XML Interview Questions and Answers
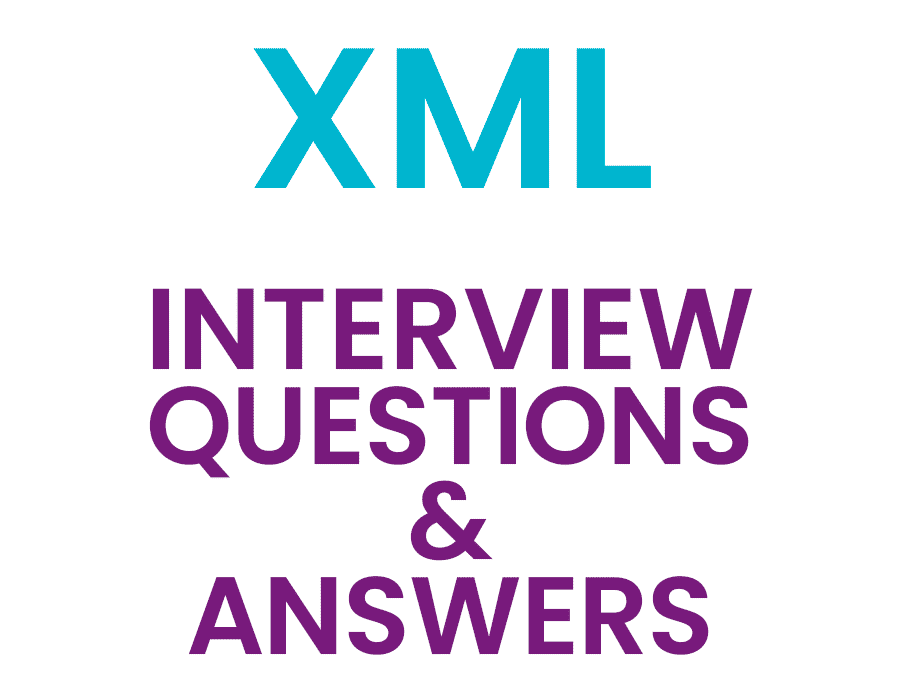
If you’re preparing for a software developer role that involves working with C# and XML, you’re in the right place. This comprehensive guide will provide you with essential xml interview questions c# developers should know, regardless of whether you’re a beginner or an expert in the field.
These questions cover a wide variety of topics, including processing large XML documents, XmlReader, XmlWriter, serialization, deserialization, XML encryption, XML namespaces, XPath navigator, XML validation techniques, and much more. So, let’s dive in and explore these xml interview questions c# developers must be familiar with to ace that interview and enhance those skills!
In C# XML, what are the considerations one must take into account when parsing very large XML documents (larger than available memory) for optimal performance and minimal memory consumption?
Answer
When dealing with very large XML documents, it is essential to consider several factors to optimize performance and minimize memory consumption:
- Use streaming: Instead of loading the entire document into memory, use an XmlReader or XmlTextReader to read the XML as a stream. This significantly reduces memory consumption since only a portion of the XML is loaded and processed at any given time.
- Avoid DOM: Do not use the Document Object Model (DOM) to parse large XML files, as this approach loads the entire XML tree into memory, which can be inefficient and consume excessive amounts of memory.
- Use XPath with caution: While XPath can be helpful in navigating and querying XML data, it can also consume large amounts of memory when processing large XML documents, as it may require loading the entire document into memory. Use it carefully in those situations.
- Consider compression: If the XML document is significantly large, consider compressing it before processing it in C#. This can help reduce memory usage, as well as the time required to transfer the document between systems or over a network.
- Optimize memory allocation: When working with large amounts of data, be mindful of object allocations, reference management, and garbage collection to minimize memory overhead and improve performance.
Explain the difference between an XmlReader and an XmlWriter in the context of C#. Which one is preferable for performance-critical scenarios, and why?
Answer
XmlReader
and XmlWriter
are classes in the C# language used for XML processing:
- XmlReader: This is a forward-only, read-only, streaming XML parser. It reads an XML document sequentially from a stream, providing a high-performance, low-memory-footprint solution for processing large XML documents. XmlReader does not support editing, meaning it cannot modify the underlying XML data.
- XmlWriter: This is a forward-only, write-only, streaming XML generator. It allows you to create and output XML data sequentially, minimizing memory consumption and providing high-performance writing capabilities for applications generating large XML documents.
In performance-critical scenarios, the preferable choice between the two depends on the task at hand:
- For reading large XML documents,
XmlReader
is the preferable choice due to its low memory footprint and efficient, streaming approach. - For writing large XML documents,
XmlWriter
is the better option, as it enables you to generate XML data sequentially, minimizing memory usage and enhancing performance.
How can you implement encryption and decryption of XML data using XML Encryption and XML Digital Signature standards in C#?
Answer
XML Encryption and XML Digital Signature standards can be implemented in C# using the System.Security.Cryptography.Xml
namespace, which provides classes for encryption, decryption, and signing of XML data:
- XML Encryption: To encrypt an XML element, use the
EncryptedXml
class to perform the encryption process. Create an instance of this class, along with an appropriate symmetric or asymmetric algorithm (e.g.,Aes
,RSA
) for the actual encryption. Call theEncrypt
method to transform the target XML element into anEncryptedData
instance. Replace the original element with the encrypted element in the XML document. To decrypt the data, reverse the process by extracting theEncryptedData
instance from the encrypted XML, creating an instance ofEncryptedXml
and using the related cipher algorithm with the correct key to call theDecrypt
method. Replace the encrypted XML element with the decrypted content. - XML Digital Signature: Use the
SignedXml
class to sign an XML document or verify the signature of a signed XML document. First, create an instance ofSignedXml
and provide the XML document to be signed. Create an instance of the appropriate asymmetric signature algorithm, such asRSACryptoServiceProvider
orDSACryptoServiceProvider
, and set theSigningKey
property of theSignedXml
instance. Create a reference to the XML element that needs to be signed, compute the signature using theComputeSignature
method and add the computed signature to the XML document. To verify the signature, load the signed XML document, extract the signature element, create a new instance ofSignedXml
, and use theCheckSignature
method to validate the signature using the public key of the signing party.
Describe the process of transforming and querying XML data using LINQ to XML in C#. Provide an example where you think this technique is particularly useful.
Answer
LINQ to XML is a modern approach for querying and transforming XML data in C#, providing a convenient and expressive syntax. It uses the System.Xml.Linq
namespace, and key classes like XElement
, XAttribute
, and XDocument
for XML representation.
Transforming and querying XML data using LINQ to XML involves the following steps:
- Load the XML data: XML data can be loaded from a string, file, or stream using the
XElement.Load
orXDocument.Load
methods. - Perform the query: Use LINQ query expressions to filter, sort, and manipulate the XML data, accessing elements and attributes using the
XElement
andXAttribute
classes. - Transform the data: Modify the XML data using LINQ to XML’s functional construction techniques, such as creating new elements, modifying attributes, or deleting elements.
- Output the result: Serialize the transformed XML data back to a string, file, or stream using the
ToString
method orSave
methods available onXElement
andXDocument
.
A scenario where LINQ to XML is particularly useful is when filtering and transforming complex XML data for display or reporting purposes:
Loading code snippet...
In this example, LINQ to XML is used to filter, select, and transform employee data from an input XML file, creating a new XML file with the processed data.
How would you handle XML namespaces in C# while working with an XML document that includes multiple namespaces? Provide a code snippet to demonstrate the best practice.
Answer
Handling XML namespaces in C# when working with an XML document that includes multiple namespaces can be done using the XNamespace
class and its facilities. XNamespace
allows you to define and manage XML namespaces in a convenient and efficient manner.
Here is a code snippet demonstrating the handling of multiple XML namespaces:
Loading code snippet...
In this code snippet, XNamespace
objects are created for two different namespaces. These objects are then used to create an XML document containing elements with the defined namespaces. The same objects are later used to query elements associated with each namespace. Using XNamespace
allows for a more elegant, maintainable, and readable code while working with multiple XML namespaces.
Now that we’ve explored XML namespaces and their usage in C#, let’s move on to discuss the XPathNavigator and XPathExpression classes.
These classes play a crucial role in efficiently navigating and querying XML data in C#.
Explain how to work with XPathNavigator and XPathExpression classes in C# to navigate and query XML data efficiently.
Answer
XPathNavigator and XPathExpression are classes in the System.Xml.XPath
namespace that provide an efficient and flexible way to navigate and query XML data using XPath expressions in C#.
To work with XPathNavigator and XPathExpression:
- Load XML data: Load an XML document using the
XmlDocument
class from theSystem.Xml
namespace:
Loading code snippet...
- Create an XPathNavigator: Create an XPathNavigator instance for the loaded XML document by calling the
CreateNavigator
method:
Loading code snippet...
- Prepare an XPathExpression: Compile an XPath expression using the
Compile
method of the XPathNavigator instance:
Loading code snippet...
- Evaluate the XPathExpression: Use any of the evaluate methods provided by the XPathNavigator class to query the XML data, such as
Evaluate
,Select
, orSelectSingleNode
:
Loading code snippet...
- Iterate and process the results: Iterate over the resulting XPathNodeIterator to process the selected nodes:
Loading code snippet...
Using the XPathNavigator and XPathExpression classes allows for efficient querying of XML data with the added benefit of using precompiled XPath expressions for repeated queries.
What strategies would you use for error handling and validation in C# when working with XML data, specifically in context of XML Schema Definition (XSD) and Document Type Definition (DTD)?
Answer
Error handling and validation with XML data in C# typically involve the following strategies:
- XSD validation: Validate the XML document against an XML Schema Definition (XSD) using the
XmlReaderSettings
class:
- Create an
XmlReaderSettings
instance - Set
ValidationType
property toValidationType.Schema
- Add the required schema(s) using the
Schemas.Add
method - Attach an event handler to the
ValidationEventHandler
to capture and handle any validation errors - Create an
XmlReader
instance usingXmlReader.Create
method with theXmlReaderSettings
instance - Load and read the XML data using the
XmlReader
- DTD validation: Validate the XML document against a Document Type Definition (DTD) using the following steps:
- Create an
XmlReaderSettings
instance - Set
DtdProcessing
property toDtdProcessing.Parse
- Attach an event handler to the
ValidationEventHandler
to capture and handle any validation errors - Create an
XmlReader
instance usingXmlReader.Create
method with theXmlReaderSettings
instance - Load and read the XML data using the
XmlReader
- statements: Enclose XML processing code within
try-catch
blocks to handle any exceptions that might occur during XML parsing, querying, or validation. Catch and handle exceptions such asXmlException
,FileNotFoundException
, orXPathException
. - Validation event handling: Implement custom logic within validation event handlers to determine the desired behavior when validation errors are encountered. This can include logging errors, aborting processing, or continuing with a default or alternative value.
By employing these strategies, you can ensure that the XML data is being processed and validated according to the defined rules, and that any errors are caught and handled appropriately.
Explain the mechanism behind XML serialization and deserialization in C#. What precautions should be taken to maintain compatibility between serialized and deserialized objects?
Answer
XML serialization and deserialization in C# involve converting objects into XML format and reconstructing objects from XML data respectively, using classes in the System.Xml.Serialization
namespace, such as XmlSerializer
.
Mechanism:
- Serialization: To serialize an object to XML data, create an instance of
XmlSerializer
for the object type, and call itsSerialize
method, providing the destination for the XML data (e.g., a file stream or text writer). - Deserialization: To deserialize XML data back into an object, create an instance of
XmlSerializer
for the target object type, and call itsDeserialize
method, providing the source of the XML data (e.g., a file stream or a text reader).
Precautions:
To maintain compatibility between serialized and deserialized objects:
- Apply the
[Serializable]
and[XmlRoot]
attributes to classes that need to be serialized and deserialized. - Use attributes from the
System.Xml.Serialization
namespace, such as[XmlAttribute]
,[XmlElement]
, and[XmlArray]
, to control the XML structure representation of objects during serialization and deserialization. - Avoid changing the names and types of serialized properties and fields, as this can break the deserialization process. If renaming is required, use the
OldName
property of the[XmlElement]
attribute to maintain backward compatibility. - When adding new properties or fields, assign default values if necessary, to ensure that deserialization of previously serialized data will not result in null or incorrect values.
- Use versioning techniques (e.g., incrementing a version number in the XML data or in the serialized class) to identify different versions of the serialized data and handle them appropriately during deserialization.
- Ensure that all referenced and required assemblies are available during deserialization; otherwise,
TypeLoadException
orFileNotFoundException
may occur. - Test the serialization and deserialization process for various scenarios and object states to ensure data integrity and compatibility.
By following these precautions, you can maintain compatibility between serialized and deserialized objects, ensuring a consistent and predictable serialization/deserialization process.
How would you implement XML data streaming in C# to communicate with remote systems over web services? Describe the process while keeping memory usage and performance in mind.
Answer
Implementing XML data streaming in C# to communicate with remote systems over web services involves the following steps:
- Choose a protocol: Select an appropriate web services protocol, such as SOAP or REST, based on the requirements of the system you are communicating with.
- Use streaming-capable classes: Utilize streaming-friendly classes like
XmlReader
andXmlWriter
for reading and writing XML data, as they provide a forward-only, streaming approach that consumes less memory and increases performance. - Employ buffered streams: Use buffered stream classes (
BufferedStream
,StreamReader
,StreamWriter
) when reading from or writing to a network stream, as buffering can increase performance when working with small chunks of data. - Asynchronous communication: To prevent blocking, use asynchronous methods to read and write XML data over the network. This allows for better responsiveness and scalability when communicating with remote systems.
- XML compression: If XML data transmission size is a concern, consider compressing the XML data using classes from the
System.IO.Compression
namespace (e.g.,GZipStream
,DeflateStream
) to reduce transmission time and save bandwidth. - Streaming large attachments: For web services that require streaming of large attachments like binary files, use protocols and methodologies that support streaming, such as MTOM (Message Transmission Optimization Mechanism) for SOAP or chunked transfer encoding for REST.
- Deserialize directly from the streaming source: If possible, deserialize XML data directly from the web service response stream, avoiding intermediate storage and reducing memory usage.
Here’s an example of using XmlReader with a REST web service that returns an XML response:
Loading code snippet...
By following these steps and using the appropriate classes and techniques, you can efficiently implement XML data streaming in C# for communication with remote systems over web services, while keeping memory usage and performance in mind.
How can you integrate C# XML processing with database systems like SQL Server and MySQL to store and retrieve XML data efficiently?
Answer
Integrating C# XML processing with database systems like SQL Server and MySQL can be done using the following methods:
- Store XML as text: One approach is to store XML data as a text data type in the database system (e.g.,
nvarchar(max)
for SQL Server orTEXT
/LONGTEXT
for MySQL). This method may be less efficient for querying and manipulating the XML data within the database, but it allows for simple storage and retrieval. To store and retrieve XML data as text, use regular ADO.NET or an ORM library (like Entity Framework) to insert, update, and retrieve the XML data as a string or as aSystem.Xml.Linq.XElement
object.
- Use database-specific XML data types: Use an XML data type when the database system supports it, like the
xml
data type in SQL Server. This enables the efficient storage, querying, and manipulation of the XML data within the database, using built-in XML functions and XPath expressions. To store and retrieve XML data using database-specific XML data types, you can:
- Utilize ADO.NET with specialized
SqlParameter
andDbParameter
instances for working with the XML data types (e.g.,SqlDbType.Xml
for SQL Server). - Configure the ORM library (like Entity Framework) to map the object properties to the XML data type.
- Transform and query XML data in the database: When using database systems with support for XML querying and manipulation, such as SQL Server or MySQL, it is possible to use built-in XML functions of the database systems for performing some XML processing tasks directly within the database:
- SQL Server: Use XQuery expressions and built-in functions like
query()
,value()
,nodes()
, ormodify()
for querying, extracting, and modifying XML data. - MySQL: Use built-in XML functions like
ExtractValue()
,UpdateXML()
, orXmlType()
for querying and manipulating XML data.
- Optimize indexing: To improve query performance with XML data in the database, consider using specialized indexing features of the database system, such as XML indexes in SQL Server.
By following these approaches, you can effectively store, retrieve, and process XML data within a database system like SQL Server or MySQL in C#.
Having explored the integration of C# XML processing with the database systems like SQL Server and MySQL, let us now shift our focus to manipulating XML data using the DOM (Document Object Model) approach in C#.
We will also discuss its limitations and benefits compared to other XML processing techniques.
Explain how XML data can be manipulated using C# and the DOM (Document Object Model) approach. What are the limitations and benefits of DOM compared to other XML processing techniques?
Answer
The Document Object Model (DOM) is an in-memory representation of an XML document and provides a tree-based structure for manipulation and traversal of XML data. In C#, the DOM approach is implemented using the System.Xml
namespace, with key classes like XmlDocument
, XmlNode
, and XmlElement
.
Manipulation process:
- Load an XML document into an
XmlDocument
object usingLoad()
orLoadXml()
methods. - Access XML elements and attributes using
XmlNode
,XmlElement
, andXmlAttribute
classes. - Modify the XML document by adding, updating, or removing elements and attributes using methods like
AppendChild()
,InsertBefore()
,RemoveChild()
, orReplaceChild()
. - Save the modified XML document using the
Save()
method of theXmlDocument
class.
Limitations:
- Memory-intensive: DOM loads the entire XML document into memory, making it inefficient for processing large XML files.
- Forward-only or streaming access is not supported: Unlike
XmlReader
orXmlWriter
, the DOM does not provide a forward-only, read-only or write-only, streaming access.
Benefits:
- Easy to navigate: DOM enables easy navigation to any part of the XML document in any direction, using the tree-based structure.
- Simplified querying and manipulation: DOM provides a high-level API, making querying and manipulation of XML data more intuitive compared to using an
XmlReader
orXmlWriter
. - In-memory operations: DOM allows for complex in-memory processing of XML data, which can be beneficial for certain tasks and transformations.
How can you work with advanced XML concepts like XML Inclusions (XInclude) and Canonical XML in C#? Provide examples where these features may be required.
Answer
XML Inclusions (XInclude):
XML Inclusions (XInclude) provides a way to include parts of other XML documents within an XML document by referencing them. In C#, working with XInclude can be done using the System.Xml
and System.Xml.Linq
namespaces.
Example scenario:
- You have a large configuration file where different sections are maintained in separate XML files. XInclude can be used to aggregate all sections into a single configuration file in XML format.
Usage in C#:
Use the XDocument
class from the System.Xml.Linq
namespace to parse the XML document with XInclude references:
Loading code snippet...
Canonical XML:
Canonical XML is a specific serialization format for XML documents, aiming to consistently represent logically equivalent XML documents in the same canonical form. This can be useful when comparing or signing XML documents. In C#, working with Canonical XML can be done using the System.Security.Cryptography.Xml.Pipeline
class from the System.Security.Cryptography.Xml
namespace.
Example scenario:
- You need to digitally sign an XML document as part of a web service protocol like SAML or WS-Security. Canonical XML can be used to ensure a consistent representation before signing and for signature validation.
Usage in C#:
Create a Pipeline
instance and configure its settings to apply canonicalization. Then, process the input XML:
Loading code snippet...
Working with advanced XML concepts like XML Inclusions and Canonical XML in C# requires using the appropriate classes, methods, and configurations, depending on the specific use case.
Describe the difference between XmlTextReader and XmlValidatingReader classes in C#. When should developers prefer one over the other?
Answer
XmlTextReader
and XmlValidatingReader
are classes in the C# language used for XML processing with distinct purposes:
- XmlTextReader: This class provides a forward-only, read-only, streaming XML parser. It is designed for high-performance and low-memory-footprint XML parsing, and it does not perform any validation of the XML content during parsing.
- XmlValidatingReader: This class is a wrapper around another
XmlReader
(usually anXmlTextReader
) and provides additional validation features during parsing. It can validate an XML document against Document Type Definitions (DTDs), XML Schemas (XSDs), or other schema types, and report validation errors and warnings through theValidationEventHandler
event.
Developers should prefer one over the other depending on their requirements:
- Use XmlTextReader when the primary focus is on high-performance, low-memory-footprint parsing, and there is no need to perform validation during parsing.
- Use XmlValidatingReader when validation against DTD, XSD, or other schema types is required during XML parsing, and you need to capture and handle validation errors.
What are some best practices for using C# to build a high-performance XML processing service with multi-threading and asynchronous operations?
Answer
To build a high-performance XML processing service with multi-threading and asynchronous operations in C#, follow these best practices:
- Use efficient XML classes: Prefer
XmlReader
for reading large XML data, as it provides a forward-only, read-only, streaming access that is memory-efficient and fast. For writing large XML documents, useXmlWriter
. - Work asynchronously: Use
async
/await
with asynchronous methods when reading and writing XML data, particularly when dealing with I/O-bound operations, like reading from or writing to files or network streams. - Parallelize CPU-bound tasks: If the XML processing involves CPU-intensive tasks, consider parallelizing the work using the
Parallel
orPLINQ
libraries, or the Task Parallel Library (TPL). - Optimize memory allocation: Be mindful of object allocations, reference management, and garbage collection to minimize memory overhead.
- Use appropriate synchronization mechanisms: When multi-threading and asynchronous operations are involved, ensure proper use of synchronization primitives like
lock
,SemaphoreSlim
,Mutex
, orMonitor
to protect shared resources and avoid common concurrency issues. - XML processing within the database: If using a database like SQL Server or MySQL, leverage the built-in XML processing capabilities of these databases to optimize query and manipulation tasks.
- Throttling: Implement proper throttling mechanisms to prevent excessive resource usage and maintain stability under heavy load.
- Caching: Use caching strategies to store and retrieve frequently accessed XML data or partial results, to reduce computation and I/O overhead.
- Load-balancing and task distribution: Distribute XML processing tasks across multiple processing units or servers, as required, to achieve better performance and resilience.
- Optimize indexing for DB XML storage: When storing XML data in a database, make use of specialized indexing features, like XML indexes, to improve query performance.
- Profile and optimize: Regularly profile and monitor the performance of the XML processing service, and optimize the code and resources accordingly based on the findings from profiling.
By following these best practices, you can build a high-performance, multi-threaded, and asynchronous XML processing service in C# that can handle large volumes of XML data efficiently.
How do you create and apply XSLT (Extensible Stylesheet Language Transformations) to an XML document in C# while ensuring optimal performance?
Answer
XSLT (Extensible Stylesheet Language Transformations) is an XML-based language used for transforming XML documents into other formats, such as HTML, plain text, or other XML structures. In C#, you can create and apply XSLT transformations using the System.Xml.Xsl
namespace, with key classes like XslCompiledTransform
.
To create and apply XSLT while ensuring optimal performance:
- Load the XSLT stylesheet: Load the XSLT stylesheet file or create one programmatically as an
XDocument
orXmlDocument
. - Compile the XSLT stylesheet: Create an instance of the
XslCompiledTransform
class and call theLoad()
method with the XSLT document:
Loading code snippet...
- Transform the input XML document: Call the
Transform()
method of theXslCompiledTransform
instance, providing the input XML document and an output destination, such as a stream or a text writer:
Loading code snippet...
The XslCompiledTransform
class provides a high-performance, compiled XSLT execution engine that can be reused for multiple transformations.
Additionally, consider these performance tips when working with XSLT:
- Use efficient XML parsing and writing classes, like
XmlReader
andXmlWriter
, for reading and writing XML data. - Optimize the XSLT stylesheet by reducing recursion, minimizing the use of variables, and applying appropriate logic to handle large XML document processing.
- Profile and fine-tune the XSLT transformations and the accompanying code to minimize bottlenecks and optimize performance.
After addressing how to create and apply XSLT to XML documents in C#, we will now examine the role of XmlResolver in C# XML processing.
In particular, we will discuss how C# developers can use XmlResolver to provide custom resolving of external resources during XML processing tasks for greater flexibility and customization.
Explain how C# developers can make use of XmlResolver to provide custom resolving of external resources during XML processing tasks.
Answer
XmlResolver
is an abstract class in the C# System.Xml
namespace that provides a mechanism to resolve external XML resources like DTDs, XSDs, XML Inclusions (XInclude), or XML external entities during XML processing tasks. XmlResolver
can be used to implement custom resolvers for resolving these external resources differently from the default behavior, such as obtaining resources from a database, remote web service, or a cache.
To create a custom XmlResolver
in C#, follow these steps:
- Create a custom class inheriting from : Create a new class that inherits from
XmlResolver
and override the relevant methods (GetEntity()
andResolveUri()
).
Loading code snippet...
- Implement custom resolving logic: Inside the overridden methods, implement the custom logic to resolve external resources (e.g., loading resources from a cache or database).
- Use the custom XmlResolver with an XmlReader or other XML processing classes: When creating an
XmlReader
,XmlWriter
,XslCompiledTransform
, or any other XML processing class that supportsXmlResolver
, set theXmlResolver
property or pass it as an argument during instantiation.
Loading code snippet...
By implementing and using a custom XmlResolver
, developers can control how external resources are resolved during XML processing tasks, providing flexibility and custom behavior as required.
Discuss how to create and implement custom XML serializers in C# that cater to specific requirements that the built-in serializers cannot handle.
Answer
When the built-in XML serializers like XmlSerializer
do not fulfill specific requirements, you can create custom XML serializers in C#. Below are the steps to create and implement a custom XML serializer:
- Create serialization and deserialization methods: Define the required serialization and deserialization methods in a new custom class. These methods should accept input and output streams or text readers and writers for reading and writing XML data.
Loading code snippet...
- Implement custom serialization logic: Implement the serialization logic inside your custom
Serialize()
method. Use classes likeXmlWriter
,XmlDocument
, orXElement
to write XML data to the output stream or writer. - Implement custom deserialization logic: Implement the deserialization logic inside your custom
Deserialize()
method. Use classes likeXmlReader
,XmlDocument
, orXElement
to read XML data from the input stream or reader. - Applying the custom XML serializer/deserializer: Use your custom
CustomXmlSerializer<T>
to serialize and deserialize objects of typeT
as needed:
Loading code snippet...
Creating and implementing custom XML serializers in C# enables you to cater to specific requirements, such as unique object structures or special XML formatting, that the built-in serializers do not support.
Describe how to work with binary-encoded XML (such as EXI or Fast Infoset) in C# for efficient parsing, querying, and serialization.
Answer
Binary-encoded XML formats like Efficient XML Interchange (EXI) or Fast Infoset aim to provide more efficient parsing, querying, and serialization compared to text-based XML representations. While the .NET Framework does not provide built-in support for these binary-encoded formats, there are third-party libraries available to facilitate working with binary-encoded XML in C#.
For example, let’s use the open-source ExiBinding
library to demonstrate the use of EXI binary-encoded XML in C#:
- Install a library: Download and install the
ExiBinding
NuGet package or another library that supports EXI or Fast Infoset. - Serialization: To serialize binary-encoded XML using EXI, create an instance of
ExiXmlTextWriter
and use it with your XML serialization logic:
Loading code snippet...
- Deserialization: To deserialize binary-encoded XML using EXI, create an instance of
ExiXmlTextReader
and use it with your XML deserialization logic:
Loading code snippet...
Working with binary-encoded XML like EXI or Fast Infoset in C# typically involves using third-party libraries to provide efficient parsing, querying, and serialization capabilities that are not available with the built-in .NET Framework classes.
Explain the concept of pull-based parsing in C# XML, the scenarios in which it is appropriate, and the technical advantages it offers over other parsing techniques.
Answer
Pull-based parsing in C# XML refers to the technique where the application actively requests the XML data while parsing, instead of relying on a parser that pushes the data to the application through events. The pull-based parsing approach is primarily implemented by the XmlReader
class in C#, which offers a forward-only, read-only, streaming access to XML data.
Scenarios where pull-based parsing is appropriate:
- Large XML documents: Pull-based parsing is suitable for processing large XML documents where loading the entire document into memory using other parsing techniques (e.g., DOM) is inefficient or impossible due to memory constraints.
- Streaming scenarios: When XML data needs to be processed as it is received (e.g., from a web service or a file stream), pull-based parsing offers a suitable approach, allowing real-time processing while more data is being acquired.
- Partial processing: In cases where the entire XML document does not need to be parsed or accessed and only specific portions are of interest, pull-based parsing is a good choice as it does not require the entire document to be loaded or parsed.
Technical advantages of pull-based parsing:
- Memory efficiency: Pull-based parsing using
XmlReader
enables you to process large XML documents without loading the entire document into memory. This is particularly useful when working with XML documents larger than the available memory, which would be troublesome with the DOM approach. - Performance: Due to its forward-only, read-only, and streaming nature, pull-based parsing can offer better performance compared to other approaches like DOM or event-based parsing for certain XML processing tasks.
- Control over parsing: With pull-based parsing, the developer has more control over the parsing process, allowing for selective processing and early termination, if needed.
- Integration with other XML classes:
XmlReader
can be used in conjunction with other XML classes likeXPathNavigator
, which provides an efficient way to query and manipulate XML data using the pull-based parsing approach.
In summary, pull-based parsing in C# XML provides an efficient and memory-friendly approach to handling large XML documents, streaming scenarios, and partial processing tasks, compared to other parsing techniques like DOM or event-based parsing.
How can you implement a C# XML processing system that is resilient to DTD or XML External Entity attacks and other security issues that may arise from processing poorly crafted or untrusted XML documents?
Answer
To implement a C# XML processing system that is resilient to DTD, XML External Entity (XXE) attacks, and other security issues, follow these security best practices:
- Disable DTD processing: When using
XmlReader
, disable DTD processing by setting theDtdProcessing
property toDtdProcessing.Prohibit
inXmlReaderSettings
:
Loading code snippet...
- Use secure XmlResolver: If you need to resolve external resources, use custom
XmlResolver
implementations that follow secure practices, like validating resource locations, whitelisting trusted resources, or limiting resource access and size. - Validate XML against a known schema: When processing untrusted XML documents, validate them against a known and trusted XML schema (XSD) to ensure they conform to the expected structure:
Loading code snippet...
- Sanitize user-generated content: If your XML processing involves user-generated content (e.g., in web applications), always sanitize and validate this content before including it in an XML document to prevent security issues like cross-site scripting (XSS) or injection attacks.
- Enforce security controls: Apply appropriate access controls, encryption, and authentication mechanisms to protect XML data and processing components. This is particularly important when dealing with sensitive information.
- Monitor the system: Regularly monitor the XML processing system for security threats, unexpected behavior, or vulnerabilities that may arise from changes in code, dependencies, or environments.
- Stay up-to-date: Keep your .NET Framework, libraries, and dependencies up-to-date to ensure that you are using the latest security fixes and improvements.
By following these security best practices, you can build a C# XML processing system that is resilient to DTD, XXE attacks, and other security issues that may arise from processing poorly crafted or untrusted XML documents.
To sum up, this article has provided an in-depth discussion of key xml interview questions c# developers need to be familiar with to excel in the IT industry. We covered a wide array of topics, from basic concepts to advanced techniques, all of which are vital for any C# developer working with XML.
We hope these xml interview questions c# developers should know have enhanced your knowledge and prepared you for your next job interview or project. Don’t hesitate to revisit this guide to refresh your understanding and stay confident in tackling the challenges that arise when working with C# and XML.
Good luck!