C# YAGNI Principle (You Aren’t Gonna Need It!)
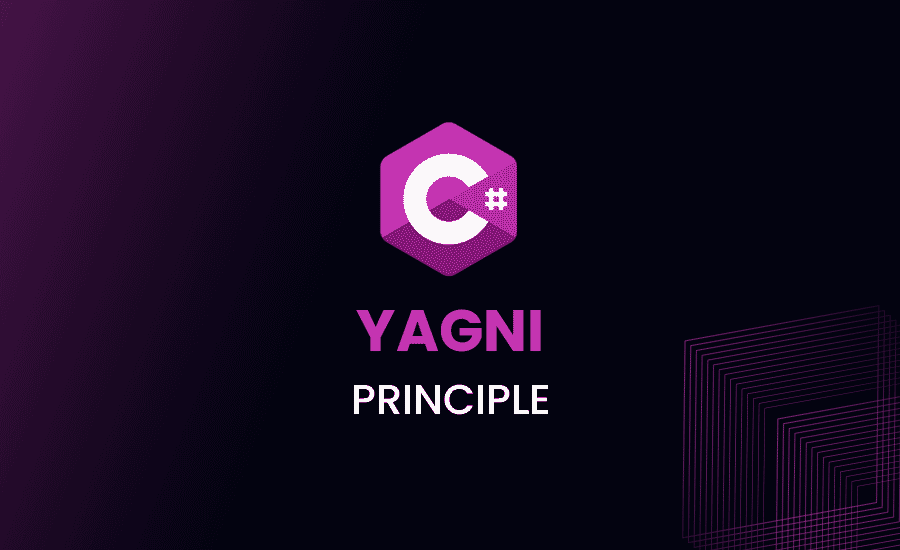
Heard of the Yagni Principle but aren’t exactly sure what it is or how it applies to C#? Don’t fret! We’ll go in-depth on the Yagni (You Aren’t Gonna Need It) principle, its importance in C# programming, and why it’s all the rage for developers prioritizing efficiency and simplicity. So strap in and let’s navigate the universe of C# with Yagni as our guiding star.
Introduction to Yagni Principle in C#
What is the Yagni Principle?
The Yagni principle operates on a simple idea: If something isn’t necessary, don’t implement it. This isn’t advocating for pre-crastination or laziness, no siree! It’s a fundamental approach to deter over-engineering and unnecessary work.
Loading code snippet...
See the difference? Big changes come from small tweaks.
Origins and Purpose of the Yagni Principle
Coined by Extreme Programming (XP) co-founder Ron Jeffries, the Yagni principle is ingrained in Agile methodologies. The essential idea? Code with the future in mind, but don’t let it dictate your present.
Yagni Principle and Agile Development
The Yagni principle isn’t just a standalone concept—it’s a key ingredient in a much larger beast known as Agile development. Let’s unravel the deep interconnections between Yagni and Agile, understand its role in Agile methodologies, and how it suits perfectly in a Scrum environment. Brace yourself; we’re going on a deep dive!
The role of Yagni in Agile methodologies
In Agile, we value responding to change versus following a set-in-stone plan, which makes the Yagni principle a perfect companion in the Agile environment. Iterative cycles in Agile are fundamentally about developing the necessary features in the current sprint, without losing sleep over what may or may not be needed in the future.
That’s Yagni in a nutshell! It thrives in the Agile ecosystem because it wholeheartedly resonates with the idea of evolving requirements. Wondering how Yagni and Agile work in tandem? Check out this code snippet:
Loading code snippet...
Isn’t that just beautifully simple? As concise as a poem and as efficient as a Swiss watch.
Applying the Yagni Principle in Scrum
Scrum, a popular Agile methodology, is often likened to a game of rugby. You pass the ball (incremental development works) forward (towards the project completion goal) in sprints (timely iterations). Just as you need mindful presence in rugby to focus on the current play, Scrum mandates developers focus on the present sprint, not intricately strategizing for distant future possibilities. Yagni, with its “implement it when you need it” philosophy, fits like a glove in a Scrum setting.
To get a better feel for the application of Yagni in a Scrum environment, let’s hunt down another code example.
Loading code snippet...
Simple? Yes. Sensible? Absolutely. Agile and Scrum, with the Yagni principle, are all about being present and conscious of the “now” in your development cycle.
Let’s ponder a real-life “8-year old situation” here. Ever handed your kiddo a jigsaw puzzle? They focus on joining one piece at a time, instead of worrying about the whole picture. They are unknowingly following the Scrum methodology with a touch of Yagni—dealing with what’s necessary “now” rather than worrying about what is needed at the end.
Bringing Yagni to your Agile and Scrum modes of operation isn’t just about reducing complexity, it’s about growing a productivity tree from a simplicity seed, and nurturing an uncomplicated, adaptable mindset. After all, real progress stems from the ability to adapt and develop with simplicity, doesn’t it? No extra baggage, just what’s needed. That’s the Agile-Yagni way.
Delving Deep into the Yagni Principle Paradigm in C#
Get ready because we’re set to deliver a deep dive into one of the pillars of smart coding – the Yagni Principle. This section is aimed at giving you an invaluable understanding of how Yagni is instrumental in C# development. While we’ve given a brief overview previously, here we’ll throw open the gates and inundate you with rich examples and real-world applications. Now, why is Yagni so significant in the complex, wide world of C#? Let’s dive in!
The Critical Role of the Yagni Principle in C# Development
In the constantly evolving landscape of C# development, the implementation of robust, efficient processes is not just a nice-to-have; it’s a competitive necessity. The value of Yagni in this context is comparable to the keystone in an arch; it holds everything else together.
Take game development in C#, for instance. Suppose you’re developing a character leveling system for your game. With an eager mind, you might envision advanced features like special abilities, power boosts, and skill trees. Driven by this, you might be tempted to build complex structures to cater to potential future updates. Sadly, most of this effort often goes to waste, as more than half of these elaborate features aren’t required in the initial rounds, depriving you of valuable time and resources.
Loading code snippet...
By adhering to the Yagni principle, you purposefully simplify your development process and save valuable resources, which can be channelized into immediate requirements.
The Tangible Benefits of Practicing the Yagni Principle in C#
So, Yagni sounds like a rosy concept in theory, but what are the practical benefits you ask? Imagine a future where your code is lean, mean, and clean. A future where you’re not pulling all-nighters debugging your unnecessarily complex system.
That’s not a utopian dream; that’s the reality with Yagni. Here are some hand-picked, game-changing benefits to add more perspective:
Reduced Complexity and Fewer Headaches
Say goodbye to those monster headaches you get seeing your overly complex code. With Yagni, you only write what’s necessary, curbing the complexity and keeping your code neat and manageable.
Increased Readability and Understandability
The easier your code is to read, the easier it is to maintain. Rather than cryptically studying line after line of code, Yagni encourages simple, direct code that anyone on your team can understand and update.
Shorter Development Time
By trimming out unnecessary functionality right from the start, you can deliver projects faster. Think of Yagni as your programming cheat code; one up for productivity!
Saved Resources: Time, Energy, and Cost
Pulses of code here and there might seem tiny, but remember, oceans are made drop by drop. Every unneeded feature you add consumes precious resources that could have been utilized for other vital parts of your project.
Lower Costs for Changes
Think of it as only cooking the food you’ll eat and not an extra buffet. Any changes to the software are only made where necessary. Less cleanup, more time saved. Nifty, right?
Loading code snippet...
These are just some of the benefits that Yagni brings to the C# programming table. However, we must bear in mind to not misinterpret Yagni as an excuse to be lazy or avoid planning. As the saying goes, “Simplicity is the ultimate sophistication.” And that’s what Yagni offers – elegance through simplicity.
Practical Application of the Yagni Principle in C#
To truly grasp the power and scope of the Yagni principle, one must delve deep into its application in actual C# development. Let’s take a journey through Yagni’s practical application, offering you a deeper understanding by throwing in detailed examples, best practices, and even an 8-year old’s exceptional capacity for uncomplicated thought. All aboard the Yagni express!
Detailed Examples of applied Yagni Principle
Recall this simple calculator application done using Yagni.
Loading code snippet...
In this example, we anticipated creating a multitude of operation implementations (like Addition
, potentially Subtraction
, Multiplication
, and more). However, the user only needs an Add
function for now. So, instead of creating a complex operation system, we’ve defaulted to a simple Add
function, saving time and resources – a testament to the Yagni principle.
But what if we need a subtraction operation in the future? Let’s not worry about that right now. We’ll cross that bridge when (or if) we get to it.
Now picture this. You’re planning a birthday party for your boisterous 8-year old. You’ve been dreaming about an extravagant show with clowns, magicians, and a grand castle-shaped cake. But here’s the kicker – your kiddo just wants a few friends over, his favorite pizza, and a simple Lego-shaped cake. He isn’t interested in the grandeur you were envisioning.
Can you see the Yagni principle in action? By avoiding what you ‘think’ might be needed and concentrating on what’s ‘really’ required, Yagni can save you considerable effort and resources.
Let’s apply this to another C# scenario. Imagine you are building a monthly budget tracker. Now, you may ‘think’ you will need an intricate system with many categories. But do you ‘know’ it? No.
Loading code snippet...
According to Yagni, at the moment, all you need is to track your expenses. So let’s keep it simple!
Loading code snippet...
See? A testimony to the wonders of the Yagni principle!
Best Practices for applying Yagni in C#
Despite its simplicity, the Yagni principle isn’t a carte blanche to do sloppy work. In fact, good software design is at the heart of practicing Yagni. Adopting the following best practices will help you apply Yagni efficiently:
- Understand requirements clearly: You can’t decide what’s unneeded if you don’t understand what’s needed. Just as you can’t bake a cake without knowing the recipe, you can’t use Yagni without a clear understanding of project requirements.
- Use a test-first approach: This ensures you build what’s necessary to pass the tests, nothing more, nothing less. It acts as a lighthouse, providing clarity on what’s required presently.
Loading code snippet...
- Regularly refactor: This allows you to constantly simplify and improve the structure of the code, maintaining the application’s design integrity while adhering to Yagni.
Loading code snippet...
After assessing, you find lastExpense
not used anywhere. So, you refactor the code by removing it. The result, leaner code because, you guessed it, Yagni!
Loading code snippet...
These strategies make Yagni quite an ace up every C# programmer’s sleeve. It might seem counterintuitive, but sometimes doing less (avoiding what’s unnecessary) equates to achieving more. And that’s Yagni in a nutshell!
Potential Pitfalls and Misunderstandings of the Yagni Principle
Before we dive deep into busting common myths and highlighting the possible risks of the Yagni principle, let’s remember that even the most efficient tool can cause a mess if not used properly, right? Time to eliminate misconceptions and prevent potential pitfalls when implementing Yagni in your coding adventures!
Common misconceptions about the Yagni Principle
Often, developers equate “avoiding unnecessary complication” with “avoiding all sorts of planning.” That’s like equating “eating sweets judiciously” with “no sweets at all”. That’s absurd, isn’t it? Here are some prevalent misconceptions breeding in the nooks and crannies of the developer community:
- Yagni means no planning necessary: Yagni doesn’t mean skipping on plan or design — it suggests that you don’t overcomplicate by overanticipating. It’s like going to a party — you need to plan what to wear but don’t need to buy a new outfit for every possible future party.
Loading code snippet...
- Yagni promotes poor coding standards: Yagni focuses on “not writing unnecessary code now”, not “writing bad necessary code now”. The former lessens the code weight while the latter crashes it!
Risks of misusing Yagni principle in C#
Just like using a chainsaw to cut bread, a misapplied Yagni principle can do more damage than good. Let’s look at the risks of misusing this principle. But before we do that, remember that even the coolest superhero (Yagni, in our case) can go awry without proper guidelines.
- Lack of planning and forethought: Leveraging Yagni doesn’t mean abandoning plans hook, line, and sinker. It’s all about finding that sweet spot between “over-engineering for future unknown” and “engineering for now known”.
Loading code snippet...
- Scraping necessary functionality: The Yagni principle advises against adding anticipatory functionally, not axing down necessary ones. Swinging Yagni as an ax can lead to removal of relevant features, making your code a hollow shell.
- Ignoring important potential problems: Taking Yagni to the extreme can cause you to overlook crucial issues that could mushroom into bigger problems in the future. It’s like ignoring a small leak in your boat because it’s not causing your boat to sink yet. But remember what happens next in the story?
Applying principles is a strategic affair. Genuine understanding and careful application are what transform it from just text to a powerful tool. Do remember, Yagni is not a shield to hide behind for not making an appropriate system, but a lens to focus on what’s necessary.
The correlation between YAGNI, KISS and DRY in C#
Let’s delve a little deeper into the synergy between YAGNI, KISS, and DRY principles. It’s like the Avengers of programming principles, each one unique yet cohesively working towards clean and efficient code.
How Yagni correlates with KISS (Keep it simple, stupid)
We’ve often heard the term “KISS” thrown around in programming frameworks. Much like Yagni, KISS encourages shedding unnecessary complexity. It’s like when you’re baking a cake. You could create an extravagant multi-tier cake with fancy icing and decorations, but if you’re just craving a chocolate cake, isn’t it easier and quicker to bake a simple chocolate cake with a chocolate ganache?
Loading code snippet...
Just like Yagni, KISS eliminates features or steps that could complicate matters down the line.
Connection between Yagni and DRY (Don’t Repeat Yourself) principles
DRY philosophy pushes the concept of non-repetition. It promotes creating methods that can perform functionality rather than repeating the same code in different places. It’s kind of like a food processor. You don’t need separate gadgets to chop, puree, and dice when one food processor can perform all the tasks.
That’s where DRY marries Yagni. By creating functions only when required, we prevent duplication and keep our code DRY.
Loading code snippet...
By promoting DRY application, Yagni also discourages unnecessary functions and promotes reusable, clean code.
Comparing YAGNI with other Principles in C# Programming
Like to compare and contrast principles? Let’s take a look at how YAGNI stacks up against Gold Plating and Over-engineering.
YAGNI vs. Gold plating
Gold plating is the software developer’s version of showing off. It refers to adding features that weren’t requested, in the hope it will impress users or clients. But remember the tale of Midas whose touch turned everything to gold? If you remember, it didn’t go so well for him, right? Much like Yagni advises, what’s shiny and golden might not always be what’s needed.
Loading code snippet...
YAGNI reminds us that we don’t need to dip our code in gold for it to shine. Clear, simplified, and readable code holds its own kind of beauty.
YAGNI vs. Over-engineering
Over-engineering is when we create a Haunted Mansion for the job of a simple tool shed! YAGNI, on the other hand, warns us against unfounded complexity and excess arsenals.
Loading code snippet...
Batman might be all about gadgets and tech, but when it comes to coding, following the YAGNI principle can keep our systems lean, efficient, and roomy for any Gotham C# city we need to save!
Conclusion
The future is bright for the Yagni principle, as ‘simplicity’ becomes the mantra of the tech world. It has been, and will continue to be, an essential part of efficient coding and clean design.
Key takeaways for using Yagni principle effectively
So, what’s the secret recipe for using Yagni?
- Keep it simple
- Don’t anticipate unnecessary complexity
- Don’t build something until you need it
- Don’t listen to the little voice saying “But what if…”
Now, don’t you feel ready to harness the Yagni power in your C# journey?