ASP.NET Interview Questions and Answers for freshers
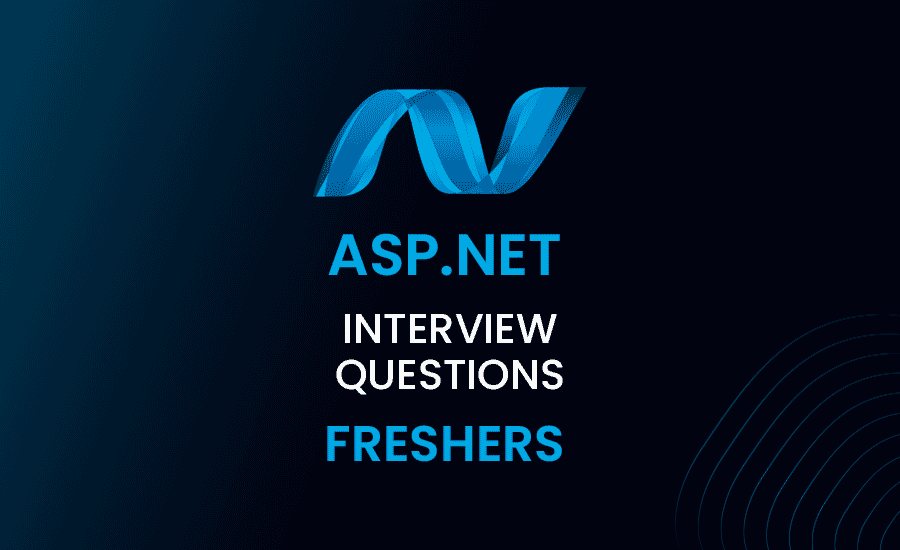
Staying relevant in the fast-paced world of web development necessitates an unyielding curiosity and willingness to understand new platforms and paradigms.
ASP.NET, as a cutting-edge technology, is no exception, and both aspiring and experienced developers will find tremendous value in mastering its intricacies. Whether you’re a fresher venturing into the world of professional web development or an expert seeking to deepen your knowledge base, our guide offers detailed insights into the most common yet basic ASP.NET interview questions.
This groundwork will not only prepare you for your upcoming interview but will broaden your understanding of these ASP.NET basics for interview contexts and beyond.
Let’s begin!
What are the main differences between ASP.NET WebForms and ASP.NET MVC?
Answer
ASP.NET WebForms and ASP.NET MVC are both web application frameworks, but they use different approaches to web development.
ASP.NET WebForms:
- -driven model: WebForms follows an event-driven model, which means the code executes in response to events (like button clicks) on the server-side.
- ViewState: WebForms uses ViewState to maintain states between requests which can lead to increased loading times with heavy usage.
- Postback: The Postback principle in WebForms can cause full page reloads which might hinder a seamless user experience.
- Less control over HTML: WebForms does not provide full control over the rendered HTML which might cause issues while working with JavaScript libraries or CSS.
ASP.NET MVC:
- Model-View-Controller (MVC): As the name suggests, ASP.NET MVC follows the MVC pattern that separates an application into three main components: Model, View, and Controller. This promotes clean code and allows us to work on individual components independently.
- No ViewState: MVC does not use ViewState to maintain the state of the elements. This reduces page load times and resources.
- Control over HTML: MVC gives us complete control over the rendered HTML, allowing greater flexibility with JavaScript and CSS.
- Unit Testable: The MVC structure is designed to be testable, making unit testing of applications easier.
What is the role of ViewState in ASP.NET?
Answer
In ASP.NET web applications, ViewState is used to preserve the state of server-side objects between page postbacks.
Here’s what ViewState does:
- Data preservation: ViewState helps in preserving the data of controls, forms, etc. during page postbacks. Since HTTP is stateless by design, ViewState provides a way to retain data across POST to the server.
- Encoding: ViewState stores the data as a HashTable and converts it into a Base64 encoded string before sending it as a part of the HTML output.
- Security: ViewState ensures data integrity and confidentiality by using Message Authentication Codes (MAC) and optionally encrypting the ViewState data.
However, heavily using ViewState can increase the size of page responses and subsequently slow down the application. You should use ViewState judiciously.
Can you describe how the Page lifecycle works in ASP.NET?
Answer
Page lifecycle in ASP.NET is a series of steps or events that occur from the moment an ASP.NET page is requested till the time it is rendered on the client and ready to be sent back. Here are the key steps involved:
- Page request: The lifecycle begins when the page is requested by a user.
- Start: During start, page properties such as Request and Response are set. The IsPostBack property is also checked to determine whether this is the first time the page is being processed.
- Initialization: All controls on the page are initialized.
- Load: During load, control properties are set using the ViewState and control state values.
- Postback event handling: If the request is a postback (a subsequent request), any event handlers are called.
- Rendering: Before rendering, view state for the page and all controls are saved. The page calls the Render method for each control and the output of rendering is written to the OutputStream class of the Page’s Response property.
- Unload: Unload is called after the page has been fully rendered, sent to the client, and is ready to be discarded. At this point, page properties such as Response and Request are unloaded and all cleanup is performed.
How does ASP.NET maintain state between page postbacks?
Answer
ASP.NET provides several techniques to maintain state between page postbacks because HTTP is stateless by natural and can’t retain information between requests. Here are the most common ways:
- ViewState: As mentioned earlier, ViewState can store data between postbacks within the page itself.
- Session State: Session State is a server-side storage mechanism that can store data specific to a session or user.
- Cookies: Cookies are small data stored on the client-side and sent back to the server with each request.
- Hidden fields: You can also store small amounts of data in hidden fields in your form.
- Query Strings: Query strings encode data in the URL, which can then be read by the receiving page.
What would you explain the concept of Master Pages in ASP.NET?
Answer
Master Pages in ASP.NET are a feature that allows you to define a common structure for multiple pages in your application.
Here’s how Master Pages work:
- Define common layout: Master Pages allow you to define a layout that will be shared across multiple pages. You can include common elements like headers, footers, navigation menus, etc.
- Content Placeholders: You define placeholders (
ContentPlaceHolder
) in the Master Page, where unique content will be inserted. - Consistent look and feel: All pages using the same master page will have a consistent look and feel.
- Ease of maintenance: Changes in the layout need to be made only once in the Master Page, and they’ll propagate down to all the pages that use that Master Page.
A typical Master Page might contain a header, a navigation menu, and a footer, with a placeholder in the body for the unique content of each page.
Drawing from a pool of originality and precedence, ASP.NET has not skipped a beat in preserving some features while innovating with others.
We’ve already carved a good understanding of Master Pages. As we move further, we will delve into the heart of ASP.NET applications with the Global.asax file, expanding your horizon of basic ASP.NET interview questions.
This shift in focus allows us to appreciate the adaptability of ASP.NET and its wide applicability in web development scenarios.
What is the purpose of a Global.asax file in an ASP.NET application?
Answer
The Global.asax file, also known as the ASP.Net application file, is a special file in ASP.NET. The Global.asax file, present within the root directory of an ASP.NET application, is optional but can be used to handle application-level and session-level events. The file is automatically parsed and handled by ASP.NET.
Here are few important functions of the Global.asax file:
- Application-level events: These include application start, application end, application error, etc. Application start event allows you to run code when the application starts and application end event lets you run code when the application is ending.
- Session-level events: These include session start and session end events. Session start runs code when a new session starts and session end lets you run code when a session ends or times out.
- Security-related events: For example,
AuthenticateRequest
takes place when ASP.NET attempts to authenticate the user’s identity.
The Global.asax file is a good place to set up global variables, event handlers, and other settings that you want to apply when your web application starts or stops.
Can you explain what a Postback event is in ASP.NET?
Answer
Postback is a mechanism where the page is automatically submitted back to the server on a user interaction with controls on the page that have AutoPostBack
property set to True
. The term “Postback” is exclusively associated with ASP.NET WebForms and not ASP.NET MVC.
Here are some specifics about Postback operation:
- During a postback event, the entire page and all its controls are reloaded and any user input in the controls is lost.
- To preserve the changes on postback, ASP.NET uses a feature called ViewState.
- Typical examples of controls that cause a postback are Button, LinkButton, DropDownList (with
AutoPostBack
set totrue
), RadioButton, etc.
How can you secure an ASP.NET application?
Answer
Securing an ASP.NET application can be done in various methods:
- Authentication: This can be performed using forms-based authentication, Windows authentication, or custom authentication methods.
- Authorization: This involves setting permissions and limiting access to certain parts of your application based on roles of users.
- Secure Communication: Use HTTPS or SSL to ensure data being transmitted is encrypted.
- Input Validation: Always validate user input to protect against attacks like Cross-Site Scripting (XSS), SQL Injection, etc.
- Use Security Headers: These help to reduce XSS, clickjacking, and other code injection attacks.
- Error handling: Conceal system errors from users to prevent exposing sensitive data.
- Session Management: Use secure session management techniques, avoid storing sensitive information in the session, implement session timeout.
What is the use of Web.config file in ASP.NET?
Answer
Web.config file is the main settings and configuration file for an ASP.NET web application. The file is an XML document that defines configuration and settings data for the web application.
Here are a few key things the file controls:
- Database Connection Strings: It can store and manage the pathway to the application’s database.
- App Settings: Custom application-level settings can be defined within the Web.config.
- Security settings: It can contain settings for authentication and authorization mechanisms for the application.
- Session State Settings: Can be used to specify where session state is stored, for example, InProc (in process), State Server, SQL Server or Custom.
- Error Settings: It can hold instructions about how to handle and where to direct users in various error conditions.
How does ASP.NET handle exceptions and errors?
Answer
ASP.NET has several ways to handle exceptions and errors:
- Try-Catch-Finally: This is the common approach to catch exceptions at the code level.
- Custom Error Pages: ASP.NET allows you to create custom error pages and redirect users to those pages when an error occurs.
- Global.asax Application_Error: This event in the Global.asax file gets triggered for all unhandled errors in the application.
- Logging: It is essential to log errors. Libraries like NLog, log4net or Serilog can be used for structured logging.
- Health Monitoring: ASP.NET health monitoring can log error details and other application events.
- ASP.NET MVC HandleError attribute: In ASP.NET MVC, this attribute can be applied to entire controllers or individual action methods to handle exceptions.
Remember to not expose sensitive information in the error details being displayed or logged.
Thus far, our exploration of ASP.NET has taken us from handling exceptions and errors to the gates of more complex topics. As we move forward, we step away from the norm and look at advanced features of ASP.NET, like HttpHandlers and HttpModules.
These concepts, although intricate, make asp net interview questions for freshers interesting and enable developers to build more efficient and robust applications.
Let’s delve deeper into these dynamic processes and how they influence the smooth operation of ASP.NET applications.
What are HttpHandlers and HttpModules in ASP.NET?
Answer
- HttpHandlers: An HttpHandler in ASP.NET is a simple .NET class that implements the IHttpHandler interface. When used, it allows developers to programmatically handle and control the processing of individual web requests made to an ASP.NET application. HttpHandlers will get called when a request comes in, then generate the required response by producing an HTML page.
Loading code snippet...
- HttpModules: HttpModules are classes that are called on every single request made to your application. You can think of them as interceptors or filters. These classes tap into the pipeline at different points, allowing you to hook custom logic naturally into request handling. These modules are used for security, statistics, URL rewriting; basically, anything that you would want to apply to numerous pages or requests.
Loading code snippet...
What is the difference between Server.Transfer and Response.Redirect methods in ASP.NET?
Answer
- Server.Transfer()
- This method transfers from one page to another page on the same server.
- It preserves server resources and avoids the unnecessary roundtrips to the client.
- This method also provides the option to preserve the Query String and Form Variables.
Loading code snippet...
- Response.Redirect()
- In contrast to Server.Transfer(), the Response.Redirect() method sends a response to the client (browser) with an HTTP 302 status, which causes the client to issue a GET request to the other page.
- This results in a round trip to the client, and the new request happens independently from the original request.
- The browser URL reflects the redirected page.
Loading code snippet...
They are both used for navigation but serve different purposes based on where and how you want to navigate.
Can you describe the concept of Session and its role in ASP.NET?
Answer
- Session is a feature in ASP.NET applications that enables you to store and retrieve values for a user as the user navigates the different pages that make up a Web application. A session, in the context of ASP.NET, is a series of HTTP requests made by the same client (perhaps a browser or a crawler).
- A Session object stores data that is to be remembered across multiple requests in a web application. In other words, session variables hold information about one single user, and are available to all pages in one application.
- It provides a way to store user-specific data, that is, data related to a single user session.
Below is a sample code using session in ASP.NET:
Loading code snippet...
What is the purpose of the App_Code folder in an ASP.NET application?
Answer
- The
App_Code
folder in an ASP.NET application is automatically set up to store reusable classes, typed datasets and business objects. This is the place where you can place your class files (.cs, .vb) that you want to be automatically compiled as part of your application. - The contents (source files, XML, HTML, and so on) of this special ASP.NET folder are compiled automatically at runtime, and the resulting assembly is accessible in all parts of the web application.
- It is a special ASP.NET folder which is present at the root of the application.
- The main advantage is that it automatically compiles the code and makes it accessible throughout the web site project.
How can you implement AJAX in an ASP.NET application?
Answer
- AJAX stands for Asynchronous JavaScript and XML. It is used for creating faster and more interactive web applications. With AJAX, you can update the contents of a page without doing a full page refresh.
- In ASP.NET, Microsoft provides built-in support for AJAX through the
ScriptManager
andUpdatePanel
controls. - ScriptManager control manages client script libraries for AJAX-enabled ASP.NET pages. This control supports partial-page rendering and Web-service calls.
- UpdatePanel control, which works with the ScriptManager control, enables you to refresh selected parts of the page instead of refreshing the whole page with a postback.
Sample code using AJAX UpdatePanel:
Loading code snippet...
In the code behind, you might handle the button click like this:
Loading code snippet...
Upon clicking the “Refresh” button, only the contents of the UpdatePanel will be refreshed, and the Label will be updated with the current time.
By now, we have firmly grasped the implementation of AJAX in an ASP.NET application. It’s time to switch gears and aim for a comprehensive understanding of the architectural style that underpins ASP.NET – Model-View-Controller (MVC).
The concept of MVC is central to ASP.NET and will surely make frequent appearances in your asp net interview questions and answers.
Bridging the gap between diverse functionalities in an organized manner is what MVC does best, and understanding its intricacies will go a long way in catering to user demands and improving web application performance.
What are the main three parts of an MVC (Model View Controller) application?
Answer
ASP.NET MVC is a framework for building web applications that applies the general Model-View-Controller (MVC) pattern to its architecture. It divides an application into three interconnected parts:
- Model: The Model represents the application’s data and business logic. It is responsible for maintaining data and its associated business rules. In ASP.NET MVC, models are typically represented by classes that retrieve and store model state in a database. For example, a “Product” model might retrieve information from a database, operate on it, and then write updated information back to a ‘Products’ table in a SQL Server database.
public class Product { public int Id { get; set; } public string Name { get; set; } public decimal Price { get; set; } }
- View: A View is the user interface of the application that displays the Model’s data. Views are responsible for transforming Model(s) into a user interface (UI), and they define exactly what gets rendered to the browser. In an ASP.NET MVC application, views are usually written using the Razor view engine syntax to embed .NET code in HTML.
@model Product <h2>@Model.Name</h2> <div>@Model.Price</div>
- Controller: The Controller acts as an interface between Model and View. It responds to user input, interacts with the Model, and selects a View to render that displays the user interface. In ASP.NET MVC, Controller classes are where user requests are routed. Every public method in a Controller is accessible as an HTTP endpoint.
public class ProductsController : Controller { public ActionResult Details(int id) { var model = database.FindProduct(id); return View(model); } }
What is the use of RouteConfig.cs file in ASP.NET MVC?
Answer
In an ASP.NET MVC application, the RouteConfig.cs
file is used to define the URL routing rules to map incoming requests to their respective controller actions.
This file is typically located in the App_Start
folder, and the RegisterRoutes()
method within it contains routing rules:
Loading code snippet...
The MapRoute
function defines the routing schema, where the “controller”, “action”, and “id” are parameters. If a request comes in for the root of the website, it will be mapped to the “Home” controller and the “Index” action method. The optional “id” parameter corresponds to the method’s arguments.
What are Action filters in ASP.NET MVC and how are they used?
Answer
Action filters in ASP.NET MVC are custom attributes that can be applied to controller actions or entire controllers to add or modify their behaviors.
Action filters provide two main features:
- Custom Logic: They allow you to execute code before or after the execution of an action method. They are useful to reduce code duplication and to separate concerns. For instance, you can create a logging filter that logs all executed controller actions.
- Result Modification: Some filters can also change the result that is returned by an action method. For instance, a cache filter can save the result of an action method to avoid unnecessary database calls.
Here’s a simple example of a custom Action filter:
Loading code snippet...
Then you can apply this filter to your action methods or controllers:
Loading code snippet...
Can you explain what Entity Framework is and how you would use it in an ASP.NET application?
Answer
Entity Framework (EF) is an open-source Object-Relational Mapping (ORM) framework for .NET. It allows developers to work with data as strong typed .NET objects, abstracting the underlying database details. Entity Framework supports a range of database operations including create, read, update and delete (CRUD), as well as transactions and migrations.
In an ASP.NET MVC application, you would typically use Entity Framework in your models to interact with your database:
Loading code snippet...
Then in a controller, you could use it like this:
Loading code snippet...
How can you deal with the data concurrency in an ASP.NET application?
Answer
Data concurrency in ASP.NET applications usually involves handling a situation where multiple users read, update, and delete resources simultaneously, which can result in inconsistent data.
Here are ways to handle data concurrency in ASP.NET applications:
- Optimistic Concurrency: In optimistic concurrency, which is the default approach for Entity Framework, you read a record, make modifications, and write the modified record back to the database. If the record was modified by another user in the meantime, you handle the concurrency exception. For example:
try { _context.SaveChanges(); } catch(DbUpdateConcurrencyException ex) { // handle exception... }
- Pessimistic Concurrency: In pessimistic concurrency, you lock the record when you read it, blocking other users from modifying it until you’ve finished your operation. In ASP.NET, this is usually accomplished with read locks at the database level.
- Last-In-Wins: This is a simple approach where the last change by a user is written to the database, overwriting any other changes without any notification. This one has the potential to lose updates and is best avoided where possible.
Remember, proper error handling and response are also required when handling data concurrency.
Embarking on the path of ASP.NET is an exciting journey of exploration and understanding. From tackling basic ASP.NET interview questions to advanced principles, this guide ensures you step into your next interview brimming with confidence.
ASP.NET is a powerful and dynamic framework, and mastering its intricacies can set you apart in the web development world. However, bear in mind that every interview is different, and a comprehensive understanding of the basics forms a strong foundation for confronting any challenging questions that might surface.
So stay keen, keep learning, and pave your way to success.