Choosing Random Elements in C#: Tutorial
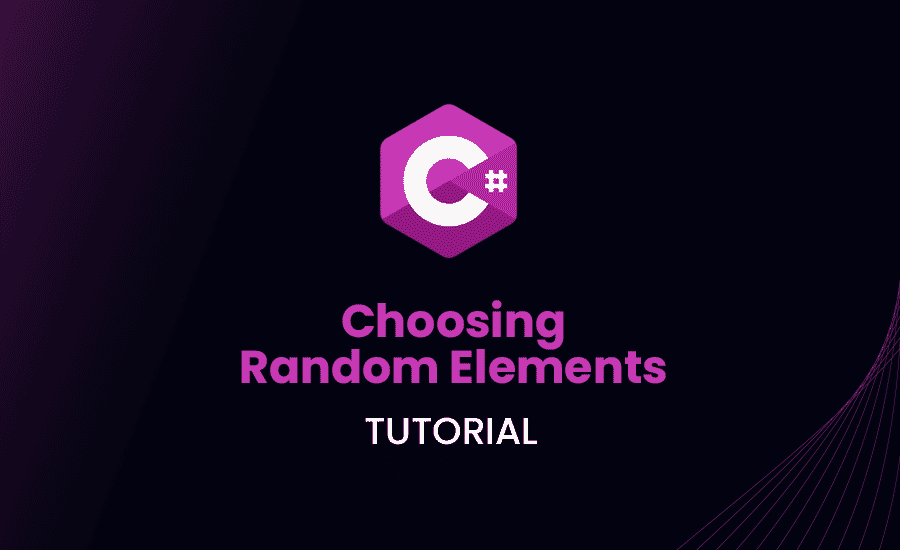
C# is hailed as one of the most versatile and powerful programming languages out there. One of the features that make it so flexible is the ability to generate and manipulate random elements. But how exactly do you go about this in C#?
That’s what we’re going to explore in this guide. By the end, you will be proficient in choosing random elements in C#, opening up new ways for you to control and manipulate data.
Getting Started with Randomizing Elements in C#
Before we go choosing random elements like stylish hats in a shop, let’s start at the beginning. Understanding randomness in C#.
Understanding Essentials of Randomness in C#
When we talk about random elements in C#, we can refer to random numbers, array elements, or elements from a list or a map. C# uses the System.Random
class to generate random numbers (int
, double
, float
) between a specified range.
The random numbers generated by the Random
class are pseudo-random. This means they appear to be random, but they follow a deterministic sequence based on a seed value passed to the Random
object upon initialization. If the seed is not provided, Random
uses system time as the default seed value.
Here is a simple example of generating random numbers in C#:
Loading code snippet...
In the above code example, we first create a Random
object rnd
. Using the Next(int minValue, int maxValue)
method, we generate a random integer between 1 and 10. The NextDouble()
method generates a random floating-point number between 0.00 and 1.00.
How To Get Random Element From List in C#
Now that we’ve got the basics of randomness in C# down, let’s play with lists. Specifically, how to get a random element from a list in C#.
Practical Examples: Retrieving Random Elements From Lists
Say you have a list of fruits and you want to select a random one for a nutritious smoothie recipe. In C#, you would do the following:
Loading code snippet...
In this code, we first create a list of fruits. Then, we use the Random
object to generate a random index that’s within the range of the list’s length. Finally, we select and print the fruit at the generated random index.
Tips and Tricks for Optimized Random Element Retrieval
When working with the Random
class, it’s important not to create a new Random
object for each random number you want to generate. Instead, create one Random
object and reuse it. This is because Random
uses the system clock as the default seed value, and the system clock’s granularity is approximately 15 milliseconds. If you create multiple Random
objects within a short period, they could end up using the same seed.
How to Get a Random Array Element in C#
Arrays, simple yet powerful, are integral parts of programming. What if you need a random array element in your code? Fear not, C# has got you covered.
rray Randomization
The process of picking a random element from an array is similar to that of a list. Here’s an example:
Loading code snippet...
In this sample code, we first declare an array of animals. Then we generate a random index in the same way as before, by creating a Random
object and fetching a random number within the range of the array’s length. We then print out the array element at the random index.
Accessing Random Elements in Arrays
Here’s another code example. This time, we’ll use a multidimensional array, demonstrating that the process works seamlessly irrespective of the array’s complexity.
Loading code snippet...
In this piece of code, we take advantage of the GetLength(int dimension)
method to get the lengths of the dimensions of the multidimensional array.
Printing Random Elements from Lists in C#:
Printing random elements from lists has a wealth of practical applications, from game development to data manipulation. It’s easier than you think!
Benefits of Printing Random List Elements
Randomization is widely used in programming. For example, it can shuffle a music playlist, randomize quiz questions, or generate random colors for a graphic. By understanding how to print random list elements in C#, you widen your coding capabilities.
The way to print random elements from a list is no different from the steps to get a random element that we previously discussed. I’m sure you feel confident already.
Practical Use-Cases of Random Print Statements
For instance, in a dice roll game, randomization can be used to choose a random number:
Loading code snippet...
In this code, we’ve simulated a dice roll by choosing a random number from a list of six numbers.
Access a Random Element from a Map in C#
Your journey to mastering randomness is nearly complete, but there’s another stop: maps. Let’s discover how to cherry-pick from these!
Understanding Maps and Random Accesses
Maps, known in C# as dictionaries, are critical data structures used for storing key-value pairs. Our adventure in randomness would be incomplete without knowing how to pick a random element from a map. Take a look at this code:
Loading code snippet...
In this snippet, we have a dictionary of countries and their capitals. We convert the dictionary keys to a list, and then we use our familiar method to fetch a random key from the keys list. We then print out the country-capital pair.
Managing Errors and Applying Best Practices in C#
One of the more tricky aspects of using randomness in programming, particularly in C#, is ensuring that your use of random elements doesn’t lead to errors, vulnerabilities, or even simply sub-optimal code. Random elements, though powerful, can be tricky if not used correctly. This section is dedicated to helping you prevent common pitfalls and apply best practices for efficiently managing random elements in C#.
Navigating Common Errors with Random Elements
Mistakes can happen, and they often do when you’re dealing with randomness. Let’s delve into some of the issues you might run into and how to avoid them.
A frequently observed mistake when working with the Random
class is creating a new Random
object each time when generating a series of random numbers. This practice leads to less random numbers, defeating the purpose of randomness. The core of the problem lies in the fact that the Random
class uses the current time as the default seeding value. If multiple Random
instances are created too close in time (e.g., in a loop), they can end up with the same seed and hence produce the same ‘random’ sequence.
Consider the following incorrect code:
Loading code snippet...
This code attempts to create ten random integers, but because the Random
object is initiated in quick succession, we end up with a set of identical numbers instead of a random sequence.
Instead, you should create one Random
instance and reuse it for subsequent random number generations:
Loading code snippet...
Another common error when working with random elements in C# is not checking if a collection is not empty before attempting to access a random element from it.
Loading code snippet...
In this code snippet, an attempt is made to access a random element from an empty list. This will throw an ArgumentOutOfRangeException
. To avoid such errors, always check if a collection is empty before trying to fetch random elements.
Best Practices for Working with Random Elements in C#
When working with random elements in C#, there are several best practices that can help you write secure and efficient code.
- Use a Cryptographically Secure Random Number Generator Where Needed: If you’re dealing with sensitive data or anything that needs a high degree of randomness (like the generation of security keys or random passwords), you should use a cryptographically strong random number generator. In C#, you can use the
RNGCryptoServiceProvider
class, which is part of theSystem.Security.Cryptography
namespace.
Here’s how you can use it to generate a random integer between a given range:
Loading code snippet...
In the above code, RNGCryptoServiceProvider
is used to fill an array of bytes with a sequence of random values. Then, BitConverter.ToInt32
is used to convert these bytes to an integer. The result is a cryptographically strong random number.
- Protect Against Empty Collections: Always check if a collection is not empty before trying to select a random element:
Loading code snippet...
In this piece of code, we first make sure the list is not empty by using the Any()
method from LINQ. If the list contains at least one element, we proceed to select and print a random element.
Conclusion
So, is randomness really that random? In reality, we lean on mathematical formulas, system clocks, and class structures to simulate randomness, but that’s more than enough for the requirements of most applications.
Remember: the world of programming is as unpredictable as the code we just wrote. So don’t wait, start experimenting right now, because who knows what you’ll discover by injecting a dose of randomness into your code? It’s time to take your C# projects to the next level. Happy coding!