If Else in C#: Guide with Examples
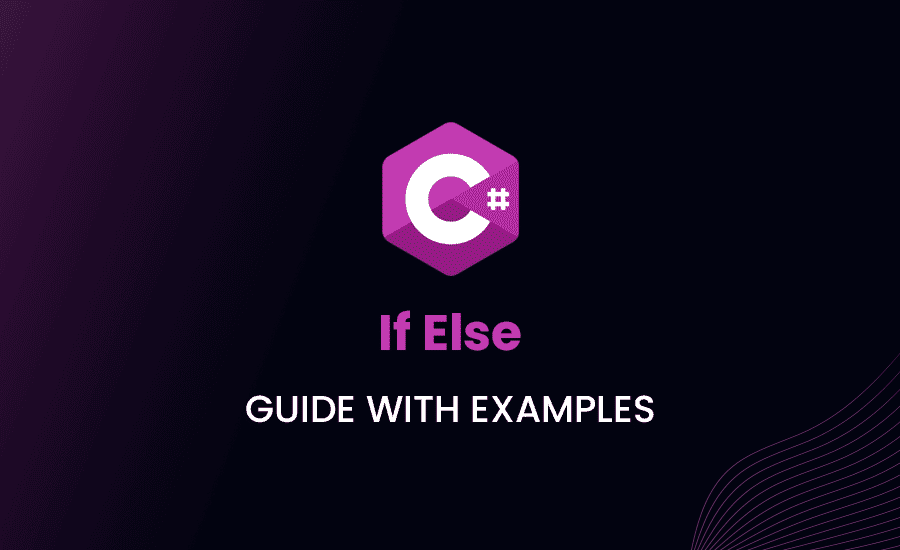
Surely, every developer, regardless of their experience level, encounters conditional statements in their coding journey. For C# programmers, the If Else statement is an essential tool in the kit, let’s dig deeper into it.
Understanding The Basics: C# If Else Statement
Ah, the If Else statement, the bread and butter of every C# developer’s code. It’s one of the first concepts in programming we learn and one that we use daily.
What is If Else In C#
Describing the if else statement is like describing how a traffic light works. Imagine it at a crossroad directing the traffic; only instead of cars, it directs code execution paths.
Loading code snippet...
This C# program checks whether the number is greater than 5. If it is, it prints one message; if not, it prints another. Simple, isn’t it?
When to use If Else Statement in C#
We use the if else
statement whenever we want to choose between two execution paths based on a condition—a task we often encounter in programming. For instance, validating inputs from a user, performing actions based on program states, etc.
Breaking Down the Syntax
Just like how every house is unique but built upon the same basic structure, every if else statement follows the same syntax.
Loading code snippet...
Moving Forward: C# If Else If Statement
Sometimes, life isn’t as simple as a “yes” or “no” question. The if else statement is simple and powerful, but what if we have more than two conditions?Let me introduce you to the C# version of “Sophie’s Choice”, the If Else If statement.
Understanding the Else If Statement in C#
The If Else If statement in C# is like a complex flowchart. It checks conditions in order until it finds a true condition, or reaches the end of the chain.
Loading code snippet...
Applying Nested If Statements in C#
Besides using Else If
, we could also use nested If statements. It’s like those Russian nesting dolls where each condition contains more conditions within. However, while they technically accomplish the same goal, they can cause confusion with their indentation levels.
C# One Line Conditional
When your code includes many simple If Else
conditions, it can start to look like the Great Wall of China – long, repetitive, and tedious. That’s where one-line conditionals, a.k.a ternary operators, shine.
How to Apply C# One Line Conditional
The ternary operator (?:
) is an abbreviated If Else statement that you can use in a single line. It’s like those mini Swiss Army knives – small but versatile.Here’s the ternary operator in action:
Loading code snippet...
This C# program declares an int
variable number, then it uses a ternary operator to check whether number is greater than 0. If yes, it assigns “Number is positive.” to the message
string variable, otherwise it assigns “Number is not positive.”.
Using Ternary Operator in C#
Ternary operators are nifty, but when overused or with too complex conditions, they could actually make your code less readable. So, it’s vital to know when to use it.
The Difference Between If and If Else Statement in C#
Earlier, we compared If Else statements to traffic lights. If that’s the case, then an If statement is a one-way traffic light – it only cares about one condition, whether it’s true.
Working with Single line If Statement
With a one-way traffic light, cars only move when the light is green. Similarly, with an if
statement, code only runs when the condition is true. Here’s how it looks in C#:
Loading code snippet...
Exploring If Else vs If in C#
Whether you should use if
or if else
depends on what you need. If you only care about one specific condition, by all means, use if
. But if you want different behaviours for different conditions, then if else
is the way to go.
And there you have it, a comprehensive guide to the If Else statement in C#, along with its close relatives. Whether you’re a coding newbie or an experienced nerd? Oops! I mean a wizard, this fundamental concept is vital to mastering coding in C#. May your coding journey be filled with precise conditions and minimal bugs. Happy coding!