Concatenating Strings in C#: Best Practices
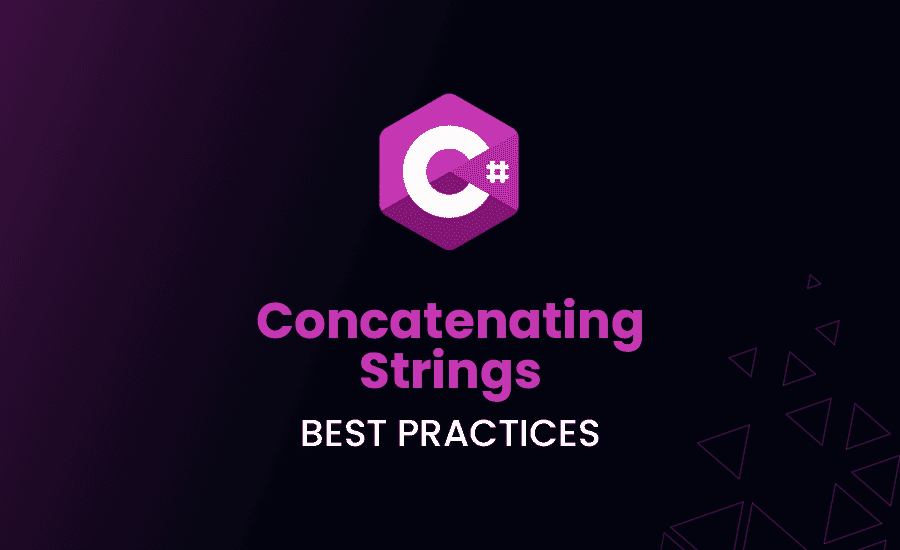
Do you ever line up your favorite bits of chocolate to form one big, delicious, super-chocolate? Is that just me? Alright, moving on from chocolates, programming is a lot like that. Particularly in C#, it’s about assembling smaller pieces to create something bigger and functional. Today, we’re going to focus on one specific process – string concatenation. Interesting, eh?
Understanding C# String Concatenation
Let’s kick- things-off by diving straight into the core concept. String concatenation is essentially the process of joining two or more strings together.
The Basics of C# String Concat
In C#, “String Concat” is almost like a superglue for your text. Want to join “Hello” and “World!” together? String concat has got you covered. Let’s look at a simple example:
Loading code snippet...
In the above snippet, we’ve utilized the String.Concat
method to join “Hello” and “World!” together. The result? HelloWorld! Smooth, isn’t it?
C# String Variable: What is it?
Still with me? Okay, then let’s explore something even more fundamental – C# String variables. A string in C# is an object of the String class. This ‘String’ class provides us with methods and properties to manipulate text by creating instances of the String class, popularly known as string variables, we can store text and manipulate it as desired.
Here’s a basic example:
Loading code snippet...
In the above code, ‘greet’ is a string variable holding the text “Hello World!”. So, when we write this string variable on the console, it outputs – you guessed it – “Hello World!”.
A Complete Guide on How to Concatenate Strings in C#
Could there be more ways to concatenate strings? Oh, totally. C# is conveniently packed with abundant methods for string concatenation. Let’s delve into some of these techniques.
How to use C# Append to String
Let’s say you have a string, and you want to add some text at the end. That’s where the Append
function steps in.
Loading code snippet...
In this example, we use the StringBuilder
class to append strings to an existing string. The Append
method is pretty versatile – it ensures efficient memory usage and faster performance when dealing with large string operations.
C# String Join: Merging Strings Efficiently
The String.Join
method is a favourite, and for decent reasons. It enables us to join strings, with the freedom to specify a delimiter (separator) that separates the strings. Yes, the power of choice is fantastic, isn’t it?
Loading code snippet...
Voila! With the above example, we’re joining words from the ‘words’ array using the String.Join
method and separating them by a space. After all, “HelloWorld!” does look a bit cramped, doesn’t it?
String.Join C#
String.Join
has a lot more tricks up its sleeves. Let’s venture deeper into understanding its prowess and handiness.
An Exploratory Approach to String.Join in C#
What if you’re dealing with a list of string objects – would String.Join
still work? Absolutely. With String.Join
, lists are as good as arrays!
Loading code snippet...
The above code joins a list of strings and separates them by a comma. Thus, versatility is the name of the game with String.Join
.
String Add C#: How It Works?
Now you’ve heard of string.Concat
, StringBuilder.Append
, and String.Join
. But how about an even simpler method for concatenation? The ‘+’ operator, that’s right. Interestingly, it works equally well for adding numbers and concatenating strings.
Loading code snippet...
In the code above, we’re using the ‘+’ operator to piece together three separate strings – “Hello”, a blank space, and “World!”.
String Append in C#
So, we’ve covered the StringBuilder.Append
method. But wouldn’t it be exhilarating to plunge deeper into String.Append
, wouldn’t it? You bet.
Understanding String Append Method
In C#, StringBuilder.Append
does not create a new string object. Instead, it modifies the existing StringBuilder instance by appending the specified string at the end of the current instance. Yes, it keeps it short and simple.
Loading code snippet...
The Append
method in StringBuilder class is quicker and more memory-efficient for larger amounts of string data. It’s like a film editor who efficiently edits movies without requiring another reel.
Examples of String Append C# Usage
But what if you want to append different types of data? Not a problem at all. Append
method accommodates all data types – be it a number, a Boolean or a char.
Loading code snippet...
In the provided example, number ‘6’ and Boolean ‘True’ have been seamlessly appended with other strings.
Best way to Concatenate Strings in C#
Now, let’s grab the bull by the horns – what’s the optimal way to concatenate strings? That’s the million-dollar question. So, let’s unravel it.
Techniques for Efficient String Concatenation
In the C# World, we have multiple ways to concatenate strings. But they fall into two main categories: compile-time concatenation methods such as +,-
and +=
operators, and runtime concatenation methods like String.Concat
, String.Join
, and StringBuilder.Append
. The important part here is determining when and how to use them efficiently.
+,-,+=
operators: Suitable for simple, small scale operations. But for larger operations, they can be quite inefficient and resource-taxing.String.Concat
: Works well with relatively small or medium-sized strings. Again, it can be inefficient for larger operations.StringBuilder.Append
: The most efficient when dealing with lengthy strings or repeated concatenations in loops.String.Join
: The best option for concatenating string arrays or lists with a common delimiter.
Remember, the trick here is to pick the right tool for the right job!
C# Prepend: Adding Value Before a String
Remember when I said concatenation is similar to lining up chocolates? Well, sometimes, you might want a particular chocolate (let’s say, the one with almonds) to be at the beginning of your super-chocolate line. Similarly, in C#, we use a process known as ‘Prepend’ to add a value or string before another string.
Understanding the Mechanism of C# Prepend
It’s pretty straightforward and similar to Append. The only difference is – instead of adding to the end of an existing string, prepend adds to the beginning.
Loading code snippet...
The code above demonstrates the Insert
method of StringBuilder
, which successfully prepends “Hello ” before “World!”. You just gotta love the attention to detail here.
C# String Content: Managing and Manipulating
C’mon, we both know that Strings are not just about concatenating or appending. It’s about managing, changing, and manipulating string content in several stimulating ways, just like how you’d manage your wardrobe to suit your style!
How to Manipulate C# String Content
C# offers several techniques to manipulate strings, such as trimming, padding, replacing, splitting, and so much more. Each technique serves a particular purpose and contributes to intensifying the dynamism of the language.
- Trimming: Remove spaces or specific characters from the start or end of a string.
- Padding: Add additional characters to the start or end of a string.
- Replacing: Replace a specific character or substring in a string.
- Splitting: Split a string into multiple substrings based on a specific delimiter.
- Formatting: Format a string to include variable values.
- Conversion: Convert a string’s case or convert a string to other data types.
- Searching: Search for a specific character, substring, or pattern in a string.
- Comparison: Compare two strings for equality or order.
Like wondering which shirt goes well with your pants, knowing which technique to use takes a bit of practice – but trust me, it’s more fun than it sounds!
Leveraging C# for Effective String Operations
We’re near the end, folks. We have unpacked a ton from the magical world of C# string concatenation. Let’s take a step back and discuss the real-world applications of these techniques and potential challenges you might encounter.
Real-world Applications of Concatenating Strings C#
From developing complex software systems to creating basic console applications, string manipulation and concatenation play a significant role in various real-world applications. Here are a few examples:
- Web Development: Concatenating URL segments, format HTML markup, or generate dynamic content in web pages.
- Mobile Apps: User input validation, strong format for display, and creating dynamic messages for user interaction.
- Game Development: Used to generate dynamic dialogue, create game status messages, or handle player’s input.
- Data Processing: Clean and format data for processing, parse text files, or extract useful information from raw data.
Potential Challenges and Solutions in String Operations
Despite C#’s impressive capabilities, you may encounter a few challenges where the language’s performance, efficiency, or style doesn’t meet your expectations. Just like your favorite superhero, these challenges make your coding journey more exciting.
- Performance: When dealing with large volume or complex string operations, performance can decrease significantly.
- Memory Usage: Extensive usage of certain string concatenation techniques can lead to increased memory usage.
- Readability: Complex concatenation operations might reduce code readability.
Remember, every challenge has a solution. For performance and memory usage, StringBuilder
can be your best friend. And for readability? Simple – use clearly named variables and breaking down complex operations into smaller, more manageable parts.
Conclusion
Phew, that was a lot, wasn’t it? But remember, mastery comes with practice – so get on with your C# journey! String concatenation in C# isn’t tricky. However, it’s all about picking the right technique for the task at hand, which brings us to the million-dollar question – wait for it – What’s your favorite chocol- err – string concatenation technique, and why? Discover your style and start creating your own super-chocolate! Or super string, if you will.
And what happens if you don’t start practicing these techniques? Well, you might be left behind in this fast-paced world of coding. So, what’s stopping you now? Roll up your sleeves and start creating magic with C#.