Timer Implementation in C#: Step-by-step Guide
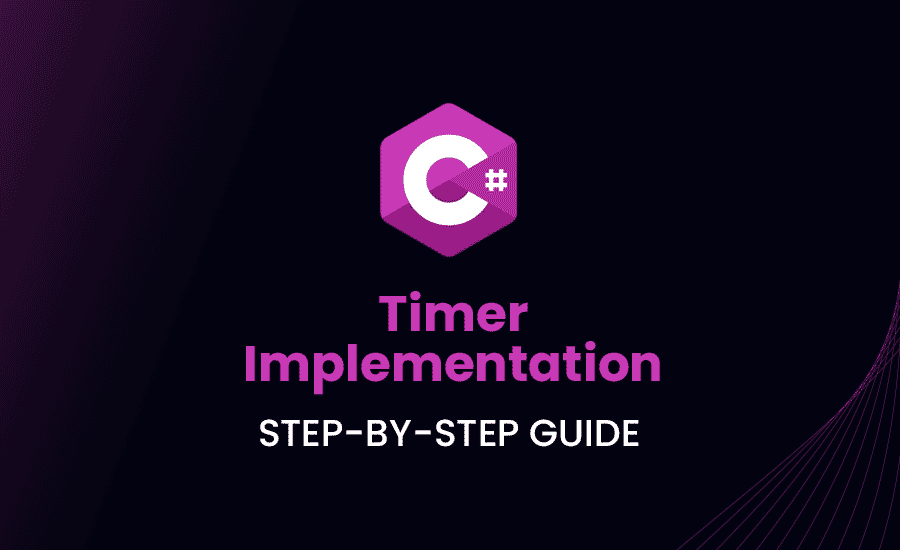
Have you ever wondered how time influences programming and how you could harness the ticking clock in your C# code? Well, don’t worry. Sit tight, grab a coffee, and get ready as we dive right into the heart of timer implementation in C#.
In this comprehensive guide, we’ll tell you all about timers, date times, and their numerous applications in C#. Along the way, we’ll consider exciting practical examples. Sounds interesting? I hope so!
Overview of C# Timer and its Usage
Picture this: You’ve written an application, and you want some pieces of it to execute at certain intervals. What comes to the rescue? A Timer! Timers in C# allow you to run functions or methods at defined time intervals or perform tasks in the future without manual intervention.
Understanding C# Timer
So, what is a Timer
in C#? To simply put, it’s a unique type of object that calls methods at specified time intervals.
Loading code snippet...
This small piece of code above just created a simple C# timer that ticks every 2 seconds. Not quite complicated, eh? But there’s more to it!
When to Use a Timer in C#
When do you need a timer in your C# code? Actually, anywhere time influences your project. Here are just a few scenarios:
- Performing a task regularly, such as checking the server’s status every 5 minutes.
- Running background tasks in desktop applications.
- Executing time-sensitive tasks, such as an online auction that ends after a certain period.
Creating a Timer in C#
Do you want to add an embedded clock in your application? Or is there a scenario where a delay in task execution is necessary? In that case, creating a Timer
comes to your rescue.
Step by Step Guide to Create a C# Timer
Making a timer is a piece of cake in C# if you know your way around it. Here’s a step-by-step easy peasy guide to help you:
- Import the
System.Timers
namespace to your project. - Create a new Timer object and set its Interval property in milliseconds.
- Write the method that does the desired task and attach it to the Timer’s Elapsed event.
- Finally, start the Timer by setting the Enabled property to true.
Loading code snippet...
C# Timer example: Creating a Working Timer
But what fun is programming without seeing the code in action? Let’s create a simple console application to see our timer ticking!
Loading code snippet...
This simple console application creates a timer that ticks every second, emitting a console message with the current time.
Working with Date Time in C#
It’s time to take a step further into the realm of time, stepping into dates. Dates wielded with timers could make your application quite versatile.
Converting C# String to Date Time and Vice-versa
As developers, we often face the need to convert strings into DateTime objects and vice-versa. Thankfully, C# provides a neat and straightforward way to do this.
Loading code snippet...
This example illustrates how it’s done. In two succinct lines of code, you have transformed strings into DateTime objects and vice-versa.
Understanding Date Time Format in C#
Now, you may ask, what’s the deal with that "yyyy/M/dd"
? That, my friend, is DateTime format in C#.
Loading code snippet...
See how "MMMM dd, yyyy"
in the ToString()
method spontaneously transformed the current date into a pleasant, human-readable format? You just unlocked the power of DateTime formatting in C#!
Getting the Current Date Time in C#
Does your application need to know the current date and time? Worry not! Acquiring the current DateTime is no sorcery in C#.
How to Get C# Current Date Time
Getting the current Date and Time in C# is as simple as calling a method of the DateTime class. It’s as if you’re asking C#, “Hey what’s the time?”, and C# responds promptly.
Loading code snippet...
Run this code, and C# will tell you the precise date and time.
Practical example: Fetching and Displaying Current Date Time
Let’s try this in a real-world console application that continually updates and displays the current time.
Loading code snippet...
This application prints the current time to the console every second. Just the perfect blend of Timer and DateTime!
Interactions between Timer and Date Time in C#
As we dive deeper into the world of C# time programming, things may seem intertwined but with more power comes more possibilities.
The relationship between C# Timer and Date Time
The Timer and DateTime classes in C# are two sides of the same coin. A Timer represents a constant ticking clock, notifying events at specific calendar times, while DateTime deals with specific moments in time.
Applying Date Time Formats in Timer
Besides, using DateTime inside a Timer not only lets you schedule tasks but also format the time as you fancy.
Loading code snippet...
This timer ticks once every second and prints the current time in hours, minutes, and seconds—a simple example of how DateTime and Timer interact in C#.
Transformations in C#: Date Time to Unix Time
In our journey, we’ve arrived at the final stage. So far, we’ve talked about time based on human calendars. But the computer world has a unique way of seeing time. Ever heard of Unix Time?
Understanding Unix Time and its Interactions with C# DateTime
Unix Time, also known as POSIX time or Unix timestamp, is a system that records time as a running total of seconds, not considering leap seconds. But, how does C# factor into this?
Loading code snippet...
Converting C# DateTime to Unix Time
Before you know it, you’ve transformed your C# DateTime object into Unix Time. But don’t just take my word for it—let’s walk through a simple console application that outputs the Unix timestamp every second:
Loading code snippet...
This console application uses a Timer to get the current Unix time every second and even displays its value in the console—a perfect demonstration of DateTime and Unix time in C#.
Common Errors in C# Timer Implementation
While C# provides us with a remarkably friendly environment to implement timers, a few recurring errors rear their heads during their implementation. Identifying these typical errors early in your development process is the first step towards bug-free, efficient timer implementations.
ObjectDisposedException error
This error happens when you try to access a timer that’s already been disposed of. To avoid this, stop the timer before disposing of it by setting the Enabled property to false and invoking the Dispose method subsequently.
Loading code snippet...
Losing Reference to the Timer
The garbage collector can collect our timer’s thread if there aren’t any live references. So, it’s wise to keep a reference to your timers, especially if they need to run for an extended period or throughout the application’s life cycle.
Loading code snippet...
Unhandled Exceptions in the Elapsed Event
Unhandled exceptions within the Elapsed event handler can crash your application. Enclosing your operations with a try-catch block can help you handle these exceptions gracefully, providing a safer environment for your timer to operate in.
Loading code snippet...
Imprecise Timer Intervals
Timers in C# aren’t well-suited for precise timings. For instance, a Timer configured with an interval of 2000 milliseconds might fire anywhere between 1999 and 2001 milliseconds. This lack of precision is vital to incorporate when planning tasks.
Optimizing Your Timer for Better Performance
Having dealt with the pitfalls, consider now improving performance. A well-crafted timer can significantly enhance the execution of your code and provide a seamless user experience.
Adjusting Timer’s Interval
A Timer in C#, when started, waits for the interval set before raising the Elapsed event. The time you set isn’t always most optimal – you might want the Elapsed event to occur immediately once the Timer is started, especially when working with longer intervals. By adjusting the Timer’s interval, we can achieve this.
Loading code snippet...
This code sets an initial interval of one minute for the timer. Once the interval has elapsed, the TimerElapsed event changes the interval to five minutes, reducing unnecessary ticks.
Conclusion
You’re no longer a novice, my friend—you’ve just taken a thorough walkthrough of using timers in C#. It’s like a magic watch was handed to you! But, remember, with great power comes great responsibility.
Keep doing magic with your code, and happy programming!