C# LINQ to XML: Parsing and Manipulating XML Documents
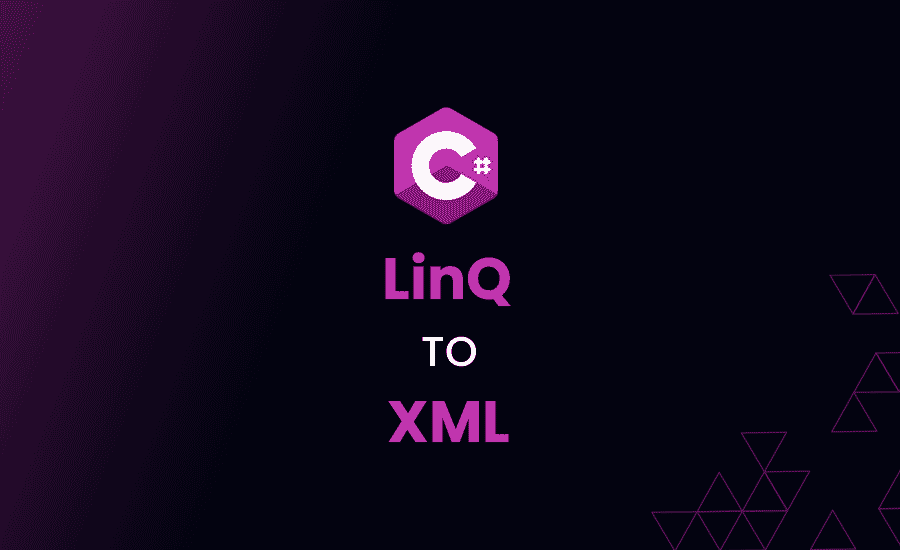
In this article, we will dive deep into the world of XML processing using C# LINQ to XML. We will explore how to parse, create, and manipulate XML documents effectively with LINQ, making your XML handling tasks a breeze. The topics covered in this article include parsing XML documents, querying XML data, modifying XML elements and attributes, and creating XML documents from scratch. So, let’s get started!
Parsing XML Documents with LINQ
In this section, we will learn how to parse XML documents using LINQ to XML, and explore various techniques to read and extract information from XML data. The following topics will be covered in this section:
- Loading XML documents
- Accessing elements and attributes
- Navigating XML trees
Loading XML Documents
There are several ways to load XML data into a LINQ to XML object. You can load XML from a file, a string, or an XmlReader
object. Here are some examples of how to load XML data using different sources:
Loading code snippet...
Accessing Elements and Attributes
Once you have loaded the XML data, you can begin to access its elements and attributes using LINQ queries. Here’s an example of how to access elements and attributes in an XML document:
Loading code snippet...
Navigating XML Trees
LINQ to XML provides various methods to navigate and traverse XML trees, such as Elements
, Descendants
, Ancestors
, and Parent
. Here are some examples of navigating XML trees using these methods:
Loading code snippet...
Querying XML Data with LINQ
In this section, we will explore how to query XML data using LINQ queries, making it easy to filter, sort, and project XML data. We will cover the following topics:
- Filtering elements and attributes
- Sorting elements and attributes
- Projecting XML data into objects
Filtering Elements and Attributes
You can use LINQ queries to filter XML elements and attributes based on specific conditions. Here’s an example of filtering XML data using a LINQ query:
Loading code snippet...
Sorting Elements and Attributes
LINQ queries can also be used to sort XML elements and attributes based on specific criteria. Here’s an example of sorting XML data using a LINQ query:
Loading code snippet...
Projecting XML Data into Objects
LINQ queries can project XML data into objects, making it easier to work with the data in your C# code. Here’s an example of projecting XML data into objects using a LINQ query:
Loading code snippet...
Modifying XML Elements and Attributes
In this section, we will learn how to modify XML elements and attributes using LINQ to XML. We will cover the following topics:
- Adding elements and attributes
- Updating elements and attributes
- Removing elements and attributes
Adding Elements and Attributes
You can add new elements and attributes to an XML document using the Add
method. Here’s an example of adding elements and attributes to an XML document:
Loading code snippet...
Updating Elements and Attributes
You can update the values of elements and attributes in an XML document using simple assignment statements. Here’s an example of updating elements and attributes in an XML document:
Loading code snippet...
Removing Elements and Attributes
You can remove elements and attributes from an XML document using the Remove
method. Here’s an example of removing elements and attributes from an XML document:
Loading code snippet...
Creating XML Documents from Scratch
In this section, we will learn how to create XML documents from scratch using LINQ to XML. You can create an XML document by creating XDocument
, XElement
, and XAttribute
objects and adding them together. Here’s an example of creating an XML document from scratch:
Loading code snippet...
In summary, we have explored various techniques to parse, create, and manipulate XML documents using C# LINQ to XML. By understanding and applying these concepts, you can easily handle complex XML tasks in your C# applications. Don’t forget to experiment with these techniques to gain a deeper understanding of LINQ to XML capabilities and improve your XML processing skills. Happy coding!
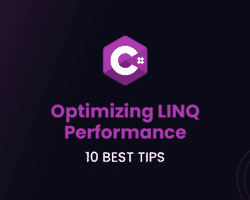
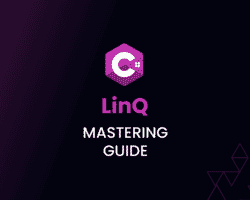
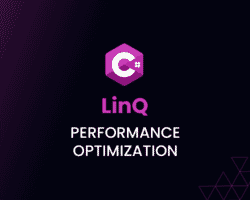
LINQ Performance Optimization
April 9, 2023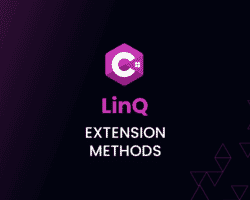
LINQ Extension Methods
April 8, 2023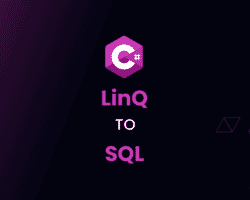
LINQ to SQL
April 7, 2023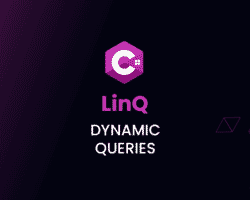
Dynamic LINQ Queries
April 6, 2023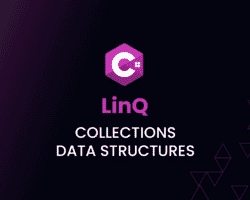
LINQ Collections and Data Structures
April 5, 2023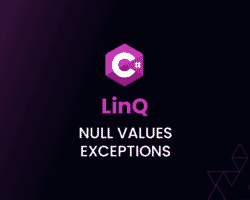
Null Values and Exceptions LINQ Queries
April 3, 2023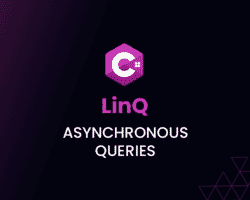