How To Use Enum in C# with Examples
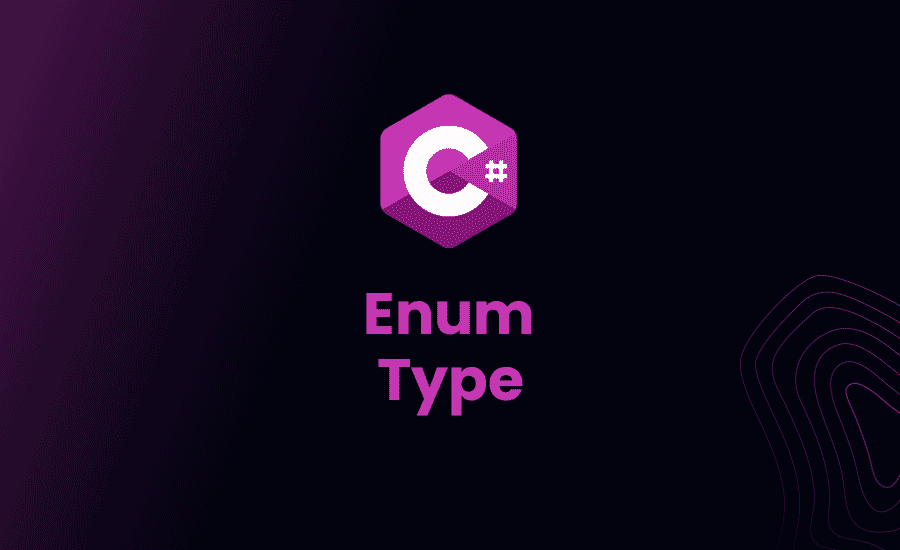
Dear coding enthusiasts, let’s delve into a topic that is crucial but often overlooked by many beginners – enums in C#. Enums, or enumerations, are a special kind of value type in C# that let you specify a group of named constants, making your code more readable and less error-prone. Sounds interesting, right?
What is C# enum?
So, what is this c# enum we speak of?
Definition of C# enum
An enum
is a value type, defined by keyword enum
, that specifies a group of named constants. This makes your code more readable and maintainable. Let’s look at the basic syntax of an enum:
Loading code snippet...
The above code represents a simple definition of an enum, which, by default, has an underlying type of int
and starts with the value 0.
The Need for C# enum in Coding
Now, you might ask, why do we need enums? Well, they come to the rescue when you have a number of values that are related and known at compile-time. By using enums, you can make your code easier to read and maintain, less prone to errors and more structured. It’s like having a box to keep all your related tools together!
C# enum declaration: The Syntax
Curious about how to declare an enum in C#?
Loading code snippet...
In here, WeekDays
is known as an enumeration (or c# enum), containing a list of constants Monday
, Tuesday
, etc. Now, these aren’t just numbers, but days that can be used more meaningfully in programs.
Implementing c# enum with Examples
Do you know the best way to understand the core concepts of programming? By implementing them, of course!
Simple C# enum example with Code Snippet
Here’s a simple yet detailed c# enum example for you:
Loading code snippet...
In this case, we have an enum TrafficLight
with values Red
, Yellow
and Green
. The variable light
is assigned the value TrafficLight.Red
.
Create enum class C#: A Step by Step Approach
Enums in C# are not classes. However, we can use classes to wrap around enums. Why do that, you ask? Well, this provides a way to extend enums with utility methods. Intrigued? Let’s dive deeper.
Advantages of enum class C# over Traditional Enum
Think of an enum class
as a toddler learning to walk. The traditional enum (without class) is like crawling– it gets the job done, but isn’t efficient. An enum class
, on the other hand, empowers you with all the power of OOP – encapsulation, inheritance and more. With greater superpowers comes greater code readability and maintainability.
C# enum class example: Real World Analogy
Here is how you can define an enum inside a class, a.k.a ‘c# class enum’.
Loading code snippet...
In the code above, we have a class Season
encapsulating the enum Value
. This enum provides specific season values that we can use.
Public Enum and Enum in Class in C#
Let’s turn the spotlight to some specific concepts in C# – Public Enum
and Enum in Class
. Both concepts are like different flavors of your favorite ice cream, each has its own unique taste but essentially, they serve the same purpose – making your coding journey smoother!
Public Enum C#
In the fascinating world of C#, public enum
plays the role of a show stopper. It’s the star which shines bright, ensuring that everyone has its visibility. In technical terms, a public enum
in C# means that it’s accessible by any class in any assembly in your program. Remarkable, right?
Behold this piece of code which gives a simple yet meaningful demonstration on public enum
:
Loading code snippet...
In this case, Animal
is a public enum
that we’ve declared in our code. By doing so, we’ve made sure that the Animal
enum, containing the constants Dog
, Cat
, and Elephant
can be accessed from anywhere in the program. So, wherever we need to represent an animal in our code, we can readily use this public enum
. Isn’t it helpful to have public enums by your side?
Where to use Public enum C# and its Benefits
Now, understanding public enum
is good, but knowing where to use it makes all the difference. Imagine you’re working on a project where multiple classes or assemblies need to access a common set of constants. Won’t it be easier to have a public enum
which can be accessed anywhere in the program? Exactly!
For instance, let’s say you’re developing a card game. In a card game, you have four suits viz, Hearts
, Diamonds
, Clubs
, and Spades
. These suits are integral to card games, and multiple classes in your game project would need to use this information.
Loading code snippet...
In the above code, CardSuit
is a public enum
. This enum, being public, is like an open book readily available for any class in your program that needs to use this information.
So, like an octopus spreading its tentacles, public enums
in C# can reach out to any class, any assembly. Trust me, public enums in C# can be a lifesaver in such scenarios!
It’s almost like playing a game of chess. Just like having your queen in the right place can get you one step closer to checkmate, using public enums at the right places in your code can make your programming journey seamlessly convenient.
Enum in Class C#: Its Need and Implementation
Wait, what? We can have enums inside a class? Yes, it’s possible! Sometimes, you’d want to keep your enums tucked away safely in a specific class. This is where enums encapsulated in a class come into play. This concept is like hiding your secret diary inside a bookshelf where it remains close at hand yet hidden perfectly!
C# enum in class: A Practical Implementation
Let’s have a look at this code example that shows how we can define an enum inside a class:
Loading code snippet...
What we’ve done here is snugly placed PlanetType
enum inside the Planet
class. This enum now behaves like a subcategory of Planet
. So, you can easily figure out the type of any Planet
object by using this enum. Placing enums securely inside classes brings a strong sense of association and hierarchy in your code, making it more comprehendible.
Stay with me while I draw a simple analogy to help you connect the dots. Imagine a cupcake stand at a children’s party. The stand (our class Planet
) has two distinct tiers (our enum PlanetType
). Now the top tier contains chocolate cupcakes (Terrestrial
planets) and the bottom tier has vanilla cupcakes (GasGiant
). So when a kid says he wants a chocolate cupcake, you simply take one from the top tier. Notice how the structure of the cupcake stand helps us quickly and easily distinguish between the cupcakes, just like our enum in class.
C# Enumeration Class and C# Class Enum
Let’s step it up a notch and dive deeper into some advanced topics in C#. Welcome to the land of Enumeration Class and Class Enum in C#.
C# Enumeration Class: Concept and Syntactical Use
The term ‘Enumeration Class’ in C# might seem like a new concept to you. All enumeration types implicitly inherit from System.Enum
which provides useful methods to work with enums.
Loading code snippet...
In the above code, converting an enum member to an integer returns its index value.
Enumeration Class C# Usage: Real Life Scenario Example
Ever wanted to get a string representation of an enum member? You guessed it, System.Enum
makes this a breeze
Loading code snippet...
In the example above, the ToString()
method of System.Enum
is used to obtain a string representation for the enum member Winter
.
Working with Class Enum C#: A Detailed Overview
Class Enums put the power of classes and enums together, letting you use the advantages of both. In a class enum, the enum is defined inside a class, providing a namespace for the enum. This keeps your code clean and lets you use the same enum names in different classes without any conflicts.
Imagine it like a cookie jar – it keeps your cookies (or in this case, enums) organized and prevents others (other classes or methods) from magically finding cookies they weren’t supposed to.
Unity Enum in C#
Coding in Unity with C# is like making a delicious sandwich. Each component plays a specific role in making the sandwich (the game) tasty and appealing. Enums in this sandwich are like that secret sauce! They bring everything together and step up the flavor. Intriguing, isn’t it?
Let’s delve into how enums are used in Unity with C#, thereby unearthing a special variant of enums, the ‘Unity enums’.
Understanding Unity enum: Its Role and Place in Unity C#
Unity engine is quite friendly with C#. It uses C# as its primary scripting language. Now, when you’re developing a game in Unity, such as an epic space battle or an intricate puzzle, you’ll often find yourself dealing with a set of predefined, related values, like game states, character types, weapon modes, etc.
This is where enums lend a helping hand. Enums are splendid at managing such sets and are an integral part of Unity’s API. You may not see them upfront but they’re there, working their magic behind the scenes, akin to the hidden stagehands in a theatre play making sure everything runs smoothly.
Picture the Unity API as an enormous puzzle, with each piece being a different feature, object, property, or method that you can use while building your game. Enums are like those corner pieces which help define the boundaries of your puzzle and give you a solid foundation to work from.
C# Enum Unity: An Example-Based Walkthrough
Now, let’s dive into an example where we use enums in Unity to manage game states. In most games, shared experiences, like controlling character actions based on game states, are managed via enums. States such as playing, game over or loading are common to most games, hence making an enum is an ideal way to manage them.
Loading code snippet...
In the above code, we’ve created the GameState
enum that contains three possible states of our game. These states are Playing
, GameOver
, and Loading
. Once defined, we can use these states throughout our game script. As demonstrated, we initialize our currentGameState
variable to GameState.Playing
meaning the game is currently in an active state.
This concept is like stepping onto an escalator. The Playing
state is when you are moving upward and exploring new floors (game levels). The Loading
state is that brief pause you experience when you step onto the escalator (the transition between game levels). Finally, GameOver
is when you reach the top (end of the game).
How to Use Unity Enum Effectively in C# Projects
Unity enum, the hidden gem of the Unity C# world! It’s a powerful tool to have in your game development toolkit. Why? Because it not only enhances the readability and maintainability of your game code but also reduces the possibility of errors.
Thus, it’s crucial to master the application of Unity enums. Always remember, the pathway to becoming a skilled game developer involves efficient utilization of Unity enums.
Consider it like being a chef at a bustling cafe – you need to manage numerous orders (states) effectively. Similarly, in a game script, you need to manage various states the game can be in. Unity enums help you do that!
Imagine having to reiterate the states every time you needed them. A daunting task indeed! But with the efficiency of Unity Enum, this task becomes effortless.
Also, take advantage of Unity’s built-in enums. Unity API has several built-in enums, like KeyCode
for key inputs, ForceMode
for different ways to apply force to objects, and many more. These are at your disposal, ready to spike up your game script like a sprinkle of hot sauce.
So, treat Unity enums as your faithful sidekick, ready to be at your service at any stage of your game script. Trust me when I say this; you’ll appreciate having them around.
Decoding C# Enum Usage: Dos and Don’ts
Alright champs, it’s time to unleash some pro tips! Understanding and implementing enums
is just one side of the story. Knowing the best practices to follow and common mistakes to avoid while using enums is like your lightsaber in the programming universe. It’s what separates the Padawans from the Jedis!
C# How To Use Enum: Best Practices
Believe me when I tell you this – having best practices up your sleeve can take your coding to the next level.
Use meaningful names for your enums and enum members to increase readability
In the arena of programming, the name is the game! Ensuring descriptive and meaningful names for your enums and its members can revolutionize the readability of your code. Yes, it turns your code from a cryptic mystery into a comprehensible story!
Bad Code:
Loading code snippet...
In this bad code example, we have an enum X
with members V1
, V2
, and V3
. But what do these denote? A reader would be lost trying to understand their purpose.
Good Code:
Loading code snippet...
In this example where good practice is followed, we declare an enum TrafficSignal
with members Red
, Yellow
, and Green
. Instantly, you know these represent the colors of a traffic signal. Easy, right?
Do not use an enum when the range of possible values for a variable is not known at compile time.
Just like you can’t fit a square peg in a round hole, enums aren’t the go-to option when your variable values are not known at compile time. Enums are bastions of constants, and trying to fit variables would be like trying to make a turtle fly!
Bad Code:
Loading code snippet...
In the above example, Temperature
enum is trying to represent freezing point and boiling point, but temperature is variable and can have a range of values.
Good Code:
Here, using a constant or variable would be more applicable.
Loading code snippet...
In this example, we correctly use constants instead of an enum to represent the freezing and boiling points of water.
Declare enum type with an explicit underlying type that is smaller than the default
This is like picking the right vehicle for your journey. For shorter or more memory-efficient code paths, you might prefer smaller underlying types for your enums, instead of the default int
.
Bad Code:
Loading code snippet...
In the above code, Direction
enum has the default underlying type int
which uses 4 bytes of memory.
Good Code:
Loading code snippet...
In this case, the Direction
enum is explicitly defined with byte
as the underlying type, thus using up less memory.
Common Mistakes to Avoid While Using C# enum
Stepping into the programmer’s shoes also means awareness of the common pitfalls. It’s these roadblocks that can transform a promising piece of code into a churning cauldron of errors!
Avoid assigning the same value to different enum members
This can lead to confusion and misleading code which is always a bad idea. Imagine you’ve two keys for the same lock, it can lead to mix-ups, right?
Bad Code:
Loading code snippet...
In this bad code example, both Key1
and Key2
have the same assigned value which can lead to confusion and errors in code
Good Code:
Loading code snippet...
In the good code example, each UniqueKey
enum member has a unique value. This helps avoid mix-ups.
Be wary of the default underlying type and zero-based approach of enums, especially if you need to start your values from a different number
Always remember – the enum’s underlying type is int
and its values start from 0 by default. Overlooking this might be like setting a trap for your own self!
Bad Code:
Loading code snippet...
In the above code, Monday
is initialized as 0, which might not be expected if we want our week to start from 1.
Good Code:
Loading code snippet...
The above Days
enum has an extra NullDay
at 0 and the rest of the days starting from 1. This respects the zero-based nature of enums and fits our requirement of Monday being 1.
Brushing Up The Concepts: An In-Depth C# Enum Tutorial
As we wrap up this extensive guide, it’s time to gather all your learning and brush up the concepts with an in-depth C# Enum Tutorial. Let’s tune up C Sharp Enums!
Guide to C Sharp Enums for Beginners
- Enum is a value type with a set of related named constants.
- By default, the underlying type of enum elements is
int
. - Enums make your code more maintainable and readable by using meaningful names instead of integral constants.
- Declaration Syntax:
enum <enum_name>{ enumeration list };
- Example:
Loading code snippet...
Intermediate to Advance Concepts in C# Enum Usage
- Enums are strongly typed constants. Hence, an explicit cast is needed to convert from enum type to an integral type and vice versa.
- Enum members are initialized to zero-based indices by default unless values are explicitly provided for them.
- Enums can have methods, but cannot inherit from another class or struct.
- While enums are not classes, they do implicitly inherit from
System.Enum
. - System.Enum provides several useful methods and properties to work with enums.
- Example:
Loading code snippet...
Tips to Master C# Enum Usage
- Practice, practice, practice! The more you work with enums, the better you’ll understand when and where to use them. Try incorporating enums in your existing projects. Look for areas in your code where enums can replace groups of named constants.
- When working with enums, remember that they start at 0 and increment thereafter. This can lead to potential errors if not accounted for.
- Experiment with different underlying types for your enums depending on what suits your needs.
- Leverage the methods and capabilities provided by
System.Enum
to manipulate enums and enhance your programming efficiency.
So there you have it, folks! All the ammo you need to master enums in C#. Whether you’re coding a console application or developing a game with the Unity engine, enums are your best friends.
They make your code cleaner, more readable and less error-prone. So, why don’t you give them a shot?
And remember to have some fun along the way! I mean, who said coding has to be dull and dry? The satisfaction you get from organizing your code beautifully with enums… it’s almost poetic, don’t you think?
As simple yet powerful as a magical spell – that’s the charm of enums for you! Cheers to clear, concise and efficient coding!
Happy programming!