How To Convert C# Enum to String
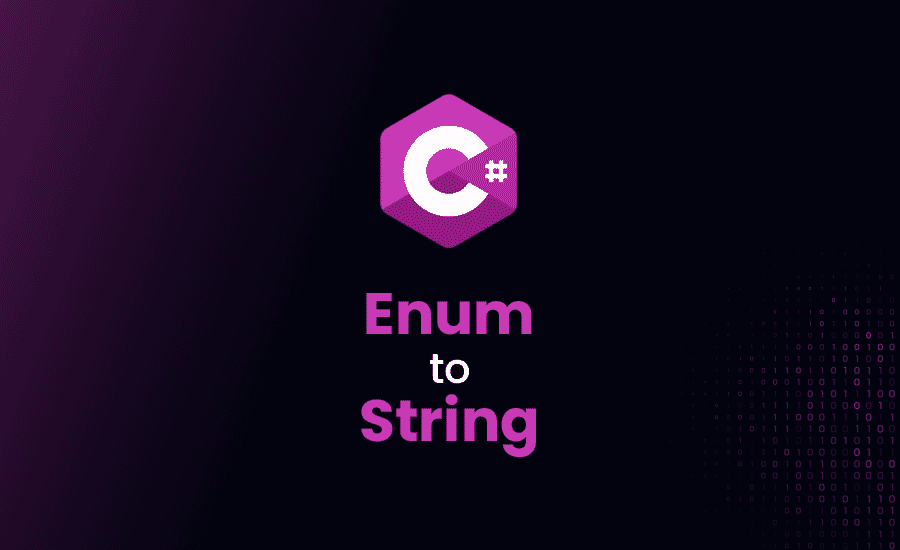
Let’s dive into the world of Enum conversions in C#. We’ll unravel the techniques, the idiosyncrasies, and the ace practices for efficiently converting Enums to strings in C#. Fasten your seatbelt, because we’re in for an exciting ride!
Efficient Ways to Convert C# Enum to String
Here, we’ll get our hands dirty with the basics. We’ll explore the ToString() method and Enum.GetName, the stalwarts of enum to string conversions.
Methodology of Converting C# Enum to String Using ToString()
Imagine your enum is like a book, and ToString() is like reading its title out loud. Here’s a basic example to clarify this:
Loading code snippet...
You see? It’s just like telling C#: “Hey, can you read this for me?” So refreshing, isn’t it?
Using Enum.GetName Method to Convert C# Enum to String
Now, let’s look at the GetName method. It’s like the cool librarian who knows exactly where to fetch the title you’re looking for from. Let’s see how to employ this method:
Loading code snippet...
Isn’t it amazing how C# can find Saturday just from a number? Kind of like a magical index in a library!
C# Enum to String Conversions: Advanced Techniques
Next up, let’s sail to the island of complex and advanced conversion techniques, discovering Extension Methods and Custom Attributes coupled with Reflection.
Understanding Extension Methods for Enum to String Conversion in C#
C# Extension methods are like putting a jet-pack on your enums. They allow us to add new methods without altering the definition of the existing classes. Let’s see how this works in code:
Loading code snippet...
Now, our DaysOfWeek enum has a new method, ToFriendlyString(). It’s like humans and enums communicating in a universal language, isn’t it cool?
C# Enum to String Conversion: Using Custom Attributes and Reflection
Next up, custom attributes and reflection, the sherlock holmes and watson in the world of C#. Let’s see them in action:
Loading code snippet...
Ever thought how helpful it would be if your enums could explain themselves? Well, they can, and we just saw it happening!
Error Handling during C# Enum to String Conversion
Error handling protects your code like an antivirus. It keeps bugs at bay and ensures smooth sailing of your code.
Common Errors and their Solutions when Converting Enum to String in C#
Enumerations are fun, but like every good thing, mistakes can happen. Here are few mistakes:
- Attempting to convert an empty enum
- Enum value that doesn’t exist
- Reverse conversion from a string that doesn’t match any enum constants
How do we solve these? Simple! Just listen to what the error messages are trying to tell you, and make the necessary corrections.
Best Practices for Error-Free C# Enum to String Conversion
To ensure happier enums, here is a list of practices;
- Always validate enum values before converting.
- Handle exceptions gracefully.
- Use switch statements for better control flow.
Performance Optimization for C# Enum to String Conversion
Speaking of performance, let’s step into the arena of optimization. Fast and efficient conversions are the name of the game here.
Evaluating Performance of Different C# Enum to String Conversion Methods
All methods are not created equal. The GetName method is swifter than ToString. Beware though, the fastest way is sometimes not the most convenient or versatile. Do you trade simplicity for speed?
Tips for Optimizing Performance during C# Enum to String Conversion
Here’s the holy grail of optimization tips:
- Prefer Enum.GetName() over ToString().
- Use switch-statements for runtime values.
- For small Enum types, precomputing a static dictionary could be beneficial.
Case Studies: Implementing C# Enum to String Conversion in Real-World Programs
Let’s descend from the clouds of theory and explore some practical applications of these conversions. Ready to see your enums at work?
Effective Enum to String Conversion in Data-Driven Applications
Enums can allude to data values in real-world apps. Imagine a scheduling application where enums represent days of the week, and we need to convert these to strings for displaying in the user interface. Using ToString() or GetName(), these conversions are a cakewalk!
The Role of C# Enum to String Conversion in Dynamic Programming
Let’s shift gear towards dynamic programming. Consider an algorithm where we use Enums to represent possible states. We’re debugging, and we want to print the states in a human-readable form. Just bring in ToString(). Voila, states are talking!
Learning how to convert C# Enum to String is like unlocking a secret portal in the expansive world of C#. It might not sound like a big deal at first, but when you get into it, when you start to explore its depths, you realize how powerful this skill really is. So, take this knowledge, and go build something extraordinary. Happy coding!