If Statement in Unity: From A to Z
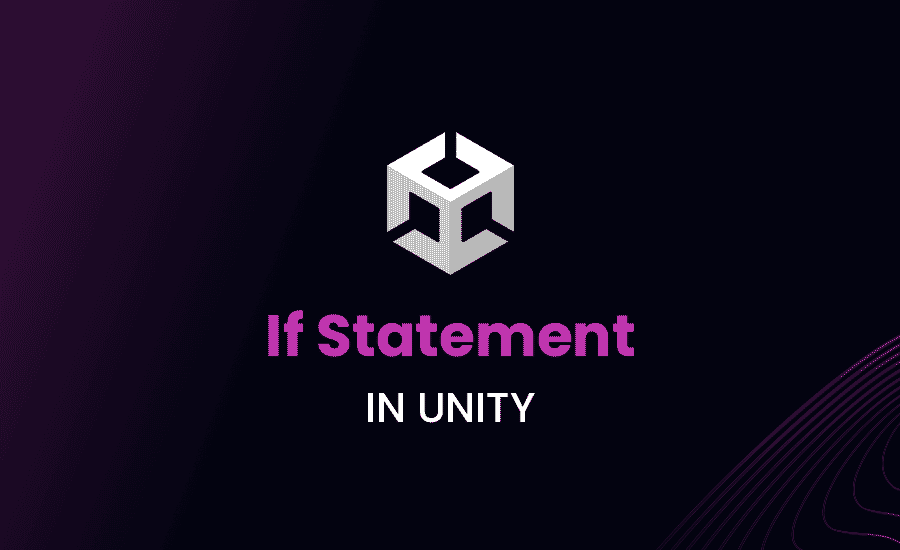
Feeling lost in the labyrinth of Unity’s conditional constructs? Well, you’re not alone. Let’s embark on an enlightening journey to comprehend “if statements” in Unity — the cornerstone of our decision-making process in programming.
Heads up! This article assumes you have basic knowledge of Unity and C# programming. Let’s get started!
If Statement in Unity
Before we plunge into the deep end, let’s get our feet wet by understanding what an “if statement” is in Unity.
What is an If Statement in Unity
In Unity, an “if statement” works exactly like a traffic light. It controls the program flow based on a condition. Just like a traffic light alters the movement of vehicles based on color signals, an “if statement” in Unity checks for a condition and, based on the evaluation, determines which code block should be executed.
Here’s a basic example:
Loading code snippet...
In this code, we’ve used an if statement to check if the score of a player is 100. If yes, we print a message to the console.
Syntax and Examples
The if statement
follows a simple syntax but offers infinite utility. Here’s a quick breakdown of its structure:
Loading code snippet...
In the condition, we can use different operators for comparison such as ==
, >
, <
, >=
, <=
, !=
. Then, we wrap in the code block we want to execute for a true condition in curly braces {}
.
The If-Else Unity Statement
Now that the traffic light (if statement) is working, let’s add a roundabout (if-else statement) for managing complex traffic!
The Purpose of If-Else Statement in Unity
The “if-else” statement in Unity allows for an alternative path in case the condition in the “if” statement turns out to be false. It’s like having a backup plan in action!
Here’s a simple example:
Loading code snippet...
In this script, we print a different message if the player’s score isn’t high enough.
Practical Scenarios to Use If-Else Statement
With “if-else” statements in Unity, you can create endless layers of conditional logic. For example, adjusting the level of difficulty based on the player’s score, managing AI behavior under different game scenarios, creating event trigger instances – you name it!
Unity If Multiple Conditions
Ever felt muddled by multiple conditions? You’re not alone. Let’s dive into how to handle Unity if multiple conditions.
How to Use Unity If Statement Multiple Conditions
More conditions mean more complexity, right? But don’t sweat. Unity is your pal. It allows you to deal with multiple conditions using “and”(&&
) and “or”(||
) operators.
Loading code snippet...
In this example, the player receives a thumbs-up only if their score is 100 or higher, and also have lives left.
Case Study: Implementing Multiple Conditions in Unity
Consider a player progression system in a role-playing game. Here different conditions can be set for player advancement. For instance, if the player has reached a certain score and found a unique artifact, then they level up. This complex logic can be handled effectively using Unity’s “if statement” with multiple conditions.
Unity Break If Statement
Now, let’s add a detour sign on our code road trip. In certain cases, we need to halt our decision-making process midway, that’s where Unity break if statement comes into play.
When and Why to Use Unity Break If Statement
Sometimes, there’s a need to escape; in loops, more often. The break command helps us leave the loop’s embrace when certain conditions are met. This way, we don’t have to complete all iterations of a loop if there’s no need.
Here’s a bite-sized code snack that shows a “break”:
Loading code snippet...
Amid this loop, if “i” becomes equal to 5, the loop is immediately terminated, cutting short the iterations. Certainly, using “break” effectively makes us control freaks – in a good way!
Real-world Usage of Unity Break If Statement
Break statements come handy in game mechanics where we don’t need to check all conditions or loop through every single option. For example, in an inventory system of a role-playing game (RPG), when you locate the item you were searching for, why bother checking through the rest of the inventory?
Unity Shader Graph If Statement
So, we’re breaking, looping and making decisions. It’s time to light things up with shader graph if statements.
Understanding What Unity Shader Graph If Statement is
The Shader Graph in Unity allows us to visually author shaders by building them with nodes. This includes an If node. The If Node accepts three inputs: A, B and In. If A is greater than B, the node returns the top input; else it returns the bottom input.
Loading code snippet...
Effective Use of Unity Shader Graph If Statement
This visual if-else ladder allows you to layer materials, change coating, or add textures based on different conditions. Such conditional practices have been pivotal in games where visual aesthetics can change based on player choices or game progression.
Learning about Bolt Scripts: Unity Bolt If Statement
Shifting gears, it’s time to bolt your knowledge with (drumroll!) Unity Bolt If Statement. This is a real game-changer (pun intended).
Introduction to Bolt and Unity Bolt If Statement
Dreaming of a code-free, visual scripting world? Welcome to Unity Bolt! Bolt brings non-coders closer to game development. Even its “if” statement is as easy as pie.
In Bolt, you connect nodes to build a flow. The “if” statement’s nodes are – Flow, Condition, True, and False. Connect them correctly and voila! You’ve an if statement flowing flawlessly!
Advantages of Using Unity Bolt If Statement
Bolt is perfect for quick prototyping or when you want to focus more on creativity and less on coding syntax. With Bolt’s visual scripting, conversations between teams without a programming background have never been easier!
Looking at Two Variables: 2 Variables in If Statement Unity
You’ve been mastering Unity “if” statements like a pro. Let’s turn the heat up and throw in another variable.
How to Use 2 Variables in If Statement Unity
Having two variables in an “if” statement is a common scenario in game programming. In the example below, we’re checking if a player’s health and shield are both depleted:
Loading code snippet...
Making Decisions with 2 Variables in an If Statement in Unity
Managing multiple variables in a condition opens doors for complex game mechanics. Imagine managing a player’s inventory where both weight and item count need to be checked, or controlling an NPC’s behavior based on its health and aggression levels. The creative possibilities are endless!
Real-Life Scenarios and Best Practices
After diving headfirst into the sea of if-statements, it’s time to sunbathe on the beach and share some practical tips and tricks. Let’s whip out some more real-world scenarios and best practices to ensure you utilize if-statements to their full potential.
Showcase of Real Game Development Scenarios
Racing Game: Activating Speed Boosts
Consider a racing game where the speed of your automobile increases if the player scores a specific number of points. To add a fun-factor – let’s say, once the player’s score crosses a hundred, their car receives a speed boost for a limited time. This could be implemented using the following script:
Loading code snippet...
In this case, when the player’s score reaches or exceeds 100 points, the condition inside the if-statement becomes true. As a result, speedBoost is set to true, and now, the player’s car will zoom through the race track!
Survival Game: Triggering Starvation Mechanics
In a survival game scenario, a player’s health and food metrics are crucial. Let’s say if the player’s health falls below 20, and they don’t have any food left, a starvation mechanic is triggered leading to a slow decrease in the player’s health over time.
Loading code snippet...
This example captures the dire scenario where if the player’s health falls below 20 and they lack food resources. The starvation mechanics kick in, enhancing the survival aspect of the game.
Multiplayer Game: Checking Player Readiness
In multiplayer games, it’s crucial to ensure that all players are ready before the game starts. Imagine you have an array of players, and you want to confirm every player is set before the game begins:
Loading code snippet...
In this scenario, we loop through each player in the array. If even a single player is not ready (where “player” would equal to “false”), the message is logged, and the loop breaks.
Tips and Best Practices when using If Statement in Unity
Making Conditions Meaningful
It’s like composing a tune – the notes (conditions) need to make sense for the melody (gameplay) to be enchanting. Remember, inappropriately used if-statements could lead to erroneous or unintended code execution.
Loading code snippet...
Avoiding Complex Nested Conditions
Avoid writing if-statements within if-statements within if-statements – they are difficult to read, understand, and debug.
Loading code snippet...
Use Comments Effectively
Throw in comments like floating buoys over a sea of code. They help you, your team (and your future self) navigate through the code and understand the purpose of the if-statements effectively.
Loading code snippet...
So, armed with this knowledge – are you ready to unleash the power of “if statements” in Unity? It’s this simple tool that opens doors to complex game mechanics. So, let’s begin command the flow of your game code and create amazing experiences. And what happens if you don’t do this? You’re ignoring an essential part of your game development toolbox. So, why wait? It’s time to jump into Unity and start if-ing around! Start now – and make an impact with your gaming world.