C# Stack: How and When to Use it?
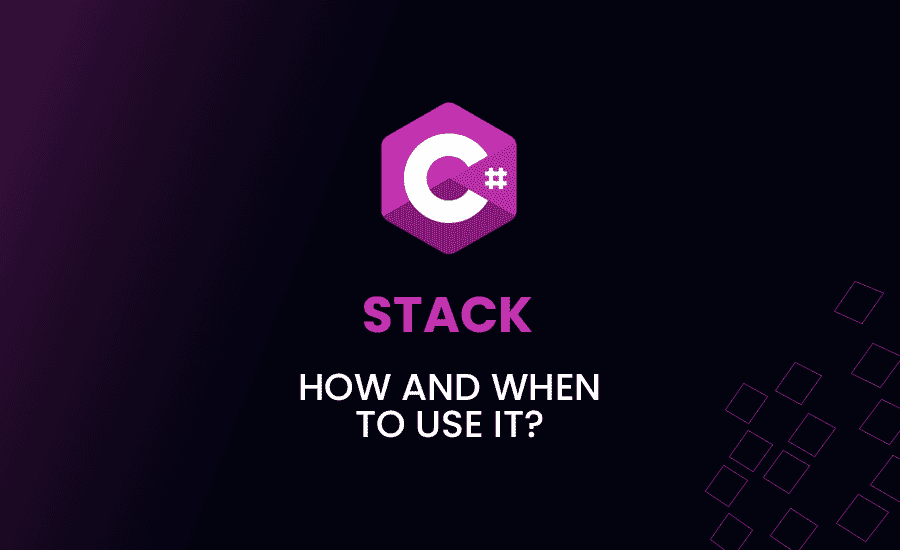
Understanding the C# Stack
Oh boy, we’re in for a thrilling journey! We’ll lift the veil from one of the intriguing aspects of C# – The C# Stack. By understanding this fundamental concept, you’re actually unlocking a vault of efficient programming possibilities.
Background of C# Stack
Alright! Ever asked yourself how it all started? Well, back in the late 1950s and early 1960s, when computer science was still an emerging field, the concept of ‘Stack’ was introduced by an Australian computer scientist named Charles Leonard Hamblin. The genius recognized the need for a new data structure to optimize computing operations. Today, nearly every modern programming language, including our very own C#, has adopted this concept, giving birth to what we now know as the C# Stack.
Loading code snippet...
This code above simply demonstrates creating a stack named ‘history’ and pushing the elements “Introduction” and “Birth of Stack” into it.
Basic Definition of C# Stack
Alright, cutting to the chase, a Stack in C# is a dynamic data structure that follows the LIFO (Last In First Out) principle.
Loading code snippet...
Here, we’re using the Pop
method to remove the last element. Remember LIFO? So, yeah, “Birth of Stack” gets removed because it was the last one in.
The Position of Stack in .NET Stack
I know what you may be thinking: ‘What the heck is the .NET Stack?’ Well, the .NET stack encompasses technologies in the .NET ecosystem in which the C# Stack fits in gracefully. Let’s not get drowned in terminologies, okay? Just think of it as the Microsoft family where C# Stack is a proud member.
Writing Stack in C#
How exciting! Now let’s get our hands dirty and delve into the hows of writing Stack in C#!
Step-by-step Guide to Writing Stack in C#
Time for action! Here, we’re going to embark on the practical task of actually writing stack in C#. Remember, practice makes perfect!
Loading code snippet...
In the snippet above, we’ve declared a stack of integers and pushed a few elements into it. Of course, we’ve printed it out to behold the amazing stack we just created!
Common Errors to Avoid When Writing Stack in C#
Now, I’ll let you in on a secret. Making mistakes is often the quickest path to becoming a pro. And trust me, you’re not alone. So, let’s talk about a few common errors and those nasties to avoid when dealing with a stack in C#.
- Neglecting to check for an empty stack before
Pop
orPeek
operationsStack<string> emptyStack = new Stack<string>(); emptyStack.Pop(); // Big no-no! Avoid this.
- Mistaking the order of elements, forgetting it’s LIFO, not FIFO (Don’t mix it with Queue, buddy!)
- Not handling
InvalidOperationException
for unexpected circumstances
Stack Vs Heap in C#
Just when you thought we were done! It’s time to gear up for an exciting face-off: Stack versus Heap. This showdown will provide some profound insights that will make your C# journey even more interesting.
Understanding Stack Memory
Let’s start with our hero, Stack. Stack Memory is used for static memory allocation and contains primitive values and reference types. It’s super-fast (Ferrari-fast), but it’s size-limited, hence the ‘StackOverflowException’ if overloaded.
Understanding Heap Memory
Contrasting our hero, we have Heap Memory. The heap is the part of memory where our C# objects dwell; it’s slower than Stack (Think, old reliable snail), but it flaips the script with its larger storage capacity for storing complex objects and large arrays.
Differences Between Stack and Heap in C#
Enough of the chit-chat, let’s bullet these down:
- Stack follows LIFO; Heap doesn’t have an order (Wild eh?)
- Stack speed? Lightning-fast! Heap speed? More like, steady.
- As for size – Stack is a neat little package while Heap is the big brother.
C# Stack Methods
Alright folks, let’s take a look under the hood. And by that, I mean let’s dive into the innards of the C# Stack by getting acquainted with its methods.
List and Explanation of Stack Methods
C# Stack is equipped with a bunch of handy methods:
- Push(T) : Pushes an item to the top of the Stack.
- Pop() : Removes and returns the item at the top of the Stack.
- Peek() : Returns the item at the top of the Stack without removing it.
- Clear() : Removes all items from the Stack.
- Contains(T) : Determines if an item is in the Stack.
- toArray() : Copies the Stack to a new array.
Loading code snippet...
In our above example, we used the Push
and Peek
method. We pushed 5 into our stack and Peek
ed to see what was at the top – it was 5, as expected!
Examples of How to Use Various Stack Methods
Heroes come in all sizes. And in the programming world, methods are our unsung heroes! So, let’s explore a few more Stack methods with some examples, shall we?
Loading code snippet...
Here, we used Pop
, Contains
and ToArray
methods. Isn’t it amazing how these methods make our stack operations straightforward?
Practical Uses of Stack in C# Programming
Programming and real-life examples mesh seamlessly. And this includes the concept of Stack in C#. Let’s tug at this string further, shall we?
Stack in Data Structure Problems
Hey, solving problems is what we do, right? By leveraging the LIFO functional principle of Stack, we can solve a myriad of data structure problems. From balancing parentheses in an expression, to reversing words in a sentence, the Stack helps us put into action an uncomplicated, efficient solution.
Using Stack in Algorithm Development
Algorithms are the heart and soul of problem-solving in computer programming. Rooted in the C# Stack, algorithm development can be a piece of cake.
Loading code snippet...
The above code uses a Stack to reverse a number. Isn’t this cool or what?
Working with Stackoverflow Exceptions in C#
Wait, what? Did I just use the scary ‘E’ word? Yes, I did! Exceptions… Stackoverflow exceptions to be precise. Let’s unravel this mystery!
What is a Stackoverflow Exception?
Not to scare you, but a Stackoverflow Exception arises when the Stack (our memory champ) is exhausted. Think of it as the Stack waving a white flag asking for a break or… reduce the load, maybe?
Identifying Causes of Stackoverflow Exceptions
Most of the time, these exceptions occur due to infinite recursion or significant space complexity. It’s like continually asking your buddy to remember more and more stuff – soon enough; they’re going to blow a fuse!
How to Deal with Stackoverflow Exceptions in C#
It’s not all doom gloom guys, as there are ways to debug and handle StackOverflow Exceptions, including:
- Reviewing code for possible infinite recursion
- Optimizing space complexity
- Catching and properly handling exceptions with
try
andcatch
blocks
Advanced Concepts in C# Stack
For those gluttons for knowledge, we’ve got a final treat! Here are some advanced concepts related to the C# Stack. Ready to go down the rabbit hole?
Understanding Stack Frames
Stack Frames or Activation Records hold the parameters and local variables of a function call. It’s like each function call gets its personal little space on the Stack. Isn’t that neat?
Memory Allocation in C# Stack
The superb efficiency of a Stack is attributed to how it handles memory allocation. Every time a block is entered, the memory is allocated on the Stack, and when we’re done (as we exit the block), it automatically gets cleaned up. Consider it self-service!
C# Stack: Final Thoughts
Well, we’ve had some ride through the innards of C# Stack, haven’t we? And we’ve learnt some cool concepts, dodged several pitfalls and even faced our fears (hello StackOverflow Exception!). At the end of the day, remember, the better we understand the Stack, the better programmers we can become.
Time to get Stack-ing! Or should I say stacking those lines of code! (Did you see what I did there?) Keep exploring, keep coding, and most importantly, enjoy the ride! Remember, in programming, and in life – sky’s the limit! So, keep pushing those limits guys. Until next time… Happy coding!