ASP.NET Web API Interview Questions for Experienced Professionals
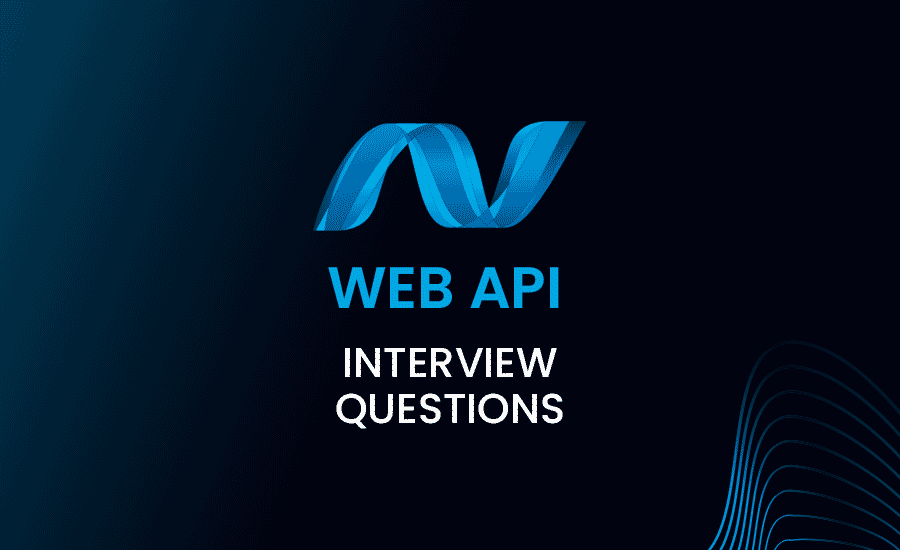
If you’re preparing for a job interview as an experienced ASP.NET Web API developer, you’ve come to the right place. In this comprehensive guide, we’ll cover the most challenging and advanced ASP.NET Web API interview questions and answers for experienced professionals.
From custom MediaTypeFormatters and OData implementation to API security and real-time communication, we’ve got you covered.
By reviewing these web API C# interview questions for experienced developers, you’ll be well-equipped to showcase your expertise and ace your next interview.
How do you implement custom content negotiation in ASP.NET Web API to support media type versioning based on custom request headers?
Answer
To implement custom content negotiation in ASP.NET Web API, you can create a custom IContentNegotiator
and override the Negotiate
method. This will allow you to inspect the request headers and select the appropriate media type formatter and version for the response.
Here’s a step-by-step guide:
- Create a custom
IContentNegotiator
implementation:
Loading code snippet...
- Register the custom content negotiator in the Web API configuration:
Loading code snippet...
- Define versioned media types in your custom MediaTypeFormatters:
Loading code snippet...
- Register the custom MediaTypeFormatters in the Web API configuration:
Loading code snippet...
Now, the custom content negotiator will inspect the X-Api-Version
header and select the appropriate formatter and version for the response.
In a situation where you need to support both OAuth2 and JWT authentication in the same Web API project, how would you configure the authentication pipeline to accommodate both?
Answer
To support both OAuth2 and JWT authentication in the same Web API project, you can configure the authentication pipeline to use multiple authentication options. In this case, you can use the OAuthAuthorizationServerMiddleware
for OAuth2 and JwtBearerAuthenticationMiddleware
for JWT authentication.
Here’s a step-by-step guide:
- Install the necessary NuGet packages:
- Microsoft.Owin.Security.OAuth
- Microsoft.Owin.Security.Jwt
- System.IdentityModel.Tokens.Jwt
- Configure the authentication options in the
Startup
class:
Loading code snippet...
Now, your Web API project will support both OAuth2 and JWT authentication. Clients can authenticate using either method, and the server will validate incoming tokens accordingly.
Describe the process of implementing exception handling and logging in a global, centralized way in ASP.NET Web API using custom exception filters and exception logger.
Answer
Implementing centralized exception handling and logging in ASP.NET Web API involves creating a custom exception filter and an exception logger. The custom exception filter can be used to handle exceptions and provide a consistent error response to the client, while the custom exception logger is responsible for logging exceptions.
Here’s a step-by-step guide:
- Create a custom exception filter by implementing
IExceptionFilter
:
Loading code snippet...
- Create a custom exception logger by implementing
ExceptionLogger
:
Loading code snippet...
- Register the custom exception filter and exception logger globally in the Web API configuration:
Loading code snippet...
Now, all unhandled exceptions in your Web API application will be caught by the custom exception filter, providing a consistent error response to clients, and logged by the custom exception logger.
How can you implement a custom throttling handler in ASP.NET Web API to limit the rate of incoming requests based on IP address or other criteria?
Answer
To implement a custom throttling handler in ASP.NET Web API, you can create a custom DelegatingHandler
that intercepts incoming requests and checks if the request should be allowed based on the specified throttling criteria, such as IP address or API key.
Here’s a step-by-step guide:
- Implement a custom
DelegatingHandler
for throttling:
Loading code snippet...
- Register the custom throttling handler in the Web API configuration:
Loading code snippet...
Now, the custom throttling handler will intercept incoming requests and enforce the specified rate limit based on the client IP address.
When implementing CORS in ASP.NET Web API, how can you handle preflight requests and manage complex CORS policies for different origins, methods, and headers?
Answer
To implement and manage CORS in ASP.NET Web API, you can use the built-in EnableCorsAttribute
to configure CORS policies for your controllers or actions. This attribute allows you to specify the allowed origins, methods, and headers in a flexible and granular manner.
Here’s a step-by-step guide:
- Install the
Microsoft.AspNet.WebApi.Cors
NuGet package. - Enable CORS in the Web API configuration:
Loading code snippet...
- Configure CORS policies using the
EnableCors
attribute on your controllers or actions:
Loading code snippet...
- To handle preflight requests, the
EnableCors
attribute will automatically respond to theOPTIONS
HTTP method with the appropriate CORS headers, such asAccess-Control-Allow-Origin
,Access-Control-Allow-Methods
, andAccess-Control-Allow-Headers
.
Now, your Web API application will handle CORS and preflight requests according to the specified policies. You can easily manage complex CORS policies by applying the EnableCors
attribute with different parameters on different controllers or actions.
Remember that you can also create custom CORS policies by implementing the ICorsPolicyProvider
interface and registering it in the Web API configuration.
This approach can provide even more control over CORS handling and preflight requests, especially in scenarios where CORS policies need to be determined dynamically or loaded from a configuration file.
Now that we’ve covered the first five advanced ASP.NET Web API interview questions and answers, let’s dive into some more complex topics.
As an experienced professional, you should be prepared to discuss various aspects of API versioning, caching strategies, and multi-tenancy. Stay tuned as we explore these critical concepts and more.
How do you implement a custom action selector in ASP.NET Web API that routes requests to different action methods based on specific request parameters or custom logic?
Answer
To implement a custom action selector in ASP.NET Web API, you can create a class that inherits from ApiControllerActionSelector
and override the SelectAction
method. In this method, you can apply custom logic to choose the appropriate action based on request parameters or other criteria.
Here’s a step-by-step guide:
- Create a custom action selector by inheriting from
ApiControllerActionSelector
:
Loading code snippet...
- Register the custom action selector in the Web API configuration:
Loading code snippet...
Now, the custom action selector will route requests to different action methods based on the specified custom logic. In this example, the action is chosen based on the X-Custom-Action
request header.
You can modify the SelectAction
method to implement your own logic for selecting the appropriate action based on request parameters or other criteria.
Describe the process of implementing a custom MediaTypeFormatter in ASP.NET Web API to support a custom serialization format or content type.
Answer
Implementing a custom MediaTypeFormatter
in ASP.NET Web API involves creating a class that inherits from MediaTypeFormatter
or one of its derived classes (e.g., JsonMediaTypeFormatter
, XmlMediaTypeFormatter
).
You need to override methods for reading and writing data to support a custom serialization format or content type.
Here’s a step-by-step guide:
- Create a custom
MediaTypeFormatter
class that inherits fromMediaTypeFormatter
or one of its derived classes:
Loading code snippet...
- Register the custom
MediaTypeFormatter
in the Web API configuration:
Loading code snippet...
Now, your Web API application will support the custom serialization format or content type for the specified types.
Clients can send and receive data using the custom format by specifying the appropriate Content-Type
and Accept
headers in their requests.
What are some potential issues with using asynchronous controllers in ASP.NET Web API, and how can you mitigate them to improve performance and avoid deadlocks?
Answer
Asynchronous controllers in ASP.NET Web API can improve the scalability and responsiveness of your application by freeing up the thread pool while waiting for I/O-bound operations to complete.
However, there are some potential issues when using asynchronous programming, such as performance overhead, complexity, and deadlocks. Here’s how to mitigate these issues:
Performance overhead: Asynchronous code can introduce some performance overhead due to the state machine generated by the C# compiler, and the additional allocations required for managing tasks. To mitigate this:
- Use asynchronous methods only for I/O-bound operations that would otherwise block the thread. Avoid using
Task.Run
to offload CPU-bound work, as this will not improve scalability and can lead to additional overhead. - Consider using ValueTask instead of Task for small, frequently called asynchronous methods to reduce allocations and improve performance.
Complexity: Asynchronous code can be more complex and harder to read, especially when dealing with error handling, cancellation, and continuations. To mitigate this:
- Use the
async
andawait
keywords to write asynchronous code that is more readable and easier to maintain. - Use
ConfigureAwait(false)
when awaiting tasks in libraries or middleware to avoid capturing the synchronization context, which can lead to deadlocks and other issues. In ASP.NET Web API, this is not strictly necessary, as it uses aSynchronizationContext
that does not cause deadlocks. However, it is still a good practice for libraries and middleware that might be used in other contexts.
- Handle exceptions consistently by using
try
/catch
blocks around awaited operations, and propagate exceptions as appropriate. - Use the CancellationToken mechanism to support cooperative cancellation of asynchronous operations, making your code more responsive and efficient.
Deadlocks: Deadlocks can occur when using asynchronous code if the synchronization context is captured and blocking calls are mixed with asynchronous calls. In ASP.NET Web API, the default synchronization context does not cause deadlocks, but it is still important to follow best practices to avoid potential issues:
- Always use
await
when calling asynchronous methods, and avoid using.Result
,.Wait()
, orTask.WaitAll()
as they can block the thread and cause deadlocks. - As mentioned earlier, use
ConfigureAwait(false)
when awaiting tasks in libraries or middleware, as this will avoid capturing the synchronization context and reduce the risk of deadlocks. - Be aware of potential issues when using third-party libraries that might not follow best practices for asynchronous programming. Make sure to use well-maintained libraries and follow their recommendations for using their APIs asynchronously.
By following these best practices and mitigating the potential issues associated with asynchronous programming, you can improve the performance, scalability, and responsiveness of your ASP.NET Web API application while avoiding deadlocks and other pitfalls.
How can you design a versioning strategy for your ASP.NET Web API, considering the pros and cons of different approaches like URL versioning, query string versioning, and custom media types?
Answer
Designing a versioning strategy for your ASP.NET Web API involves considering the trade-offs of different approaches, including URL versioning, query string versioning, and custom media types (using the “Accept” header).
Here are the pros and cons of each approach, along with implementation guidelines:
URL Versioning: In this approach, the API version is included as part of the URL, typically in the form of a path segment (e.g., /api/v1/users
).
- Pros:
- Cons:
- Implementation: Use attribute routing and include the version number in the route template.
Query String Versioning: In this approach, the API version is passed as a query string parameter (e.g., /api/users?version=1
).
- Pros:
- Cons:
- Implementation: Create a custom
IHttpRouteConstraint
to match the version query string parameter and use it in the route configuration.
Custom Media Types (Accept Header Versioning): In this approach, the API version is specified in the “Accept” header using a custom media type (e.g., application/vnd.myapi.v1+json
).
- Pros:
- Cons:
- Implementation: Create a custom
MediaTypeFormatter
for each version and associate it with the corresponding custom media type.
When designing a versioning strategy, consider the pros and cons of each approach based on your specific use case and requirements. In many cases, URL versioning is a good choice due to its simplicity and clarity for clients.
However, if adhering to REST principles and supporting content negotiation are important requirements, custom media type versioning may be more suitable. Query string versioning can be an option if you prefer a more flexible and less visible versioning mechanism.
You can also combine multiple approaches if needed to accommodate different client needs and preferences.
How would you implement server-side caching in ASP.NET Web API to improve performance, and what are the best practices for cache invalidation and cache management?
Answer
Implementing server-side caching in ASP.NET Web API can help improve performance by reducing the need for time-consuming operations, such as database queries or complex calculations.
Here’s a step-by-step guide to implementing caching, along with best practices for cache invalidation and management:
Choose a caching mechanism: ASP.NET provides several caching mechanisms, such as in-memory caching (MemoryCache
), distributed caching (using Redis, SQL Server, or other providers), and output caching. Select a caching mechanism based on your requirements and infrastructure.
Cache data: Store the results of time-consuming operations in the cache, and retrieve them when needed. For example, use the MemoryCache
class to store and retrieve data:
Loading code snippet...
Set cache policies: Use appropriate cache policies to control the cache’s behavior, such as expiration, sliding expiration, and cache dependencies. In the example above, an AbsoluteExpiration
policy is used, which expires the cache after 1 hour.
Cache invalidation: Invalidate the cache when the underlying data changes to ensure clients receive up-to-date information. For example, remove a product from the cache when it’s updated:
Loading code snippet...
Best practices for cache management:
- Use appropriate cache granularity: Avoid caching large datasets or entire responses, as this may lead to memory pressure and slow down the application. Instead, cache smaller, more focused pieces of data or partial responses.
- Consider cache eviction policies: Use policies like Least Recently Used (LRU) or Least Frequently Used (LFU) to efficiently manage cache memory and evict less important data when needed.
- Leverage cache-control headers: Use
Cache-Control
andETag
headers to control client-side caching and enable conditional requests, which can further reduce the load on your server. - Monitor cache performance: Use performance counters or other monitoring tools to track cache hit rates, cache sizes, and eviction rates to ensure your caching strategy is effective.
By implementing server-side caching in your ASP.NET Web API application and following best practices for cache invalidation and management, you can significantly improve the performance and responsiveness of your API while reducing the load on your server and database.
We’ve reached the halfway point in our list of essential web API interview questions and answers for experienced C# developers. As you continue to read, you’ll find even more in-depth questions to help you showcase your expertise.
From implementing advanced authentication and authorization scenarios to working with OData, we’re here to ensure you’re ready to tackle any challenge in your upcoming interview.
In a multi-tenant Web API application, how would you handle tenant-specific configurations, such as connection strings or API keys, to ensure proper isolation and security?
Answer
In a multi-tenant Web API application, it is crucial to handle tenant-specific configurations properly to ensure isolation and security.
Here are some strategies and best practices for managing tenant-specific configurations:
- Tenant identification: First, establish a mechanism to identify the tenant for each incoming request. You can use the subdomain, a custom header, or a JWT claim to determine the tenant. Create a middleware or a message handler to extract the tenant information from the request and store it in the request context or a scoped service for later use.
- Tenant-specific configuration storage: Store tenant-specific configurations, such as connection strings or API keys, in a secure and isolated manner. Use a separate database, table, or configuration store for each tenant, or use a shared store with proper tenant identifiers. Make sure to encrypt sensitive data and use proper access controls to ensure security.
- Tenant-aware services and components: Design your services, components, and data access layers to be tenant-aware. Inject tenant-specific configurations into these components as needed. For example, create a factory to provide tenant-specific
DbContext
instances with the appropriate connection strings:
Loading code snippet...
- Tenant-scoped services: Register tenant-specific services as scoped services in the dependency injection container. This ensures that each tenant’s instance of the service is isolated and uses the correct configuration. For example:
Loading code snippet...
- Auditing and logging: Implement proper auditing and logging mechanisms to track tenant-specific activity and errors. This can help identify potential security issues and ensure the correct operation of your multi-tenant Web API application.
By following these strategies and best practices, you can handle tenant-specific configurations securely and effectively in your multi-tenant Web API application, ensuring proper isolation and security for each tenant’s data and resources.
How can you secure sensitive data in transit between an ASP.NET Web API and a client, using techniques like encryption or digital signatures?
Answer
Securing sensitive data in transit is essential to protect your application and users from unauthorized access, tampering, or interception.
Here are some techniques and best practices for securing data in transit between an ASP.NET Web API and a client:
Transport Layer Security (TLS): Use TLS (formerly known as SSL) to encrypt the communication channel between the client and the server. This provides confidentiality, integrity, and authenticity for the data in transit.To enable TLS:
- Obtain an SSL/TLS certificate from a trusted certificate authority (CA).
- Configure your web server (e.g., IIS, Kestrel) to use the certificate and enable HTTPS.
- Enforce HTTPS by using the
[RequireHttps]
attribute, HSTS (HTTP Strict Transport Security), or URL rewriting rules in your ASP.NET Web API application.
Message-level encryption: In some cases, you may need additional encryption at the message level to protect sensitive data. This can be achieved using symmetric or asymmetric encryption algorithms, such as AES or RSA. Here’s a high-level overview of the process:
- Encrypt sensitive data before sending it to the client or the server.
- Transmit the encrypted data over a secure channel (e.g., HTTPS).
- Decrypt the data on the receiving end using the appropriate decryption key.
Digital signatures: Use digital signatures to ensure data integrity and authenticity. This can help detect tampering or forgery during transit. The process generally involves:
- Creating a hash of the data using a cryptographic hash function (e.g., SHA-256).
- Encrypting the hash using the sender’s private key (for asymmetric algorithms like RSA) or a shared secret key (for symmetric algorithms like HMAC).
- Sending the encrypted hash (i.e., the signature) along with the data.
- On the receiving end, creating a new hash of the received data and decrypting the signature using the sender’s public key (for RSA) or the shared secret key (for HMAC).
- Comparing the new hash and the decrypted signature to verify the data’s integrity and authenticity.
Authentication and Authorization: Ensure that only authorized clients can access your ASP.NET Web API by implementing proper authentication and authorization mechanisms, such as JWT tokens, OAuth2, or API keys. This helps protect sensitive data from unauthorized access and reduces the risk of data leaks or breaches.
By using these techniques and best practices, you can secure sensitive data in transit between your ASP.NET Web API and clients, protecting your application and users from unauthorized access, tampering, or interception.
How would you implement advanced authentication and authorization scenarios in ASP.NET Web API, such as role-based access control, claims-based authorization, or custom policies?
Answer
Implementing advanced authentication and authorization scenarios in ASP.NET Web API helps protect your application’s resources and ensure that only authorized clients can access them.
Here’s how you can implement role-based access control, claims-based authorization, and custom policies:
Role-based access control (RBAC): RBAC is a common approach for controlling access to resources based on the roles assigned to users. To implement RBAC in ASP.NET Web API:
- Set up an authentication mechanism to authenticate users and provide role information, such as using JWT tokens with role claims, OAuth2, or ASP.NET Identity.
- Use the
[Authorize(Roles = "RoleName")]
attribute on controllers or actions to restrict access based on user roles. For example:
Loading code snippet...
Claims-based authorization: Claims-based authorization uses claims associated with a user to determine their access rights. Claims are key-value pairs that represent user attributes or actions they are allowed to perform. To implement claims-based authorization:
- Set up an authentication mechanism that provides claims for users, such as JWT tokens with custom claims or ASP.NET Identity with claim-based roles.
- Use the
[Authorize]
attribute with custom policies that check for the required claims. For example, create a policy that requires a specific claim:
Loading code snippet...
- Apply the policy to controllers or actions using the
[Authorize]
attribute:
Loading code snippet...
- Custom policies: In some cases, you may need more complex authorization logic that goes beyond roles and claims. In this case, you can create custom policies using the
IAuthorizationRequirement
andAuthorizationHandler
classes:
Loading code snippet...
- Create a custom
AuthorizationHandler
class to implement the authorization logic:
Loading code snippet...
- Register the custom handler in the
Startup
class:
Loading code snippet...
- Apply the custom policy to controllers or actions using the
[Authorize]
attribute:
Loading code snippet...
By implementing these advanced authentication and authorization scenarios in your ASP.NET Web API, you can provide granular access control and better protect your application’s resources, ensuring that only authorized clients can access them.
Role-based access control, claims-based authorization, and custom policies offer various levels of flexibility and complexity, allowing you to adapt your authorization strategy to the specific needs of your application.
Remember to follow security best practices when implementing these scenarios, such as:
- Enforcing HTTPS to protect your authentication tokens and authorization headers from being intercepted or tampered with.
- Storing sensitive data, such as API keys or secrets, securely in a protected configuration store or a secret manager like Azure Key Vault.
- Regularly reviewing and updating your authorization policies to ensure that they continue to meet your application’s requirements and security standards.
How can you use OData in ASP.NET Web API to enable advanced query capabilities, and what are the best practices for handling performance and security concerns?
Answer
OData (Open Data Protocol) is a standard protocol for querying and updating data over the web. It allows clients to perform advanced queries, such as filtering, sorting, and paging, using a standardized syntax.
To enable OData in your ASP.NET Web API application, follow these steps:
Install the OData NuGet package: Install the Microsoft.AspNet.OData
package using the Package Manager Console or the NuGet Package Manager in Visual Studio.
Loading code snippet...
Configure OData: In the Startup
or WebApiConfig
class, enable and configure OData using the AddOData
and MapODataRoute
extension methods.
Loading code snippet...
Create OData controllers: Create OData controllers that derive from ODataController
and use the [EnableQuery]
attribute to enable OData query options for specific actions.
Loading code snippet...
Now that you have OData enabled in your ASP.NET Web API application, it’s important to consider performance and security best practices:
Performance considerations:
- Limit query options: Use the OData configuration options (e.g.,
config.Count().Filter().OrderBy().Expand().Select().MaxTop(null)
) to limit the query capabilities exposed by your API. This helps prevent overly complex or expensive queries. - Set maximum page size: Use the
MaxTop
option to set a maximum page size for OData queries, preventing clients from requesting an excessive amount of data in a single request. - Use server-driven paging: Enable server-driven paging using the
PageSize
property of the[EnableQuery]
attribute. This enforces a consistent paging strategy and reduces the risk of large query results causing performance issues. - Optimize database queries: Monitor the database queries generated by OData and optimize them if necessary. Use tools like Entity Framework Profiler or SQL Server Profiler to analyze and improve query performance.
Security considerations:
- Validate query options: Use the
[EnableQuery]
attribute’s properties, such asAllowedOrderByProperties
andAllowedLogicalOperators
, to restrict and validate the query options allowed by your API. - Prevent information disclosure: Be cautious when exposing sensitive data through OData. Use the
IgnoreDataMember
attribute or the OData model builder’sIgnore
method to exclude sensitive properties from the OData model. - Authorize access: Implement proper authentication and authorization mechanisms, such as JWT tokens, OAuth2, or API keys, to ensure that only authorized clients can access your OData endpoints.
By following these best practices and guidelines, you can effectively use OData in your ASP.NET Web API application to enable advanced query capabilities while addressing performance and security concerns. This will help you provide a powerful and flexible API for your clients while maintaining a secure and performant application.
Additionally, consider these best practices when working with OData in ASP.NET Web API:
- Versioning: Implement a versioning strategy for your OData API to maintain backward compatibility as your API evolves. Consider using URL versioning, query string versioning, or custom media types to achieve this.
- Caching: Implement server-side caching for OData query results to improve performance and reduce the load on your database. Be mindful of cache invalidation and management strategies to ensure that your clients receive up-to-date data.
- Monitoring and diagnostics: Regularly monitor your OData API’s performance, usage, and error rates to identify potential bottlenecks, security issues, or areas for improvement. Use tools like Application Insights, ELK Stack, or custom logging solutions to collect and analyze this data.
By following these best practices and recommendations, you can create a robust and efficient OData-enabled ASP.NET Web API that offers advanced query capabilities to your clients while addressing performance and security concerns. This will help you build a scalable, secure, and high-performing web API that meets the needs of your application and its users.
When working with real-time data in ASP.NET Web API, how can you implement WebSockets or SignalR to enable efficient, low-latency communication between the server and clients?
Answer
WebSockets and SignalR are technologies that enable real-time communication between the server and clients, providing efficient and low-latency data exchange. They are particularly useful when working with real-time data in your ASP.NET Web API application.
WebSockets
WebSockets is a protocol that enables bidirectional communication between a client and a server over a single, long-lived connection. To implement WebSockets in ASP.NET Web API:
- Install the WebSockets NuGet package: Install the
Microsoft.WebSockets
package using the Package Manager Console or the NuGet Package Manager in Visual Studio.
Loading code snippet...
- Create a WebSocket handler: Implement a custom
WebSocketHandler
class to handle the WebSocket communication.
Loading code snippet...
- Register the WebSocket handler: In the
Startup
orWebApiConfig
class, register the custom WebSocket handler using theMapWebSocketRoute
extension method.
Loading code snippet...
- Client-side communication: Implement client-side WebSocket communication using JavaScript or a WebSocket client library.
SignalR
SignalR is a library for ASP.NET applications that simplifies the process of adding real-time functionality, such as chat or live updates, to your application. It automatically chooses the best transport method (e.g., WebSockets, Server-Sent Events, or long polling) based on the client’s capabilities. To implement SignalR in ASP.NET Web API:
- Install the SignalR NuGet package: Install the
Microsoft.AspNet.SignalR
package using the Package Manager Console or the NuGet Package Manager in Visual Studio.
Loading code snippet...
- Create a SignalR hub: Implement a custom
Hub
class to handle the real-time communication.
Loading code snippet...
- Configure SignalR: In the
Startup
orWebApiConfig
class, enable and configure SignalR using theMapSignalR
extension method.
Loading code snippet...
- Client-side communication: Implement client-side SignalR communication using the SignalR JavaScript library or a SignalR client library for other platforms.
By implementing either WebSockets or SignalR in your ASP.NET Web API application, you can enable efficient, low-latency communication between the server and clients, providing real-time data updates and interaction.
This can greatly improve the user experience and responsiveness of your application, especially when dealing with real-time data and scenarios.
In addition to implementing WebSockets or SignalR, consider the following best practices to ensure a reliable and scalable real-time communication solution:
Performance considerations:
- Scale out: When deploying your application in a multi-server environment, use a backplane like Redis, SQL Server, or Azure Service Bus to synchronize real-time data across instances.
- Optimize client-side updates: Be mindful of the performance impact of updating the user interface frequently. Use techniques like debouncing, throttling, or batching updates to reduce the load on the client.
- Manage connection lifecycles: Close WebSocket or SignalR connections when they are no longer needed to free up server resources.
Security considerations:
- Authenticate and authorize: Implement proper authentication and authorization mechanisms, such as JWT tokens or OAuth2, to ensure that only authorized clients can access your real-time endpoints.
- Validate and sanitize input: Always validate and sanitize any data received from clients before processing it or sending it to other clients to prevent security vulnerabilities like cross-site scripting (XSS) or SQL injection.
- Use HTTPS: Enforce HTTPS for your real-time communication to protect data in transit from being intercepted or tampered with.
Monitoring and diagnostics:
- Monitor performance and usage: Regularly monitor the performance, usage, and error rates of your real-time communication to identify potential bottlenecks, security issues, or areas for improvement.
- Log and trace: Use logging and tracing tools to collect and analyze diagnostic data, helping you identify and troubleshoot issues in your real-time communication implementation.
By following these best practices and recommendations, you can create a reliable, secure, and high-performing real-time communication solution in your ASP.NET Web API application using WebSockets or SignalR.
This will enable efficient, low-latency communication between the server and clients, improving the user experience and responsiveness of your application when working with real-time data.
As we approach the end of our comprehensive collection of web API interview questions for experienced C# professionals, we’ll delve into topics such as WebSocket and SignalR implementation, custom routing conventions, and handling large file uploads or downloads.
These questions will further test your knowledge and experience in ASP.NET Web API, preparing you for even the most demanding interview situations.
How can you optimize the performance of an ASP.NET Web API application by implementing response compression, and what are the potential trade-offs and pitfalls?
Answer
Response compression is a technique that reduces the size of the HTTP response sent from the server to the client, leading to faster transmission times and reduced bandwidth usage.
In an ASP.NET Web API application, you can implement response compression using middleware like the built-in Gzip compression or third-party libraries. Here’s how to enable response compression:
Gzip Compression
- Install the compression NuGet package: Install the
Microsoft.AspNet.WebApi.Extensions.Compression.Server
package using the Package Manager Console or the NuGet Package Manager in Visual Studio.
Loading code snippet...
- Configure compression: In the
Startup
orWebApiConfig
class, configure Gzip compression by adding theGzipCompressionHandler
to theHttpConfiguration
instance’s message handlers.
Loading code snippet...
Potential trade-offs and pitfalls
While response compression can improve the performance of your ASP.NET Web API application, there are some potential trade-offs and pitfalls to consider:
- Increased server CPU usage: Compression algorithms consume CPU resources on the server. For high-traffic applications or those with limited CPU resources, this can become a bottleneck. Monitor server CPU usage and consider adjusting the compression level or using a load balancer to distribute the load across multiple instances.
- Compression and encryption: When using HTTPS, some compression techniques can make your application vulnerable to security attacks, such as the BREACH attack. To mitigate this risk, consider using HTTPS with dynamic compression only for non-sensitive data or using a secure variant of compression, like Brotli.
- Vary header: Ensure that your application sets the correct
Vary
header in the HTTP response to inform caching proxies that the response may vary based on theAccept-Encoding
request header. This helps avoid issues where a compressed response is sent to a client that does not support the specific compression algorithm. - Selective compression: Not all content types benefit equally from compression. For example, text-based formats like JSON, XML, or HTML are highly compressible, while binary formats like images or videos are typically already compressed. Consider applying compression selectively to content types that will benefit the most.
- Client support: Some older clients may not support certain compression algorithms or may have limited support for them. Ensure that your application properly negotiates the compression algorithm to use based on the
Accept-Encoding
header sent by the client.
By implementing response compression in your ASP.NET Web API application and considering the potential trade-offs and pitfalls, you can optimize its performance and reduce the bandwidth usage for both your server and clients.
This can lead to faster response times and a better user experience, particularly for users on slow or metered internet connections.
How would you implement a custom routing convention in ASP.NET Web API to support non-standard RESTful API patterns or legacy URL structures?
Answer
In ASP.NET Web API, routing conventions define how URLs are mapped to controller actions. By default, the framework follows a RESTful convention, but you may need to support non-standard patterns or legacy URL structures.
In such cases, you can create a custom routing convention by implementing the IHttpRouteConstraint
interface or by using attribute routing.
Implementing a custom IHttpRouteConstraint
- Create a custom route constraint: Implement a class that inherits from
IHttpRouteConstraint
. In theMatch
method, apply your custom routing logic.
Loading code snippet...
- Register the custom route constraint: In the
WebApiConfig
class, create a new route with your custom route constraint.
Loading code snippet...
Using attribute routing
- Enable attribute routing: In the
WebApiConfig
class, enable attribute routing by calling theMapHttpAttributeRoutes
method.
Loading code snippet...
- Apply custom route attributes: In your controllers, use the
Route
attribute to define custom URL patterns for specific actions.
Loading code snippet...
By using either a custom IHttpRouteConstraint
or attribute routing, you can implement custom routing conventions in your ASP.NET Web API application to support non-standard RESTful API patterns or legacy URL structures.
This allows you to maintain compatibility with existing clients or support specific use cases that deviate from the default RESTful routing convention.
When implementing custom routing conventions, keep the following best practices in mind:
- Consistency: Ensure that your custom routing conventions are consistent across your entire API, making it easier for developers to understand and use your API.
- Documentation: Clearly document your custom routing conventions so that developers using your API are aware of any non-standard patterns or requirements.
- Versioning: When making changes to your routing conventions, consider implementing API versioning to avoid breaking existing clients.
What are the best practices for handling large file uploads or downloads in ASP.NET Web API, and how can you implement streaming or chunked transfer to improve performance and reduce memory usage?
Answer
Handling large file uploads and downloads in ASP.NET Web API can be challenging, particularly when it comes to performance and memory usage. By implementing streaming or chunked transfer, you can improve performance and reduce memory usage in your application.
Here are some best practices for handling large file uploads and downloads:
Large File Downloads
- Use IHttpActionResult: Implement a custom IHttpActionResult to return a
Stream
for the file content.
Loading code snippet...
- Return a FileStreamResult from your controller:
Loading code snippet...
Large File Uploads
- Use MultipartFormDataStreamProvider: To handle large file uploads, use the
MultipartFormDataStreamProvider
class to stream the file directly to disk or a remote storage service.
Loading code snippet...
Best Practices
- Use streaming: Both for uploads and downloads, use streaming to avoid loading the entire file into memory. This reduces memory usage and allows handling of much larger files.
- Use asynchronous methods: Use async/await pattern to ensure that your application remains responsive and can scale to handle multiple concurrent requests.
- Validate file size and type: For security and performance reasons, validate the file size and content type before processing the file. Limit the accepted file types and sizes to reduce the risk of attacks or excessive resource consumption.
- Use chunked transfer: For large downloads, consider using chunked transfer encoding to send the file in smaller chunks. This can improve download performance and reduce the impact on server resources.
- Implement resumable uploads: For large file uploads, consider implementing a resumable upload mechanism that allows clients to upload a file in smaller parts. This enables clients to resume uploads in case of network interruptions and reduces the impact of timeouts or connection issues.
By following these best practices for handling large file uploads and downloads in ASP.NET Web API, you can ensure that your application performs well and uses resources efficiently.
Streaming and chunked transfer techniques can help improve performance, reduce memory usage, and provide a better experience for users, especially when dealing with large files or slow, unreliable network connections.
How can you create a custom action selector in ASP.NET Web API to support custom logic for selecting the appropriate action method based on the request?
Answer
An action selector in ASP.NET Web API is responsible for selecting the appropriate action method to handle an incoming request.
By creating a custom action selector, you can implement custom logic to determine the most suitable action method based on the request’s properties, such as HTTP method, headers, or query parameters.
To create a custom action selector, follow these steps:
- Create a custom action selector class: Derive a class from
ApiControllerActionSelector
and override theSelectAction
method to implement your custom logic.
Loading code snippet...
- Register the custom action selector: In the
WebApiConfig
class, replace the default action selector with your custom action selector.
Loading code snippet...
By creating a custom action selector in your ASP.NET Web API application, you can implement custom logic for selecting the appropriate action method based on the request’s properties.
This allows you to handle complex scenarios or support non-standard routing conventions that may not be covered by the default action selector.
When implementing a custom action selector, ensure that your logic is efficient and maintainable. Consider using a combination of custom action selectors, attribute routing, and custom route constraints to build a flexible and easy-to-understand routing system for your application.
How can you implement API documentation and testing tools, such as Swagger or Postman, with ASP.NET Web API, and what are the best practices for maintaining up-to-date documentation throughout the API lifecycle?
Answer
Implementing API documentation and testing tools like Swagger or Postman in your ASP.NET Web API application can help you maintain up-to-date documentation and facilitate testing during the API lifecycle.
These tools also make it easier for developers to explore and interact with your API.
Implementing Swagger with ASP.NET Web API
- Install Swashbuckle: Install the Swashbuckle NuGet package, which adds Swagger support to your ASP.NET Web API project.
Loading code snippet...
- Configure Swagger: In the
App_Start
folder, locate theSwaggerConfig.cs
file created by Swashbuckle. Customize the settings in this file to configure the Swagger UI and API documentation generation.
Loading code snippet...
- Enable XML comments: In your project properties, go to the Build tab and check the “XML documentation file” option. This will generate an XML file containing the comments from your code, which Swashbuckle will use to generate the API documentation.
- Add XML comments: Add XML comments to your controllers and action methods to provide descriptions and examples for the Swagger UI.
Loading code snippet...
- Access the Swagger UI: Run your application and navigate to
/swagger
to access the Swagger UI.
Using Postman with ASP.NET Web API
- Export API as OpenAPI: Use Swashbuckle to generate an OpenAPI (formerly known as Swagger) JSON file for your API. This can be done by accessing the
/swagger/docs/v1
endpoint in your application. - Import OpenAPI in Postman: In Postman, click on the “Import” button and select the “Raw Text” tab. Paste the OpenAPI JSON content and click on “Import”. Postman will create a collection with all your API endpoints and their descriptions.
Best Practices for API Documentation
- Keep comments up-to-date: Ensure that your XML comments are updated whenever you make changes to your API. This will keep your documentation accurate and useful.
- Be descriptive: Write clear and concise descriptions for your endpoints, parameters, and return values. This will help developers understand the purpose and behavior of your API.
- Include examples: Whenever possible, include examples in your comments to demonstrate the expected input and output for your API endpoints.
- Use versioning: Implement API versioning to maintain separate documentation for different versions of your API. This will help developers understand the differences between versions and avoid breaking changes.
- Automate documentation generation: Integrate documentation generation into your build and deployment process to ensure that your documentation is always up-to-date and available.
By implementing tools like Swagger and Postman in your ASP.NET Web API application, you can maintain up-to-date documentation and facilitate testing throughout the API lifecycle.
These tools help developers explore, understand, and interact with your API more easily, improving developer experience and making it easier to integrate with your API.
Following best practices for API documentation will ensure that your documentation remains accurate, useful, and easy to understand, ultimately leading to a more successful API adoption and usage.
Conclusion
We hope this extensive list of ASP.NET Web API interview questions and answers for experienced developers has been helpful in preparing you for your next interview.
By mastering these web API interview questions and answers for experienced in C# and .NET, you’ll be well on your way to proving your expertise and landing that dream job. Remember, practice makes perfect, so don’t hesitate to review these questions and refine your answers.
Good luck on your interview, and may you find success in your professional journey!