StringBuilder in C# – Basic & Advanced Tips
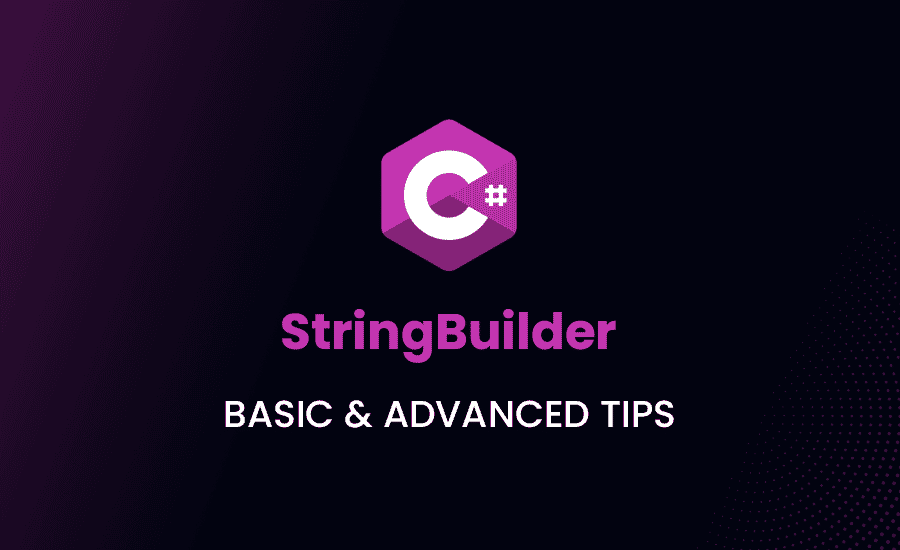
As a C# developer, you may be familiar with string concatenations and manipulations. But, have you ever paused to consider their performance impact or if there is a better way? If you’ve nodded in agreement, this is the perfect moment, as we will delve into the depths of StringBuilder in C#. Buckle up and join me on this fun ride!
Understanding StringBuilder in C#
Hey, let me grab your attention for a moment! Imagine the numerous ways you’re currently manipulating and concatenating strings in your code – costly, right? That’s where StringBuilder comes to your rescue!
What is StringBuilder in C#?
StringBuilder in C#, my friend, is a mutable sequence of characters. It’s a fascinating class in the .NET Framework, fast and efficient in situations where you need to perform repeated modifications to a string. Think about the numerous times, you’ve had to append new data to strings!
Loading code snippet...
The above code snippet paints a picture of how a StringBuilder comes to life in C#. It’s astonishing how StringBuilder leverages memory and performance by not creating new string instances each time you append.
Differences between C# String vs StringBuilder
You might question, “Why not stick to using string for simple manipulations?” Well, it’s high time we delved into the differences between C# String and StringBuilder. As you may be aware, strings in C# are immutable, meaning that every time you modify a string, a new instance is created. On the flip side, StringBuilder is mutable, allowing you to make changes without the overhead of creating new instances.
Say goodbye to the costly string concatenation and hello to the efficient StringBuilder!
Working with StringBuilder in C#
Alright, hold your breath! We’re about to dive into the gritty details of how to work with StringBuilder in a real-world setting.
C# StringBuilder Example
Wondering how StringBuilder is implemented in a real-world C# scenario? Let’s dive into an example:
Loading code snippet...
This scenario demonstrates Builder’s ability to append to an existing string without creating new string instances. Notice how the Append method was used to add a new string to the already declared StringBuilder. Exciting, isn’t it?
Using StringBuilder Append in C#
In our journey with StringBuilder, we will inevitably cross paths with the Append method. The Append method, my friend, is your ultimate tool when adding more strings to your existing StringBuilder. It does this efficiently without having to create a new string each time you append, which means saying goodbye to the unneeded overhead.
Here’s an example:
Loading code snippet...
With this simple example, I hope you recognize how Append can turn your string manipulations into a breezy experience.
Advanced operations in StringBuilder
Do you ever get the feeling that coding is similar to cooking? You mix ingredients (codes) to create a delicious meal (program). Well, StringBuilder has a lot of features that serve as ingredients to your solutions, and today, it’s time to add more flavor.
How to split data using StringBuilder in C#
So, you’ve been appending. Now, it’s time to throw a curveball into the game: splitting! Yup, StringBuilder can do that too. Let’s check out an example:
Loading code snippet...
Common Tips and Useful Scenarios for Using StringBuilder
- Use StringBuilder when you need to make a significant number of modifications to a string.
- The Append method is your best friend when adding to your StringBuilder.
- Convert StringBuilder to string using ToString() when you want to perform operations like splitting.
Summary and Conclusion
Throughout this session, we’ve used StringBuilder to our advantage, from understanding its purpose to witnessing its power in action. The ball is now in your court: apply StringBuilder to make your code more efficient and say goodbye to unnecessary overheads!
So, are you ready to bake up some memory-efficient and faster codes in C#? Are you ready to embrace the power of StringBuilder and slay those dreadful overheads? Let’s get coding then!