WCF Interview Questions And Answers
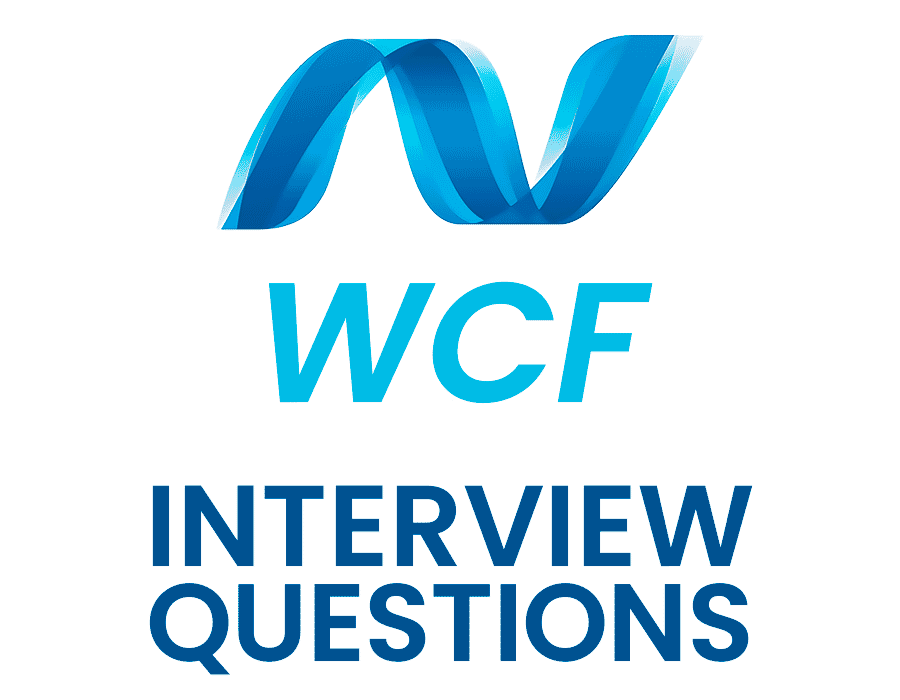
In today’s competitive job market, being well-prepared for an interview is essential. If you’re an aspiring WCF developer, having a solid grasp of WCF concepts can help you stand out from the crowd. To support you in your journey, we’ve compiled a comprehensive list of WCF interview questions and answers to help you tackle even the toughest interview questions.
So, whether you’re new to WCF or an experienced professional brushing up on your knowledge, these WCF interview questions and answers will provide valuable insights and help you prepare for your next interview with confidence.
Dive in and explore the most common and intriguing WCF scenario-based interview questions that you might encounter in your journey.
What is the primary difference between a BasicHttpBinding and a WSHttpBinding in WCF? Explain their use cases in detail.
Answer
BasicHttpBinding
and WSHttpBinding
are two binding options provided by WCF to define how a service communicates with a client. They have some key differences, as described below:
- Interoperability:
BasicHttpBinding
offers a higher degree of interoperability with non-WCF clients, whileWSHttpBinding
provides richer features for WCF-to-WCF communication. - Transport Protocol: Both bindings use HTTP as their primary transport protocol, but
WSHttpBinding
also includes other advanced protocols like WS-* specifications. - Security: By default,
BasicHttpBinding
has no security, but you can configure transport-level security (SSL). In contrast,WSHttpBinding
has message-level security by default, which ensures confidentiality, integrity, and authentication. - Session Management:
BasicHttpBinding
is stateless, whileWSHttpBinding
is stateful, supporting reliable sessions that allow maintaining the state between client and service.
Use Cases:
- Use
BasicHttpBinding
when interoperability with non-WCF or basic web service clients is a priority, when you require simple, easy setup, and when security requirements are minimal. - Choose
WSHttpBinding
when you need advanced WS-* features, when message-level security, transactions, and reliable sessions are required, or when using advanced WS-* standards (e.g., WS-ReliableMessaging) is essential.
How can we use different bindings together for different endpoints in the same WCF service?
Answer
To use different bindings for different endpoints in the same WCF service, you need to define multiple endpoints in your service configuration file. Follow the steps below:
- Define the service bindings in the
<bindings>
section of the configuration file. You can configure multiple bindings with different settings. - Create multiple endpoints for the same service contract with different bindings and/or addresses in the
<services>
section of the configuration file.
Example:
Loading code snippet...
In this example, a WCF service named MyService
exposes two endpoints: one with basicHttpBinding
and another with wsHttpBinding
. Clients can connect to the service using the binding that best suits their requirements.
Explain the role of InstanceContextMode in a WCF service’s behavior. How can it affect the performance and resource usage of the service?
Answer
InstanceContextMode
is an essential part of a WCF service’s behavior. It determines how instances of service are created, which in turn impacts performance and resource usage. InstanceContextMode
can have one of three values:
- PerCall: A new service instance is created for each client request. This provides better isolation, but increases resource usage and may impact performance.
- PerSession: A service instance is created for each client session. Multiple requests within the same session will reuse the service instance. This enhances performance by reusing resources, but may lead to increased memory usage if many concurrent sessions are active.
- Single: Only one service instance is used for all client requests. This provides the best performance in terms of resource usage, but may lead to concurrency issues.
Each InstanceContextMode
has its advantages and trade-offs. To determine the appropriate mode for your WCF service, consider the level of isolation required, the expected number of concurrent clients, and resource availability.
List the essential components of WCF architecture and explain their purpose.
Answer
The essential components of WCF architecture are:
- Service Contract: Defines the interface of the service, including the operations and data contracts. It outlines the structure of the messages exchanged between the client and service.
- Binding: Specifies how the service communicates with the client, including the transport protocol, encoding, and security options.
- Endpoint: Defines where the service is exposed and accessed. It includes an address (URI), a binding, and a service contract, often represented as “ABC” (Address, Binding, Contract).
- Service Runtime: Manages the behavior and execution of a service, handling aspects such as error handling, instancing, transactions, and security.
- Messaging Layer: Provides an abstraction for message processing, enabling various message exchange patterns, encoding, and communication protocols.
- Channel Layer: Allows communication between the client and service by creating, managing, and disposing of channels, which are responsible for message transport.
- Hosting Environment: Provides a runtime and management environment for WCF services. It can be IIS, a Windows Service, or a Managed Application.
- Metadata: Describes the service’s contract, bindings, and endpoints so the clients can interpret it and generate proxies to interact with the service.
What is the purpose of the OperationContract and ServiceContract attributes in WCF? Explain their significance in creating a WCF service.
Answer
In WCF, the OperationContract
and ServiceContract
attributes serve the following purposes:
- ServiceContract: Applied to an interface or a class, it indicates that they define a service contract in WCF. A service contract is an agreement between the service and its clients on the operations and data contracts used for communication. Example:
Loading code snippet...
- OperationContract: Applied to method signatures within a service contract, it specifies that those methods represent operations exposed by the WCF service. Clients can call these operations remotely. Example:
Loading code snippet...
The use of OperationContract
and ServiceContract
attributes is essential to create a WCF service because they define the service’s interface, which clients use to access the service. These attributes also help the WCF runtime to generate metadata for the service, enabling clients to create proxies and interact with the service.
As we’ve discussed the importance of ServiceContract and OperationContract in defining a WCF service, let’s now delve into another key aspect of WCF development – implementing secure authentication and authorization mechanisms.
So, the next question in our list of WCF interview questions will focus on this crucial topic to ensure your services are well-protected.
How can we implement authentication and authorization in WCF services securely?
Answer
To implement authentication and authorization securely in WCF services, follow these steps:
- Choose a Security Model: Select the appropriate security model based on your requirements. For example, use transport security (e.g., SSL) for BasicHttpBinding or message-level security for WSHttpBinding.
- Configure the Binding Security: Configure the chosen security mode in your WCF service’s binding configuration. For instance, set the
securityMode
,clientCredentialType
, and other relevant options. - Enable Authentication: Implement the desired authentication mechanism, such as Windows, custom username/password, or token-based authentication, by extending classes like
UserNamePasswordValidator
or implementing custom token support. - Service Authorization: Configure the service’s authorization policies using the
ServiceAuthorizationManager
class or set access control by specifying allowed roles or users in configuration or via attributes on the service. - Client-side Configuration: Configure the client-side binding and credentials to match the service’s authentication and authorization settings.
Example of a username/password-based custom validator:
Loading code snippet...
Describe the process of data serialization and deserialization in a WCF service. How can we customize the serialization process according to our requirements?
Answer
Data serialization in a WCF service refers to converting the data objects sent between the client and the service into a format suitable for transmission over the wire. Deserialization is the reverse process of converting the transmitted data back into objects on the receiving end.
By default, WCF uses the DataContractSerializer
to perform serialization and deserialization of data contracts. The default behavior can be customized using attributes like [DataContract]
and [DataMember]
. However, you can also override the serialization process by implementing custom serializers.
Here’s a high-level description of the process:
- Define data contracts using the
[DataContract]
and[DataMember]
attributes on classes and properties, respectively, to specify which parts of an object should be serialized. - For custom serialization, implement the
IXmlSerializable
interface on your data contracts that require XML-based serialization or theISerializable
interface for binary serialization. - Override the
ReadXml(XmlReader)
andWriteXml(XmlWriter)
methods (forIXmlSerializable
) orGetObjectData(SerializationInfo, StreamingContext)
and a custom deserialization constructor (forISerializable
) according to your serialization requirements. - In the WCF service configuration, specify the appropriate
DataContractSerializer
or your custom serializer, if applicable, for the<dataContractSerializer>
element.
Example of using IXmlSerializable
for custom XML serialization:
Loading code snippet...
In this example, we implemented custom XML serialization for a MyCustomData
class using the IXmlSerializable
interface, allowing us to control how the object is serialized and deserialized as XML.
What is the purpose of message contracts in WCF? Explain how it can be utilized for customizing SOAP messages and its headers.
Answer
Message contracts in WCF, defined using the [MessageContract]
attribute, allow developers to customize the structure of the SOAP messages exchanged between clients and services. They provide granular control over the content of the SOAP envelope and headers, enabling the addition, modification, or removal of SOAP elements and ensuring compliance with specific messaging requirements or protocols.
Using message contracts, developers can:
- Define the structure of SOAP messages and specify which parts of the message belong to the body or headers.
- Customize the namespaces, names, or data types of the SOAP elements.
- Additionally, message contracts can be combined with data contracts to define complex message structures.
Example:
Loading code snippet...
In this example, we define two message contracts, MyRequestMessage
and MyResponseMessage
, that include custom SOAP headers (RequestId
and ResponseId
) and body members (Data
and Result
). The IMyService
contract then uses these message contracts to define the operation ProcessRequest
, so that the service and clients communicate using the customized SOAP messages.
Explain the role of endpoints in a WCF service, and describe the different components that make up an endpoint.
Answer
Endpoints in a WCF service serve as the connection points between the service and its clients. An endpoint represents an address where clients can send messages and communicate with the service. WCF services can expose multiple endpoints for different purposes, such as supporting different bindings or providing multiple access points for clients.
An endpoint consists of:
- Address: The URI where the service can be accessed, specifying the transport protocol, location, and any path details. Example:
http://localhost:8000/MyService/Basic
. - Binding: Defines the communication details for the endpoint, including the transport protocol, message encoding, and security options. Example:
basicHttpBinding
orwsHttpBinding
. - Contract: Represents the service contract implemented by the service, which specifies the set of operations, data contracts, and fault contracts that the service exposes. Example:
IMyServiceContract
.
Together, these components are often referred to as “ABC” (Address, Binding, Contract), which represents the fundamental building blocks of a WCF endpoint.
Endpoints are configured in the service configuration file under the <services>
section, by defining an <endpoint>
element with the required information.
Example:
Loading code snippet...
In this example, we define an endpoint for the MyService
with the address http://localhost:8000/MyService/Basic
, using the basicHttpBinding
and implementing the IMyServiceContract
service contract.
How can we implement error handling and fault contracts in a WCF service to handle exceptions effectively?
Answer
WCF provides a mechanism for handling errors and exceptions through fault contracts. Fault contracts enable the service to communicate a service-specific error to the client without exposing internal implementation details or sensitive information.
Here’s how to implement error handling and fault contracts in a WCF service:
- Define a fault contract data class with properties for error details, and decorate it with the
[DataContract]
attribute. - In the service contract interface, add the
[FaultContract(typeof(MyFault))]
attribute to each operation that may generate a fault. - In the service implementation, catch specific exceptions and throw a
FaultException<MyFault>
with the fault details as a parameter. You can also implement a global error handler usingIErrorHandler
andIServiceBehavior
to handle unhandled exceptions and throw fault exceptions consistently. - On the client side, handle and process fault exceptions received from the service.
Example:
Loading code snippet...
In this example, we define a MyFault
fault contract and associate it with the service operation ProcessData
. When a fault occurs, the service throws a FaultException<MyFault>
, and the client handles it accordingly.
Now that we’ve covered essential error handling and fault contracts, it’s time to step up the game and explore performance-related aspects of WCF services.
In the upcoming section, we’ll focus on advanced WCF interview questions centered around concepts like throttling and concurrency control, which play a vital role in ensuring the maximum performance and scalability of your WCF services.
What is the concept of throttling in WCF services? How can it help to improve the performance of the service under heavy load?
Answer
Throttling in WCF services is a mechanism that allows you to limit the number of concurrent threads, instances, and sessions, ensuring that the service doesn’t get overwhelmed under heavy load. It helps prevent performance issues and potential denial-of-service (DoS) attacks by restricting the resource consumption of the service. WCF throttling is determined using three primary properties in the ServiceThrottlingBehavior
class:
MaxConcurrentCalls
: The maximum number of calls the service can handle simultaneously.MaxConcurrentInstances
: The maximum number of service instances that can exist at the same time.MaxConcurrentSessions
: The maximum number of sessions the service can have concurrently.
By tweaking these properties, you can improve the performance of the service and better manage its resource usage under heavy load. It’s essential to find the optimal balance of these values according to the specific service implementation and the hardware resources available.
Describe the process of hosting a WCF service in IIS. What are the advantages and disadvantages of using this hosting method?
Answer
Hosting a WCF service in IIS (Internet Information Services) is one of the most common methods of deployment due to its simplicity and built-in features. IIS is a web server for hosting web applications, including WCF services. To host a WCF service in IIS, follow these steps:
- Create a new or configure an existing IIS application for the WCF service.
- Add a
.svc
file for the WCF service in the IIS application folder. - Define the service’s configuration in the
web.config
file, including bindings, behaviors, and other settings.
There are several advantages to using IIS as a hosting environment for WCF services:
- Easy management and deployment using IIS Manager.
- Automatic process recycling and application pool isolation.
- Integrated support for authentication, authorization, and logging.
- Support for various protocols such as HTTP, HTTPS, and NET.TCP
- Start on demand: The service is started when the first request arrives, conserving system resources when idle.
However, there are some disadvantages as well:
- Limited to Windows-based systems.
- Dependency on IIS, which can increase complexity if you’re not familiar with it.
- May not fully utilize all WCF features, such as non-HTTP transport protocols (unless using IIS 7.x with Windows Process Activation Service).
How can we implement concurrency control in a WCF service to handle multiple requests from clients simultaneously?
Answer
Concurrency control in a WCF service is the management of multiple requests executing concurrently to ensure data consistency, performance, and overall service stability. WCF provides several mechanisms for controlling concurrency:
- InstanceContextMode: Determines the relationship between service instances and client requests. It has three options: PerCall, PerSession, and Single. Depending on the selected option, you can control the number of concurrent instances of your service.
- ConcurrencyMode: Controls how many threads can enter the service instance at the same time, and has three options: Single, Reentrant, and Multiple.
- : Doesn’t allow concurrent access; only one request at a time can access the service instance.
- : Allows the service instance to process another request while waiting for a callback from a previous one.
- : Allows multiple requests to access the service instance simultaneously.
Here’s an example of configuring a WCF service for concurrent execution:
Loading code snippet...
- Synchronization: You can use synchronization primitives (such as
Monitor
,Mutex
,Semaphore
, orlock
statement) to restrict access to specific sections of your service code, ensuring that multiple threads don’t cause issues by accessing shared resources simultaneously.
It’s crucial to choose the appropriate combination of InstanceContextMode and ConcurrencyMode based on your service’s requirements, as it can affect the service’s scalability and performance.
What are the different types of WCF service behaviors, and how can they be used to modify the behavior of a service at runtime?
Answer
WCF service behaviors are a set of properties that influence the behavior of a WCF service during runtime. These behaviors allow you to configure various aspects of the service, such as instance management, error handling, concurrency, and metadata publication. The main types of WCF service behaviors include:
- ServiceBehavior: Applied at the service level and controls service-wide aspects, such as instance context mode, concurrency mode, transaction timeouts, and include exception details in faults. Example:
Loading code snippet...
- OperationBehavior: Applied at the operation level, influencing individual operation behavior. It can be used to configure transaction scope, operation-level concurrency, and serialization of return values. Example:
Loading code snippet...
- EndPointBehavior: Applied at the endpoint level and used to configure behaviors specific to a particular endpoint, such as message encoding, authentication, and authorization settings. Example:
Loading code snippet...
Adding and configuring these behaviors gives you fine-grained control over your WCF service’s runtime behavior and allows you to adapt the service to specific requirements or scenarios.
Explain the concept of duplex communication in WCF. How can we implement callbacks between the client and the server in a WCF service?
Answer
Duplex communication in WCF refers to a bidirectional communication pattern between the client and the server where both parties can initiate communication independently. This pattern enables a WCF service to communicate back to the client using a callback mechanism, which allows the service to notify the client about certain events or updates.
To implement duplex communication, follow these steps:
- Define a callback contract that specifies the operations which the WCF service can call on the client. This contract should be marked with the
[CallbackContract]
attribute. Example:
Loading code snippet...
- In your main service contract, apply the
[ServiceContract]
attribute with theCallbackContract
property set to the callback contract. Example:
Loading code snippet...
- Implement the WCF service, including methods for the callback. Example:
Loading code snippet...
- On the client side, implement the callback contract and create a duplex channel to the WCF service using
DuplexChannelFactory
. Example:
Loading code snippet...
Having delved into the fascinating world of duplex communication and its implementation in WCF services, let’s now shift our focus towards another essential component of distributed applications: Message Queuing.
The next set of WCF scenario-based interview questions and answers will explore the role of MSMQ in WCF services and how it ensures the reliability and durability of message exchanges in a distributed environment.
What is the role of Message Queuing (MSMQ) in WCF services? How can it be used to ensure reliability and durability of messages in a distributed environment?
Answer
Message Queuing (MSMQ) is a message-based communication technology that allows distributed applications to communicate asynchronously using message queues. In WCF services, MSMQ provides a reliable and durable messaging mechanism to ensure that messages are not lost during transmission, even in case of system failure or network issues.
MSMQ can be incorporated in WCF services using the MsmqIntegrationBinding
or the NetMsmqBinding
. Key benefits of using MSMQ in WCF services include:
- Asynchronous communication: MSMQ allows messages to be exchanged asynchronously between the client and the service, enabling the sender to continue processing other tasks without waiting for a response.
- Reliable message delivery: MSMQ ensures that messages are delivered in the correct order and without duplication. It also supports transactional messages, which guarantees that the message is delivered only once and at most once.
- Message durability: Messages are stored in a queue and persist even if the system or service fails, ensuring that the messages are processed when the service becomes available again.
To use MSMQ with WCF services, follow these steps:
- Install and configure MSMQ on the system hosting the WCF service.
- Create a WCF service contract and implementation.
- Configure the WCF service’s endpoints to use MSMQ bindings.
- Host and deploy the WCF service.
- Implement the WCF client proxy to send messages via MSMQ.
By integrating MSMQ with WCF services, you can ensure reliable and durable message processing in a distributed environment.
What are the various transaction modes available in WCF services? Explain how we can enable transactions in a WCF service.
Answer
Transactions in WCF services provide a mechanism to ensure consistency and integrity of data across multiple operations. WCF supports the following transaction modes:
- No transactions: No transaction support (default behavior).
- Lightweight transactions: Local transactions within the service’s boundary.
- Distributed transactions: Transactions that span across multiple services and resources, using the Microsoft Distributed Transaction Coordinator (MSDTC).
To enable and use transactions in a WCF service, follow these steps:
- Implement your WCF service with the necessary transaction support.
- In the service contract, apply the
[OperationContract]
attribute with theTransactionFlow
property set to one of the following:
TransactionFlowOption.NotAllowed
: Transactions are not allowed (default).TransactionFlowOption.Allowed
: Transactions are allowed but not required.TransactionFlowOption.Mandatory
: Transactions are required for the operation. Example:
Loading code snippet...
- In the service implementation, apply the
[TransactionScopeRequired]
property of the[OperationBehavior]
attribute to enable transaction management for the operation. Example:
Loading code snippet...
- Choose a binding that supports transactions, such as
WSHttpBinding
withTransactionFlow
enabled orNetTcpBinding
.
By following these steps, you can enable and configure transactions in WCF services, ensuring data consistency and integrity across multiple operations.
What is the purpose of the ChannelFactory and Proxy in WCF? Explain the process of creating a WCF client using these components.
Answer
ChannelFactory
and Proxy are essential components in WCF for creating client-side communication channels to interact with a WCF service.
- ChannelFactory: Creates channels of a specific type that can be used to communicate with a service. It encloses the configuration and management of channels for a specified service contract and endpoint.
- Proxy: Acts as an intermediary between the client and the service, enabling the client to invoke methods on the service as if it were a local object. The proxy uses the channels created by the
ChannelFactory
to communicate with the service.
To create a WCF client using ChannelFactory
and Proxy, follow these steps:
- Create a reference or share the service contract interface between the client and the server projects.
- In the client project, instantiate a
ChannelFactory
for the service contract and provide the client endpoint configuration. Example:
Loading code snippet...
- Create a proxy by calling the
CreateChannel()
method on theChannelFactory
instance. Example:
Loading code snippet...
- Use the proxy to invoke methods on the WCF service. Example:
Loading code snippet...
- Close the proxy after completing the communication with the WCF service. Example:
Loading code snippet...
By using the ChannelFactory
and Proxy, you can create instances of WCF clients programmatically to communicate with WCF services efficiently.
Explain the concept of extensibility in WCF services. What are some examples of WCF extensibility points that can be used to modify the service’s functionality?
Answer
Extensibility in WCF services refers to the ability to customize or extend various aspects of the service during its lifetime without modifying the core functionality. WCF includes several extensibility points that allow you to change message processing, behavior, and interaction patterns. Some common extensibility points include:
- Behaviors: Custom behaviors can be added at the service, endpoint, or operation levels. Examples include custom error handling, caching, instrumentation, and validations.
- Message Inspectors: Allow you to intercept, inspect, and modify messages during the processing pipeline. Useful for logging, tracking, or transformation of messages.
- Channel Extensions: Allow you to create custom communication channels or modify existing ones. Useful for tasks such as custom message encoding, compression, or encryption.
- Operation Invokers: Allow you to control how service operations are invoked. This extensibility point enables applications to implement custom method invocation, pre- and post-processing of operations, or introduce specialized error handling.
- Parameter Inspectors: Enable inspection or modification of input and output parameters for service operations. This can be used for parameter validation, logging, or transformation.
- Service Host: Allows you to control how WCF services are hosted. You can create a custom service host to implement custom initialization, error handling, or lifetime management.
These extensibility points serve as a foundation for robust and adaptable WCF services. By leveraging them, you can create advanced WCF implementations tailored for specific scenarios and requirements.
How can we ensure data privacy and security when exchanging messages between a WCF client and service? Discuss the role of message encoding and encryption in this context.
Answer
Ensuring data privacy and security in WCF services is vital to protect sensitive information during message exchange between the client and the service. There are two primary mechanisms available for achieving this in WCF:
- Message Encoding: Message encoding defines how the data in a message is serialized and deserialized during transmission. Encoding options in WCF include Text, Binary, and Message Transmission Optimization Mechanism (MTOM). Binary encoding is more compact and faster than Text, providing increased performance and reduced bandwidth usage. However, encoding alone doesn’t ensure message privacy.
- Message Encryption: To provide privacy and security, messages can be encrypted using various security modes in WCF, such as:
Transport
: Encrypts messages at the transport layer using protocols like SSL/TLS. This security mode is efficient but only suitable for point-to-point communication.Message
: Encrypts messages at the message layer using WS-Security specifications. This mode provides end-to-end security and supports more complex scenarios; however, it may have performance overhead.
To ensure data privacy and security in WCF services:
- Choose an appropriate message encoding based on the service’s requirements.
- Pick a security mode (e.g., Transport or Message) that meets your privacy andencryption needs.
- Configure the chosen security mode in the service and client configuration (e.g., binding) with appropriate security settings, such as credentials and encryption algorithms.
By combining message encoding with encryption, WCF services can securely exchange messages and protect data privacy during communication between the client and the service.
By following these steps, you can implement duplex communication and facilitate callbacks between the client and the server in a WCF service.
We hope that this compilation of WCF interview questions and answers has given you a solid foundation to tackle even the most challenging WCF scenario-based interview questions. By understanding these concepts and applying them in real-world WCF development, you’ll be well-equipped to showcase your expertise and stand out in your next interview.
Remember that hands-on experience and continuous learning are key to success, so keep refining your skills and exploring new aspects of WCF development. Good luck on your journey to becoming a highly sought-after WCF developer!