database operations without blocking the application, improving responsiveness and throughput.
- Database Sharding and Replication: Distribute database load and improve performance and reliability by implementing database sharding (splitting data across multiple databases) or replication (keeping multiple copies of data synchronized).
These strategies and techniques can be used independently or in combination to optimize the performance of an ASP.NET application that relies heavily on databases.
How can you use attribute routing to create complex URL patterns in an ASP.NET MVC application?
Answer
Attribute routing is a powerful feature in ASP.NET MVC that allows you to define and configure URL patterns for your application directly within controller action methods or controller classes using attributes. You can use attribute routing to create complex URL patterns and improve the maintainability and readability of your routes.
To use attribute routing in an ASP.NET MVC application, follow these steps:
- Enable Attribute Routing: Enable attribute routing during the application startup in
RouteConfig.cs
orStartup.cs
by calling theMapMvcAttributeRoutes
method.
Loading code snippet...
- Define Route Attributes: Define the custom URL patterns for your controller actions or controllers by specifying the
[Route]
attribute.
Loading code snippet...
By using attribute routing, you can create complex URL patterns directly within your controller actions or controller classes, providing better maintainability and readability for your application’s routing configuration.
Describe the use of ApiController and ControllerBase in ASP.NET Core, and how they differ from their counterparts in ASP.NET.
Answer
In ASP.NET Core, ApiController
and ControllerBase
are two base classes that provide common functionalities and conventions for building API controllers and MVC controllers, respectively. They differ from their counterparts in ASP.NET in several ways:
- ApiController: The
ApiController
attribute in ASP.NET Core is an attribute that can be applied to controllers that are meant for building APIs. When a controller is decorated with[ApiController]
, the framework enables various API-specific behaviors and conventions, including automatic model validation, binding source inference, and problem details for error responses. In classic ASP.NET, the equivalent is theApiController
class that you inherit from to create Web API controllers with similar features.
Loading code snippet...
- ControllerBase: The
ControllerBase
class in ASP.NET Core is a base class for both API controllers and MVC controllers that provides common functionalities, such as actions, filters, model binding, validation, and helper methods. In classic ASP.NET MVC, there are two separate classes:ApiController
for Web API controllers andController
for MVC controllers.
Loading code snippet...
In summary, the use of ApiController
and ControllerBase
in ASP.NET Core provides a more unified and streamlined approach for building API controllers and MVC controllers compared to their classic ASP.NET counterparts. With the introduction of the [ApiController]
attribute in ASP.NET Core, you can enable API-specific behaviors and conventions while still inheriting from the ControllerBase
class.
With a firm understanding of the different lifecycle events in an ASP.NET Web Forms application, let’s conclude our asp net questions and answers collection with a detailed examination of Thread Pooling in ASP.NET. This last segment will provide valuable insights into optimizing server performance and tackle the final key points for a successful asp net life cycle interview.
Explain the role of the different lifecycle events in an ASP.NET Web Forms application.
Answer
The lifecycle of an ASP.NET Web Forms application consists of a series of events that occur during the request processing. Understanding these events and their role is crucial to effectively interact with the page and its controls at the right time. The core lifecycle events in an ASP.NET Web Forms page are as follows:
- PreInit: This event is raised before the initialization of the page and its controls. It is useful to set the master page, theme, or other configuration settings dynamically before they are applied to the controls. You can also create controls dynamically during the PreInit event.
- Init: This event occurs after the controls on the page are initialized. At this point, controls and their properties are loaded, but the ViewState is not yet restored. You can perform actions such as setting default control values or registering control-level events in the Init event.
- InitComplete: This event signifies the completion of the initialization phase. During InitComplete, the ViewState for controls has been restored, and any additional initialization tasks can be performed.
- PreLoad: This event occurs just before the page’s Load event. In PreLoad, modifications to controls can be made before loading control state and ViewState.
- Load: The Load event occurs after the control’s state has been restored from ViewState, user input data has been evaluated, and any control-level or user-level event handlers have been executed. You can perform tasks such as data binding, updating user-interface elements based on user input, or validating and processing user input during the Load event.
- Control events: After the Load event, the control-level events are raised (e.g., Button click or DropDownList selected index changed). You can handle these events to perform specific tasks based on user interactions with the controls.
- PreRender: The PreRender event is raised just before the HTML markup for the page and its controls are generated. It’s the last opportunity to make final updates, set control properties, or alter control hierarchy before rendering.
- SaveStateComplete: After the ViewState for controls has been saved, the SaveStateComplete event is raised. At this stage, you can perform tasks that do not affect ViewState, such as logging or tracing.
- Render: During the Render phase, the HTML markup for the page and controls is generated. You can override the Render method to customize the output or interact with the HTML output.
- Unload: The Unload event is the last event in the page lifecycle and signifies the end of the request. This event is useful for cleanup tasks, such as closing resources, disposing of objects, or other housekeeping activities.
Understanding the role of these lifecycle events in an ASP.NET Web Forms application and their execution order is crucial for implementing proper control manipulation, data binding, and user interaction handling.
How can you use the Repository pattern and the Unit of Work pattern to structure data access in an ASP.NET application?
Answer
The Repository pattern and the Unit of Work pattern are design patterns that help structure data access in an ASP.NET application, providing separation of concerns, encapsulation, and maintainability. The patterns can be used together or independently, depending on the application requirements.
- Repository pattern: The Repository pattern is a data persistence abstraction layer that isolates the domain objects and business logic from the data access technology, such as ADO.NET or Entity Framework. It provides a centralized interface for querying, adding, updating, and deleting data, improving code maintainability and reusability.
To implement the Repository pattern, follow these general steps:
- Create interfaces for each repository to define the methods for data access operations (e.g.,
IProductRepository
). - Implement concrete repository classes for each data entity, based on the interfaces (e.g.,
ProductRepository
). - Use dependency injection to inject the concrete repositories into the application’s business logic or domain layer.
- Unit of Work pattern: The Unit of Work pattern is responsible for coordinating and managing transactions and data persistence operations across multiple repositories or data operations. It ensures that all data modifications are either committed or rolled back together, providing a consistent state.
To implement the Unit of Work pattern, follow these general steps:
- Create an
IUnitOfWork
interface to define the methods for committing or rolling back changes. - Create a concrete
UnitOfWork
class that implementsIUnitOfWork
and coordinates the operations of multiple repositories. - Use dependency injection to inject the
UnitOfWork
instance into the application’s business logic or domain layer and manage the transaction scope.
By using the Repository pattern and the Unit of Work pattern together, you can structure data access in an ASP.NET application in a maintainable, organized way, while providing separation of concerns, encapsulation, and transaction management.
Explain Thread Pooling in ASP.NET, and how it can be used to optimize server performance.
Answer
Thread pooling is a mechanism used by .NET and ASP.NET to manage and reuse a collection of threads for executing tasks concurrently. The goal of thread pooling is to reduce the overhead associated with creating and destroying threads, maximize resource utilization, and improve application responsiveness and performance.
In an ASP.NET application, the thread pool is responsible for managing threads used for executing incoming requests. When a request arrives, a thread is taken from the thread pool to process the request. Once the request processing is completed, the thread is returned to the pool, making it available for future requests.
The benefits of using thread pooling in ASP.NET are:
- Resource Optimization: Thread pooling reduces the overhead of creating and destroying threads, which can be computationally expensive. Reusing threads from the pool helps optimize system resources (CPU and memory).
- Scalability: Thread pooling helps improve the scalability of the application by managing the number of concurrent threads, preventing thread exhaustion and ensuring balanced load distribution.
- Responsiveness: Thread pooling can improve application responsiveness by maintaining a set of idle threads that can be quickly assigned to incoming tasks without the delay of thread creation.
- Control and Configuration: The .NET thread pool provides APIs and configuration options to fine-tune the thread pool’s behavior, such as setting the maximum and minimum number of threads, adjusting the optimal idle time, or managing long-running tasks.
To use thread pooling in ASP.NET, you typically rely on the built-in .NET thread pool. You can use the ThreadPool class methods, such as QueueUserWorkItem
or SetMinThreads
, to interact with the thread pool and manage tasks. Additionally, using asynchronous programming techniques like async/await or Task Parallel Library (TPL) in your ASP.NET application can help you take full advantage of the thread pool and improve application performance.
How do you implement data binding in ASP.NET, and what are the key differences between one-way and two-way data binding?
Answer
Data binding in ASP.NET is the process of connecting data sources to user interface elements, enabling automatic synchronization of data between the two. Data binding can be implemented using one-way or two-way data binding techniques:
- One-way data binding: One-way data binding is a process where data from the data source is displayed in the user interface, but any changes made in the UI are not automatically updated back to the data source. One-way data binding is commonly used to display read-only data or when manual intervention is required to update the data source.
To implement one-way data binding in ASP.NET Web Forms, you can use, for example, a GridView control, bind it to a data source (e.g., SqlDataSource
), and display the fetched data in a tabular format:
Loading code snippet...
In ASP.NET MVC and ASP.NET Core, you would typically use Razor syntax to bind data from your model or view model to your views:
Loading code snippet...
- Two-way data binding: Two-way data binding is a process where data from the data source is displayed in the user interface, and any changes made in the UI are automatically updated back to the data source. Two-way data binding enables a more dynamic and interactive user experience, as the data source is automatically updated based on user input.
To implement two-way data binding in ASP.NET Web Forms, you can use data-binding expressions, such as <%# ... %>
, inside your controls:
Loading code snippet...
ASP.NET MVC and ASP.NET Core do not provide built-in two-way data binding like Web Forms. However, you can achieve a similar effect by using model binding and form POST actions to update the data source based on user input:
Loading code snippet...
In summary, data binding in ASP.NET can be implemented using one-way or two-way data binding techniques, with the key difference being how data is synchronized between the user interface and the data source. One-way data binding only updates the UI based on the data source, while two-way data binding automatically updates the data source based on user input.
What is the role of the IHttpContextAccessor interface in an ASP.NET Core application, and how does it differ from the HttpContext class in earlier versions of ASP.NET?
Answer
The IHttpContextAccessor
interface in ASP.NET Core is used to obtain the current HttpContext
instance from anywhere in your application, outside of controller actions or middleware. The IHttpContextAccessor
role is essential for scenarios where the HttpContext
is not automatically available, such as background tasks or services.
In earlier versions of ASP.NET, such as Web Forms or MVC, the HttpContext
class provided a global Current
property that allowed you to access the current context instance from any part of the application, directly using HttpContext.Current
.
The main differences between the IHttpContextAccessor
in ASP.NET Core and the HttpContext
class in earlier versions of ASP.NET are:
- Dependency Injection: In ASP.NET Core, the
IHttpContextAccessor
is an interface that needs to be registered in the dependency injection (DI) container during the application startup, which allows you to inject it wherever needed:
Loading code snippet...
In earlier versions of ASP.NET, the HttpContext
class was a concrete class with a static Current
property, and there was no need for dependency injection.
- Testability: Using the
IHttpContextAccessor
interface in ASP.NET Core makes it easier to test your application’s components, as you can mock or substitute theIHttpContextAccessor
andHttpContext
instances during testing, whereas using the staticHttpContext.Current
in earlier versions of ASP.NET made testing more challenging. - Lifetime management: In ASP.NET Core, the
IHttpContextAccessor
helps manage the lifetime of theHttpContext
instance according to the request scope, ensuring proper disposal and reducing memory leaks risks. In earlier versions of ASP.NET, theHttpContext.Current
property was managed globally, which could lead to potential issues with thread safety or memory leaks.
In summary, the IHttpContextAccessor
in ASP.NET Core is an interface to access the current HttpContext
instance in a more flexible, testable, and safe manner than the static HttpContext.Current
property in earlier versions of ASP.NET. The use of dependency injection and improved lifetime management make IHttpContextAccessor
a preferable choice for accessing the HttpContext
in modern ASP.NET Core applications.
We hope you found this comprehensive article helpful as you prepare for your asp net interview. These carefully selected asp net interview questions and answers have been designed to cover a wide range of topics and cater to different experience levels.
By understanding and mastering these asp net questions and answers, you will undoubtedly enhance your expertise and confidence to excel in your interview as an ASP.NET developer. Keep in mind that practical knowledge is equally essential, so don’t forget to practice and apply what you’ve learned to real-world scenarios.
Best of luck on your journey to success in the world of ASP.NET development!