‘Using’ Keyword in C#: Full Guide
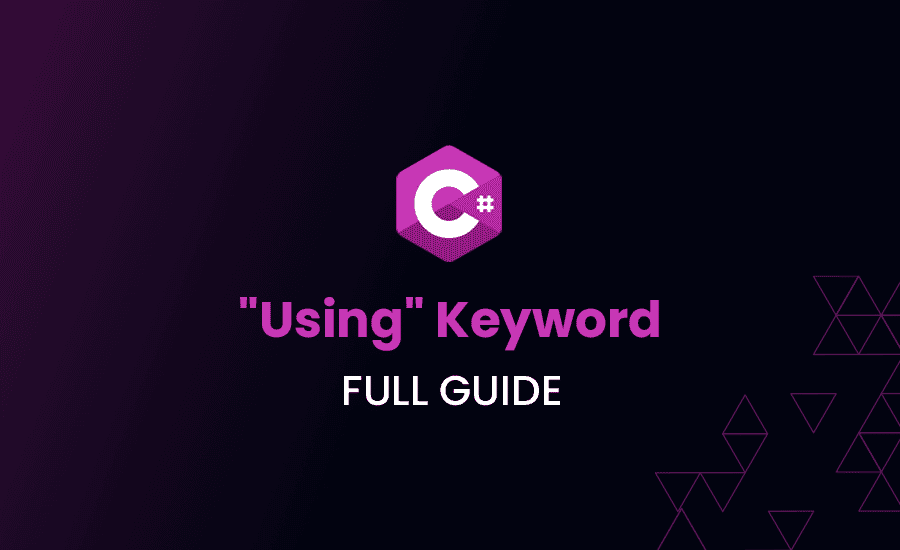
Discover the power of using
keyword in C# and start writing cleaner, more efficient code today!
Introduction to Using Keyword in C#
Hey there! So you’ve started your journey with C#. That’s fantastic, and I bet you’re jazzed to dig into its every nook and cranny. Well, guess what? Today we’re venturing into the realm of using
keyword—a small yet powerful word that can transform your coding game!
Understanding the C# Language
First off, let’s dip our toes into the C# waters. As you know, C# is a versatile, type-safe, and object-oriented language—quite the triple threat, right? It’s got bells, whistles and everything in between.
Key Features of C#
C# is teeming with handy features that can simplify complex tasks, like in-built garbage collection, exception handling, and more. But one feature that’s often misunderstood or overlooked is the using
keyword. Sounds intriguing, huh?
Importance of C# Using Keyword
“If using
is so important, why don’t I know much about it?”, you might ask. Well, it’s high time we pulled back the curtain on this C# linchpin.
The Role of the ‘Using’ Keyword in C#
The using
keyword does have a bit of a superhero complex—it frees up resources after operation completion, takes care of exceptions, and makes code more readable. It’s essentially the Ironman in the Avenger’s world of programming.
Loading code snippet...
This snippet reads a file by utilizing the StreamReader
object inside the using
statement. Right after you’re done with reading the file, the StreamReader
gets disposed of, saving you memory leaks. Handy, eh?
Why Use the ‘Using’ Keyword in C#
So, why bother with using
? Because it’s all about making your life easier!
But don’t just take my word for it, here’s the evidence:
- Automatic Resource Management:
using
is your very own clean-up crew. It makes sure to dispose of non-managed resources. No need to bother withDispose()
orClose()
,using
does the heavy lifting! - Improved Code Readability:
using
keeps your code compact and easy on the eyes. Nobody enjoys reading convoluted and stuffy code, after all!
Deep Dive into C# Using Keyword
Alright, fellow explorer, let’s dig deeper into the obscure depths of the using
keyword.
Syntax of the ‘Using’ Keyword in C#
The syntax for using
is quite intuitive. You start your line with using
, followed by brackets, with the object which needs disposing of. Following that, well, it’s your world! Write lines of code, make that dot-matrix printer design you always wanted!
Loading code snippet...
This code runs Action
using the DisposableResource
, a hypothetical resource. Once done, DisposableResource()
is disposed of automatically.
Demonstrating the ‘Using’ Keyword in Practice
Enough chit chat, let’s roll up our sleeves and jump into some serious using
action!
Loading code snippet...
In this little number, we’re using using
to work with a FileStream
. using
ensures that after we’re done with the FileStream
, it gets closed, no loose threads!
Common Mistakes when Using ‘Using’ in C#
Even seasoned C# professionals make mistakes from time to time. Here’s how to avoid those infamous using
slip-ups.
Mistake and How to Avoid It
One common pitfall happens when we try to access a disposed object. using
disposes of your object after usage. So, trying to access it is like trying to squeeze juice from a squeezed lemon—inconvenient and messy!
Here’s what not to do:
Loading code snippet...
To dodge this mistake, always access your objects within the using
scope.
Best Practices for Using ‘Using’
Ready for some extra tips to make your life easier? Here they are:
- Always use
using
when working with IDisposable objects. - Nest multiple
using
blocks when dealing with several disposable resources.
Advanced Concepts Related to C# Using Keyword
If you thought using
was all about disposing of resources, think again!
‘Using’ in Complex Scenarios
using
can shine brightly even in complex scenarios. Remember, consistent housekeeping is key in multi-threading. So, why not leverage using
to clean up after each thread execution?
Loading code snippet...
This snippet showcases how using
can dispose of a ManualResetEvent
in a threading scenario.
Alternatives to ‘Using’ in C#
Though using
is fantastic in many scenarios, there are alternatives that are worth a glance. One such alternative is the finally
block—but that’s another story for another time.
Conclusion: Optimizing Your Coding With C# Using Keyword
So, there you have it! You’ve just embarked on a journey exploring the using
keyword. It isn’t a journey everyone takes— but hey, that’s why they need a daring adventurer like you!
Happy exploring!