Reversing a String in C#: Step-by-Step Guide
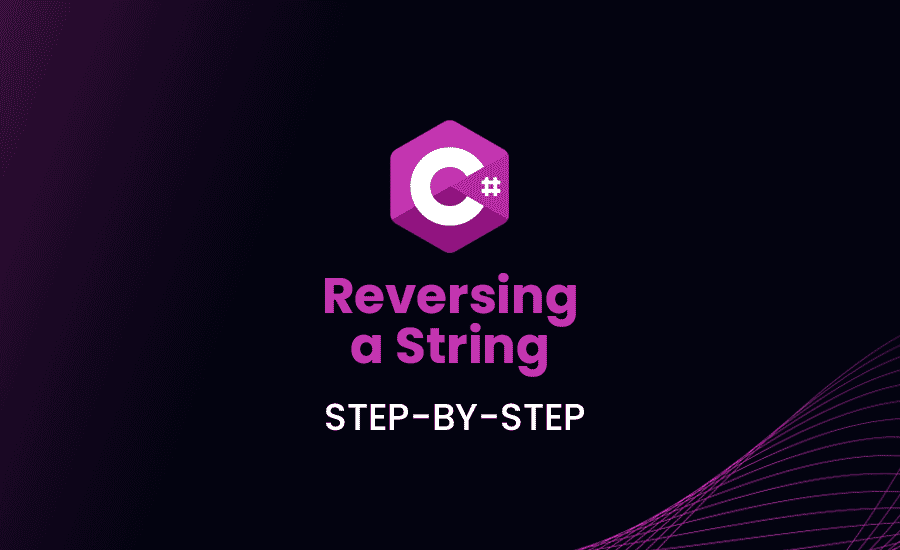
Understanding the logic behind reversing a string in C# can be quite enlightening. Are you asking why? Well, it helps you not only with textual data manipulation but also hones your problem-solving acumen. Right, now let’s flip the script with strings in C#!
You may be like Neo in the Matrix, in front of two pills, one to continue down a recursive path and the other to reverse it all. Ready? Let’s dive in.
Introduction to Reversing a String in C#
Strings and their manipulation form the core of many programming and coding concepts in C#. But what is string reversal and why should you bother about it?
- String reversal is an integral part of programming that involves reversing the order of characters in a string.
- It plays a crucial role in various operations, including palindrome checking, algorithm development, problem-solving, and data structure concept enforcement.
Confused? Don’t be. We’re about to break it all down and make it as simple as pie.
Understanding Strings in C#
In C#, strings are an array of characters, each having a unique index. They’re not just a jumbled array, but like a pearl necklace, where each pearl has its specific place. Just as you’d remember a serial number by starting from the left, computers start indexing arrays from 0. Let’s take a simple Hello
string for instance. Here we go!
Loading code snippet...
Yes, that’s right! The first letter is not at position 1, but 0. Programming often means forgetting everything you know and learning it from a different perspective.
Detailed Overview of the C# String.Reverse Function
Before we proceed, let’s understand something crucial: there’s no built-in String.Reverse
method in C#. You’re about to tell me I’m wrong, aren’t you? But trust me, it is for arrays, not strings. Such an idea sprouts easily because strings are character arrays, but not everything applying to the father applies to the son!
So, how to reverse a string? There is more than one way to do it, and we are going to rectify this unrequited need for a String.Reverse
method with some practical examples.
How to Reverse a String in C#: Step-by-Step
When you venture into reversing a string in C#, there are two basic requirements you need to have: an installed .NET platform and a text editor or integrated development environment (IDE) that supports C#. Once you have these tools ready, brace yourself, put on your coding helmet, and start on this thrilling ride to string reversal.
C# Reverse String Method
Remember how I told you there was no in-built method? Well, I didn’t say we couldn’t write one ourselves. Allow me to introduce the revered manual approach. This technique precisely defines the term “reversing a string” as we’ll manually swap the characters from the beginning and the end. Kinda like those annoying “You hang up, no, you hang up first” phone conversations. Only here, it makes perfect sense.
Loading code snippet...
The beauty of this method is that you have control over each character, somewhat like a puppeteer–a code puppeteer!
Different Ways of Reversing a String in C#
Shall we explore other ways to reverse a string in C#? From using arrays to string manipulation, the possibilities are as vast as a coder’s coffee choice!
Deep-Dive into the C# Reverse String Array
Here’s another plot twist: Did you know you could reverse a string using an array? This method takes the elements of the string (as a character array) and reverses the array. It’s somewhat like reading a book backwards.
Loading code snippet...
Pretty cool, right? But, this is just dipping your toe into the pool.
Using C# String Reverse Method
Let’s get back to our previous model where we manually reversed a string. But hey, you want to feel lazy sometimes and get your work done. That’s where the LINQ approach comes in handy.
The Efficiency of the C# Reverse String Method
The LINQ approach can appear quite quirky due to its chain-like structuring- connecting various extension methods. But remember, efficiency comes with practice.
Loading code snippet...
So what just happened? We used the Reverse method (mainly for arrays) on the string, turned it back into a string, and voila–the reversed string is ready!
Advanced Tips and Tricks for String Reversal in C#
How about we dive deeper into the coding ocean? You thought reversing a string was a simple task, akin to flipping a pancake? Well, the simple exercise of string reversal can take on monstrous proportions when it comes to advanced programming. Let’s delve into the broader realm and explore some advanced touches to reversing a string in C#.
Using the StringBuilder
As opposed to simple strings, StringBuilder
in C# is mutable, meaning you can change its value without creating a new object everytime. StringBuilder
can be beneficial when you’re incessantly append characters to a string, like in a loop.
Let’s ponder over an example to make things clearer.
Loading code snippet...
In this code, we’re initiating a StringBuilder class. We go through the original string character by character in reverse order (i = sampled.Length - 1; i >= 0; i--
) and append each character to the StringBuilder. This contraption generates our desired reversed string.
Using Stack
Next up, we look at how Stack can help reverse a string. A Stack is a Last-In, First-Out (LIFO) data structure – think of it as a pile of plates. The plate you place last (on the top) is the first one you’ll pick up. Let’s apply this principle to our string reversal.
Loading code snippet...
Here we push all the characters in the string into a stack and then retrieve them. Because of the stack’s LIFO nature, the characters come out in reverse order, rendering a reversed string.
Using Recursion
Another advanced string reversal method is to use recursion, that’s repeatedly applying a solution to a smaller instance of the same problem. Sounds complex, right? Let’s break it down.
Loading code snippet...
In this code, we’re repeatedly slicing off the first character of the string and joining it at the end, until we have a single (or no) character left.
Concluding Remarks on String Reversal in C#
So, here we are at the end of our journey through the wonderful world of reversing strings in C#.
What were some big revelations? First, there’s no in-built function to reverse a string in C#. Second, we can manually reverse a string, and third, a lot of other methods can get the same results, each having its unique sighting over the coding land.
The whole idea is about solving simple problems with smart solutions–the very essence of programming. So, keep exploring, keep coding, and most importantly, never stop reversing!