C# KISS Principle (Keep It Simple, Stupid!)
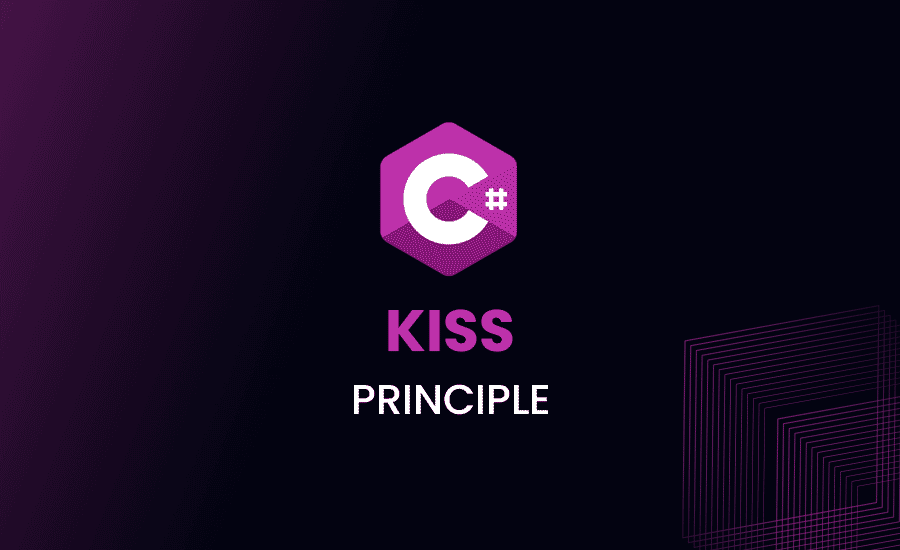
C# developers, have you ever wondered if there’s a magic secret sauce to writing well-organized, easy-to-understand, and maintainable code? Allow me to introduce the KISS principle. In the next sections, we’ll dive into this fascinating concept, looking at what it is, how it can benefit your C# projects, and how to properly incorporate it into your coding routine. Ready to kiss complexity goodbye? Let’s dive in!
The Philosophy behind the KISS principle
Somewhere along your programming journey, you have likely come across the term ‘KISS’. It’s an acronym for ‘Keep It Simple, Stupid’, and it’s a guiding principle that can revolutionize the way you approach your code.
Defining the KISS principle
The KISS principle advocates for simplicity. It encourages programmers to avoid unnecessary complexity and instead, stick to simplicity whenever possible. But why is that important, you ask?
Origin and Evolution of the KISS principle
Believe it or not, the KISS principle dates back to the 1960s, a brainchild of the U.S. Navy intending to ensure that things remain simple and easy to repair or operate. And it’s been making waves in the programming world ever since!
Importance of the KISS Principle in Software Development
Simple codes are more manageable, less prone to bugs, and more open to modifications. A simpler design means lower costs and faster delivery times. And as a developer, who doesn’t like that?
The KISS Principle in C# Programming
Now that we’ve established the foundation, let’s see how we can apply this principle in our C# coding practice. You might be surprised how much smoother your programming life can be!
Understanding the Application of KISS Principle in C#
The implementation of the KISS principle in C# involves a simple approach: producing code that is easy to read, understand, and maintain. It might look something like this:
Loading code snippet...
As you can see above, the first example overcomplicates the method with additional conditions that might not be necessary for this task. The second example is a much simpler implementation.
Benefits of Applying the KISS Principle in C# Programming
When you adhere to the KISS principle, a number of perks come your way:
- Your code becomes easier to understand, even by junior developers or those not familiar with the project.
- Debugging becomes less of a headache because it is easier to locate and fix bugs in simpler code.
- Maintenance time is reduced, making it easier to implement new features or enhancements.
Practical Examples of KISS Principle in C#
Let’s consider a contrived example where, instead of a simple loop to iterate over data, one might be tempted to use fancy terms like recursion. However, a loop would be much simpler, easier to understand, and efficient.
Loading code snippet...
In the first example, the recursive method may look fancy, but it’s overkill for such a simple task and can also lead to a stack overflow with large inputs. The loop in the second example does the same task in a much simpler manner.
Incorporating the KISS Principle into your C# Project
Time to roll up your sleeves and dive into the art of incorporating the KISS principle into your very own C# projects. In the sections that follow, we’ll explore a variety of strategies, tips, and tricks to help you identify code complexity and simplify it, all in the name of KISS. So grab your programmer’s toolbox and let’s start cracking this task!
Strategies to Keep your C# Program Simple with KISS
Before we tackle the complexities within your code, let’s equip ourselves with the tools of the trade. To make your code KISS-friendly, keep the following strategies in mind:
- Reduce Nesting: Nesting makes your code look like a Russian nesting doll – hard to decipher what’s inside and where it ends. Reduce nesting and your code will breathe easier. A clever use of ‘return’ or breaking your code into smaller, reusable functions can help.
Look at the below example:
Loading code snippet...
In this example, by avoiding deep nesting and using early returns, the code becomes easier to read and understand.
- Avoid Over-optimization: Premature optimization is the root of many headaches in programming. Remember the KISS principle and write code that is easy to understand. Don’t over-optimize at the expense of readability, unless the performance bottleneck has been proven by measurable data.
- Use Descriptive Naming: Let names tell a story about your code. Be it a variable, class, or method; a well-chosen name can make your code much more readable. Just remember; clarity over brevity.
Imagine a method to find a player’s score in a game. Which one from the below examples do you think is better?
Loading code snippet...
How to Identify Code Complexity in your C# Project
Noticing complexity in your code is like finding a needle in a haystack. But fear not! Here are some flags which can point out if your code needs a KISS makeover:
- Lengthy code: While length does not directly correlate with complexity, a method or class that’s hundreds of lines long is a possible sign of over-complication. Try to make your methods, classes and structs compact and focussed.
- Deeply nested structures: While loops, conditionals, and switches nested within each other may seem like you’re solving a fun puzzle, they make your code harder to read and maintain. A flat structure can simplify your code significantly.
- Replicated Code: Code duplication, also known as “copy-paste” programming, can make your code base bloated and makes updates far more painstaking. A DRY (Don’t Repeat Yourself) approach is always preferable.
Now that we know what to look out for, what can we do about it?
Ways to Simplify Complex Code in C# using KISS Principle
Ensuring that your code adheres to the KISS principle requires discipline and dedication. Here are some ways that you can start streamlining your codebase:
- Refactor your code: Polishing and cleaning your code continuously is called refactoring. This practice aims to improve your code without altering how it functions.
Loading code snippet...
Remember, it’s like cleaning your room. Would you rather live in a cluttered mess or a neat and orderly space?
- Use comments judiciously: Comments can be super helpful explanations in your code, but they are not a remedy for complex code. Ill-placed or wrong comments can cause more confusion than they solve. Hence, let your code talk more than your comments.
- Leverage C# features: C# has many fantastic features aimed at writing clean, simple code. You could use properties instead of getters and setters,
var
keyword to let the compiler infer the type, string interpolation for cleaner string formatting, null-conditional operators (?. and ?[]) to access members and elements only when the receiver is not-null, avoiding NullReferenceException, and much more!
There you have it! By applying these strategies, your code will start to become more readable, maintainable, and most importantly, KISS-friendly. So let the simplification begin! The road may seem long, but remember, as the famous proverb goes, every journey begins with a single step!
Common Mistakes C# Developers Make when Applying the KISS Principle
As with all principles, it’s crucial to correctly understand and correctly apply the KISS principle in your C# development. Misinterpretation or over-application can lead to potential stumbling blocks that may result in overly simplified code or, alternatively, unnecessary complexity—neither of which are productive. Let’s deep dive into these common pitfalls and understand how to avoid them.
Over-simplification Vs. KISS Principle in C#
Over-simplification often results from the mistaken belief that the KISS principle means getting rid of as much code as possible. While striving for simplicity is commendable, it’s important to note that a simplified solution should not lack clarity or create ambiguity.
Consider this illustrative example of a method that calculates a student’s final grade based on a collection of scores. An over-simplified approach might attempt to perform all calculations within a single method, leading to an overly dense and less maintainable code.
Loading code snippet...
In the bad practice example, the CalculateFinalGrade
function does multiple tasks—it calculates the average and determines the letter grade. Moreover, it isn’t efficient. The properly simplified version, in contrast, splits the tasks into two simple, understandable functions. This is easier to understand, debug, and maintain.
Here’s a real-world analogy: Imagine making a sandwich. Suppose all the steps from gathering the ingredients, prepping them, assembling the sandwich, and cleanup are all jumbled together. It would be chaotic, right?
Applying the KISS principle is like organizing these steps. One method to fetch the ingredients, another to prep them, yet another to assemble your sandwich, and finally one to clean up. Each task is simpler and easier to manage.
Misinterpretation of the KISS Principle in C#
Using the least amount of code possible does not necessarily achieve simplicity. The KISS principle isn’t about the number of lines of code, but about the clarity and maintainability of that code. The reason for this is straightforward: simple and clear code is easier for others to understand and modify.
Loading code snippet...
In the bad practice example, we are writing code to iterate through each character in the string and print it out. This might seem “simpler” because it avoids the Console.WriteLine
method. However, not only is this code longer, but it’s also harder to understand at first glance. The good practice example instead directly utilizes the Console.WriteLine
method, which is both shorter and clearer.
A real-world comparison would be taking a car apart and carrying each piece from one location to another individually, versus simply driving the car to the new location. The first is a gross misinterpretation of the KISS principle—even though it avoids using the “complex” mechanism of driving, it’s making the task much harder.
The second approach is a much better interpretation of the KISS principle. It’s simpler, even if you had to use the “complex” mechanism of driving.
The Role of the KISS Principle in Agile Development and SCRUM
In the effective synergy of Agile and SCRUM methodologies with the KISS principle emerges a powerful strategy for software development.
These methodologies not only complement each other but work to reinforce best programming practices, aiming for more maintainable and less defect-prone codes. In the imminent sections, we’ll delve deeper into how we can blend the KISS principle with Agile and SCRUM development to supercharge our C# programming.
Incorporating the KISS Principle in Agile Development with C#
Agile development, with its incremental and iterative approach, promotes flexibility and the ability to respond to changes. It’s all about producing working software in short sprints, and as such, the KISS principle proves instrumental here by encouraging simplicity and quicker, more efficient coding.
Let’s consider a situation where you are coding a feature for handling user preferences. These could be related to the application’s themes, notification settings, and so on.
A traditional approach might be to devise an elaborate preferences class with properties for every different setting. However, by sticking to the KISS principle, we should start with a simple class having only the most essential properties.
Loading code snippet...
In subsequent sprints, as new real preferences emerge, we gradually add them to the class. In this way, Agile and KISS wrap up together to reduce the initial complexity, resulting in leaner and more adaptable code.
Application of KISS Principle in SCRUM and C# Development
The SCRUM framework works wonders in incorporating the KISS principle in the development process. Being an Agile methodology, SCRUM’s iterative nature gives developers room for adjusting the product features along the way.
Given its self-organising team vigour, SCRUM accords developers the liberty of decisively shaping the code structure and design. It enables developers to engage their discretion in identifying unnecessary complexities and removing them, an essence of the KISS principle.
Typically, stories or use-case scenarios are developed with a SCRUM team as a guide to eliminate ambiguity and detail the features to be developed. But hold on a sec, how can we foster straightforwardness using the KISS principle? Here’s how:
Loading code snippet...
Can you glimpse the difference? When we break down the user stories into single fragments, coding becomes more responsive and less complicated, and importantly, adheres to the KISS principle!
Exploring the Relationship between the KISS principle and C# Best Practices
In the universe of best coding practices, the KISS principle is like a friendly guide, leading us towards clarity and simplicity. But it doesn’t work in isolation. Just as a concerto requires different instruments to create harmony, great code makes use of several guiding principles in sync. Buckle up, as we delve deeper into the intriguing interplay between the KISS principle and other C# best practices.
Comparing the KISS principle with Other C# Coding Standards and Principles
The world of C# programming isn’t all about complexity. In fact, some of its best practices are all about eradicating unnecessary complication!
DRY Principle
DRY, which stands for “Don’t Repeat Yourself,” discourages the duplication of code. Instead of copying and pasting code, DRY proposes the use of abstractions to avoid repetition. Consider this example:
Loading code snippet...
In the above example refactoring, the drawing code is simplified by taking in an abstraction of our shape. Now we can draw any shape implementing the IShape
interface!
SOLID Principles
SOLID stands for five design principles intended to make software designs more understandable, flexible, and maintainable. They’re more like the secret recipe that gives your code that flavor of professionalism.
Quite often, these principles align beautifully with KISS. For instance, the Single Responsibility Principle (SRP), which states that a class should have only one reason to change, promotes simplicity in design.
The Interplay between the KISS principle and C# Best Practices
While the KISS principle advises us to keep things simple and avoid unnecessary complexity, other principles like DRY, SOLID, and YAGNI help to keep our code clean, maintainable, and free of unnecessary duplication. It’s like having a toolbox filled with different tools for specific problems.
How KISS Interacts with DRY
Consider you’re working on a project where you find yourself copying and pasting segments of code throughout the project. While you might think this speeds things up, this could lead to trouble down the road (bugs, anyone?). Applying the DRY principle, we refactor repeated code into a single reusable method. This not only simplifies the code but also makes it easier to maintain. Viola, we just applied KISS!
Loading code snippet...
In this example, you can see how DRY aids KISS by eliminating the repetition and making the code cleaner and simpler.
KISS and SOLID: A Match Made in Heaven
Remember the SOLID principles? They can often work in perfect harmony with KISS to give you code that’s easy to maintain and understand.
For instance, consider the Interface Segregation Principle (ISP). It recommends creating specialized interfaces so that clients only have to know about the methods that are of interest to them. In essence, this removes any unnecessary complexity from the client’s perspective, thereby adhering to the KISS principle!
So, as we see, the KISS principle isn’t a stand-alone concept. In its alliance with other principles like DRY, YAGNI, and SOLID, it can help us achieve a synergy that produces clean, maintainable, and high-quality C# code. Isn’t that a sight to behold in your code editor?
Considerations when Applying the KISS Principle in C#
Now that we’ve seen the many advantages of the KISS principle, it’s time to venture into the less explored territories – the caveats. The application of KISS, like many other practices in programming, requires careful planning and mindful execution. Let’s see some instances where we might need to go a step further than just keeping it simple.
Balancing Simplicity and Performance in C# Programming
Striking a balance between simplicity and performance in C# can often feel like a tug-of-war. With the KISS principle, we are urged to keep things simple and avoid unnecessary complexity. However, performance considerations in certain situations may require optimized and potentially more complex solutions. These scenarios raise a question. How do we keep it simple and performant at the same time?
Loading code snippet...
The simplicity of the linear search is enticing – it’s easy to read and understand. However, it may not be the most efficient approach, particularly for large data sets or frequently executed searches. On the other hand, the binary search is less intuitive but offers a remarkable performance boost on sorted data.
That leaves us with the task of identifying what is essential complexity, i.e., complexity necessary to meet performance requirements, and what is accidental complexity, that can and should be eliminated while adhering to the KISS principle.
Understanding when to go Beyond the KISS Principle in C#
The KISS principle is an excellent guide, but it’s not a strict order. There are instances where we need to embrace a certain degree of complexity to meet our software’s demands. Yes, we want to avoid turning our code into a brainteaser, but avoiding essential complexity can cripple our program’s efficiency or functionality.
Take, for example, the use of threading and parallel programming in C#. Multithreaded applications are admittedly more complex than single-threaded ones. However, they provide us with the advantage of utilizing system resources more efficiently, significantly speeding up computation time in specific situations.
Loading code snippet...
The single-threaded process is straightforward; execute the first process, wait for it to finish, then run the second. However, this can lead to wasted time if ‘ThisProcess()’ and ‘ThatProcess()’ are complex and lengthy but do not depend on each other. In contrast, a multi-threaded program launches both processes concurrently.
Even though we added a layer of complexity in multi-threaded programming, it’s evident that there are real-world situations where we need to extend beyond the basic KISS principle. After all, no principle is an island. Maintaining an equilibrium between simplicity for the human reader and functionality for the machine reader is a mark of a skilled developer.
So, in the end, KISS is not so much about ditching complexity altogether but rather knowing when to embrace simplicity and when to smartly handle necessary complexity. That’s the real art of programming, isn’t it?
Conclusion: Sustaining Software Development Efficiency with the KISS Principle in C#
And there you have it! We’ve journeyed through the world of the KISS principle in C#, discovering its benefits, techniques, and even tackling some common misunderstandings along the way. So, are you ready to kiss (pun totally intended) unnecessary complexity goodbye and welcome a wave of efficiency into your code?
Reflecting on the Impact of KISS Principle on Your C# Projects
The KISS principle isn’t just about making code simpler; it’s about increasing its maintainability, readability, and testing ease. By now, you have discovered how the KISS principle has the potency to impact not only your C# projects but also your overall coding philosophy.
So, what do you think? Isn’t it time to welcome the KISS principle into your coding arsenal and bid farewell to unnecessary complexity? It’s time to keep it simple, fellow developers!