ASP.NET Web API Interview Questions and Answers
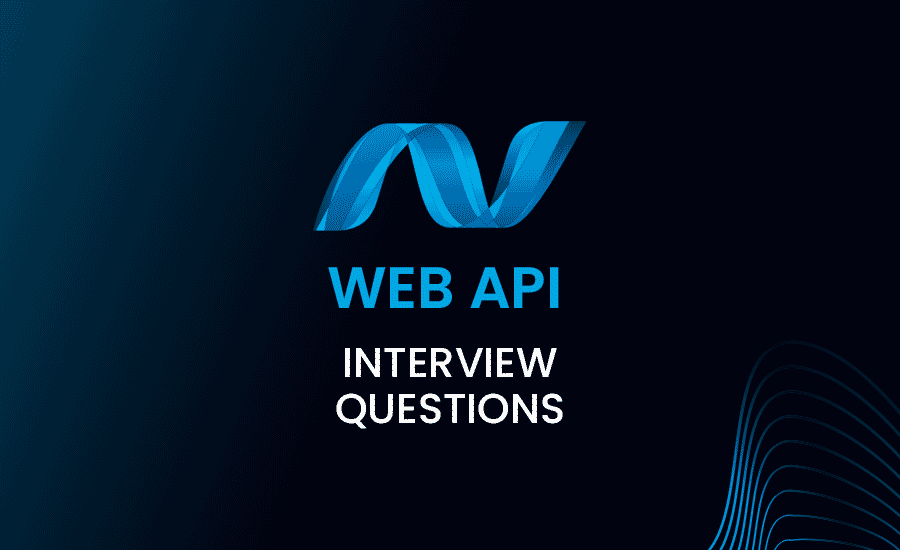
As a developer, preparing for an interview can often be both an exciting and challenging task. With the ever-evolving nature of technology and programming languages, it is crucial to stay up-to-date on the latest trends and developments in your field.
One such area that has gained significant traction in recent years is the world of web APIs, particularly ASP.NET Core Web API. This powerful framework is extensively used for building robust and scalable RESTful APIs in C#.
In order to help you ace your upcoming interview, we have compiled an extensive list of ASP.NET Web API interview questions and answers, covering topics such as authentication, authorization, versioning, and more.
Whether you are a seasoned web developer or just starting your journey in the world of web APIs, our comprehensive guide will provide you with the knowledge and insights needed to confidently navigate your next interview.
In ASP.NET Web API, which method is used to enable Cross-Origin Resource Sharing (CORS) for specific controllers and actions?
Answer
To enable Cross-Origin Resource Sharing (CORS) for specific controllers and actions in ASP.NET Web API, you can use the [EnableCors]
attribute. The [EnableCors]
attribute can be applied at the controller level, action level or at the global level.
Here’s how to enable CORS for a specific controller:
Loading code snippet...
In the [EnableCors]
attribute, you can define:
origins
: Allowed domain origins (use a comma-separated list for multiple domains).headers
: Allowed HTTP headers in requests (use*
to allow any headers).methods
: Allowed HTTP methods (use*
to allow any methods).
You can also enable CORS for a specific action within a controller:
Loading code snippet...
How is attribute routing in ASP.NET Web API different from conventional routing? Provide an example of attribute routing in a controller.
Answer
Attribute routing is a feature in ASP.NET Web API that allows you to define routes for your Web API actions directly on the controller or action, using route attributes. This is different from conventional routing, where routes are defined in a central location (e.g., in the WebApiConfig
class) and associated with the controller and action via the route name and parameters.
Advantages of attribute routing:
- Greater control and flexibility over the routes associated with actions.
- Improved organization and readability of your code, as routes are defined directly on their related actions.
- Attributes can be combined for more complex route patterns.
Here’s an example of attribute routing in a controller:
Loading code snippet...
In this example, the RoutePrefix
is defined at the controller level, and the Route
attributes define specific routes for each action within that controller. Note how the route constraints, like {id:int}
, can be used to specify the parameter’s data type.
What is the primary advantage of using IHttpActionResult as a return type instead of HttpResponseMessage in ASP.NET Web API?
Answer
Using IHttpActionResult
as a return type simplifies the code and improves testability compared to using HttpResponseMessage
in ASP.NET Web API.
Advantages of using IHttpActionResult:
- More readable and concise: Your action methods will focus on the core business logic while abstracting the creation of the HTTP response.
- Better testability: It is easier to test your controllers when returning
IHttpActionResult
since it separates the actual response generation from the rest of the action logic. - Increased flexibility: Using IHttpActionResult allows you to easily create and use custom action results.
For example, using HttpResponseMessage:
Loading code snippet...
Using IHttpActionResult:
Loading code snippet...
As seen in the example above, the IHttpActionResult implementation results in cleaner and more maintainable code.
How would you implement versioning in ASP.NET Web API, and what are the various approaches that can be used for API versioning?
Answer
Implementing versioning in ASP.NET Web API is important to maintain the compatibility of the API as it evolves. There are several approaches to achieve versioning, including:
- URI-based versioning: Include the version number directly in the API’s base URL.
Loading code snippet...
- Query string parameter-based versioning: Add the version number as a query string parameter.
Loading code snippet...
- Header-based versioning: Include the version information in a custom HTTP request header.
Loading code snippet...
- Content negotiation-based versioning: Use the
Accept
header and vendor-specific media types to include the version information.
Loading code snippet...
To implement versioning, you can use built-in features provided by the Microsoft.AspNet.WebApi.Versioning
NuGet package or implement custom routing logic and routing constraints in your WebApiConfig.
How can you handle exceptions globally in ASP.NET Web API to provide a consistent response to the clients?
Answer
To handle exceptions globally in ASP.NET Web API, you can create a custom exception filter by implementing the IExceptionFilter
interface or by inheriting from the ExceptionFilterAttribute
class. This allows you to catch and handle exceptions at a global level, providing a consistent response format to clients.
Here’s an example of a custom exception filter:
Loading code snippet...
After implementing your custom exception filter, register it in the WebApiConfig:
Loading code snippet...
By using a custom exception filter, you can provide a consistent exception handling mechanism and response format for the entire application.
Now that we’ve delved into exception handling and CORS, let’s move forward and explore some aspects of ASP.NET Web API that are crucial for building flexible and efficient APIs.
We will be discussing topics such as attribute routing, media type formatters, content negotiation, and more, which are essential aspects for creating highly maintainable web APIs in C#.
Can you explain the role of Media Type Formatters in ASP.NET Web API, and how they handle request-response content negotiation?
Answer
Media Type Formatters in ASP.NET Web API are responsible for serializing request data and deserializing response data in different media types. They play a crucial role in content negotiation between the client and the server. When a client sends an HTTP request to the Web API, it includes an Accept
header specifying the preferred media type(s) for the response. The Web API then uses its registered Media Type Formatters to find the best match and format the response data accordingly.
ASP.NET Web API includes built-in Media Type Formatters for commonly used media types such as JSON (using JsonMediaTypeFormatter
) and XML (using XmlMediaTypeFormatter
). You can also create custom Media Type Formatters to support additional media types or customize the serialization and deserialization process.
The Media Type Formatters in Web API:
- Handle serialization and deserialization of request data and response data.
- Participate in content negotiation process based on the client’s
Accept
header and available formatters. - Can be customized or extended for additional media types or custom logic.
To view or modify the list of registered Media Type Formatters, you can access the config.Formatters
collection in the WebApiConfig
:
Loading code snippet...
Briefly explain the process of creating a custom Media Type Formatter in ASP.NET Web API, and how to register it in the Web API pipeline.
Answer
Creating a custom Media Type Formatter in ASP.NET Web API involves the following steps:
- Create a new class that inherits from one of the base formatter classes (
MediaTypeFormatter
,JsonMediaTypeFormatter
, orXmlMediaTypeFormatter
), depending on your needs. - Override relevant methods to implement custom serialization and deserialization logic, such as
WriteToStreamAsync
for serialization andReadFromStreamAsync
for deserialization. - Register the custom formatter in the
config.Formatters
collection within theWebApiConfig
class.
Here’s a simple example of a custom Media Type Formatter:
Loading code snippet...
To register the custom Media Type Formatter, add it to the config.Formatters
collection in the WebApiConfig
:
Loading code snippet...
How can you implement authentication and authorization in ASP.NET Web API using token-based authentication?
Answer
To implement authentication and authorization in ASP.NET Web API using token-based authentication, you can follow these steps:
- Set up an OAuth 2.0 provider, such as IdentityServer, to issue and manage access tokens for your Web API.
- Configure your Web API to use the appropriate authentication middleware, such as JWT-based bearer tokens or other token types, to validate access tokens in incoming requests.
- Implement the
[Authorize]
attribute on controllers or action methods to require authentication for specific endpoints.
Here’s an example of how to configure ASP.NET Web API to use JWT bearer token authentication:
- Install the
Microsoft.Owin.Security.Jwt
NuGet package. - In the
Startup
class, configure the OAuth Bearer Authentication middleware:
Loading code snippet...
- Use the
[Authorize]
attribute on the controllers or action methods where authentication is required:
Loading code snippet...
With this setup, the Web API will validate incoming JWT bearer tokens issued by the authentication server, allowing access only to those clients with a valid token.
How can you use caching in ASP.NET Web API to improve performance and reduce response time, while ensuring the client receives current data?
Answer
Caching can significantly improve the performance of your ASP.NET Web API by storing the results of expensive operations and serving them directly from the cache instead of recomputing them. To use caching in ASP.NET Web API:
- Use caching mechanisms provided by the .NET Framework, such as
MemoryCache
for in-memory caching orObjectCache
for more advanced caching scenarios. - Implement caching logic within your Web API action methods, usually by checking for cached data before executing an expensive operation.
- Control cache freshness and client caching behavior by specifying appropriate HTTP caching headers, such as
Cache-Control
,Expires
,ETag
,Last-Modified
, etc.
Example of using in-memory caching in a Web API action:
Loading code snippet...
To ensure the data is not stale, you should set appropriate cache expiration times based on your specific requirements and use HTTP caching headers when applicable. Remember that caching should be used with caution and tested thoroughly to avoid serving outdated data to the clients.
Describe the process of implementing Dependency Injection in ASP.NET Web API using a third-party DI container, such as Autofac or Ninject.
Answer
Implementing Dependency Injection (DI) in ASP.NET Web API using a third-party DI container improves the modularity, maintainability, and testability of your code by allowing you to decouple components and manage their dependencies efficiently. This example demonstrates how to set up DI using Autofac, but the process is similar for other containers like Ninject.
- Install the following NuGet packages:
Autofac
Autofac.WebApi2
- Create an
IoC
(Inversion of Control) container configuration method in theWebApiConfig
class:
Loading code snippet...
- Modify your
WebApiConfig.Register
method to configure Autofac as the dependency resolver:
Loading code snippet...
- Call
Register
from theGlobal.asax
Application_Start
method:
Loading code snippet...
Now your ASP.NET Web API project is set up with Dependency Injection using Autofac. The DI container will automatically resolve the dependencies of your controllers and other registered components. You can easily swap implementations or mock dependencies for testing, without having to change the code that consumes them.
As we continue to investigate the world of ASP.NET Web API, we’ll now shift our focus to more advanced features and techniques that can enhance the overall API experience for both developers and clients.
From OData support and batch processing to implementing partial updates, these advanced topics will provide you with a solid foundation for taking your web API development skills to the next level.
What is the OData protocol, and how can you implement OData support in your ASP.NET Web API project?
Answer
OData (Open Data Protocol) is a standardized REST-based protocol for querying and manipulating data using HTTP, AtomPub, and JSON. It enables clients to perform advanced queries, filtering, sorting, and paging on the data exposed by the API while maintaining standardized conventions.
To implement OData support in your ASP.NET Web API project, follow these steps:
- Install the
Microsoft.AspNet.OData
NuGet package. - In the
WebApiConfig
class, enable OData by adding the required OData routes and conventions:
Loading code snippet...
- Change the base class of your API controller(s) to
ODataController
and apply the[EnableQuery]
attribute to your queryable action methods:
Loading code snippet...
Now clients can query your exposed OData endpoints using the OData protocol with standard query options like $filter
, $orderby
, $top
, $skip
, and more.
How would you implement batch processing for multiple requests in ASP.NET Web API, and what are the advantages of using batch processing?
Answer
Batch processing in ASP.NET Web API involves combining multiple HTTP requests into a single HTTP request, sending that bundled request to the server, and handling the response containing multiple individual HTTP responses. Batch processing can help reducing the number of round-trips between the client and the server, and improve the overall performance, especially in high-latency scenarios.
To implement batch processing in ASP.NET Web API, follow these steps:
- In the
WebApiConfig
class, add a batch route to support batch requests:
Loading code snippet...
- In your client-side code, create a batch request by combining multiple individual HTTP requests into a
HttpRequestMessage
, and send that batch request to the Web API using the/api/batch
endpoint:
Loading code snippet...
Advantages of using batch processing in ASP.NET Web API:
- Reduces the number of round-trips between client and server by combining multiple requests into a single request.
- Potentially increases performance, especially in high-latency scenarios.
- Can be useful for transactional processing, where multiple operations should be atomic (either all succeed or all fail).
Describe the concept of partial updates in ASP.NET Web API, and how can you implement it using the HTTP PATCH method.
Answer
Partial updates in ASP.NET Web API refers to the concept of updating only a subset of an entity’s properties, rather than replacing the entire entity. This can help reduce the data transferred between the client and the server and improve performance. Partial updates can be implemented using the HTTP PATCH
method, which is designed specifically for updating partial resources.
To implement partial updates using the HTTP PATCH
method in ASP.NET Web API:
- Add a reference to the
Microsoft.AspNet.WebApi.OData
NuGet package, which provides support for theDelta<T>
class, a convenient way to represent partial updates. - In your API controller, create a
PATCH
action method that accepts aDelta<T>
parameter representing the changes to the entity:
Loading code snippet...
- On the client-side, send a
PATCH
request with the partial update data:
Loading code snippet...
What is the role of action filters in ASP.NET Web API, and how they differ from authorization filters and exception filters?
Answer
In ASP.NET Web API, action filters, authorization filters, and exception filters are all types of filters that are used to add pre- and post-processing logic to the Web API pipeline. They allow you to modify or validate requests and responses, and are executed at different stages of the pipeline.
- Action Filters are executed before and after an action method is executed. They can modify the incoming request, cancel the action execution, or process the response returned by the action method. Action filters are useful for implementing cross-cutting concerns like logging, caching, and input validation. You can create a custom action filter by implementing the
IActionFilter
interface or inheriting from theActionFilterAttribute
class.
Example of a simple logging action filter:
Loading code snippet...
- Authorization Filters are executed before the action method and are used to implement access control logic, such as determining whether the current user is authorized to perform the requested action. You can create a custom authorization filter by implementing the `IAuthorizationFilter` interface or inheriting from the `AuthorizationFilterAttribute` class.
- Exception Filters are executed when an exception is thrown during the action execution. They can be used to catch and process unhandled exceptions, as well as return a unified error response to the client. You can create a custom exception filter by implementing the `IExceptionFilter` interface or inheriting from the `ExceptionFilterAttribute` class.The main differences between action filters, authorization filters, and exception filters are their specific roles, the stage of the pipeline they are executed in, and the interfaces or base classes they implement or inherit from.
How would you implement ModelState validation in ASP.NET Web API to handle invalid inputs and data binding issues?
Answer
ModelState validation in ASP.NET Web API is used to validate the data passed as input to action methods, ensuring that it adheres to the data model requirements. ModelState validation relies on the `ModelState` property of the controller, which collects validation errors detected during the data binding process.To implement ModelState validation in your ASP.NET Web API project:1. Add data annotation attributes, such as [Required]
, [StringLength]
, and [Range]
, to your data model properties for specifying validation rules:
Loading code snippet...
2. In your API controller, check the ModelState.IsValid
property to determine if the submitted data is valid according to the data model validation rules:
Loading code snippet...
Now, when a client submits an invalid input, the Web API will return a 400 Bad Request
HTTP response with the validation errors.
By using ModelState validation in your Web API project, you can effectively handle invalid inputs and data binding issues, providing meaningful error messages to clients while ensuring data integrity.
Having covered a variety of key concepts and techniques, let’s now dive into some deeper areas of ASP.NET Web API that might be of interest, including ModelState validation, parameter binding, and hosting options.
Understanding these more nuanced aspects of the framework is essential for building robust web APIs that can handle a wide range of scenarios and complexities.
What is the purpose of the ApiController.Basic() method, and how does it differ from ApiController.BasicAllowingNullToken() in ASP.NET Web API?
Answer
In ASP.NET Web API, the ApiController.Basic()
and ApiController.BasicAllowingNullToken()
methods are used to validate the incoming HTTP request’s authorization header against the expected Basic Authentication format. Both methods analyze the “Authorization” header to ensure that it contains a valid “Basic” authentication token.
The difference between Basic()
and BasicAllowingNullToken()
lies in their handling of empty or null tokens:
Basic()
: This method returns a400 Bad Request
response if the “Authorization” header is present but the Basic Authentication token is null or empty. In other words, it expects a non-empty token in the request if the authentication scheme specified is “Basic”.BasicAllowingNullToken()
: This method allows null or empty Basic Authentication tokens and does not return a400 Bad Request
response in such cases. This can be useful when you want to support optional authentication in your API – i.e., when you want to allow certain actions to be performed by both authenticated and anonymous users.
In both cases, you should separately verify the user’s credentials (typically done by comparing the obtained token with the one stored in the server-side database) to determine whether access should be granted or not.
Here’s an example of how to use the Basic()
method to extract the username and password from the request’s “Authorization” header:
Loading code snippet...
Remember that Basic Authentication exposes credentials (albeit base64-encoded) in the request header; hence, it is recommended to use HTTPS when implementing Basic Authentication in your Web API for secure communication.
Describe the differences between using IHttpActionResult and creating custom HttpResponseMessage to return HttpErrors in ASP.NET Web API.
Answer
In ASP.NET Web API, you can use both IHttpActionResult
and HttpResponseMessage
as return types for your action methods to encapsulate the response that should be sent back to the client. When you need to return an error response, such as a 404 Not Found or a 400 Bad Request with a custom error message, you can use either approach. However, there are some differences and advantages to using each method:
- IHttpActionResult: This is an interface that encapsulates the creation of an
HttpResponseMessage
. It provides a set of built-in helper methods for returning specific HTTP status codes along with their corresponding messages. For returning errors, you can use theBadRequest(string message)
andNotFound(string message)
methods, among others.
Using IHttpActionResult
for error responses:
Loading code snippet...
Advantages of using IHttpActionResult:
- Provides cleaner and more expressive code.
- Improves testability by decoupling the creation of the
HttpResponseMessage
from the action method logic. - HttpResponseMessage: This class directly represents an HTTP response, including the status code, headers, and content. For returning errors, you can create a new
HttpResponseMessage
with the corresponding HTTP status code and a customHttpError
object as the content.
Using HttpResponseMessage
for error responses:
Loading code snippet...
While both methods can be used to return error responses, IHttpActionResult is generally preferred due to its cleaner syntax, better expressiveness, and improved testability.
What is the role of parameter binding in ASP.NET Web API, and how can you create a custom parameter binder for managing complex data types in action methods?
Answer
Parameter binding in ASP.NET Web API is the process of extracting data from the HTTP request and converting it into arguments that are passed to the action methods. Web API uses a set of default parameter binders to automatically handle data binding for simple data types, such as primitive types, complex objects, and collections.
However, there may be cases where you need to handle binding for complex or custom data types that cannot be converted by the default parameter binders. In such situations, you can create a custom parameter binder by implementing the IModelBinder
interface and overriding the BindModel()
method. This allows you to control how the data is bound to your action method’s parameters and handle any data conversion or validation issues.
To create a custom parameter binder in ASP.NET Web API:
- Implement the
IModelBinder
interface and override theBindModel()
method:
Loading code snippet...
- Register the custom model binder in the
WebApiConfig
class:
Loading code snippet...
- Use the custom data type as a parameter in your action method:
Loading code snippet...
By creating a custom parameter binder, you can manage complex data types in your action methods while maintaining a clean and structured approach to handling data binding and validation.
Explain the concepts of self-hosting and IIS hosting in the context of ASP.NET Web API, and discuss their advantages and disadvantages.
Answer
ASP.NET Web API supports two different hosting options: self-hosting and IIS (Internet Information Services) hosting. Each hosting option has its own benefits and drawbacks, depending on the requirements and constraints of the application.
Self-hosting involves hosting your Web API directly within your application, typically using the HttpSelfHostServer
class. With self-hosting, you can create an instances of a Web API server within any .NET application (e.g .console app, Windows service, or WPF app), without the need for an external web server like IIS.
Advantages of self-hosting:
- Greater control over the pipeline, as the application directly hosts the Web API server.
- Can be hosted in a variety of application types, not limited to IIS.
- Easier setup and deployment, as no IIS configuration is required.
Disadvantages of self-hosting:
- Lacks some features and optimizations provided by IIS, like process recycling, performance tuning, and security features.
- Requires manual configuration and management of the Web API server.
- Potentially less scalable and less suitable for high-performance applications.
Loading code snippet...
IIS hosting involves hosting your Web API within the IIS web server infrastructure. This is the most common hosting option for web-based applications and is typically achieved using the HttpServer
class. IIS hosting provides a range of features and optimizations required for production environments.
Advantages of IIS hosting:
- Offers features like process recycling, performance tuning, and security enhancements that are optimized for web applications.
- Provides better scalability and performance for web-based APIs.
- Simplifies management and configuration by leveraging the IIS administration tools.
Disadvantages of IIS hosting:
- Requires IIS configuration and setup, which can be complex.
- Limited to applications running within the IIS infrastructure.
- Less control over the pipeline compared to self-hosting.
To host your Web API in IIS, create a new ASP.NET Web API project and deploy it to the IIS server using standard deployment procedures.
In summary, choosing between self-hosting and IIS hosting depends on your application’s requirements, including performance, scalability, and management needs. Self-hosting is more suitable for simple applications with minimal infrastructure requirements, while IIS hosting provides a robust environment for large-scale, production-ready web APIs.
What are the key differences between ASP.NET MVC controllers and Web API controllers, in terms of functionality, core components, and routing?
Answer
ASP.NET MVC and ASP.NET Web API are two frameworks within the .NET ecosystem designed to build web applications and services, respectively. While they share some core components and patterns, there are several key differences between MVC controllers and Web API controllers regarding their functionality, core components, and routing mechanisms.
- Functionality:
- ASP.NET MVC Controllers are designed for building traditional web applications that generate HTML views for the browser. They typically use server-side rendering and follow the MVC design pattern, with actions returning
ActionResult
objects (such asViewResult
orPartialViewResult
). - ASP.NET Web API Controllers are specifically designed for building RESTful APIs that return data (usually in formats like JSON or XML) to clients, such as web browsers, mobile apps, or other servers. They don’t render HTML views and instead follow a resource-based architecture, with actions returning
HttpResponseMessage
or, more commonly,IHttpActionResult
.
- Core Components:
- MVC Controllers inherit from the
System.Web.Mvc.Controller
base class and utilize theActionResult
return types. - Web API Controllers inherit from the
System.Web.Http.ApiController
base class, which provides functionality for managing HTTP requests and responses, and useHttpResponseMessage
orIHttpActionResult
return types.
- Routing:
- ASP.NET MVC uses conventional routing based on the controller and action names. The default routing pattern is “{controller}/{action}/{id}”, where the controller, action, and optional id parameter values are matched from the request URL.
- ASP.NET Web API supports both conventional and attribute-based routing. Attribute-based routing allows defining custom route templates directly on the controllers and actions, providing more control over the URLs exposed by the API. Web API also supports advanced routing scenarios like route constraints, optional parameters, and default values.
Though there are some key differences between MVC and Web API controllers, it’s important to note that starting with ASP.NET Core, these distinctions have become less relevant. In ASP.NET Core, both MVC and Web API share the same Controller
base class and routing system, unifying the development experience for both web applications and APIs.
Congratulations on making it through our comprehensive list of ASP.NET Web API interview questions and answers! We hope that this guide has provided you with valuable insights and a deeper understanding of web API development in C#.
Whether you’re being questioned on authentication, authorization, or more advanced aspects like OData support and batch processing, we’re confident that you’ll now be better prepared to tackle any web API interview questions that come your way. Remember to keep exploring and expanding your understanding of web APIs, and don’t forget to practice and review these concepts periodically to stay sharp.
Good luck with your next interview, and happy coding!