TryParse in C#: Full Guide
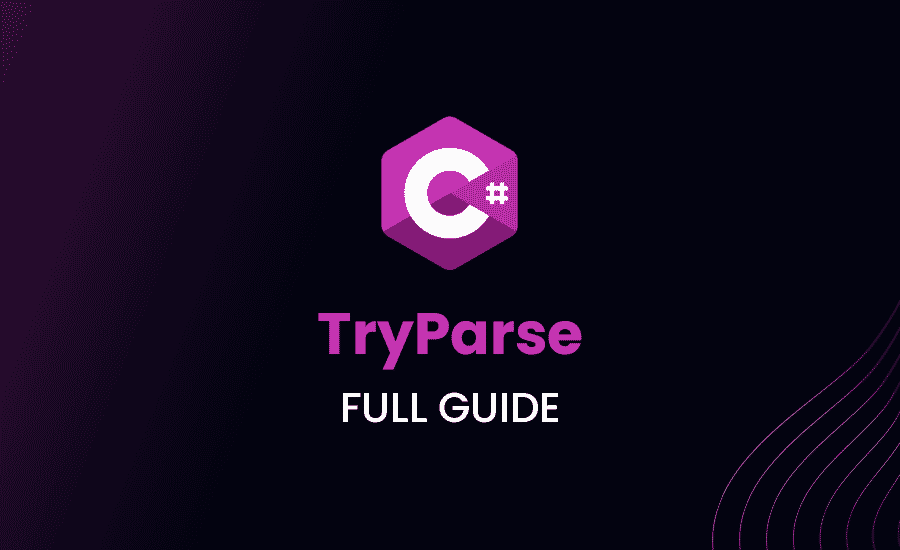
Have you ever wanted to master the TryParse method in C#? You’re in the right place; let’s dive deep into the world of C# parsing.
Understanding TryParse in C#
Ah, TryParse
. A real hero in our coding journey, wouldn’t you agree? It gracefully turns potential epic disasters into harmless little mistakes. But what does TryParse
do in C# exactly? Let’s see!
TryParse
is a special bit of magic that tries to convert a string into a specific data type. Instead of throwing an error when you hand it a strange input (like trying to convert “banana” into a number), it simply says, “Hey, I couldn’t do that!”, providing a false
Boolean result and leaving you unharmed. Great, isn’t it?
Loading code snippet...
In this code snippet, we’re politely asking TryParse
to convert the strings “123” and “banana” into integers. And as you already guessed, the first attempt works like a charm, but the second attempt flops—because, well, a banana is still a banana and not the number.
C# TryParse Overview
There’s so much more to TryParse
than meets the eye. Deep breath; we’re venturing into the exciting realm of parsing various data types! We’ll even venture into the lesser-known territories like decimals, hexes, and enums.
Working with Various Data Types
“But wait,” I hear you ask, “what data types can I TryParse
?” Well, brace yourself for some good news! It handles quite a few. Let’s get our hands dirty with some examples.
C# int TryParse
To start with an obvious one, TryParse
can translate strings into good old integers. Here, look at this:
Loading code snippet...
As we can see, parsing “456” to an integer is a breeze for TryParse
. However, it rightly chokes up on parsing an “apple” into an integer. It’s an apple after all!
C# Integer TryParse
Wondering what’s the difference between int
and Integer
in C#? Technically, nothing! int
is basically an alias for System.Int32
. So, Integer.TryParse
doesn’t exist in C#. When working with integers, stick to int.TryParse
. Crisis averted!
C# DateTime TryParse
What about dates and times? Can TryParse
handle them? You bet! Let’s see what happens when we try to parse string as DateTime.
Loading code snippet...
In the first attempt, the appropriately formatted date and time are successfully parsed. But the second attempt? Nope! The formatting here could be ambiguous to TryParse
.
C# Enum TryParse
Put your glasses on, folks! Now we’re going into some deep TryParse
territory: Enums. Yes, you heard right. Enums!
Loading code snippet...
As you see in our code snippet, parsing “Green” delivers a success. But when we try “Orange”, TryParse
draws a blank. And it adheres to case sensitivity too!
Double TryParse C#
Who doesn’t enjoy the precious precision of doubles? Here’s how TryParse
lets us play around with these delicate data types.
Loading code snippet...
“Tada!” exclaims TryParse
after parsing the “2.71828” string to a double. Isn’t that fantastic?
Float.TryParse C# Example
Floats are just like doubles, except we can’t shove in more decimal points. Let’s see how TryParse
handles these guys.
Loading code snippet...
Voila! It performs the conversion effortlessly. Isn’t TryParse
wonderful?
TryParse Boolean C#
Time for some truth! Or falsehood. Let’s see how TryParse
parses Booleans.
Loading code snippet...
Challenge triumphantly passed! It triumphantly parses the string into our Boolean variable.
Advanced TryParse Techniques
You know how superheroes have their secret weapons? For TryParse
, those are hexes, decimals, and strings. Want to see how? Fasten your seatbelts!
C# TryParse Hex
Hexadecimal values are everywhere around us. And TryParse
is all ready to take them on. Wait and watch!
Loading code snippet...
Advanced TryParse Techniques
Parsing standard data types is one thing. Budgeting for advanced types can often induce head scratching. But don’t worry, TryParse
has you fully covered. Let’s check out the proficiency of TryParse
with hexadecimal values, decimals, and more.
C# TryParse Hex
In the programming universe, hexadecimal values are omnipresent—the RGB color values for your new application’s UI, addresses in machine code, and whatnot. Now, these aren’t expressly in our friendly base-10 system. That’s where TryParse
flaunts its versatility.
Loading code snippet...
In this code snippet, “A” and “B” are parsed into 10 and 11 respectively, but “Z” returns false. There’s simply no numerical equivalent for a “Z” in the hexadecimal system.
Decimal TryParse C#
Decimals, owing to their scope for precision, are the darlings of division-heavy calculations. For example, calculating the batting average in Cricket or figuring out the fuel efficiency of your car call for decimals. And, TryParse
openly welcomes them.
Loading code snippet...
Here, TryParse
handles both instances like a boss. It converts both the string “3.14159265359”, the value of Pi up to eleven decimal places, and “-7.389056099”, which could be a obtained result in some high-math function, into decimal values. But, when it encounters “_9.87”, it fails and returns zero, indicating unsuccessful parsing.
TryParse String C#
Converting a string to a name? A mixed type perhaps? Let’s try to parse some complex types.
Loading code snippet...
In this case, TryParse
tries to parse “123HappyCoding” to an integer, but fails. Because the string has non-numeric characters. Then, it parses “567”, a string consisting of numeric characters, perfectly into an integer.
Conclusion of TryParse in C#
So there we have it, folks—a whirlwind tour of TryParse
in C#. From integers and floats to hexes and enums, we’ve seen it all. TryParse
really shines in saving us from the dreaded FormatException
and letting us deal with the unexpected in style.
C# TryParse
offers us a protective shield that makes code robust, adaptable, and error-resistant. Don’t just take my word for it; give it a shot! Unleash the power of TryParse
and watch as your code turns into a fortress that’s impervious to all kinds of exceptions.
Keep exploring and enjoying the magic of C#. Until next time, happy coding!