HttpClient in C#: Modern Web Communication
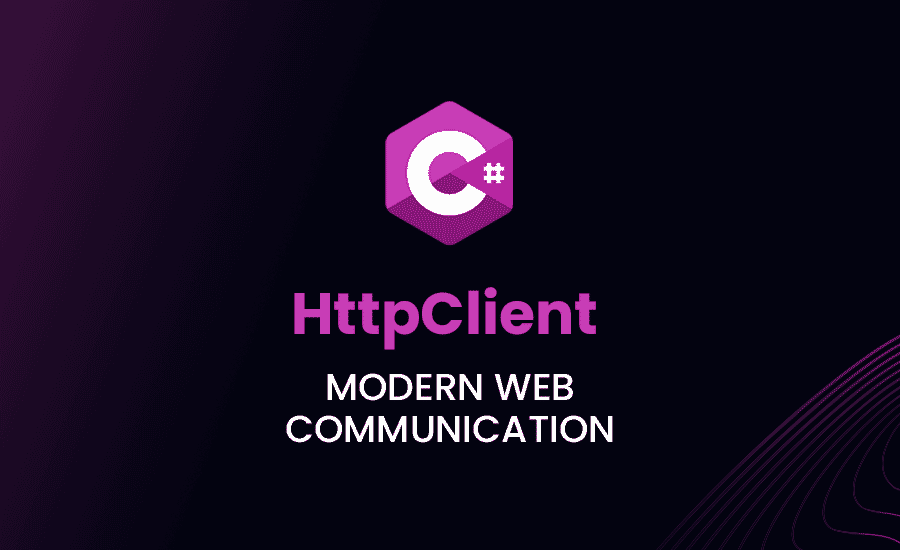
Introduction to HttpClient in C#
HttpClient is an exquisite library provided by .NET for sending HTTP requests and receiving HTTP responses from a resource identified by a URI. Before we start flexing our coding muscles, let’s pave the way by understanding why HttpClient is crucial in modern day development.
Why HttpClient C# is Critical in Modern Web Development
If you are working on anything web-related in C#, chances are, HttpClient is your new best friend. Think of it like a Swiss Army Knife of making HTTP requests. From talking to REST APIs and handling various methods like GET, POST, DELETE, to more intricate operations like handling authentication and setting timeouts, HttpClient has got your back.
Understanding the Basics of HttpClient C#
Alright, let’s get our hands dirty and dive into the nuts and bolts of HttpClient. Ready to geek out? Because we’re about to go on a journey through HttpClient land!
C# HttpClient Example: A Deep Dive
HttpClient lets developers consume HTTP resources from .NET applications seamlessly and efficiently. A typical pattern to use it is like this:
Loading code snippet...
This code is simplicity personified, right? It creates an HttpClient instance, sends a GET request, checks the response status, and reads the response content.
Exploring The Methods of C# HttpClient: GET, POST, PUT, DELETE
So we’ve seen the GET method in action. But HttpClient is not a one trick pony. It provides a robust set of methods mirroring HTTP methods, including:
- GET:
- POST:
- PUT:
- DELETE:
Making HTTP Requests Using C# HttpClient Post
Post-method in HttpClient is like a mailman delivering your packages (data, in our case) right at your doorstep (server).
How to Send Post Requests Using C# HttpClient
Sending a POST request is as simple (and fun!) as this:
Loading code snippet...
Just like our mailman picks up package from our home (JSON data, in this instance), HttpClient takes this content and delivers it to the configured URL.
C# HttpClient Post JSON: How to Send JSON Data
See the JsonConvert call up there? That’s Newtonsoft at work – a handy library for handling JSON like a pro! The JsonConvert.SerializeObject
call transforms the object into a JSON string, ideal for sending over the cyber-waves.
Getting to Grips with C# HttpClient Timeout
“Patience is a virtue”…but not when it comes to HttpClient. Let’s keep our HttpClient on a leash with a timeout!
C# HttpClient Retry Policy: How to Set It Up
Setting up a retry policy is straightforward:
Loading code snippet...
This HttpRequestWithRetry
function takes a delegate that represents an HTTP operation, and the retry count. If the operation fails, it retries until it either succeeds or hits the retry limit.
Handling Authorization in HttpClient C#
Does HttpClient know how to unlock the door when it finds one? Absolutely! Let’s check out how HttpClient handles authorization.
HttpClient in C# and Authorization Header: How to Add It
In life, keys open doors. In HttpClient, Authorization headers open HTTP doors:
Loading code snippet...
Our magical key here is the “Bearer” token” in the Authorization header.
User Agent and Redirect in C# HttpClient
Ever wanted your HttpClient to be a master of disguise or a pro pathfinder? Meet User-Agent and Redirects!
Setting up User Agent with C# HttpClient
Have a look at how seamlessly HttpClient changes its disguise:
Loading code snippet...
How to Follow a Redirect with C# HttpClient
And here’s how HttpClient becomes a pro pathfinder by following redirects:
Loading code snippet...
If the status code screams “redirect” (301 in geek parlance), HttpClient simply follows the new path.
HttpClient vs WebClient in C# : A Comparative Analysis
We’ve talked a lot about HttpClient, but what about WebClient? is it an apples vs. apples comparison or are we dealing with apples and oranges here?
Overview: C# WebClient vs HttpClient
Think of WebClient as the older sibling – easier to use, but less feature-rich. HttpClient, the younger one, is more powerful, flexible, and extensible, albeit a bit more complex. It really depends on what you need for your particular scenario.
Conclusion
With HttpClient by our side, communicating with the world (the world wide web, to be precise) through your C# application just got a whole lot easier. Congrats, you just unlocked a new C# superpower! Now go forth and code your way to greatness! Remember: With great power comes great responsibility… or was that something else? Anyways, happy coding!