C# LINQ: Collections and Data Structures
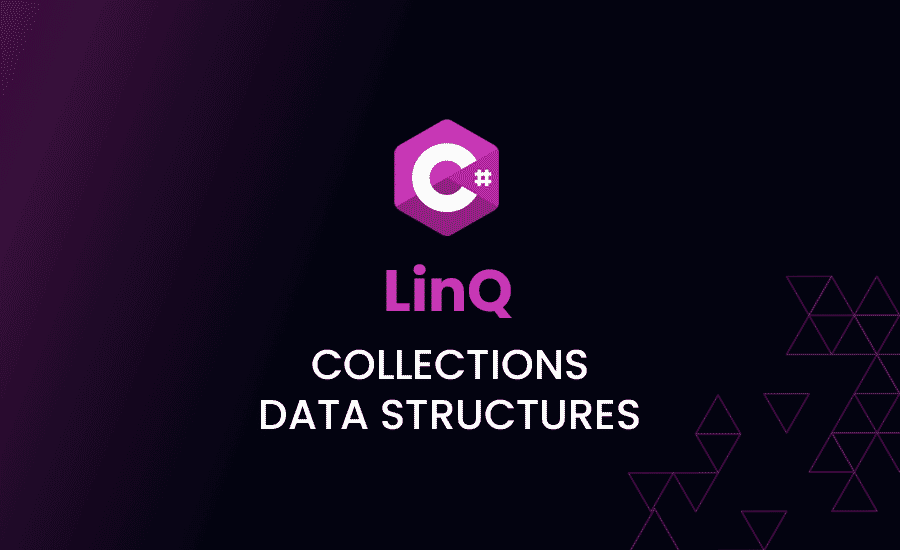
In this article, we will dive deep into LINQ and its capabilities when working with collections and data structures in C#. We will explore various LINQ methods and techniques to manipulate arrays, lists, and dictionaries effectively. So, let’s get started and become masters of C# LINQ in the world of collections and data structures!
Introduction to LINQ with Collections
Collections are an essential part of any programming language, and C# is no exception. LINQ (Language Integrated Query) is a powerful feature in C# that allows you to query and manipulate collections in a concise and expressive way.
In this section, we will cover the basics of LINQ with collections and see how it can help us work with arrays, lists, and dictionaries more efficiently.
Using LINQ with Arrays
Arrays are the most basic data structure in C#, and LINQ can be used to perform various operations on them, such as filtering, projecting, and aggregating values. Here is an example of using LINQ with an array of integers:
Loading code snippet...
Working with Lists
Lists in C# are dynamic arrays that can grow or shrink in size. LINQ can be used to query and manipulate lists, just like arrays. Here is an example of using LINQ with a list of strings:
Loading code snippet...
Manipulating Dictionaries
Dictionaries in C# are collections of key-value pairs, useful for storing and retrieving data based on keys. LINQ can also be used to query and manipulate dictionaries. Here’s an example of using LINQ with a dictionary:
Loading code snippet...
Advanced LINQ Techniques for Collections
Now that we have covered the basics of using LINQ with collections let’s dive into some more advanced techniques that can help us manipulate and query data more effectively.
Projection and Transformation
Projection is a powerful feature of LINQ that allows you to transform the elements of a collection into a new form. The Select
method is used for projection, and it takes a lambda expression as an argument to define the transformation. Here’s an example:
Loading code snippet...
Aggregation and Reduction
LINQ provides several aggregation methods that allow you to reduce a collection to a single value. Some common aggregation methods are Sum
, Average
, Min
, Max
, and Count
. Here’s an example of using aggregation methods with a list of integers:
Loading code snippet...
Grouping Data
The GroupBy
method in LINQ allows you to group elements in a collection based on a specified key. Here’s an example of grouping a list of strings by their first character:
Loading code snippet...
Sorting Data
LINQ provides two sorting methods: OrderBy
and OrderByDescending
. These methods can be used to sort elements in a collection based on a specified key. You can also use the ThenBy
and ThenByDescending
methods to sort elements based on multiple keys. Here’s an example:
Loading code snippet...
Joining Collections
LINQ provides several methods for joining two collections based on matching keys, such as Join
, GroupJoin
, Union
, Intersect
, and Except
. Here’s an example of using the Join
method to join two lists based on a common key:
Loading code snippet...
Conclusion
In this article, we have covered various aspects of using LINQ with collections and data structures in C#. We have gone through the basics of using LINQ with arrays, lists, and dictionaries and explored advanced techniques such as projection, aggregation, grouping, sorting, and joining.
With this knowledge, you can now harness the power of LINQ to effectively manipulate and query your collections in C#. Keep practicing and experimenting with LINQ to become a true master of C# collections!
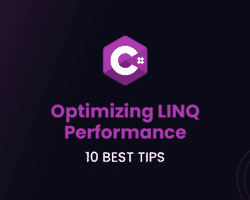
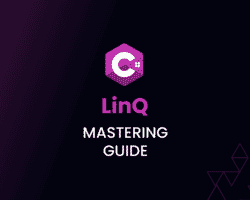
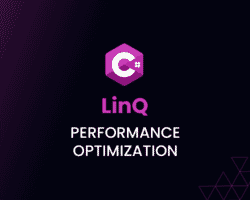
LINQ Performance Optimization
April 9, 2023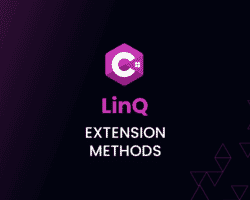
LINQ Extension Methods
April 8, 2023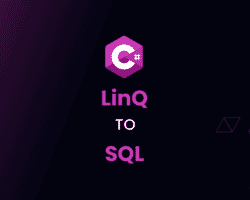
LINQ to SQL
April 7, 2023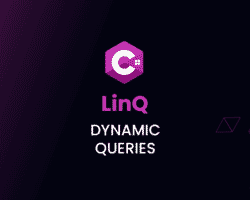
Dynamic LINQ Queries
April 6, 2023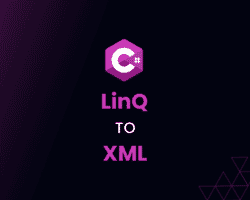
LINQ to XML
April 4, 2023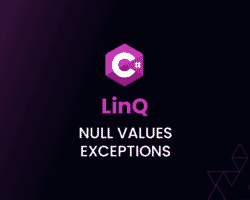
Null Values and Exceptions LINQ Queries
April 3, 2023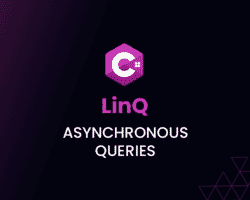