What is Func Keyword in C#? Main Usages
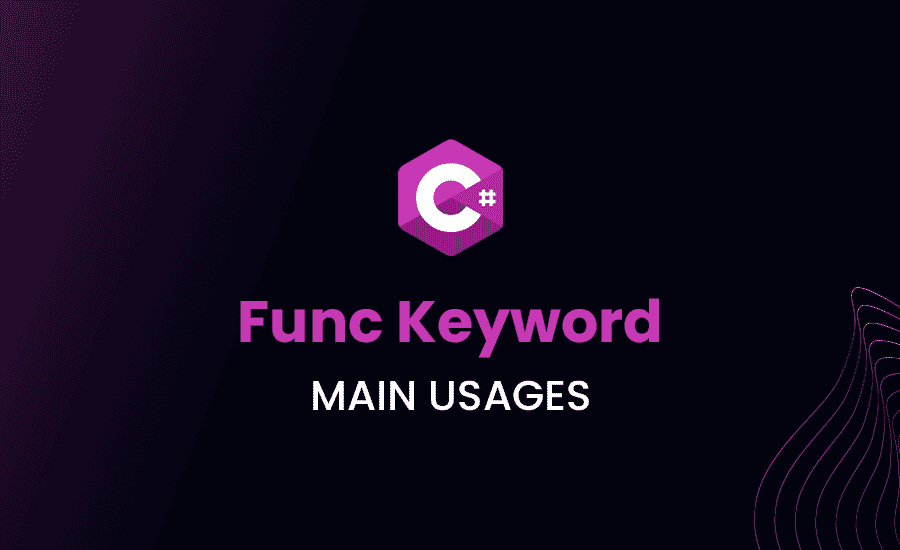
Ever wonder what makes C# such a versatile and powerful object-oriented programming language? It’s all about its commuting features like the Func keyword. Intrigued, aren’t you? Let’s grab a coffee and delve into the fascinating world of Func Keyword in C#.
Introduction to C# Func Keyword
Hang on to your hats, folks! We are just getting started.
Brief Overview of Func Keyword in C#
Func is a delegate type defined in the System namespace of .Net Framework. The rock-bottom definition is easy, right? But what does it do? Imagine Func as a chameleon – ready to adapt atop a flexible parameter model.
Loading code snippet...
We’ve got a Func delegate here that takes two integers as input and returns a boolean value. The delegate checks if the first integer is smaller than the second.
Importance of Func Keyword in Modern C#
Why should you tweet about Func? Because Func keyword acts as a bridge between delegates and lambda expressions. This fusion provides more expressive ways to encode algorithms, enhancing the flexibility in function calls.
Loading code snippet...
An exciting combo! We have a Func delegate here that takes an integer, squares it using a lambda expression – voila!
What is Func Keyword in C#
You’re getting the hang of Func now, right? Let’s dig deeper.
Definition and Functionalities of C# Func Keyword
The Func keyword in C# presents a leap towards a functional programming paradigm. Believe it or not, it can take up to 16 input parameters. It always returns a result – that’s a mandatory contract. This last generic type parameter is always the return type.
Loading code snippet...
Seen here, a Func delegate includes three input integers and returns their sum. Easy peasy, right?
Understanding the Structures of Func Keyword in C#
Func Keyword, an Esperanto in the C# world, is the vessel for hosting anonymous methods or lambda expressions. Remember the rule: It always has a return type, unlike Action delegate. Even if you’re not explicitly declaring, it’s void by default.
Loading code snippet...
Boom! A Func delegate with no input parameters, returning a random number between 1 and 100.
Guide to C# Func Keyword Usage
Now that we’re through theory, let’s master the practical use of Func keyword in C#.
Basic Examples of C# Func Keyword Usage
First, we’ll start with some basic examples. Here, we’re creating our first Func delegates that take one or more input parameters and return a result.
Loading code snippet...
The above Func delegate is receiving one integer as input and returning the doubled result.
Advanced Usages of the Func Keyword in C#
You’ve mastered the basics. Now, let’s navigate the rough waters of advanced usage of Func. Ever heard of lazy evaluation? That’s our pit stop!
Loading code snippet...
This is a Func delegate simulating a delay as in data fetching from a database. Noticed the lazy evaluation, right? The actual execution of the function happens when we invoke the delegate, not when we assign it.
C# Func Keyword and Lambda Expressions
Are you ready for another twist? Func and Lambda expressions make an impressive team. Let’s explore!
Connection Between Func Keyword and Lambda Expressions in C#
As you’ve already seen, Func delegates frequently team up with Lambda expressions. This partnership offers concise and expressive ways to write algorithms without the need for full method definitions.
Loading code snippet...
In this example, the Func delegate uses a lambda expression to find the maximum of two numbers. Fully functional and yet so compact!
Practical Examples of Combining Lambda Expressions with Func Keyword
Let’s walk through some practical examples of using Func keyword and lambda expressions together.
Closer look at the code below, Func delegate partnered with lambda expressions to calculate the product of an array of integers. Are you not entertained?
Loading code snippet...
C# Func Keyword Best Practices
A journey with the C# Func keyword is surely thrilling, but it also needs smart navigation and a solid understanding. With mastery comes the responsibility of knowing the best practices and safeguards regarding its usage. So, here we are, helping you flex the best use of Func keyword in C# and avoid common pitfalls along the way. Ready to level up your game? Let’s dive straight in!
Useful Tips for Using Func Keyword in C#
Knowing some useful tricks and tips up your sleeve about the Func keyword can make your coding experience smoother and cleaner. Here’s what a seasoned C# programmer would advise:
- Keep it Short: The very strength of Func delegates lies in their conciseness. It’s always beneficial to keep your func expressions short and straightforward. Prolonged, multi-lined Func delegates can make your code look cluttered and obscure its readability.
Loading code snippet...
The second expression is much concise and easier to read, isn’t it?
- Use Action for Void Return: Func keyword in C# always returns a value. If your delegate doesn’t need to return a value, consider using the Action delegate instead to enhance code clarity.
Loading code snippet...
Here, the Action delegate printSum
avoids the unnecessary empty string return of the Func delegate and executes the Console.WriteLine() directly.
- Leveraging Lambda Expressions: The beauty of the Func keyword shines when you use it along with Lambda expressions. It’s an elegant tool for writing cleaner, functional-style code.
Loading code snippet...
Here, the isPrime
delegate succinctly checks for a prime number using a lambda expression, a truly powerful representation of the Func delegate.
Common Mistakes and How to Avoid Them
- Runtime vs Compile Time Checking: Remember, Func delegates enjoy the comfort of runtime checking. It’s imperative to thoroughly test your delegates to avoid an unexpected exception during runtime.
- Remembering the Return Type: A common mistake beginners often make is forgetting that the last type parameter for the Func keyword is always the return type.
Loading code snippet...
In these code snippets, the first one will give a compile-time error. The developer mistakenly anticipated ‘int’ as the return type, which is incorrect. In the second snippet, ‘int’ is indeed the return type, making it a correct Func declaration.
What’s stopping you from diving in and exploring the wonders of Func keyword? C’mon, use the Func, and let’s code!