C# Hashtable Class: Explained with Examples
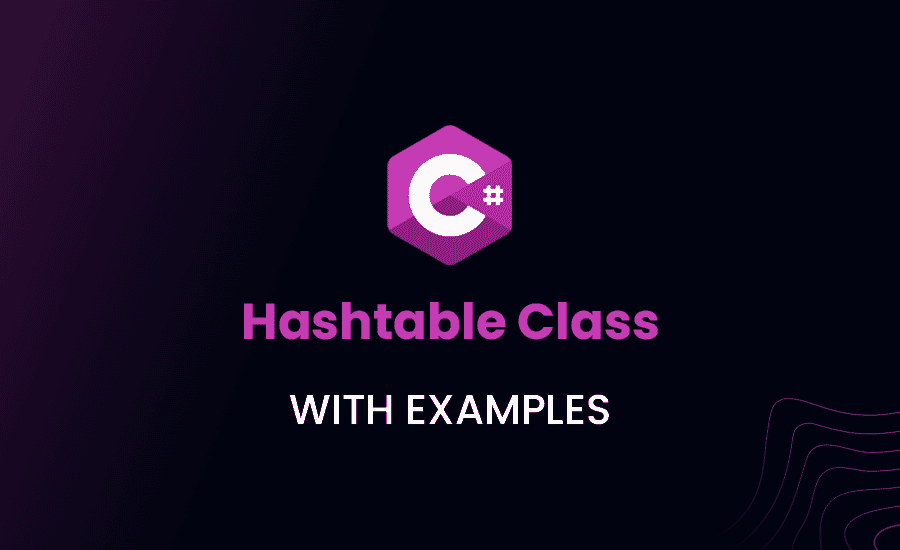
C# has gifted developers with many data structures, but for certain scenarios, nothing comes close to the efficiency of the Hashtable class. Dip your toes in, and let’s decode the power of Hashtable together in this comprehensive guide!
Introduction to Hashtable in C#
We can’t dive into Hashtable without first understanding what it is. Hashtable, present in the System.Collections namespace, is a non-generic collection that stores key-value pairs.
Imagine you’re juggling a bunch of different soccer balls, each one associated with a particular player by the number on the ball. Hashtable is just like that – it helps you keep track of which value (‘soccer ball’) is connected to which key (‘player’). Not so hard, is it?
Now, let’s talk about when Hashtable really comes into its own.
When to Use Hashtable in C#
Like many things in life, selecting the appropriate data structure is all about the right place and the right time. Hashtables are your go-to when:
- You want rapid retrieval. The complexity of search operations in Hashtable is O(1), which basically means it’s super quick!
- You need to store data in key-value pairs. Just like our soccer ball example.
- Each key you have is unique. Remember, no two players can have the same ball!
Now that we’ve got the timing right let’s look under the hood to see how this powerful structure works.
How Hashtable Works Internally in C#
Hashtables have an interesting way of storing data. It saves the data in an array format where each data value hash is used to calculate the storage location. That’s why it’s faster to locate an individual item in the hashtable.
Imagine you’re in the biggest library in the world – but the books are not sorted in any way. You could end up spending a whole day trying to find a single book! Hashtable acts as a super efficient librarian here, sorting those books in such a way where each book and its location in the library can be instantly recalled.
But how does this system work, you ask? Let’s delve a little deeper.
Methodology of Hashtable in C#
When a hashtable is created, a certain amount of memory, known as a ‘bucket’, is allocated to it. A bucket is like a shelf in our hypothetical library. Now, whenever you want to add a value to the hashtable, a hash function is applied to the key associated with the value.
In simpler terms, our ‘key’ is the book you want to store and the ‘hash function’ is the method the librarian uses to decide where the book belongs on our ‘bucket’ or shelf. The result obtained from this hash function determines the index of the bucket.
That’s the magic of Hashtable – accessing any value in the bucket shelving system becomes a piece of cake!
Loading code snippet...
In this example, numbers 1, 2 and 3 are the keys, and “Goalkeeper”, “Defender”, “Midfielder” are their corresponding values. We’re storing them in the hashtable, and then retrieving the value using the key, just like fetching a book from a shelf!
So far so amazing, right? Hang in there, we’re just getting started. Our next stop on this Hashtable adventure is the fun world of hash functions.
Unraveling the Power of Hash Functions: Creating Hash in C#
Alright, let’s roll up our sleeves and get ourselves into the nitty-gritty of hash functions. These are special functions used in hashtable to calculate the index for storing data.
Just like the librarian has a unique way of sorting the books in our big, chaotic library. The hash function helps us to store and retrieve data quickly.
But the big question is, how exactly do these hash functions work to create hash in C#?
Loading code snippet...
In this example, our hash function GetHashCode
receives a key, converts it into a string, and applies a bitwise AND operation with 0x7FFFFFFF (a hexadecimal number). The resulting hash code is used to determine the index of the bucket where the data will be stored. So, you see, hash functions aren’t some secret magic, it’s simple mathematics!
Now, it is important to mention that there are several ways to generate hash codes in C#. The above method is just one of them.
Before we move on, let’s take a moment to discuss some of the applications and benefits of using hash functions.
Applications and Benefits of Using Hash Functions
The magic of hash functions is the way they make our coding lives easier. Here are a few reasons why we love them:
- Rapid data retrieval: Instead of searching an item in O(n) time, we can use a hash function and find it in impressive O(1) time.
- Data security: Hash functions are widely used in cryptography. They can help to secure data, as each unique key can produce a different hash code.
- Data integrity: By comparing hash codes, we can easily check whether data has been modified or not.
Now, it’s time for us to dive into another interesting aspect of hashtable. Can we serialize this data structure in C#? Let’s find out!
Understanding the Concept of Serializing Hashtable in C#
A serialized hashtable is like a suitcase where you neatly pack your hashtable before going on a long journey (or sending it over a network). Serialization is the process of converting the state of an object into a form that can be persisted or transported.
So if we want to transport a Hashtable over a network or want to store it in a file for later use, we serialize it.
Can We Serialize Hashtable in C#: A Technical Inquiry
The big question: Can we serialize Hashtable? The answer is: absolutely yes!
Loading code snippet...
Fear not, this isn’t rocket science. This code simply creates a Hashtable, adds a couple of values to it, and then —with a little help from BinaryFormatter and FileStream— serializes the Hashtable and stores it in a file called “MyHashtable.ht”. Fun, isn’t it?
The most fun part about programming is learning about different ways to achieve the same task. Talking about different ways, let’s take a detour and understand how Hashtable and HashMap vary in C#.
Comparing Hashtable and HashMap in C#
Both Hashtable and HashMap follow the principle of hashing. However, they are distinct entities. Hashtable is a class in C#, while HashMap is a class corresponding to Java. Despite the differences, the core concept of storing and retrieving data based on hashing remains common.
A Brief Overview of HashMap in C#
HashMap is a similar concept to Hashtable, but it’s primarily used in the Java world. It also works on the principle of Hashing, just like Hashtable. It, too, stores key-value pairs and provides quick access to data.
However, despite the similarities, there’s a lot that sets HashMap and Hashtable apart.
Making the Right Choice: Hashtable vs. HashMap
Here are a few points that will help you identify the differences and make the right choice between Hashtable and HashMap:
- Thread Safety: Hashtable in C# is thread-safe — it can be shared between multiple threads without causing data corruption. On the other hand, HashMap is not thread safe.
- Null Keys and Values: Hashtable doesn’t allow null keys or values. If you try to store null, it will raise a runtime exception. But HashMap does allow one null key and any number of null values.
- Performance: Since Hashtable is thread safe, it’s slower than HashMap. Synchronized methods usually have poor performance in comparison to their non-synchronized counterparts.
Remember, a wise developer always makes an informed decision based on the requirement!
Now, we’re getting to the fun part! Let’s see Hashtable in action. We are going to run some codes. We’re not just going to read it, but actually do it!
Examples and Use-Cases of Hashtable in C#
There’s no better way to understand Hashtable than by trying it out yourself!
How to Implement Hashtable in C#: A Step-by-Step Guide
In this first example, let’s create a Hashtable and add some key-value pairs to it.
Loading code snippet...
Here, we’re creating a new Hashtable, adding some interesting keys and values (all fruits), and then printing them out. When you run this code, it will print out each of the keys and values that we’ve stored in my_ht
.
Real-World Problems Solved By Hashtable in C#
Developers often use Hashtable to solve real-world problems. Think of it as a bridge between your problem and the solution.
Here’s an example: imagine you’re a school teacher, and you want to quickly access the grades for each of your students. Hashtable is the perfect tool for this!
Loading code snippet...
In this example, we are creating a Hashtable where each key is a student’s name, and each value is that student’s grade. This way, we can look up the grade of any student in no time at all. Pretty handy, right?
Wrapping Up: Unveiling the Power of Hashtable in C#
By diving headfirst into C# Hashtable class, we have not only figured out how to use this powerful data structure, but also how it works behind the scenes.
From the surface applications to the hidden secrets of hashing, from serialization to real-world examples, we’ve glimpsed almost all aspects of Hashtable.
Key Takeaways: Gaining Advantage from Hashtable in C#
So, to sum up our lessons:
- Hashtable is an essential part of C# that allows quick data retrieval by utilizing the concept of hash functions.
- Hashtable is just like a library membership card where the barcode (key) is used to look up your data (books).
- It’s essential to wisely choose the data structures for your applications – Hashtable isn’t always the answer, but when it is, it’s your best friend.
- Not only we can serialize Hashtable, but we also have the power to alter the hash function according to our needs.
- Differentiating between Hashtable and HashMap can avoid confusion and helps in making informed choices.
And with these takeaways, I hope you’ve discovered a passion for Hashtable and have a clear understanding of where it will come in handy the most!
Yes, it was quite a journey. Overwhelming? Maybe a little, but once you get the hang of it, C# Hashtable will be a breeze for you.
Remember, practice makes perfect. So, don’t hesitate to roll up your sleeves, play around with the codes, make mistakes, and have fun during your programming journey because the enjoyment is in the process. Happy coding!