Getters and Setters in C#: Detailed Explanation
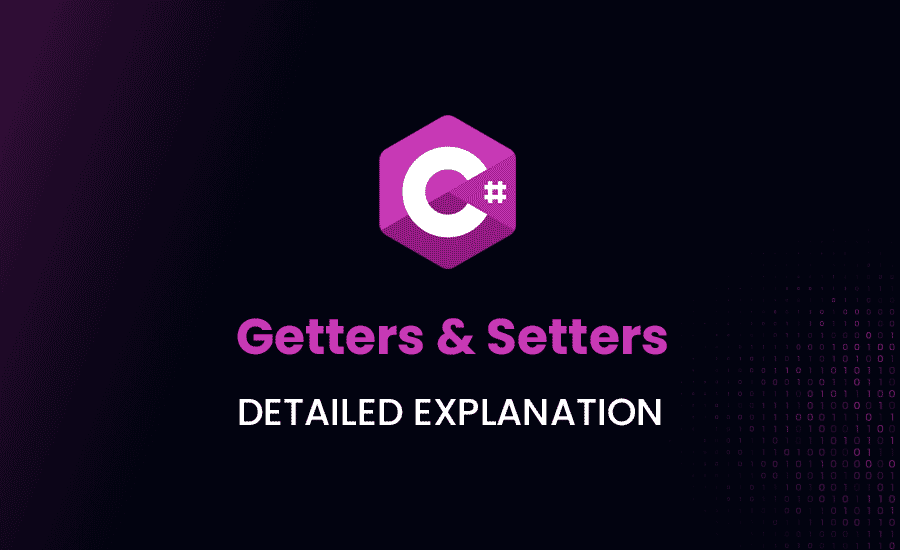
Have you ever felt like your C# programs could be more efficient? Felt like you can’t quite access your data the way you want to? Well, guess what! C#’s getters and setters might be your programming heroes. So, let’s embark on this code-laden journey and explore the universe of getters and setters in C#!
Getters and Setters C#
Imagine the most enticing detective novel you’ve ever read, filled with secrets and locked rooms. Getters and setters are like those unforgettable characters who are the masters of keys! Now isn’t that interesting for a C# concept?
Defining What are Getters and Setters in C#
You might be wondering, “What exactly are these getters and setters?”. Well, in terms you ‘d use at your buddy’s barbeque, getters and setters in C# are methods used to control the access to class variables. In other words, they’re like bouncers at a club, guarding who gets to see the VIP—your data.
Here’s a quick example;
Loading code snippet...
In this snippet of joy, _x
is our Embarrassing Secret. We don’t want it directly accessed by anyone, remember? That’s where X
swoops in as our Bold Bouncer, granting access via getters and setters!
Understanding What Setters and Getters Do in C#
“But, in which scenario would I use which one?”, I hear your inner developer wondering. Simply put, a getter (get) fetches the value of a variable, while a setter (set) assigns a value to it.
Take a look at this example:
Loading code snippet...
Notice how we’re using X
to access _x
indirectly? That’s the power of getters and setters in action, folks!
Why You Need Getters and Setters in C#
OK. So, you’ve had your taste of what getters and setters are and what they do. Let’s move on to another burning question – “Why do I need to use them?”
Do I Need Getters and Setters in C#
Getters and setters control access to your data. Think of it like this: Would you want your 3-year-old nephew playing with your priceless Ming Dynasty vase? Absolutely not! Similarly, you don’t want every part of your code meddling with your valuable data.
Loading code snippet...
Now that’s the guard dog you want! By creating a setter with a condition, you’ve ensured that _myValue
can only be set to a positive integer. Sadly, there’s no guard dog for your vase yet!
How to Implement Getters and Setters in C#
“Time for some taskmaster code examples!”, I hear you say. Absolutely! Let’s understand how to use getters and setters in C# with some nifty examples.
How to Use Getters and Setters in C#
Here’s a simple task: creating a Person
class that holds a person’s name, but the name can only be set once. Sounds like a job for our bouncer set
, right?
Loading code snippet...
You see, our setter just saved our class from potential data manipulation chaos!
C# Generate Getters and Setters
“But what if I’ve got a class filled with properties?”, fret not. Visual Studio is here to save your day (and your fingers from typing) with the ‘Encapsulate Field’ refactoring command.
Simply right click on the field you want to encapsulate, go to Quick Actions and Refactorings
and click Encapsulate field
. Boom! A ready-made getter-setter pair!
Best Practices when Utilizing Getters and Setters in C#
Expanding Knowledge on Getters and Setters in C#: GUIDING PRINCIPLES AND BEST PRACTICE.
Understanding the ins and outs of setters and getters in C# is one thing, but learning how to utilize them effectively is another ball game. Let’s journey deeper and explore some best practices that will help transform your programming style.
C# Getters and Setters Best Practices
Knowing when and how to use getters and setters effectively is crucial. Here’s a set of golden rules to consistently create reliable and well-structured code:
- Loose coupling, high cohesion: Regard this as your Code of Arms while dealing with getters and setters. Strive to minimize dependencies among classes (loose coupling) while ensuring that related properties belong together (high cohesion).
- Getter methods should not change the state of the object. They are intended to provide information, ‘getting’ the required data for you. Let’s consider this in code:
private double speed; public double Speed { get { speed =+ 5; // Not advisable return speed; } }
You’ll notice that within the getter, we’re modifying the speed
, which isn’t ideal. A getter should be as unharmful as reading a book – it tells us an interesting story, but doesn’t affect the story itself.
- Setters shouldn’t process complex calculations or operations. They should be used primarily for setting values. Think of setters as the unsung heroes who do a lot of heavy lifting behind the scenes, but their job is not to solve the world’s problems. They are hard-working, but they’re not superhuman.
private int radius; private double area; public int Radius { set { radius = value; area = Math.PI * Math.Pow(radius, 2); // Not advisable } }
In the code above, the setter is doing more than just setting a value. It’s calculating an area
. Instead, the area
calculation should be done in a method – like calculateArea()
– to maintain clean code and single responsibilities for your methods.
- Always check input validity in the setter. It’s a cruel world out there! You don’t want to let a sneaky illegal value infiltrate your clean data. Consider this sample:
private string email; public string Email { set { if (value.Contains (“@“) && value.Contains (“.”)) email = value; else throw new Exception (“Invalid email address!"); } }
In this piece of code, our setter is working like a vigilant guard at the gate, checking all incoming emails for validity with a simple validation rule.
Avoiding Common Pitfalls in Using Getters and Setters
Getting stuck on the slippery slope of overusing getters and setters is easier than you might think. Here’s how to avoid falling into the trap:
- Use getters/setters only when you need to control access. They are not decorative items to put in every part of your code. If your data does not require any specific behavior or restrictive access, then you don’t need to use getters and setters. Let your data roam freely in the wild fields of your code!
- Avoid using setters/getters interchangeably between tightly coupled classes. Tightly coupled classes depend heavily on each other, and adding setters and getters in between tends to complicate things unnecessarily. It’s like adding speed bumps on a Formula One track. Needless to say, it’s not going to make the race any smoother.
Applying Your Knowledge: C# Setters and Getters Examples
Having covered the theoretical aspect, it’s time to dive into practical waters. There’s no better way to understand the application of knowledge than through real-world examples. Let’s get our hands dirty with C# code!
Understanding Practical Scenarios: C# Getters and Setters Examples
Let’s reiterate by taking another look at our good old Product
class that applies getters and setters in C#:
Loading code snippet...
In this context, our setter is the gatekeeper, ensuring that no negative stock
value can enter our Product
class. Thus, our data always remains valid.
It’s time to further broaden our getter and setter horizons. Let’s create a BankAccount
class. We’ll give it a balance field with a public getter, but a private setter (a common practice to protect important fields). This way, the BankAccount
class can tightly control how balance changes.
Loading code snippet...
In the example, only the Deposit
method changes the balance, ensuring it meets any business rules (such as not depositing negative amounts). Here, encapsulation through a private setter helped us control changes to balance and simplify what could otherwise be a complex system.
Conclusion: Becoming Proficient With Getters and Setters in C#
From understanding the basics, to real-world examples and best practices, we’ve taken quite the journey uncovering the secrets of getters and setters in C#. Now, isn’t that a journey worth taking again?