Continue in C#: Tutorial + Examples
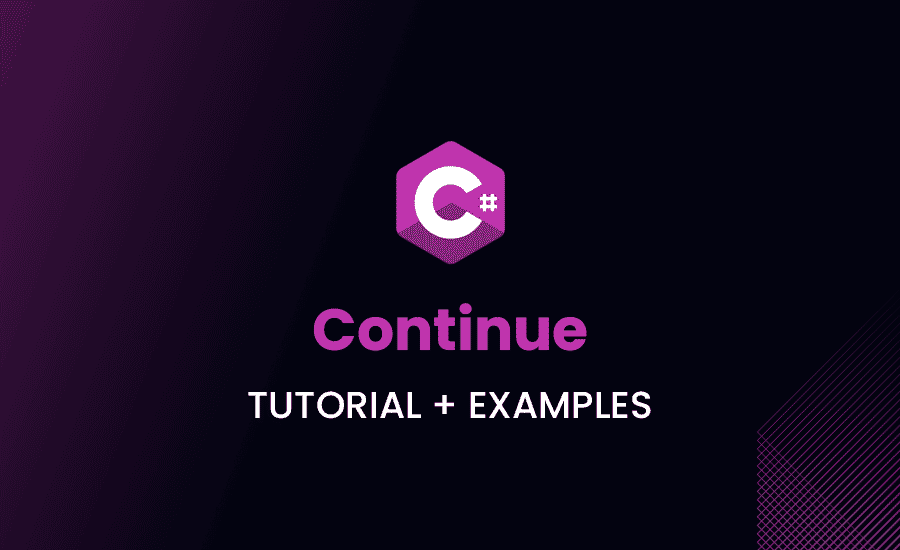
Congratulations on stepping into the captivating world of C# coding! Have you ever thought about how to make your loop iterations more efficient? Or have you wondered how you could skip certain logic within loop constructs without totally breaking out of the loop? If so, you’re in the right place! Let’s begin our journey with ‘Continue’ in C#.
Continue in C#
Now that we’ve covered the basics, it’s time to delve into ‘Continue’ in C#. Are you ready to add another powerful tool to your C# toolbox?
Understanding the ‘Continue’ Statement in C#
The ‘Continue’ keyword is used within a loop structure in C# to pass control to the next iteration of the loop, bypassing any code that follows it inside the loop body. Interesting, right?
Loading code snippet...
In this example, when the value of i
reaches 5, the loop skips the Console.WriteLine(i)
statement and jumps to the next iteration. ‘Continue’ literally says “skip the rest of this loop and get me to the next one, pronto!”
Syntax and Usage of ‘Continue’ in C#
Using ‘Continue’ is as easy as pie! In a nutshell, whenever your program encounters ‘Continue’, it hops back to the start of the loop for the next iteration.
Loading code snippet...
Just like in the previous example, when i
equals 5, the program skips the Console.WriteLine(i)
statement and ushers in the next iteration. Notably, remember to increase the counter after ‘Continue’ to avoid infinite loops.
Practical Examples of C# Continue
Let’s see some practical examples of ‘Continue’ in action. Hands-on coding is often the best teacher, so I’d encourage you to give these examples a try on your own.
Using ‘Continue’ In a For Loop
We can use ‘Continue’ in our loop constructs to skip specific iterations. Let’s give ‘Continue’ a whirl in a C# ‘for’ loop.
Loading code snippet...
This example only prints odd numbers between 1 and 10. Neat, huh?
C# Foreach Continue: Syntax and Examples
‘Continue’ plays nicely not only with ‘for’ and ‘while’ loops but also with ‘foreach’. Let’s give that a go.
Loading code snippet...
In this example, the program prints all the names that do not start with “Jo”. “John” and “Joe” are skipped by ‘Continue’. Handy, don’t you think?
Contrast between Continue and Break in C#
It’s also essential to differentiate ‘Continue’ from another loop control keyword: ‘Break’. While they may seem similar, they behave quite differently.
C# Continue Vs Break: Their Differences Explored
‘Continue’ and ‘Break’ command different actions inside a loop. While ‘Continue’ skips to the next iterations, ‘Break’ exits the loop altogether.
Loading code snippet...
In this example, when i
is equal to 5, the ‘Break’ statement terminates the loop entirely.
Practical Situations in using Continue over Break
There can be scenarios where you need to skip specific iterations but not exit the loop. That’s where ‘Continue’ is your hero, and ‘Break’ might be a little too drastic.
For example, if you’re processing a list of transactions, and you want to skip any transactions above 1000 dollars but continue processing others, ‘Continue’ would be the perfect choice.
The Application of Continue in C#
Let’s now explore some more practical applications for the ‘Continue’ statement in C#. From controlling loop iterations to handling errors, ‘Continue’ has got you covered.
Continue For C#: How to Efficiently Control Loop Iterations
‘Continue’ is a powerful tool for controlling loop iterations. It commands the loop to progress to the next iteration, bypassing the rest of the code within the loop for the current iteration.
Loading code snippet...
In this example, we only process transactions with even numbers and skip the odd ones, making our loop iteration more efficient.
The Role of ‘Continue’ in Error Handling
In C#, ‘Continue’ is instrumental in handling exceptions or errors encountered during loop iterations. Instead of terminating the program, it skips the problematic iteration and moves to the next one.
Loading code snippet...
In this example, instead of crashing when it encounters a division by zero, the program skips the problematic division and continues with the next number. Robust, isn’t it?
Advanced Usage of C# Continue
You’re doing great so far! Let’s venture a bit further and look at the role of ‘Continue’ in more advanced C# scenarios.
Leveraging ‘Continue’ in Complex C# Programs
Leveraging ‘Continue’ becomes even more crucial in complex programs. A well-timed ‘Continue’ statement can be a life-saver when you have nested loops or extensive logic in a loop. It can help to skip unnecessary computations and optimize your program’s performance.
Loading code snippet...
In this nested loop example, we only process records with both i
and j
as even numbers, skipping all other iterations. Whoa, that’s some serious control over our loops!
Common Pitfalls When Using ‘Continue’ in C#
Remember the wise old saying, “With great power, comes great responsibility”? The same applies to the ‘Continue’ statement. While it’s a potent tool, it’s essential to use it wisely.
Ensure that you avoid an infinite loop by updating counters or conditions before ‘Continue’, so the loop does not run endlessly. This can especially happen in ‘while’ and ‘do-while’ loops. Confusion between ‘Continue’ and ‘Break’ is another common pitfall.
Conclusion
Through this tutorial, we’ve discovered the magic of the ‘Continue’ statement: the trooper that helps skip unwanted code in loop iterations and soldier on with our computational battles. From basic usage in ‘for’, ‘while’, and ‘foreach’ loops to handling complex scenarios and error exceptions, ‘Continue’ marches on bravely, never saying die (or “break” for that matter).