How to Join Strings in C#: Tutorial
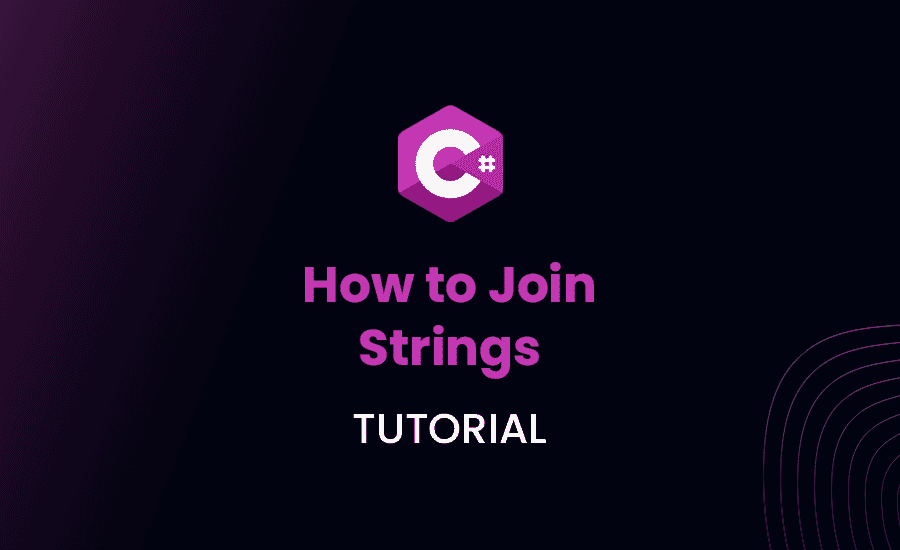
Well, it’s time to tie-up those loosened C# string ends that have been troubling you. The universe of C#, as you know it, is about to expand! Ever wondered how to achieve an elegant ballet of string joining that your fellow developers will envy? Keep reading and be ready to delve into the intricacies of lassoing strings together in C#.
Understanding the Basics of Joining Strings in C#
Before we hop onto the stage and begin our performance, let’s put our dancing slippers (or rather, our developer shoes) on and take a step back to peer into the mirror. There we see the reflection of the fundamental act – string concatenation!
A Brief Overview of String Concatenation
Simply put, string concatenation is the process of combining two or more strings end-to-end to form one long string, kind of like creating a wordy train. It is one of the common tasks that you would do frequently, especially when working with manipulating data strings in a C# based development.
Loading code snippet...
Above, str1 and str2 are two separate strings that are being combined with the help of the plus operator, resulting in “Hello World”. A pretty straightforward procedure, eh? But hold on, there’s so much more you can do!
Core Ways to Join Strings in C#: An In-Depth Look
There are multiple ways to join strings in C#. Let’s break down the moves one by one, and get ready to dance!
Using the String.Join Method in C#
The first dance move in our routine, ladies and gentlemen, is the String.Join
method! The String.Join()
function is a simple yet powerful tool in your C# toolbox, making the process of combining words effortless.
C# String.Join Example: A Practical Illustration
Loading code snippet...
Here, we have the words “Hello” and “World” joined together with a comma. As you see, String.Join()
takes the separator and elements as parameters, allowing us to sew together strings with different types of string separators. But wait! Can we join a list of strings as well? Let’s find out!
C# Join List of Strings
Believe it or not, you can show off your dance moves by joining a list of strings too, and it’s as smooth as the waltz!
Case Study: Applying ‘Join’ on List of Strings
Loading code snippet...
In this scenario, we’ve tied together the strings in the list with a comma using String.Join()
. Perfect! What else can we join? What about an array of strings?
How to Join Array of Strings in C#
You guessed it! You can call forth your inner dance floor champion and join an array of strings as well. With your String.Join()
method in hand, you can gracefully glide through any array of strings.
An Actual C# String Array Join Example
Loading code snippet...
Once again, we used the handy String.Join()
method to concatenate the string array elements with a comma. But is String.Join()
the only superstar on the floor? Back to the dance-off!
String.Join vs StringBuilder in C#
Let’s turn our spotlight on another contender that’s been eagerly waiting in the wings: the StringBuilder
class. Get ready for an exciting face-off to see who stands out in this dance-off of efficient string joining.
What is StringBuilder?
StringBuilder
is a mutable string type that can be used when you need to modify the string data multiple times with high performance.
How does StringBuilder Operate?
It modifies the original string rather than creating new ones, thus improving the performance. Now, let’s see the StringBuilder
in action.
Loading code snippet...
In this example, we used the Append
method of StringBuilder
to join strings. So, who wins in the String.Join
vs StringBuilder
dance-off? Both are excellent dancers, but it depends on the complexity and the performance demands of your application.
Advanced Tips and Tricks for String Joining in C#
In C#, string joining is more than just a mere binding of strings. It’s an art, it’s a science, and it’s definitely something that deserves a little extra attention. In this stage of our tutorial, we’ll dig deeper into the subject and explore a way to make the string joining process more refined and effective. We’ll look into these skills:
- Ignoring empty values while joining strings
- Dealing with diverse data types
- Handling exceptions in string joining
How to Use String.Join to Ignore Empty Values in C#
In programming, we often encounter situations where dealing with null or empty elements becomes a pain, especially when joining strings. No worries, with String.Join()
, those worries become things of the past!
Practical Implementation: String.Join Ignore Empty Values in C#
Consider if you come across an array of strings as follows:
Loading code snippet...
Here, our array includes some empty and null values. To get a combined string that doesn’t incorporate these values, we can call upon String.Join()
but with a little twist of Where()
function in the mix. Using Where()
helps us to filter out these unwanted null or empty strings.
Loading code snippet...
In the above line of code, an anonymous function is being passed to the Where
method to filter out null and empty strings from the array before joining them using String.Join()
function. This way, only the strings “Hello” and “World” get joined, ignoring the empty and null elements.
Joining Strings of Different Data Types
In some cases, you might deal with strings derived from different data types. Holding hands with String.Join()
, we can nimbly dance through this challenge as well.
Practical Example: Joining Strings from Different Data Types
Loading code snippet...
With String.Join()
, we can easily join strings derived from any data type without any hiccups.
Exception Handling in String Joining
Null and empty strings are not the only hurdles in your string joining pathway. Sometimes, a NullReferenceException
or some similar issues may throw a spanner in your works. But hey, who’s afraid of exceptions when you know how to handle them?
Exception Handling: Practical Approach
Loading code snippet...
In the above example, we’ve prepared our code for a NullReferenceException
by using a try-catch block. This way, our script can handle situations when null values lurk in our array of strings.
Common Pitfalls and How to Avoid Them
Before jumping onto the next challenging development task, it’s worthwhile to highlight some of the common pitfalls that can make your journey with string operations in C# somewhat tricky, and more importantly, how to dodge them like a professional.
Limitations of String Join Operations
As we know, every operation in programming comes with its own limitations, and string join operations are no exception. Let’s have a swift glance on a couple of them:
NullReferencesException:
Dealing with null
values is an inherent part with String.Join()
. A null
reference exception can happen if a null object is used where an object is expected. If you’re not careful to guard against these, you might be in for a sudden surprise.
Loading code snippet...
In this example, the array consists of a null value and String.Join()
fails miserably with a NullReferenceException
. But worry not! To dodge this issue, it’s always good to ensure your array or list doesn’t contain any null
values. We can filter out the nulls using the Where()
method:
Loading code snippet...
By using Where()
, we’re making sure that no null values interfere into our string joining dance.
Performance:
Streaming thousands of strings together can easily result in performance degradation, particularly when you’re handling a big data set. Remember the basic rule of thumb – the String.Join()
method offers excellent service for joining a small number of strings, but can be a performance hog when asked to belt out with a large number.
Loading code snippet...
In this example, joining a million strings with String.Join()
results in a noticeable delay – not exactly an ideal scenario.
To counteract this issue, utilize our trusty friend StringBuilder
for the heavy-duty performance:
Loading code snippet...
Here, StringBuilder
shines by handling the same operation much more efficiently, thanks to its mutable capabilities that allow it to modify the original string instead of creating new ones.
Best Practices to Follow When Joining Strings in C#
String operations are an essential part of your day-to-day coding routines. It’s therefore important to incorporate best practices for efficient execution.
- Do use
String.Join()
when dealing with a smaller set of strings. It’s simple and efficient for basic chores.
Loading code snippet...
- For more demanding tasks involving larger data sets, consider adopting
StringBuilder
to safeguard performance.
Loading code snippet...
In this example, StringBuilder
provides a much-needed boost when joining a large dataset.
We started with the basics of string joining and then moved on to understanding the various methods available in C#, namely String.Join()
and StringBuilder
. We explored practical applications with examples and also discussed handy tips to escape common pitfalls.
So now, are you ready to sway to the rhythm of string joining in C#? Don’t wait and let your C# strings perform an enthralling ballet as they join together!