Comparing Strings in C#: What’s the Best Way?
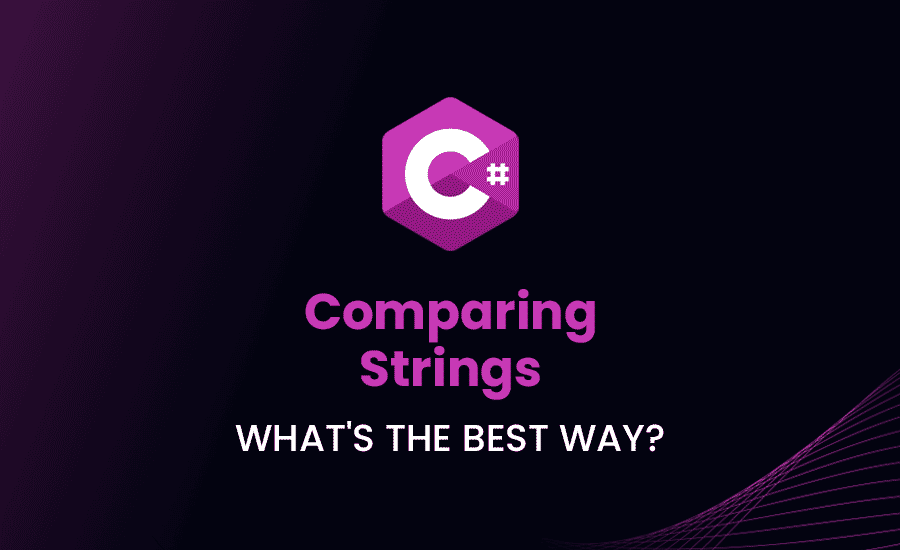
How often do you compare strings in your C# programming? Isn’t it a lot? Today, we are going to journey through the world of comparing strings in C#, breaking it all down to the tiniest details! Hang onto your hats, because we’re about to dive deep!
Comparing Strings in C#: The What, Why and How
Do you often find yourself scratching your head when it comes to comparing strings in C#? Do not worry, we got the antidote to your ailment! Wrap yourself up for a ride down the Concept Avenue, where we discuss the basics, and the ‘why’ we even need to compare strings in C#!
The Basics of Comparing Strings in C#
In C#, we usually compare strings using methods like String.Compare
, String.Equals
, or simple equality operators like ==
and !=
. If these methods are news to you, then let us initiate you to this fascinating universe.
You see, in the world of C#, String.Compare
is akin to our trusty handy-dandy Swiss army knife – handy and undeniably utilitarian. The String.Compare(string strA, string strB)
method is one of these tools.
Loading code snippet...
In this code, String.Compare
performs a case-sensitive comparison. Wicked, right? I know you’re thrilled to keep going, so buckle up!
Why We Need to Compare Strings in C#
So why are we here, discussing string comparisons? There’s a myriad of reasons. String comparison is often a quintessential part of many common tasks in C#, such as checking user credentials, sorting lists, or even responding to commands in a chatbot. But we’re just scratching the surface here, there’s more coming!
Different Methods of Comparing Strings in C#
Now that we’ve glimpsed the concept, let’s dig deeper into the methods of string comparison. We’re about to traverse the landscape of String.Compare
, equality operators and the art of comparing two strings in C#. Hop on!
Using String.Compare Method
The String.Compare
method is our trusty sidekick that rises to the occasion every time we face pairs of strings to compare. It provides an numerical output, making the method not just flexible, but also insightful of the comparison result. Let’s look at it in action.
Loading code snippet...
Isn’t it simple and efficient? Stay with me, because we are about to kick things up a notch.
Using Equality Operators
Do you remember the good old ==
and !=
operators? Yes, they pop up here as well. These are perfect for when you just need to check if two strings are equal or not. Let’s bring out an example to illustrate.
Loading code snippet...
This will print “The strings are not equal” since the ==
operator is case-sensitive. Likewise, !=
operator checks if the strings are different. Isn’t it amazing how versatile these operators are?
How to Compare Two Strings in C#
You’ll likely compare two strings more often than you’d care to admit. If you fancy some options, C# gracefully provides. Alongside the String.Compare
method and the ==
, !=
operators, there’s also String.Equals
. Let’s see it working its magic.
Loading code snippet...
Notice how String.Equals
can also handle case-insensitive comparisons? C# indeed never ceases to surprise!
Ignoring Case in String Comparisons
Have you faced a situation where Apple
is not the same as apple
? Does it make you wish you could tell C# to chill a bit on the case-sensitivity? Well, guess what, you can! Let’s delve into what C# offers to help us master the art of case-insensitive comparisons.
Understanding C# String Compare Ignore Case
A case-insensitive comparison can save the day when Apple, apple, aPpLe
are just apples. To do so, C# provides an overload of the String.Compare
method where you input a boolean to tell C# to ignore the case.
Loading code snippet...
When ignoreCase is set to true, String.Compare
ignores the case while comparing strA and strB. There’s your magic spell to control the case sensitivity!
Implementing Case Insensitive Comparisons in C#
How about we bring the String.Compare(strA, strB, ignoreCase)
spell to life? Hang tight, as we are about to turn the peas into an exquisite peas porridge.
Loading code snippet...
This little snippet shows how String.Compare
can handle case-insensitive comparisons. Thrilling, isn’t it?
C# String Compare Case Insensitive: Practical Examples
So how can this help you? Imagine a scenario where the case doesn’t matter. Maybe you are comparing usernames, passwords, email addresses, or any strings that should be considered equal despite case differences. That’s where String.Compare(strA, strB, ignoreCase)
can come to the rescue.
Alphabetical Comparisons of Strings in C#
Ever wondered if Banana
comes before Apple
in C#? Knowing how to compare strings alphabetically can help answer such questions. Brace yourself, as we dive into the alphabet of C# strings.
The Basics of Comparing Strings Alphabetically in C#
Remember the String.Compare
method? This neat little method does more than just compare two strings; it can compare them alphabetically! If the result of String.Compare
is less than zero, the first string is less than the second (i.e., it comes before in the alphabet). There’s more to String.Compare
than meets the eye!
Let’s look at some code to illustrate this.
Loading code snippet...
The output confirms that ‘Apple’ (str2) comes before ‘Banana’ (str1) in alphabetical sorting. Fairly intuitive, isn’t it?
Practical Use Cases for Alphabetical Comparisons
Where might you ask would you need to compare strings alphabetically? Think about sorting a list of names, organizing files by their names or even sorting a list of products. The applications are as vast as the universe itself!
Comparing Strings to Enumerations in C#
While strings are great, C# introduces us to enums, or enumerations, to group named constants better. Now the real question is, can you compare a string to an enum? The answer, my friend, is a resounding yes! Let’s explore how!
Fundamentals of String-to-Enum Comparisons in C#
To compare a string to an enum, C# gives us Enum.TryParse
, Enum.Parse
, and Enum.IsDefined
methods. Of these, Enum.TryParse
and Enum.Parse
can convert a string into its equivalent enumeration constant. In contrast, Enum.IsDefined
determines whether a particular string or integer value exists in the enumeration.
Check out this snippet:
Loading code snippet...
Enum.IsDefined
checks if ‘Banana’ is a member of the Fruit enumeration. Pretty neat, isn’t it?
C# Compare String to Enum: How-To Guide
What if you want to convert the string to the equivalent enum member? No worries! C# has you covered with methods like Enum.TryParse
and Enum.Parse
.Here, we will use Enum.TryParse
.
Loading code snippet...
Enum.TryParse
tries to convert ‘Fri’ into its equivalent DayOfWeek enum member. See how versatile C# is?
Comparing a C# Array of Strings
We’ve been doing a lot of one-on-one comparisons so far. But what if the situation demands an array of strings? That’s right; C# has that covered too. Let’s put those arrays under the lens and see how C# treats them.
Understanding an Array of Strings in C#
Remember how C# stoically carries us beyond simple string comparisons? That frontier includes arrays, or lists of strings. Comparing arrays in C# is a bit more complex but fret not: whether you want to compare the full arrays or just some elements, C# has tools for the job.
Consider this snippet:
Loading code snippet...
This uses Enumerable.SequenceEqual
to compare two string arrays element by element. Ingenious, right?
C# Compare Array of Strings: Techniques and Implications
C# string array comparisons go beyond checking if two arrays are the same. Think of tasks like finding common elements in multiple arrays or checking if an array contains a particular string. These are not just coding exercises; real-world applications are rife with such requirements.
Real-World Situations of Strings Comparisons in C#
Enough of theory, let’s stroll through some actual applications. Real-world scenarios will help you grasp the power of string comparisons. From checking user credentials to sorting a list of words, let’s explore how these methods are used in practical situations!
How Companies are Leveraging String Comparisons in C#
Companies often need to compare strings. Think about a company with a command-line tool that responds to commands entered as strings. Such a tool would need to compare the input string to predefined commands to decide what action to take.
Or, consider a social media platform. When checking usernames or emails during registration, string comparisons become crucial. Recognizing the importance of string comparison methods in C# and learning how to use them is an invaluable skill that cannot be underestimated.
Exploring Error Prevention Using String Comparisons
String comparisons can also be a guarding angel to avoid errors. For instance, when processing user input, comparing input strings against valid options can help prevent invalid operations. It’s like having a friendly watchdog guarding your code.
Conclusion Of Comparing Strings C#
If comparing strings in C# were an iceberg, we’ve now explored it from summit to base. From the basics to various methods, and from case-insensitivity to string arrays – we’ve journeyed far into the realm of C# string comparisons. What’s more, we’ve connected them with real-world applications.
Tips and Tricks to Optimize Your String Comparisons
But the tour wouldn’t be complete without some parting tips:
- Remember,
String.Compare
and==, !=
are case-sensitive by default. Use the relevant overloads for case-insensitive comparisons. String.Compare
can be handy for sorting strings alphabetically.- Enum.IsDefined, Enum.TryParse, and Enum.Parse are excellent for comparison between strings and enums.
- When comparing arrays of strings,
Enumerable.SequenceEqual()
can save the day.