Efficient Session Handling in MVC: Tips, Tricks, and Alternatives
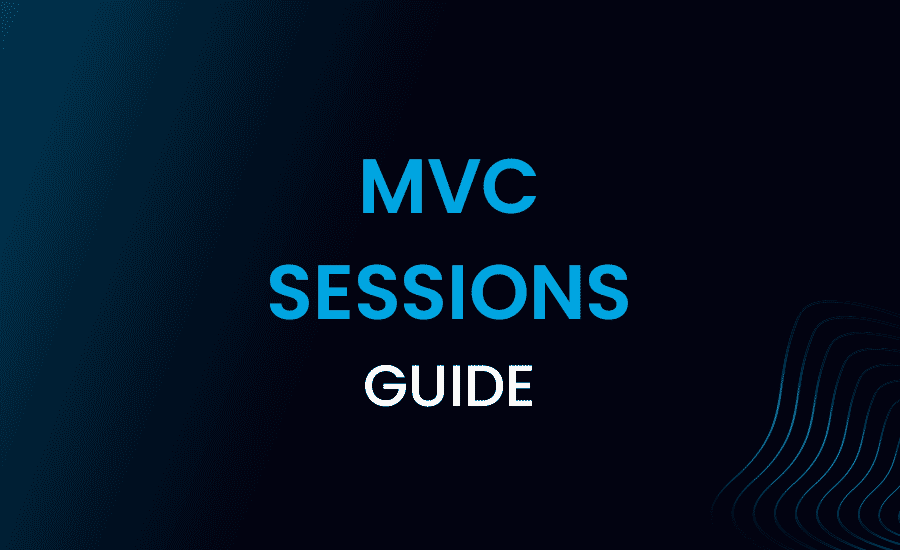
MVC, or Model-View-Controller, is a design pattern that separates the application logic into three interconnected components: Model, View, and Controller. This architectural pattern is popular for developing web applications because it promotes maintainability, scalability, and testability.
Components of MVC
- Model: Represents the application’s data and business logic. It interacts with the database and handles data processing.
- View: Responsible for displaying the data to the user. It receives data from the model and renders it.
- Controller: Manages user input and coordinates the model and view. It processes user requests and communicates with the model and view accordingly.
Benefits of MVC
- Separation of concerns: Each component has a specific responsibility, making the code more modular and easier to maintain.
- Reusability: Components can be reused across different parts of the application or even in other applications.
- Testability: Each component can be tested independently, improving the overall quality of the application.
What is a Session?
A session is a mechanism for maintaining state between requests in a stateless web environment. It allows you to store data about a user’s interaction with your application, such as their preferences, shopping cart items, or authenticated status. This information is stored on the server, and a unique session identifier is used to associate the user with their data.
Session use cases
- User authentication: Store user credentials after successful login to maintain their authenticated state across multiple requests.
- Shopping carts: Keep track of items added to a user’s cart as they navigate your online store.
- Personalization: Store user preferences to provide a customized experience during their visit.
Session Management in MVC
MVC applications can handle session management in various ways, depending on the desired behavior and storage options.
Session state
Session state is a built-in mechanism in ASP.NET MVC for managing session data. It stores data in a key-value dictionary, allowing you to easily access and modify session data throughout your application.
Session storage options
- In-process: Stores session data in the application’s memory. This is the default option but may not be suitable for large applications or distributed environments.
- State server: Stores session data in a separate process or server, improving scalability.
- SQL Server: Stores session data in a SQL Server database, providing better durability and availability.
Implementing Session in MVC
There are several ways to implement session management in MVC applications.
Using HttpSessionStateBase
HttpSessionStateBase is the standard session object in ASP.NET MVC. You can access it using the Session
property of the Controller
class. This allows you to store, retrieve, and remove session data easily using key-value pairs.
Loading code snippet...
Custom session objects
Another approach is to create custom session objects that encapsulate your session data. This can make your code more organized and easier to maintain. You can create a custom class for storing session data and use extension methods to work with the session:
Loading code snippet...
TempData
TempData
is another feature in ASP.NET MVC that allows you to store data temporarily between requests. It uses session storage under the hood but is limited to one request, making it suitable for scenarios like redirecting after form submission:
Loading code snippet...
Session Security
When working with sessions, it’s crucial to consider security risks, such as session fixation and session hijacking.
Session fixation
Session fixation occurs when an attacker tricks a user into using a session ID they already know, allowing them to hijack the user’s session once they are authenticated. To prevent session fixation, regenerate the session ID after a successful login:
Loading code snippet...
Session hijacking
Session hijacking happens when an attacker steals a user’s session ID and uses it to impersonate the user. To protect against session hijacking, follow these best practices:
- Use HTTPS to encrypt data transmitted between the client and server.
- Set the
HttpOnly
attribute on session cookies to prevent access through JavaScript. - Implement proper access controls and authentication mechanisms.
Performance Considerations
Managing sessions can impact your application’s performance, so it’s essential to consider the following factors:
Scalability
As your application grows, you may need to scale your session storage to handle the increased load. Consider using state servers or SQL Server for session storage in large applications or distributed environments.
Session timeouts
By default, session data is automatically removed after 20 minutes of inactivity. You can configure the session timeout value in your application’s configuration file. Be cautious when setting longer timeouts, as they can consume more server resources.
Alternatives to Session in MVC
There are alternatives to using sessions in MVC applications, such as ViewState, cookies, and application state.
- ViewState: Stores data on the client-side in a hidden field within the page. It’s suitable for small amounts of data but may not be ideal for sensitive information.
- Cookies: Stores data on the client-side in small text files. Cookies have size limitations and can be disabled by the user.
- Application state: Stores data on the server-side, accessible by all users. It’s useful for storing global data but not for user-specific data.
Conclusion
Session management is an essential aspect of developing MVC applications, allowing you to maintain state across multiple requests. In this article, we explored different ways to implement session management in MVC applications, security concerns, performance considerations, and alternatives to sessions. By understanding these concepts, you can create more robust and user-friendly web applications.
FAQs
- What is the primary purpose of a session in MVC? A session is used to maintain state between requests in a stateless web environment, such as user authentication, shopping carts, or personalization.
- How can I prevent session hijacking in my MVC application? To protect against session hijacking, use HTTPS to encrypt data, set the
HttpOnly
attribute on session cookies, and implement proper access controls and authentication mechanisms. - What are the drawbacks of using in-process session storage? In-process session storage stores data in the application’s memory, which may not be suitable for large applications or distributed environments due to scalability concerns.
- What is TempData, and when should I use it? TempData is a feature in ASP.NET MVC that allows you to store data temporarily between requests. It is suitable for scenarios like redirecting after form submission, as it is limited to one request.
- What are some alternatives to using sessions in MVC applications? Alternatives to sessions include ViewState, which stores data on the client-side in a hidden field within the page; cookies, which store data on the client-side in small text files; and application state, which stores data on the server-side, accessible by all users.