C# LINQ Performance Optimization: Tips and Tricks
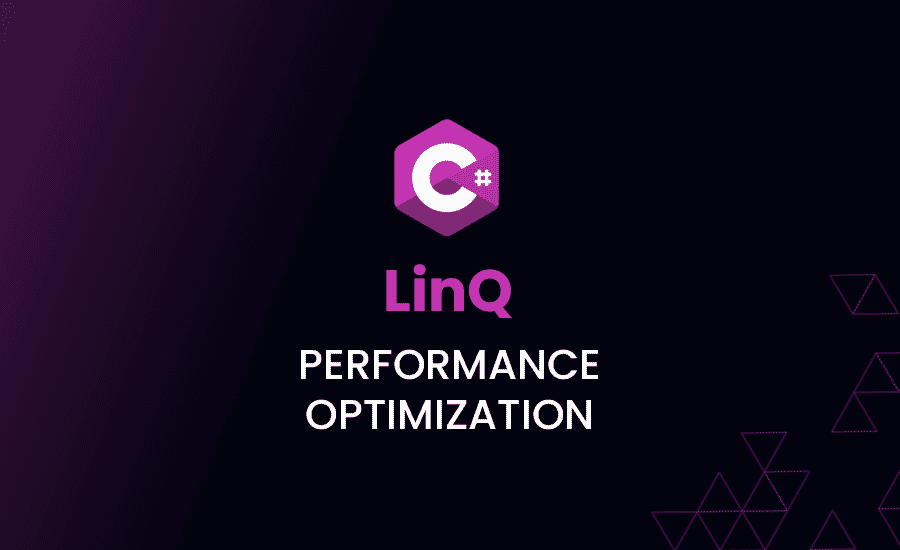
LINQ (Language Integrated Query) is an incredibly powerful feature in C# that simplifies complex data manipulations and queries. However, if not used correctly, it can lead to performance issues. In this article, we will explore various tips and tricks to optimize your LINQ queries and improve the performance of your C# applications. Let’s dive in!
Use the Right Data Structure
The choice of data structure can greatly impact the performance of LINQ queries. When working with collections, consider the following:
- Use
List<T>
for small to medium-sized collections where frequent additions and removals are needed. - Use
HashSet<T>
when you need to perform fast lookups and ensure unique elements. - Use
Dictionary<TKey, TValue>
when you need to store key-value pairs and perform fast lookups by key.
Opt for Compile-Time Query Execution
LINQ queries can be executed either at runtime (using IEnumerable<T>
) or compile-time (using IQueryable<T>
). Compile-time query execution can result in better performance, as it allows the query to be analyzed and optimized before execution. Consider the following example:
Loading code snippet...
In the second example, the query is executed at compile-time, potentially leading to better performance.
Use the
The Any
and All
methods are helpful when checking for specific conditions in a collection. However, using them incorrectly can lead to performance issues. Consider the following:
- Use
Any()
instead ofCount()
when checking if a collection has at least one element. - Use
All()
to check if all elements in a collection meet a specific condition, instead of usingWhere()
andCount()
.
Loading code snippet...
Prefer Lazy Evaluation
LINQ supports deferred execution, which means that the query is not executed until the results are actually needed. This can lead to performance improvements, as the query is only executed when necessary. Consider the following example:
Loading code snippet...
In the second example, the query is executed only when the results are iterated or accessed, potentially improving performance.
Use
The Select
and Where
methods are essential for LINQ queries, but if not used correctly, they can lead to performance issues. Consider the following:
- Use
Select
to project only the necessary fields, instead of returning the entire object. - Chain
Where
clauses to filter out unnecessary data early in the query.
Loading code snippet...
Leverage Parallel LINQ (PLINQ)
Parallel LINQ (PLINQ) enables you to execute LINQ queries in parallel, potentially improving performance for large data sets and CPU-bound operations. To use PLINQ, simply call the AsParallel()
method on your collection:
Loading code snippet...
Keep in mind that parallel execution can introduce additional overhead and may not always result in performance improvements. Use PLINQ judiciously and test your application to ensure optimal performance.
Use Index-Based
When filtering large collections, using the index-based overload of the Where
method can often result in performance improvements. The index-based overload allows you to filter items based on their index in the collection, which can be useful in specific scenarios:
Loading code snippet...
In the second example, we filter the collection based on both the item’s IsValid
property and its index in the collection, resulting in a more efficient query.
Avoid Multiple Enumerations
Multiple enumerations of a LINQ query can lead to performance issues, as the query is executed multiple times. To avoid this, consider materializing the results into a concrete collection like List<T>
or Array<T>
:
Loading code snippet...
In the second example, we materialize the query results into a List<T>
to prevent multiple enumerations and improve performance.
Optimize LINQ to SQL Queries
When using LINQ to SQL, it’s essential to optimize your queries to minimize the amount of data transferred between your application and the database. Here are some tips:
- Use
Select
to project only the necessary fields from the database. - Filter data using
Where
before applying other operations, such asGroupBy
orOrderBy
. - Use
Take
andSkip
for pagination instead of loading all the data and then filtering in the application. - Use
CompiledQuery.Compile
to cache and reuse frequently executed queries.
Loading code snippet...
In the second example, we filter and project the data directly in the database, reducing the amount of data transferred and improving performance.
Use Predicate Builders for Dynamic Queries
When building dynamic LINQ queries, consider using predicate builders to combine multiple filter conditions efficiently:
Loading code snippet...
Using a predicate builder can lead to more efficient queries and improved performance when dealing with complex or dynamic filter conditions.
By following these tips and tricks, you can optimize your LINQ queries and improve the performance of your C# applications. Remember that performance optimization is an ongoing process, and it’s essential to continually analyze and fine-tune your code for the best results. Happy coding!
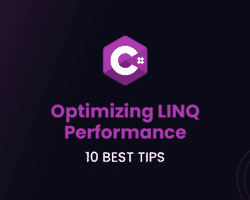
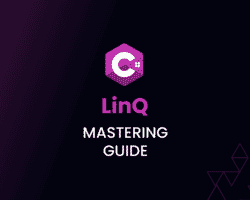
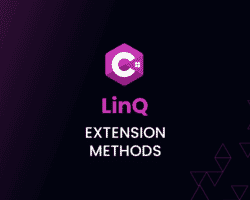
LINQ Extension Methods
April 8, 2023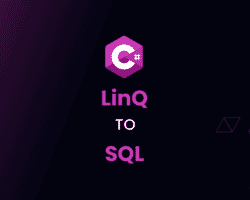
LINQ to SQL
April 7, 2023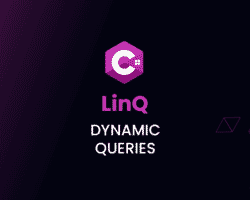
Dynamic LINQ Queries
April 6, 2023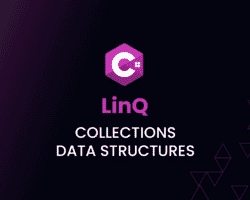
LINQ Collections and Data Structures
April 5, 2023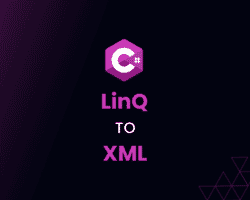
LINQ to XML
April 4, 2023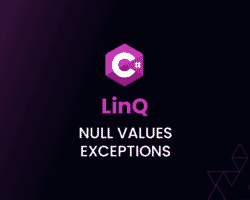
Null Values and Exceptions LINQ Queries
April 3, 2023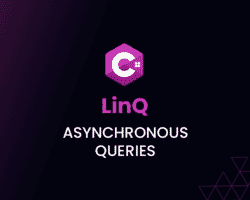