Sorting Lists in C#: Easy Tutorial
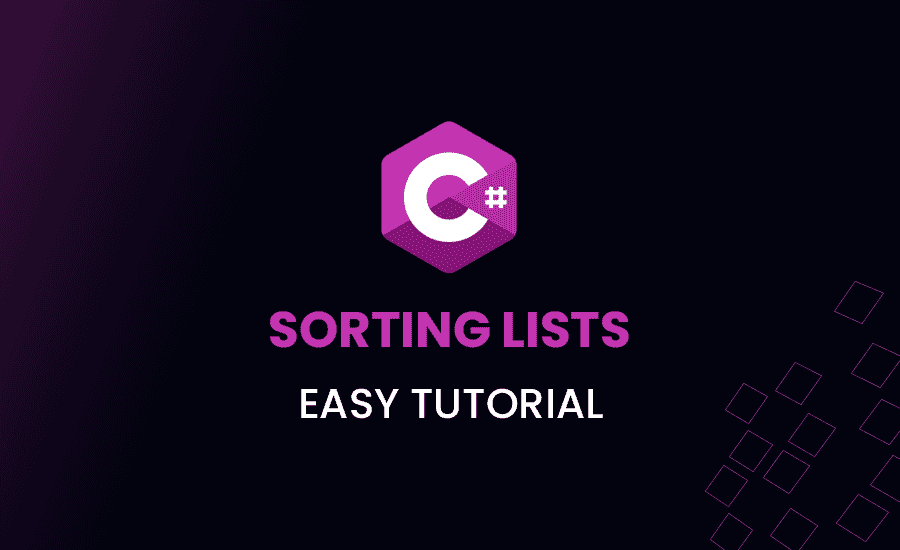
Wow, I bet you’re a doer. You’re here because you’re interested in learning the ins and outs of sorting lists in C#. And guess what? You’re in the right place! We’re going to cover everything from the nitty-gritty basics to advanced sorting techniques. Ready to improve your C# know-how? Let’s do this.
Understanding C# List Sorting
You’re probably asking yourself, “What’s so special about C# list sorting?” Right? Well, it’s not just about arranging items. It’s about controlling data in a way that makes your software faster, smarter, and more efficient. So, how about we take a closer look?
Brief Introduction to C# List Sort
A List in C# is an ordered collection of items. Sorting those items allows you to arrange data as per specific variables, which in turn, aids in data analysis and algorithm efficiency. Not sure what I mean just yet? Let’s put it into code terms.
Loading code snippet...
This example demonstrates how to create a list of integers and sort it. After calling the Sort()
method, the list numbers
will be sorted in ascending order, i.e., {1, 4, 5, 8, 10}
. Cool, right?
Climate towards C# List Sorting
The overall attitude towards C# list sorting is positive. Most programmers appreciate its simplicity yet, extensive applicability. Yet, a few humps may pester beginners. But hey, don’t sweat, that’s why we’re here!
How to Sort a List in C#
Whether you’re a newbie or you’ve coded in C# for a while, sorting a list could cause a few pinches here and there. But once you get it down, you’ll find it’s actually quite straightforward.
Basic steps on how to sort the list in C#
First off, we need to create a list. Next, we apply the Sort()
method. Sounds easy, huh? Let’s look at an example:
Loading code snippet...
With that, your list, previously {"Apple", "Cherry", "Banana", "Date"}
, now transforms to an alphabetically sorted list {"Apple", "Banana", "Cherry", "Date"}
. Yeah, it’s that simple!
Common challenges in C# list sorting
Despite its simplicity, programmers often encounter hiccups when sorting lists in C#, especially with complex cases. Navigating null values, sorting by multiple properties, or handling special character strings? Those could be tricky. But, guess what, we’re going to tackle them in this article!
C# Sorting a Specific Type of List
Even though C# has a general sorting method applicable to most lists, some specific types require a unique approach, like linked lists and lists with DateTime
objects. But worry not, we’ve got you covered!
How to sort a linked list C#
A linked list is a sequence of nodes where each node points to the next node. To sort it, we convert it to a list, sort the list, and convert it back. Surprised? Let’s look at the code!
Loading code snippet...
In this example, our linked list linkedNumbers
is sorted into {3,5,7}
using Linq’s OrderBy method. We simply convert it to a list, sort, and then change it back. Neat, right?
Exploring C# int list sort
Sorting a list of integers is even more straightforward. If you’ve been paying attention, you already know the drill – creating, sorting, et voila! Here’s what it looks like in action.
Loading code snippet...
Now you’ve got a neatly ordered list {2, 3, 5, 7, 9}
. Could it get any simpler?
Techniques to sort list by date C#
Sometimes, you might want to arrange a list containing DateTime
objects, like sorting tasks by their due dates. The Sort()
method still comes to your rescue. Let’s see how.
Loading code snippet...
There you have it. The dates in chronological order. Sort()
has once again worked its magic!
Advanced Sorting Techniques in C#
While the Sort()
method is quite versatile, for more complex scenarios, you may need to get into some of the more advanced options. Here, we’ll explore techniques such as sorting a list by a property, a field, or even alphabetically.
C# sort list by property: A Comprehensive Guide
Want to sort a list of objects by a specific property? For instance, let’s talk about a list of students. If you wanted to sort them by name or age, how would you do that? Thanks to C#’s Lambda expressions, you can easily achieve this!
Loading code snippet...
There you go, our list of Student objects is now sorted alphabetically by Name.
Techniques to sort list by field in C#
The field of an object can get easily sorted. It’s almost identical to sorting by properties, only that fields are not obtained through methods.
Loading code snippet...
How to sort list alphabetically in C#
Alphabetical sorting goes back to the basics. We use the standard Sort()
method, and it orders our string list in alphabetical order. It’s just how it naturally works!
Loading code snippet...
Well, it can’t get easier than this, your string list will now look like this: { "Apple", "Banana", "Grapes", "Mango", "Zebra" }
. It’s all in the ABCs!
A Guide to sort list by multiple properties in C#
Sometimes you might want to sort a list of objects by multiple properties. Like having a list of people that you want to sort first by their last name, then by their first name. Seems tricky, right? Not when you break it down!
Loading code snippet...
With that, your list arranges first by LastName
, then by FirstName
. This feature is a handy tool for complex sorting scenarios.
Ascending Vs Descending Sort: C# List Sorting Techniques
Most of the time, you’d want to sort a list in ascending order. But sometimes, you might need to flip things upside down. So, let’s discuss both ascending and descending sorting in C#.
Tips on C# list sort ascending
Normally, the Sort()
method orders a list in ascending order by default. You’ve seen this through our examples, whether with numbers, strings, or dates. Right? If you need a refresher, here’s a simple example.
Loading code snippet...
The list numList
turns out as {2, 3, 5, 7, 9}
. It hits the ground running from the lowest to the highest number, just like we planned.
Insights to C# list sort descending
Want to turn the tables and sort your list in descending order? Usually, you reckon you need to call the Sort()
method and then reverse your list. But what if I told you that you could achieve it in one line! You don’t believe me? I bet you can’t wait to find out how!
Loading code snippet...
Our list numList
sorts as {9, 7, 5, 3, 2}
. Yes, you’ve read it right! We started from the highest to the lowest number with a single line of code. Impressed yet?
Evaluating C# Sorted List Performance
Now that you’ve mastered the art of list sorting in C#, it’s crucial to note that what matters most is the efficiency of your code. So, how do you evaluate this?
Challenges in C# sorted list performance
While list sorting in C# is highly efficient, you might sometimes encounter performance issues, especially when dealing with large lists. Typically, this happens when you are constantly adding and removing objects from your list. To overcome this, sorted dictionaries or priority queues come to your aid.
Best Practices to Enhance C# Sorted List Performance
Let’s explore a few tips to gear-up your sorted list performance:
- Use
Sort()
only when necessary and when all elements have been added to the list. - For lists, avoid using
Add()
, go forAddRange()
. It’s faster! - Use
Capacity
constructor when the expected number of elements is known; it saves the expense of growing the List.
Final Thoughts: Mastering List Sorting Techniques in C#
You did it! You’ve trudged through the intricacies of list sorting in C# from the basics to advanced techniques. If there’s one takeaway, it’s that list sorting techniques are fundamental to improving your program’s performance. And remember, keep practicing. The more you code, the better you get. So, what are you waiting for? Get coding!