For Loop in C#: Concept and Implementation
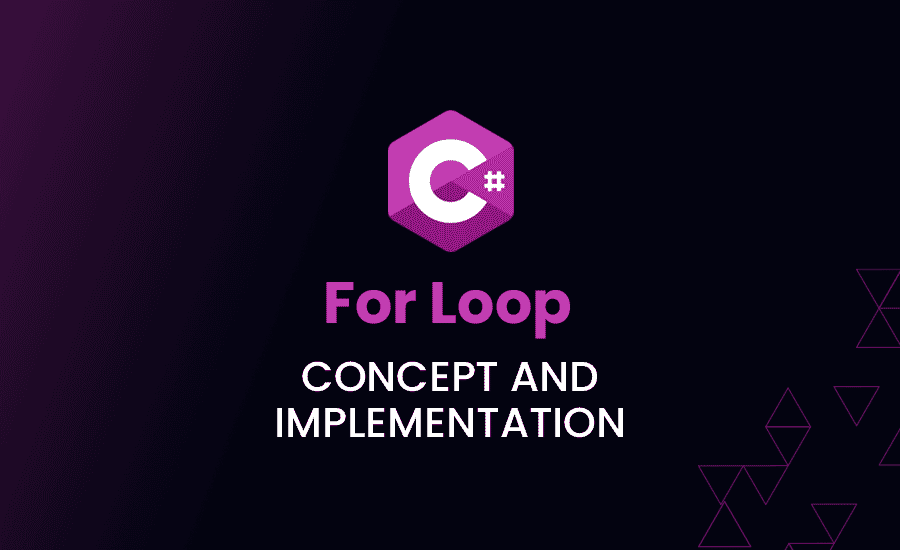
This article provides a guide to for
loops in C#, covering everything you need to know about its syntax, usage examples and some best practices.
Introduction to Looping in C#
Ever had to repeat an action several times in your code, like a parrot repeating a phrase? Or maybe you felt like deja vu was hitting you hard while coding? If so, then loops are your salvation.
What is Looping in C#
In C#, a loop is used to execute a block of code repeatedly until a specified condition is met. It is the bread and butter of any programming language when dealing with multiple repetitive tasks. But hold on, there’s more! Let’s dive deeper.
Types of loops in C#
In C#, there are four types of loops, and each has its strengths and uniqueness. You’ve got the for
loop, while
loop, do-while
loop, and the foreach
loop. It’s like having a different power tool for every job, isn’t it awesome?
Understanding the C# For Loop
Now let’s get to the star of the show, the for
loop. Let’s answer the fundamental questions – What it is? How does it work?
What is a C# For Loop
A for
loop is a control flow statement for specifying iteration, which allows code to be executed repeatedly. Think of it like the movie director, and it calls the shots on how many times a specific scene (your code) should be repeated.
How does a C# For Loop work
A for
loop works by first initializing a variable, then it checks the condition. If the condition is true, it executes the block of code. After executing the code block, it updates the variable and again checks for condition. This process repeats until the condition becomes false. Here’s some code;
Loading code snippet...
Here, i
is initialized to 0. Then i < 10
condition is checked. If it’s true (and it is true initially), the statements inside the loop execute. Once done, i
gets incremented by 1, and the condition is checked again. The loop keeps going until i < 10
becomes false, and then it moves onto the next part of your code. Easy right?
The Anatomy of C# For Loop Syntax
But, of course, you don’t want to only use for
loops for counting from 0 to 9, right? You want to be able to use it for more complex situations. That’s where understanding its syntax becomes vital.
Structure of C# For Loop Syntax
The syntax of a for
loop in C# is:
Loading code snippet...
This might look like some secret agent code, but let’s break it down:
Explanation of each part in C# For Loop Syntax
- Initializer: This sets the starting value for the counter variable. It’s like when you reset your game and start back at level 1.
- Condition: This is a boolean expression that is evaluated before each iteration. The loop continues as long as this condition is true.
- Incrementor/Decrementor: This modifies the counter variable after each iteration. It’s like going to the next level in your game.
An example would be:
Loading code snippet...
In this case, int i = 0
is the initializer, i < 10
is the condition, and i++
is the incrementor.
Dive Deeper into C# For Loop Step
But wait! What if you want to count by twos, or tens, or even go backward? That’s when for
loop Step comes in.
What is C# For Loop Step
The “Step” is actually referring to the increment/decrement part of the for
loop. It’s not just limited to adding one, let’s say you’re in a hurry and you want to iterate by 2 or any number for that matter. It’s all game here!
How to Modify C# For Loop Step
You can easily modify the step by changing the increment/decrement section of the for
loop syntax. Let’s skip count by 2 using for
loop:
Loading code snippet...
Notice i += 2
? That’s Instagram-worthy skipping through numbers!
Breaking Down the C# For Statement
Ready to go even deeper? No ‘for’ loop discussion can be complete without talking about the for
statement itself.
Structure of C# For Statement
The for
statement provides a compact way to iterate over a range of values. Programmers often refer to it as a counter-controlled loop. Here’s the syntax:
Loading code snippet...
Just like before, but a little more formal.
The Role of C# For Statement in Loops
The for
Statement is where the element of control comes in with for
loops. It’s the director, controlling the flow of the loop, making checks and stepping through the iterations.
Think of it as a strict teacher overseeing you as you practice your scales in piano lessons: “Do these scales this many times”.
Rich Repository of C# For Loop Examples
Reading about for
loops is great, but seeing them in action is even better. Let’s give you some concrete examples to help solidify your understanding.
Simple C# For Loop Examples
Here is a simple example of a for
loop that prints numbers from 1 to 5 on the console:
Loading code snippet...
Voila! It’s like magic right? But you’re the magician!
Advanced C# For Loop Examples
Now, let’s say you want to print the first ten numbers of the Fibonacci sequence. Like the famous mathematician, Fibonacci himself, you can do this:
Loading code snippet...
Now you’re not just a coder, you’re also a mathematician-in-the-making!
Closing the Loop: Troubleshooting Common Issues in C# For Loop
Even the most experienced coders run into problems with for
loops from time to time. Before you tear out your hair in frustration, let’s look at some common issues and how to fix them.
Common Mistakes when using C# For Loop
Here are some common pitfalls to watch out for:
- Forgetting to initialize the loop variable: Remember the starting value!
- Not setting up the right condition for the loop: This could cause an infinite loop, and nobody wants that!
- Misunderstanding the iteration part: Be sure you understand how your loop variable is being updated.
Solutions to typical C# For Loop Errors
The good news is, these problems are easy to fix:
- Don’t forget to set an initial value.
- Make sure your condition is correct and will change eventually.
- Set your iteration to something that makes sense. Counting by one (
i++
) is common, but remember, you have other options.
Usage of the C# For Loop in Real-world Applications
Theoretical knowledge is great but being able to apply what you’ve learned in a real-world context is even better. We’ll take a look at some concrete situations where you’d use a for
loop in C#.
Practical Scenarios where C# for loop applies
Here are some noteworthy applications:
- Reading and processing items from an array or list
- Implementing mathematical algorithms
- Automating repetitive tasks
Case Study: Real-world problem-solving using C# For Loop
A real-life example could be the creation of a digital clock using a for
loop to ensure that the minutes and seconds don’t exceed 59, and hours don’t exceed 23.
Loading code snippet...
This example might make you feel like a time wizard!
Recap of Key Learning: C# For Loop
You’ve come so far and learned so much! Now that we’re wrapping up, we’d like to highlight the essentials that you may want to keep bookmarked:
- Anatomy of a
for
loop - Stepping in C#
- Implementing a statement in
for
loops - Common errors and how to debug them
- Real-world application and usage
And remember, no problem is too big for a coder with a for
loop at their disposal!