Array Instantiation in C#: Guide with Examples
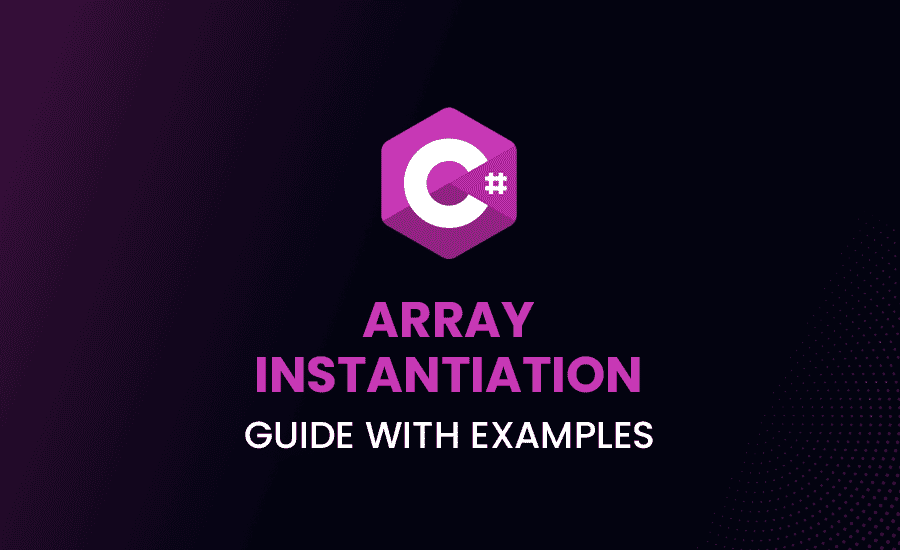
Have you ever been perplexed while dealing with array instantiation in C#? Don’t worry. By the end of this comprehensive guide, you will not only comprehend the fundamentals of array instantiation but also become proficient enough to implement it in real-world programming scenarios.
Understanding C# Array Instantiation
To master array instantiation in C#, we must first understand the gist of arrays. Arrays form a critical part of any programming language, acting as crucial data structures.
What is an Array and How is it Used in C#?
An array in C# can be defined as a collection of variables that hold values sharing the same data type. It simplifies data manipulation as it allows you to access a group of related data using a single variable, making your code leaner and cleaner. Do you see what we did there? Leaner and Cleaner, gotta love those cute programming rhymes!
Loading code snippet...
Here we are declaring an array of integers in C#. Simple right? But there’s so much more to it!
Basics of Array Instantiation C#
In C#, the new
keyword is used to instantiate (create an instance of) an array. Let’s break down this process.
Loading code snippet...
In the above example, we defined an ‘int’ array using the keyword new
followed by the data type and size within square brackets.
Exploring the Different Ways to Instantiate an Array in C#
There are numerous ways to instantiate an array in C#, but we’ll simplify it by discussing the frequently used methods.
How to Instantiate an Array in C#
Here’s how you can instantiate an array in C#:
Loading code snippet...
Just a line of code and voila, you have yourself an array ready!
C# Instantiate Array of Objects
An object array in C# can hold values of different data types. Let’s see how to instantiate an array of objects:
Loading code snippet...
The above array can hold five different data type values, it’s like a house party where different data types are invited.
C# Initialize Array with Values
Just like setting a table before dinner, an array can be initialized at the time of its declaration. It’s quite a time saver!
Loading code snippet...
In this code snippet, we have already filled our array with values during instantiation. Efficient, isn’t it?
In-Depth Look at Array Declaration in C#
Array declaration is as pivotal as declaring your love. Too dramatic? Well, array declaration is indeed a crucial segment of C# programming.
How to Declare an Array in C#
Array declaration involves specifying the type of elements and the number it will hold. It’s like choosing the type and number of guests for a party, sounds exhilarating!
Loading code snippet...
In this instance, “numbers” is declared as an array which will hold numeric data.
Array Declaration C#
When we think about array declaration in C#, there are a few simple steps to it.
Loading code snippet...
Array declaration in C# is done in two steps: declaration and instantiation. Simple as ABC, right?
Methods to Initialize an Array in C#
Array initialization is analogous to baking. You have your ingredients and oven ready (declare and instantiate), now it’s time to bake (initialize)!
C# Initialize Empty Array
Wondering how to initialize an empty array? Here’s how:
Loading code snippet...
This declares an array of integers with no elements, suitable for temporary placeholders.
How to Initialize an Array C#
Wondering how to fill your array with hearty values? Let’s see:
Loading code snippet...
In this example, numbers
is an array named that holds integer values. Remember, it’s like inviting guests over for dinner.
A Look at C# Constant Arrays
A constant array? Sounds like an oxymoron, right? But in C#, it’s a widely-used concept that involves read-only arrays.
Understanding the Concept of C# Constant Array
Later on, we’ll further explain this intriguing aspect of C#: const
. Can’t keep this a secret any longer!
Loading code snippet...
A constant array is not possible in C#, but we have an alternative solution in the form of readonly
.
C# Define an Array
You are just one step away from defining your array now!
Loading code snippet...
This way, we just saved time, doing declaration, instantiation, and initialization all at once!
Common Questions about Array Instantiation
The terrain of array instantiation is across-the-board, and there might be some questions lingering in your mind. That’s completely okay! Peer through some of the common questions and their answers to have a better understanding.
Why does Initialize Array with Values matter in C#?
Consider a scenario where you have to feed the initial data as inputs to your program. This is where initializing an array with values comes handy!
What is the best practice for array declaration in C#?
Well, define what your array has to hold, instantiate it, and plug in the values according to your needs. It is a party, remember? Pick your guests wisely!
How is constant array used in real-world programming?
Though C# does not support constant arrays, readonly
is a commonly used alternative in situations where an array should not be modified.
The realm of array instantiation in C# is vast and fascinating. It simplifies complicated tasks by creating a single variable for a group of values. Mastering it would indeed provide you with an extra edge in your coding abilities. And remember, keep coding and keep creating those awesome arrays! Have you ever come across a coding challenge that you smashed using your array skills?