Xamarin Interview Questions and Answers
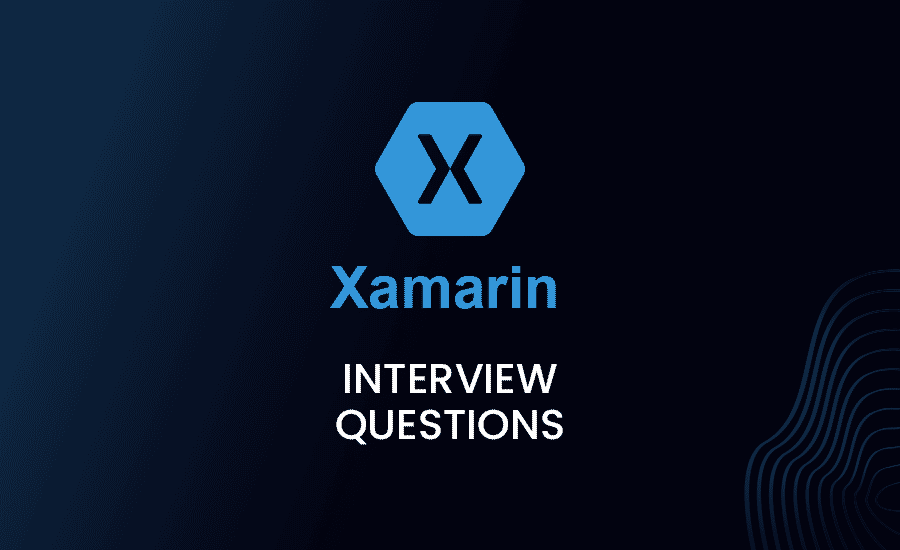
If you’re seeking a career in mobile application development or hoping to land a role as a proficient Xamarin developer, you’ve made a smart choice. The global demand for skillful Xamarin developers is on the rise and all you need is the right set of Xamarin relevant knowledge to make your mark.
While technical know-how is indispensable, being prepared to ace Xamarin interview questions is equally crucial.
In this comprehensive guide, we present some of the most commonly asked Xamarin, Xamarin Forms, and Xamarin Native interview questions to help you navigate the gauntlet of your upcoming interview with aplomb. Let’s get started.
How do you compile the Xamarin.iOS code for a native performance?
Answer
Xamarin.iOS leverages AOT (Ahead of Time) compilation to compile the Managed C# code into native ARM assembly code.
Here’s a brief on how it works:
- The C# source code is first compiled into IL code (Intermediate Language) using the .NET Compiler.
- Xamarin’s AOT compiler then compiles this IL code into native ARM assembly code.
This process ensures a native level of performance since ultimately, you’re running assembly code directly in the hardware. Xamarin does this to comply with Apple’s policy which doesn’t allow Just-In-Time compilation.
However, note that the complexity of the application and its utilization of system resources also significantly impact the overall performance.
How does the garbage collection work in Xamarin and how can it impact on mobile app performance?
Answer
Garbage Collection (GC) in Xamarin works in two steps i.e, Managed and Native.
- Managed: Xamarin uses Mono’s Simple generational GC, which works with managed objects in your code. Xamarin allows the Mono GC to handle all memory management for these objects.
- Native: Native objects (such as UI controls) are traced via the object graph to any associated managed instances and earmarked for collection.
The impact of GC on app performance can be significant due to the fact that garbage collection is generally a blocking operation where all the app’s threads are stopped. That’s why excessive or long garbage collection cycles can lead to UI jitter or sluggishness. Proper understanding and management of objects in Xamarin is essential to prevent such issues.
How would you handle background tasks in Xamarin.Forms across different platforms?
Answer
Xamarin.Forms doesn’t have a built-in mechanism to manage background tasks due to the differences in backgrounding techniques across platforms. Background tasks are platform-specific and it’s generally recommended to handle such tasks in the platform-specific projects.
For Android, you can use services and for iOS, you can use iOS Backgrounding APIs.
However, Xamarin also provides the Xamarin.Essentials Background Task API that abstracts the native Android and iOS backgrounding methods to run short-lived tasks in the background.
This way, it’s possible to write common code across platforms for simpler background tasks.
How does Xamarin handle platform-specific UI differences, like Material Design on Android and Human Interface on iOS?
Answer
Xamarin.Forms allows to share UI code across platforms but also respects platform-specific nuances like material design in Android and Human Interface in iOS. Here’s how:
- You can create native UI on each platform and utilize the Xamarin.Forms
DependencyService
to invoke these platform-specific UIs from the shared code. - Xamarin.Forms also provides platform-specific APIs that grant access to the underline platform controls. This allows devs to tweak control properties that are only available on specific platforms.
- Xamarin.Forms introduced Visual with its version 4.0. Visual is an API that allows for consistent UI across platforms. For example, you can use Material Design’s components not only Android but also on iOS or UWP by using Material Visual.
How can you handle iOS platform’s special characteristics like 3D touch or motion co-processor using Xamarin?
Answer
For such platform-specific features, Xamarin.iOS provides bindings to the native APIs, allowing you to take advantage of these features in your C# code.
- 3D Touch: Xamarin.iOS supports both Home Screen Quick Actions and Peek and Pop API.
- Motion coprocessor: Xamarin.iOS provides the Core Motion framework to access data from the device’s motion coprocessor. You can use the CMMotionActivityManager class to receive updates about the device’s motion and the CMPedometer class to get the step count.
Additionally, you may introduce platform-specific code in your shared Xamarin.Forms project with the help of DependencyService or by using Xamarin.Forms’ OnPlatform mechanism.
Having learned about handling platform-specific features that iOS offers, you might wonder how Xamarin.Forms further modernizes the cross-platform development approach.
Xamarin.Forms not only allows the sharing of business logic but enables UI code sharing across different platforms. One of the concepts that make Xamarin.Forms stand out is Xamarin.Forms Shell. In the next question, we explore it in detail.
How does the concept of Xamarin.Forms Shell change the traditional Xamarin Forms navigation paradigm?
Answer
Xamarin.Forms Shell was introduced as a way to simplify the app development experience by handling the complexities of mobile application structure and navigation.
Shell provides a declarative, simple way to define the flyout menus, tab bars, and navigation system of your app. Before Shell, these elements needed to be set up repetitively and individually in each app, making the process complex and error-prone.
Here are some of the ways Shell alters the traditional Xamarin.Forms navigation paradigm:
- Unified Design Across Platforms: With Shell, you can create a consistent application structure and navigation across different platforms. This eliminates the need for platform-specific coding for navigation-bar settings, tab bars, or hamburger menus.
- Single Place Configuration: Shell provides a single place to configure your app visual hierarchy instead of defining navigation in each page.
- URI-Based Navigation: Shell introduces URI-based navigation facilitating deep-linking within an app and receiving data from an app-link or web-link. This Declarative UI makes it much simpler to define relationships between pages.
- Integrated Search Handler: Shell also offers an integrated search-handler feature that provides search-box UI across the pages. It also exposes APIs to append the search bar on the top of the page.
Loading code snippet...
What are your steps to optimize the Xamarin Android Application startup time?
Answer
Optimizing the startup time of a Xamarin.Android app is crucial for enhancing the overall user experience. Here are some steps to achieve that:
- Use Latest Xamarin.Android Version: The latest Xamarin.Android versions contain a lot of optimizations to improve app performance, including startup times.
- Review Your Startup Sequence: Minimize work done during startup. Use lazy initialization for services and operations that aren’t immediately needed on startup.
- Use AOT (Ahead of Time) Compilation with LLVM: AOT compiles managed code (C#) to native code at build time, making startup faster.
- Use Startup Tracing: This is a feature of the Xamarin.Android platform that is used for improving an app’s startup time by initializing only essential methods during startup.
- Enable ProGuard or R8: These are code optimizer tools that shrink the code and resources of your app, including unused parts of the code.
- Use Fast Deployment in Debug Mode: Fast Deployment allows changes in the managed code to be deployed quicker.
How would you manage memory effectively for a Xamarin application?
Answer
Proper memory management is crucial for any application, including Xamarin. Here are some tips on doing it effectively:
- Objects Properly: Always dispose of unmanaged resources properly using the Dispose or using statement.
- Avoid Memory Leaks: Memory leaks happen when memory resources are not released even though they are no longer being used. To avoid this, Unsubscribe from events when no longer needed and not holding references for longer than needed.
- Use Weak References: If an object needs to refer to another object but doesn’t specifically require that object to stay alive, it can use a weak reference.
- Avoid Large Object Heap (LOH): Large objects (85,000 bytes or more) are stored in a separate heap. Minimize usage of large objects as it leads to memory fragmentation and increased GC time.
- Understand Lifecycle of Each Page: Make sure not any resources are being held up by pages even when they are not active.
- Utilize Profiling Tools: Xamarin Profiler and other profiling tools can track memory allocations and identify memory leaks.
How can you utilize Xamarin.Essentials for cross-platform app development?
Answer
Xamarin.Essentials is a set of essential APIs that abstract device-specific functionality to make them useable in shared code.
- Shared Functionality Across Platforms: Xamarin.Essentials provides APIs for common device functionality like Connectivity, App Information, Preferences/Settings, FileSystem, and more, with a shared code perspective.
- Consistency: Xamarin.Essentials promotes code sharing and consistency across platforms, thus reducing the time and complexity of developing cross-platform apps.
- Easy Integration: Xamarin.Essentials can be easily added to any existing or new Xamarin.Forms, Xamarin.Android, Xamarin.iOS, or .NET MAUI project.
Loading code snippet...
Discuss how you can target wearable devices with Xamarin. Which are the required SDKs?
Answer
Xamarin extends its potential with the ability to develop applications for wearables like Android Wear and Apple Watch.
- Android Wear: For the Android platform, Xamarin provides the
Android Wear SDK
to build apps. You need to install Android Wear emulator or an actual wearable device for testing the application. - Apple Watch: On the iOS platform,
watchOS SDK
allows developers to create apps for Apple Watch. Xamarin.iOS supports the creation of applications using WatchKit, the framework for developing Apple Watch apps. Both WatchKit app and WatchKit extension are required to run the application in Apple Watch.
Xamarin wearable apps can:
- Display notifications using native user interfaces.
- Synchronize data between a mobile device and a wearable device.
- Run independently on a wearable device.
Remember, the SDK version in use should match the version of the wearable device OS for compatibility.
Now that we have a solid understanding of how Xamarin supports application development for wearable devices, it’s time to shift our focus towards the challenges tied to Xamarin.Forms, particularly, data bindings.
Mastering data bindings is essential as they serve as a link between your application’s UI and business logic, minimizing the need for boilerplate glue code.
Let’s delve into some common issues that might arise with data bindings in Xamarin.Forms.
How can you describe some specific issues you might encounter when using data bindings in Xamarin.Forms?
Answer
- Property not updating: When you are dealing with data bindings, you might face an issue where the UI is not updating when the property’s value changes. This usually happens when the object doesn’t implement the INotifyPropertyChanged interface. In Xamarin.Forms, any property that needs to trigger a UI Change should implement this interface.
Example:
Loading code snippet...
- Type mismatch: Another issue might be a type mismatch between the source property and the target property. If you try to bind a string to a Color property for example, an invalid cast exception will be thrown.
- Binding to non-existent properties: Sometimes, while renaming or removing an old property, we forget to change the bindings, and we bind a property that doesn’t exist. Make sure that the properties that you are binding exist in your ViewModel.
- Thread Issues: Making UI changes from non-UI threads can lead to potential issues. It’s always safer to ensure that your property changed notifications are running on the UI thread.
How would you test a Xamarin mobile application for different screen sizes, resolutions, and hardware features?
Answer
Xamarin provides many ways to test your mobile application:
- Xamarin.UITest: This is a testing framework that enables Automated UI Acceptance Tests written in NUnit to be run against iOS and Android applications. It integrates with Xamarin’s test cloud service.
- Xamarin Test Cloud: You can use Xamarin Test Cloud to test your mobile applications on thousands of different device configurations.
- Emulator & Simulators: Xamarin applications can be tested using various emulators and simulators provided by Android and iOS. It’s important to test applications on devices of different screen sizes, resolutions and hardware features, to ensure that the application runs smoothly across devices.
- Physical Device: Testing on a physical device is the best way to see how your application will perform in the real world.
Explain the process of managing and accessing local databases using Xamarin.Forms.
Answer
Xamarin.Forms supports database-driven applications using SQLite, a lightweight database engine that is suitable for mobile apps. SQLite-net is an open-source library providing simple API’s for managing local SQLite databases in Xamarin.Forms.
Here are the steps for managing and accessing local databases:
- Specify SQLite-net NuGet packages: This package contains simple, easy-to-use object/relational mapping for the SQLite database in Xamarin.
- Create the SQLite Database: This involves creating a database, if it does not already exist, and creating the tables.
- Connnection: SQL.NET provides the SQLiteConnection class for .NET programming with SQLite. Establishing a successful connection can depend on the mobile platform in use.
- Performing CRUD operations: Just like with any other database, you perform Create, Read, Update, and Delete operations.
- Close Connection: It’s important to close the SQLiteConnection after the database tasks are finished.
How can Xamarin.Forms be integrated with Azure mobile app services?
Answer
Microsoft Azure Mobile App Service is a fully managed platform that provides features for building powerful mobile applications. Here are steps to integrate Azure with Xamarin.Forms:
- Create Azure Mobile App Service: First you need to create a new Mobile App Service in your Azure portal.
- Install Azure Mobile Client Libraries in Xamarin.Forms: To communicate with the Azure portal, you need to install the Mobile Client Libraries in your Xamarin.Forms application.
- Integrate Azure Database: In the Azure platform, you will easily setup the mobile app backend using Azure SQL databases for tables and stored procedures.
- Sync offline data: By using Azure, you can sync data which is added when offline, and push it to the server when the client is online again.
- Push notifications: Azure provides built-in support for push notifications.
- Setup Azure Authentication: Another major feature that can be used in your mobile applications from Azure is the social sites’ authentications like Facebook, Google, Microsoft and Twitter.
What is the role of DependencyService in Xamarin.Forms and how would you use it in your application?
Answer
DependencyService is a dependency resolver. It’s used in Xamarin.Forms applications to provide a way to register and locate dependencies. Those dependencies can be platform-specific implementations of a service.
It works in three major steps:
- Define Interface: Define an interface in your shared code.
Loading code snippet...
- Implement Interface on Each Platform: Implement the defined interface in each platform-specific project.
Loading code snippet...
- Resolve Dependency: Then you can use DependencyService in your shared code to resolve the interface and calls the platform-specific implementation.
Loading code snippet...
By using DependencyService, we can access the native functionalities and resources from Shared code in Xamarin.Forms.
Learning about the role of DependencyService in Xamarin.Forms bring us closer to understanding the communication mechanisms within the app.
One such mechanism that plays an imperative role in intra-app communication is the MessagingCenter. As we proceed to the next question, we take a closer look at MessagingCenter and its function in Xamarin.Forms applications.
How does ‘MessagingCenter’ in Xamarin.Forms work and how you can use it to communicate between different parts of your application?
Answer
MessagingCenter is a useful tool in Xamarin.Forms that allows decoupled communication between different components of your application. It works using the publisher/subscriber pattern where one part of your code publishes a message and another part of your code subscribes to that message and performs actions on receiving the message.
Loading code snippet...
In this example, a message named “MessageKey” with a string payload of “Hello from Publisher!” is published. Anywhere in the app that is subscribed to “MessageKey” will receive this message and can act accordingly.
How do you manage the Xamarin.iOS Bundle and its resources efficiently?
Answer
The Xamarin.iOS Bundle is a packaged output of your Xamarin.iOS application, containing the compiled code, resources, and metadata that iOS needs to run your app. Managing it efficiently involves various factors:
- Using Bundler Resource Packages (BRP): BRPs allow resources to be divided into separate packages, which can reduce the initial app download size.
- Optimizing image assets: Use vector images where possible; otherwise, ensure your raster (.png, .jpg, etc.) images are appropriately scaled and compressed.
- Managing localization files: Use iOS’s built-in localization framework to manage different language resources without bloating your bundle size.
- Lean Xamarin linking: Use Link SDK assemblies only or Link All to remove unused IL code from the assemblies in your app, reducing the final app size.
How would you handle image assets for different screen densities in Xamarin.Android?
Answer
In Xamarin.Android, handling image assets for different screen densities primarily involves using Android’s built-in res/drawable
directories mechanism. You need to place appropriately scaled versions of your bitmap images (for example, .png, .jpg, or .gif) in folders like drawable-ldpi
, drawable-mdpi
, drawable-hdpi
, drawable-xhdpi
, drawable-xxhdpi
, and drawable-xxxhdpi
.
A 1:1.5:2:3:4:6 ratio is typically used respectively for these folders when scaling your master bitmap image.
You should also consider using vector images (with the .xml or .svg format) where possible, as these can scale to any size without losing quality and without needing separate resources for each density.
Can you describe the use of Xamarin Inspector and Xamarin Profiler in the context of performance?
Answer
Xamarin Inspector is a tool that allows you to interact with your running app in real time. With it, you can inspect the UI, experiment with changes, debug data bindings, and much more. Xamarin Inspector can help you catch design-time issues that could eventually impact the app’s performance.
Xamarin Profiler is an invaluable tool for tracking performance issues in your app. It provides information about several critical performance aspects like memory allocation, garbage collection events, and method timing, helping you identify and fix performance bottlenecks.
It’s important to use these tools as parts of your regular development and testing cycles to ensure your app runs as efficiently and smoothly as possible.
How do you configure the environment settings for Xamarin.Forms? How do these settings affect your application?
Answer
Environment settings for Xamarin.Forms can be configured via the Project Settings in Visual Studio or application-level settings provided by the respective platform (AndroidManifest.xml for Android and Info.plist for iOS). These settings primarily control the behavior of your application, and among many other things, allow you to:
- Set the minimum and target versions of the platform SDKs your app should use.
- Configure permissions your app needs to function correctly.
- Set up app icons, themes, and other UI-related settings.
- Manage feature-based configurations like enabling ARCore in Android or ARKit in iOS, if your app uses Augmented Reality.
Changes to these settings can directly affect how your application operates, how it appears to the user, and what features it can access on the device. Therefore, proper management and understanding of these settings are critical to app development with Xamarin.Forms.
Congratulations on making it through this rigorous list of Xamarin developer interview questions! By now you should have a robust understanding of the complexities and enhancements Xamarin brings to the world of mobile application development.
However, remember that understanding the theory behind these tools and frameworks forms only part of the picture – practical knowledge and hands-on experience are key. Coupled with your persistence and problem-solving insights, these interview questions and answers will undoubtedly help you conquer your Xamarin interview.
Happy learning and best of luck for your forthcoming interview!