Using Switch Statement in C#
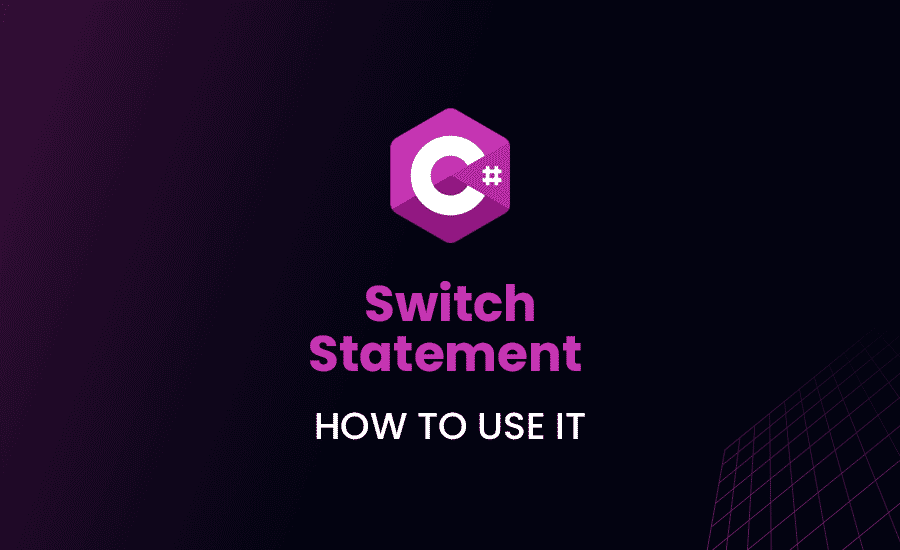
Let me take a wild guess here! You’re coding in C# and you’re finding yourself in a bit of a pickle dealing with conditions, aren’t you? Been there, done that! It’s time to come to the switch statement’s rescue. Let’s unravel its power and see how it can make your life easier, while also making your code cleaner, more manageable, and efficient. Sound like a plan? Great, let’s dive right in!
Introduction to Switch Statement in C#
Ever found yourself in a situation writing a zillion ‘if’ statements to deal with conditions? Let me tell you, friend, those days are long gone! Let’s talk about a secret weapon that goes by the name of ‘Switch Statement’. In this section, let’s understand what this so-called Switch statement is, and what magic it can do once unleashed.
What is a Switch Statement in C#
In simple terms, a switch statement is a sort of control statement in C# that allows a variable to be tested for equality against multiple variants. It’s your ‘One Ring’ to rule all the different ‘if’ statements.
Loading code snippet...
From the above simple example, you can infer that the switch statement checks for the value of ‘option’. Each case represents a different output for the values 1 and 2. If it doesn’t match any, then the ‘default’ case is executed.
Now, can you imagine doing the same with ‘if’ statements? Doesn’t this feel like a breeze? See, I told you it’s a secret weapon!
Deep Dive into C# Switch Case Feature
Dealt with ‘if’ statements? Check! Understood what a switch statement is? Double Check! Time to deep dive and understand the notorious duo of ‘Switch Case’ in C#. In this section, I’ll show you how to use and master this powerful feature and turn your comparative code into a sleek masterpiece.
How to Use C# Switch Case
Before I show you how to use a switch-case, let me clarify—think of the switch as a dispatcher. It dispatches execution to one or many blocks of code called ‘Case’. Here’s the syntax:
Loading code snippet...
“Alright, you’ve spoken enough! Show us the magic!” Well, fine! Here is a simple calculator using a switch-case that determines the operation to be performed based on operator input.
Loading code snippet...
It’s not as grim as ‘if’ statements, is it? The cleanliness of the code! Ah! The beauty! The brilliance! Can you see it too? This is the power of switch-case!
C# Case Statement: Best Practices
Before we put this section to bed, let’s go through a couple of best practices when dealing with the switch case.
- Try to avoid nesting switch statements. This decreases readability and increases complexity.
- Always use a ‘default’ case, even if it’s just for logging or throwing an exception. This helps for future debugging.
- When possible, use enumeration instead of primitive data types for the switch expression. This makes the code more self-documenting.
Unraveling C# Switch Expression
C# 8.0 introduced a new concept called ‘Switch Expressions’. This is some high-level stuff! It’s like the switch case, but with superpowers! It gives you a cleaner, simplified syntax. Let’s unravel this together in the next sections, where I’ll show you the syntax and upgrade our calculator to use switch expressions.
Understanding C# Switch Syntax
Here’s how the switch syntax has been simplified using switch expressions:
Loading code snippet...
In this syntax, TestVariable
is the value to match and TestValue
and AnotherTestValue
are the match expressions. The underscore _
is used as a catch-all matching all other values that were not matched by previous case expressions.
Unveiling C# New Switch Syntax
Using our good old calculator example, let’s upgrade it using the new switch syntax.
Loading code snippet...
Note that the code is way cleaner and can return a value, unlike the classic switch statement.
Practical Examples of C# Switch Expressions
After all this information, it’s time to put it into practice, right? Let’s hammer down the concept with some real-world examples using our shiny new switch expressions.
How to Implement C# Switch Expression Examples
Say we are building a game and we need to make decisions based on the character class. Let’s see how we can do that using a switch expression.
Loading code snippet...
This way, we get a clean and effective way to deal with different outcomes based on the character class. Boom! Game development just got a bit easier!
Advanced C# Switch Syntax Examples
As we’re all now smooth operators handling the switch expression, let’s turn it up a notch. Let’s take a look at nested switch expressions.
Loading code snippet...
With nested switch expressions, we have increased the power of the switch statement and the possibilities are now endless!
Conclusion: Switch Statement in C# Demystified
Take a breather! That’s a lot to take in, I know. But look at what we have accomplished. From understanding what a switch statement is to demystifying C# Case statements, Switch expressions, and even advanced nested switch expressions! Ladies and gentlemen, we are now the masters of switch statements in C#!
How Mastering Switch Statement Can Enhance Your C# Coding Skills
Mastering the switch statement can enhance your C# coding skills in multiple ways, from improving code readability to decreasing complexity.
- Improve readability and simplicity: Switch statements are more compact and provide clear, concise logic differentiation.
- Decrease complexity: Switch simplifies multiple conditions handling, keeping the code DRY.
- Enhance performance: Switch statements with many cases are faster than equivalent ‘if-else’ chains.
Becoming a switch maestro, you’ll wield the power to write cleaner, faster, and more efficient code. After all, don’t we all strive to become better coders, touching the zenith of coding greatness? So take all this, my compadre, and go code!
Now, if you’re still playing coy, ask yourself- aren’t you keen to start optimizing your code right now? After all, who doesn’t love a cleaner, more efficient C# codebase? Ignore the benefits of switch statements in C#, and your future self might not be happy about it! So, why delay? Switch up your coding game today!