ArrayList in C#: What You Need To Know
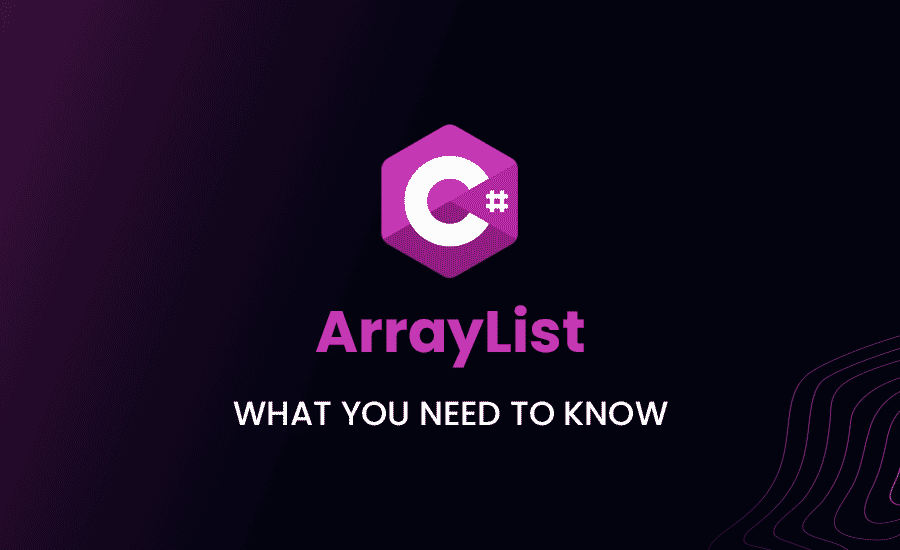
Introduction to ArrayList in C#
Oh code warrior! Ever tangled with the mighty ArrayList in C#, or is it an unexplored terrain for you? Fear not, for in the sections that follow, we shall conquer this beast together. We’ll fathom what exactly an ArrayList
is in C#, deconstruct the pros and cons of using it, and fuel your curiosity bit by bit.
What is ArrayList in C#
Picture this – you’re trying to juggle multiple data items, but alas, you don’t quite know how many. Enter ArrayList
. In the world of C#, an ArrayList
is a non-generic type of collection, meaning it can hold any type of data you throw at it. How convenient is that!
Here’s a simple example to kick us off:
Loading code snippet...
Advantages and Disadvantages of using ArrayList in C#
Having an ArrayList in your arsenal is like having a Swiss army knife – versatile and dynamic. But like every sword, it has two edges. Before we delve deeper, let’s summarise our main pros and cons:
Pros:
- Dynamic size: An ArrayList can dynamically resize itself as required.
- Versatility: It can hold elements of different data types.
Cons:
- Less efficient: Due to its dynamic nature and versatility, ArrayList can be less efficient than an array.
- Type Safety: This might be its downfall in certain scenarios where specific data type is important.
ArrayList Syntax in C#
Alright brave explorer, let’s crack the code (or rather, write it). We’ll examine how to create, declare, access, modify and delete elements in an ArrayList. Keep a keen eye, we have mysteries to unravel!
How to Create and Declare ArrayList in C#
An ArrayList is declared and created in much the same way as other objects in C#. Don’t believe me? Check this out:
Loading code snippet...
Accessing, Modifying and Deleting Elements
C#’s ArrayList intrinsically provides methods to access and manipulate its elements. Fetching an element or changing it – these are no longer Herculean tasks!
Here’s an example:
Loading code snippet...
Understanding the Difference Between Array and ArrayList in C#
Ever wondered in the wild landscapes of coding, what sets an Array and an ArrayList apart? Buckle up, for we’ll unfold this mystery next, our adventure is far from over.
Defining Arrays in C#
In the heartland of C#, an array is a systematic arrangement of similar elements, created with a specific size in mind. Here’s a simple illustration to bring the point home:
Loading code snippet...
Defining ArrayList in C#
We’ve already journeyed through ArrayList territory. Just to jog your memory, an ArrayList
is a dynamic collection that can store many cheers in its own kind of data party.
Loading code snippet...
Difference Between Array and ArrayList in C# with Example
So what differentiates an Array from an ArrayList or vice versa? That’s right – it’s the ability to dynamically resize and support multiple data types that sets ArrayList
apart. Ready to go deeper into the trenches? Let’s compare apples to apples, or rather, Arrays
to ArrayLists
.
Loading code snippet...
Reflecting on these examples, are you beginning to grasp the dynamic potential and the versatility offered by ArrayLists?
Array vs ArrayList in C#
Armed with clarity on the fundamental difference between Array and ArrayList, let’s do a performance check. After all, knowing which one to pull from your toolbox when, is the mark of an experienced code slinger.
Performance Comparison: Array vs ArrayList in C#
You might be thinking “Surely, with all its dynamic sizing and versatility, ArrayList must be more efficient than Array, right?” Well, not quite. See, arrays are simpler and more efficient when it comes to fetching and updating data due to their static nature and explicit type definition. So, it really depends on the scenario.
Use Cases: When to use Array and when to use ArrayList
Let’s break it down into a simple rule of thumb:
- Use Arrays when:
- The number of elements is known.
- The data type of elements is explicit and uniform.
- Use ArrayLists when:
- The number of elements is dynamic.
- Flexibility to store elements of different data types is needed.
Understanding Linked List and ArrayList in C#
Having wrestled Arrays and ArrayLists, let’s move on to another interesting topic: LinkedLists. Are LinkedLists just a chain of ArrayLists, or is it something more intriguing? Let’s find out.
What is LinkedList in C#
LinkedLists in C# are a generic type of collection, meaning they are designed to hold specific type of data. They are represented as a group of nodes in a sequence, each linked to the next via a reference pointer.
Here is a basic example:
Loading code snippet...
Difference Between ArrayList and LinkedList in C#
ArrayLists and LinkedLists, despite sounding similar, have considerable differences. While ArrayLists provide dynamic and flexible storage, LinkedLists provide efficient addition and removal of elements, particularly in large collections.
This boils down to a fundamental principle: ArrayLists are backed by arrays, while LinkedLists are built with individual nodes linked to each other.
Working with Strings in ArrayList
String operations in an ArrayList – sounds like a mouthful, doesn’t it? But let’s not get intimidated. Let’s take the bull by the horns, or rather, take the ArrayList by the strings.
C# check if one string array contains another
Wondering how this would work? Behold the power of C#:
Loading code snippet...
This checks if the ArrayList
contains any string from the words
array. By the end of it you’ll be like “Aha, I’ve nailed this!”.
Convert DataTable to ArrayList in C#
Having ventured into the vast kingdom of ArrayLists, traversed through the valleys of Arrays and LinkedLists, and danced with strings, let us approach our final destination: converting DataTable to ArrayList.
Basic Conversion Syntax
Through the might of C#, we can achieve this feat with elegance and ease. Here’s proof:
Loading code snippet...
This nifty piece of code will convert each row in the DataTable into an ArrayList.
Use Cases
This DataTable-to-ArrayList conversion can come in handy for processing rows from a DataTable, especially when iterating through rows and performing more complex operations.
Whew! That was indeed a long but interesting journey, wasn’t it? But hey, by the end of it, you’ve learned so much about ArrayLists, Arrays, LinkedLists, how to interact with strings and even converting DataTable to ArrayList. You’re pretty much an ArrayList wizard now. Time to go forth and conjure some fascinating programs.
But remember, as with all tools, understanding when and how to use them is key. Do not be a hammer looking for nails. Instead, let your understanding guide your choice of tool. Happy coding!