WPF Interview Questions and Answers
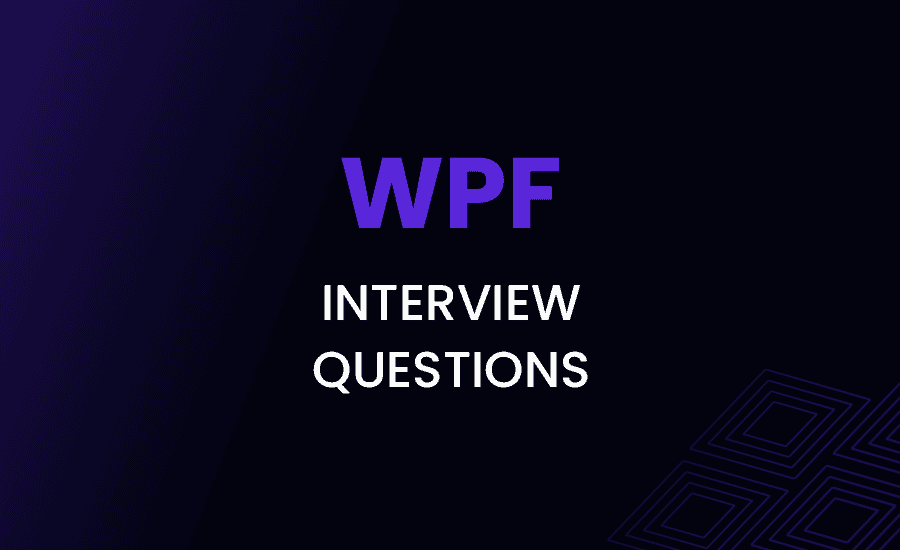
If you’re preparing for a WPF developer position, one requirement is to be well-rounded in your understanding of the Windows Presentation Foundation framework. This comprehensive guide lessens the complexity of that task.
It will arm you with several commonly asked C# wpf interview questions and vital wpf c# interview questions.
Prepare yourself for some deep-diving wpf mvvm interview questions as well. This list of extensive questions requires thoughtful and conceptual responses, showcasing your expertise and experience in WPF.
How would you explain the role of XAML in WPF application development?
Answer
XAML, standing for eXtensible Application Markup Language, plays a central role in creating UIs in WPF (Windows Presentation Foundation) Applications. Here’s how:
- UI Definition: XAML forms a declarative XML-based language that allows for defining UI elements. This means it is used to create, initialize, and set up the visual aspects of your application, including windows, controls, and other visual elements.
- Separation of Concerns: XAML facilitates the separation of UI design (View) from business logic (ViewModel). This enables designs to be created by designers using design tools that generate XAML, like Blend for Visual Studio, while developers focus on the functionality and business logic using C# or VB.NET.
- Data Binding: XAML, in conjunction with WPF, provide extensive support for data binding. This allows you to bind elements to data sources or other elements for dynamic updates, easing the process of keeping your UI in sync with your data sources.
For example, a button in XAML might be defined as follows:
Loading code snippet...
Can you describe some major differences between WPF and WinForms?
Answer
WPF and WinForms are two different approaches to creating Windows-based applications, each with their own strengths and features:
- Rendering: WinForms uses GDI+ for rendering its UI, whereas WPF uses DirectX. This implies that WPF can take advantage of hardware acceleration for enhanced performance and can support advanced graphics and animations.
- UI Definition: In WinForms, the UI is typically created imperatively in code, whereas in WPF, the UI is usually defined declaratively using XAML, allowing for a clear separation of design and code.
- Binding and Data Management: WPF supports robust data binding, allowing UI elements to be bound to data sources for automatic synchronization. This is not as straightforward in WinForms, which typically requires manual updating of UI components based on data changes.
- UI Flexibility and Customizability: WPF supports extensive customization of controls, styles, and templates, providing greater freedom to create custom application appearances, whereas WinForms has limited support for custom controls.
How do you implement dependency properties in WPF?
Answer
Dependency properties are a fundamental aspect of WPF. They enable WPF to compute the value of a property based on the value of other inputs. This is critical for features such as data binding, animations, and styles.
To declare a dependency property, you need to create a static readonly field of type DependencyProperty, and use the DependencyProperty.Register method in the field’s initialization, as follows:
Loading code snippet...
In the above snippet, "MyProperty"
is the name of the property, typeof(int)
specifies the type of the property, and typeof(MyClass)
sets the owner type of the property. new FrameworkPropertyMetadata(0)
establishes the default metadata for this property, including its default value.
In WPF, how can one implement data binding between two controls?
Answer
In WPF, data binding between controls can be set up in XAML. You can bind the property of one control to the property of another control by using the ElementName
property of the Binding
class.
Here is an example where the content property of a Label control is bound to the value of a TextBox control:
Loading code snippet...
In this example, any text you type into the TextBox will automatically appear in the Label control as well, thanks to data binding.
What role does the WPF Dispatcher play in making UI changes from a non-UI thread?
Answer
In WPF, all UI-related activity usually happens on the main thread or the UI thread. However, in certain scenarios, like performing background tasks or interacting with non-WPF technologies, we may need to update the UI from a non-UI thread.
Attempting to directly modify the UI from a non-UI thread typically results in an exception, as WPF’s UI components are not thread-safe.
Dispatcher comes into play here. It allows us to marshal work to the UI thread from a non-UI thread. With methods like Invoke()
and BeginInvoke()
, the Dispatcher class provides the mechanism to schedule the work on the UI Thread.
Here’s an example of how you might use it:
Loading code snippet...
In this example, a new action is created to update the text of a TextBlock control, which is then passed to the Invoke method of the Dispatcher. This action gets executed on the UI thread, thus safely updating UI elements.
Moving on from the core fundamentals of WPF, let’s steer our focus towards its intricate framework patterns.
The follow-up questions take a deeper look into the widely used pattern in WPF development, the MVVM, and its substantial benefits to project development.
Can you illustrate the MVVM pattern commonly used in WPF, and how does it benefit project development?
Answer
The MVVM (Model-View-ViewModel) pattern is a design pattern that facilitates a clear separation of the development of the graphical user interface (the view) from the business logic (the model) by introducing the ViewModel, which acts as an intermediary between the view and the model.
- Model: These are the data-access classes that bring data into and out of the application like databases, web services, etc.
- View: This represents the UI components of your application – all the screens and windows that make up what the user sees and interacts with.
- ViewModel: It acts as an interface between Model and View and introduces an abstraction of the view that exposes public properties and commands.
Benefits of this pattern are:
- Separation of Concerns: Designers can focus on the View, and developers can work on the ViewModel and Model parts in isolation, leading to a clearer development workflow.
- Testability: The separation allows for easy unit testing of the VM and Model without involving the user interface.
- Data Binding: The ViewModel exposes data from the Model in a View-friendly format, facilitating straightforward data binding in the View.
Here is a simplistic example of how you would bind a ViewModel to a view in XAML:
Loading code snippet...
In this case, a MainViewModel
is being set as the DataContext, or source of bound data, for the window.
What are some of the best practices to optimize the performance of a WPF application?
Answer
WPF applications can sometimes face performance issues due to their rich graphical rendering. Here are some common best practices to optimize the performance:
- Use the right layout containers: Choosing the appropriate panel for your needs can reduce unnecessary overhead. For instance, Canvas and StackPanel can be more performance-friendly than Grid in certain situations.
- Virtualization: If displaying large data collections, using ItemsControl with proper virtualization can significantly improve the performance.
- Bitmap Caching: For complex animations, turning on bitmap caching can speed up rendering.
- Avoid unnecessary data binding: Too many bindings can slow down your application. Make sure to remove any that aren’t necessary and optimize those that are.
- Asynchronous Programming: Perform long-running operations like file or database access on background threads to avoid UI freezing.
Can you describe how WPF manages memory and resources, particularly when dealing with complex graphics?
Answer
WPF makes use of DirectX and hardware acceleration where possible to handle complex graphics which generally provides a higher level of performance compared to GDI+-based systems like WinForms.
- WPF graphic contents are stored as Direct3D textures, which takes advantage of video memory where possible. This can off-load memory handling from the main system and make full use of modern-day graphics hardware.
- Unlike GDI which holds resources on a per-HWND basis, WPF shares resources across HWNDs (Windows) in an application. This reduces the need for multiple instances of the same resource and helps reducing the memory footprint.
For memory management, automatic garbage collection is largely responsible for cleaning up unused resources, but developers should still:
- Make sure to unregister any event handlers or timers on window close or when not needed anymore, to break any potential circular references and to ensure the garbage collector can successfully clean up.
- Dispose off unmanaged resources timely using the IDisposable interface.
- Use weak events where possible to avoid long-lived strong references preventing memory cleanup.
- Be mindful of static and otherwise long-lived objects that can retain references to large resource structures, unwittingly keeping them alive and inflating the memory footprint.
Could you explain how commands work in WPF, and how they differ from event handling?
Answer
Commands in WPF represent an object-oriented way to handle functionality that is triggered by the user, but separate from the event system that one might be used to from WinForms.
A command is an object that encapsulates what happens when a user interacts with a UI element and the conditions under which the interaction is valid.
A command differs from an event in that:
- CanExecute: Rather than simply responding to a user interaction like a click event, a command additionally has a
CanExecute
method, which determines whether the action can currently be performed. This allows WPF to automatically enable or disable UI elements based on command availability. - Loose coupling: Commands avoid the necessity of the view having any knowledge of the specific actions to perform, making them more flexible and better facilitating the MVVM pattern.
- Commands are most often used with input gestures, menu items, and buttons.
Here’s an example of a command in a ViewModel:
Loading code snippet...
In this case, a new RelayCommand (assuming it implements ICommand) is being created with methods for execution and determining executability.
How do Converters work in WPF, and can you provide an example of when you would use one?
Answer
In WPF, value converters are used when a binding needs to convert a value from one type to another. They are commonly used when the source and target of a binding are of different types and conversion is needed in between.
A converter must implement the IValueConverter
or IMultiValueConverter
interface, requiring the Convert
and ConvertBack
methods.
Here’s an example of a value converter that converts a boolean to visibility enum:
Loading code snippet...
In this case, the converter takes a boolean value and returns a Visibility enum, which would be useful for hiding and showing UI elements based on a boolean condition. ConvertBack is used for two-way bindings but is not implemented here.
You would use this in XAML like so:
Loading code snippet...
In this case, the IsVisible
boolean is being converted using BooleanToVisibilityConverter
, so that true
corresponds to Visibility.Visible
and false
to Visibility.Collapsed
.
Having traversed through in-depth discussions on data binding, converters, and commands within the WPF environment, let us handle the equally essential front of styles, templates, and namespaces.
The subsequent queries revolve around concepts that help to foster code reuse, maintainability, and logical organization in any WPF application project.
How do you accomplish bi-directional data binding in WPF, and what are some potential issues to watch out for?
Answer
In WPF, you can accomplish bi-directional data binding by setting the Mode
property to TwoWay
on your data binding:
Loading code snippet...
In a TwoWay
binding, changes in the target property automatically update the source property and vice versa.
Potential issues to watch out for:
- Performance Impact: Frequent updates in one end, leading to updates in the other end can lead to performance issues.
- Inconsistent Data/State: If there is a chance that updates at each end could contradict each other, you should carefully handle how your data updates occur.
- Exception Handling: If a conversion fails or a source/property cannot be found, the binding engine will throw an exception. So, handle these exceptions gracefully.
- PropertyChanged Event: Make sure to implement
INotifyPropertyChanged
event in your model or ViewModel to notify the changes. Else, two-way binding won’t work properly.
Can you use Styles and Templates in WPF to promote code reuse and maintainability?
Answer
Styles in WPF are used to apply a set of property values to an element. Templates, on the other hand, are the visual structure of an element. Both can help to promote code reuse and maintainability.
- Styles: You define a style once and apply it across multiple elements. It helps to maintain a consistent look-and-feel and simplifies any future changes.
- Templates: Templates allow for customization/redesigning of the existing controls without changing their behavior. It promotes reusability eg. you can create a template for a button that looks and acts like a CheckBox.
Example of a Style:
Loading code snippet...
Example of a Template:
Loading code snippet...
Can you explain what “visual trees” and “logical trees” are in WPF?
Answer
In WPF, the elements on your screen go through several stages of processing before they are displayed. Two important representations of your user interface are the logical and visual trees.
- Logical Tree: The logical tree represents the hierarchy of elements as you declared them in your XAML file. It includes all kinds of elements, like controls, content and layout elements. It’s used for high-level operations such as event routing, resource lookup, property value inheritance etc.
- Visual Tree: The visual tree is a more detailed breakdown of the logical tree. It describes the entire rendered interface, including visual components that you don’t declare in XAML. For example, it would break a
Button
control into constituent visual pieces such as aBorder
, aContentPresenter
, and aTextBlock
. It’s used primarily for rendering and hit testing.
Understanding these two trees can be crucial when fine-tuning the performance or functionality of your WPF application.
How would you debug a WPF application with complex data bindings?
Answer
Debugging data bindings can generally be done with a combination of the following techniques:
- Output Window: WPF writes out detailed error messages to the Output Window in Visual Studio when a binding fails. This may give you a hint about binding properties mismatches.
- TraceSource: You can use
PresentationTraceSources.TraceLevel
attached property to get a detailed trace of a specific binding.
Loading code snippet...
- Snoop Utility: It’s a third-party tool that allows you to explore the visual tree of a running WPF application. It also shows you all the bindings on a selected element and errors related to those bindings.
- Visual Studio’s Live Visual Tree and Live Property Explorer: Similar to Snoop, it allows you to inspect the visual tree of a running WPF application and see the bindings and errors.
How would you handle exception reporting in a robust WPF application?
Answer
Here are a few strategies you can use to handle exceptions in a robust way in a WPF application:
- Structured Exception Handling: Use
try/catch/finally
blocks to handle exceptions where the error can occur. - Global Exception Handlers: Handle the
Application.DispatcherUnhandledException
,AppDomain.CurrentDomain.UnhandledException
andTaskScheduler.UnobservedTaskException
events to catch any exception that was not handled elsewhere. - Throwing exceptions: Do not throw exceptions unless it’s an actual unexpected error. Exceptions are expensive and hence should not be used for flow control.
- Logging: Incorporate a logging library (like NLog, Serilog) to log any caught exceptions. This can be very helpful when diagnosing problems that occur only in production.
- Error Reporting: Consider using a crash reporting tool, like Raygun, which automatically catches unhandled exceptions and provides detailed error reports for better diagnoses.
Remember that ignoring all errors might lead to unexplained behaviour and further problems down the line. Always aim to fail fast in order to identify and fix issues quickly.
After discussing the heavy hitters of exception reporting and debugging in WPF, we now transition into an important and dynamic element of WPF development—creating dynamic screens and control elements.
The upcoming questions will throw light on this aspect and its importance in the overall UX.
If you need to create a WPF screen with dynamic controls (in number and type), how would you achieve this?
Answer
In WPF, you can dynamically create controls in code and add them to your UI. Below is an example of creating a Button dynamically and adding it to a StackPanel:
Loading code snippet...
However, in WPF, it is often more natural to use Data Templating for this kind of scenario. Here’s an example of using DataTemplate
with DataTemplateSelector
to decide the UI based on the bound data type:
Loading code snippet...
In your ViewModel:
Loading code snippet...
Here, MyDataTemplateSelector
will decide which DataTemplate
to use for each data item, based on their runtime type.
How does WPF handle the device-independent resolution and how does it help in creating scalable UIs?
Answer
WPF stands for Windows Presentation Foundation, one of the fundamental characteristics of WPF is its device-independent resolution. What makes WPF special is that it measures in device-independent units to ensure the UI maintains consistent sizing on all display settings.
In WPF, 1 unit is equivalent to 1/96th of an inch, regardless of the system DPI setting. This translates to approximately 1 pixel on a system configured for 96 DPI.
Therefore, elements in WPF are vector-based and not pixel-based, making them resolution and device independent. This means a WPF UI will scale properly and remain crisp & precise in different environments – no matter what monitor, DPI setting or resolution is in use.
Please demonstrate how you would create a custom control in WPF.
Answer
In WPF, creating a custom control involves the creation of a new class that derives from the Control
base class. The control’s appearance is defined by its control template, which you specify as XAML in a ResourceDictionary
.
Let’s say we want to create a simple custom control – a NumericTextBox
that only accepts numeric inputs.
First, create a new Class:
Loading code snippet...
In the class, override the OnPreviewTextInput
method:
Loading code snippet...
You can use this custom control like any other control in your XAML:
Loading code snippet...
Discuss the role that namespaces play in a WPF application and how they help in the organization of a larger project.
Answer
Namespaces in a WPF application play a very important role. They assist in the organization of classes that form part of an application. By organizing classes into namespaces and sub namespaces, you make your project structure cleaner and understandable.
For instance:
Loading code snippet...
Namespaces are also used in XAML to integrate controls defined in different assemblies or namespaces:
Loading code snippet...
Can you explain how animations work in WPF and list some typical use-cases for them?
Answer
WPF provides a powerful animation framework. Animations in WPF are time-based changes in the properties of an object.
Here’s an example of how we can animate the Width
property of a Button
:
Loading code snippet...
In this example, when the Button
is clicked, it triggers an animation that changes the Width
property of the Button
from 100 to 200 over 2 seconds.
Typical uses for animations:
- Create a visual feedback: eg. animate a button when it’s pressed/clicked.
- Attract the user’s attention: eg. shake a window when an error occurs.
- Indicate that a process is running: eg. an animated spinning wheel during a long-running task.
- Improve the overall user experience: eg. slide in/out upon navigation.
WPF is a powerful and multifaceted framework that developers use across a wide range of applications. Through this in-depth series of WPF interview questions, we hope you feel equipped and confident to excel in your upcoming WPF developer interview.
Remember, developing in WPF is not solely about knowing the concepts, but understanding how to apply these principles to create efficient, maintainable, and user-friendly applications.
Happy Prepping!