Selenium 5 Years Experience Interview Questions and Answers
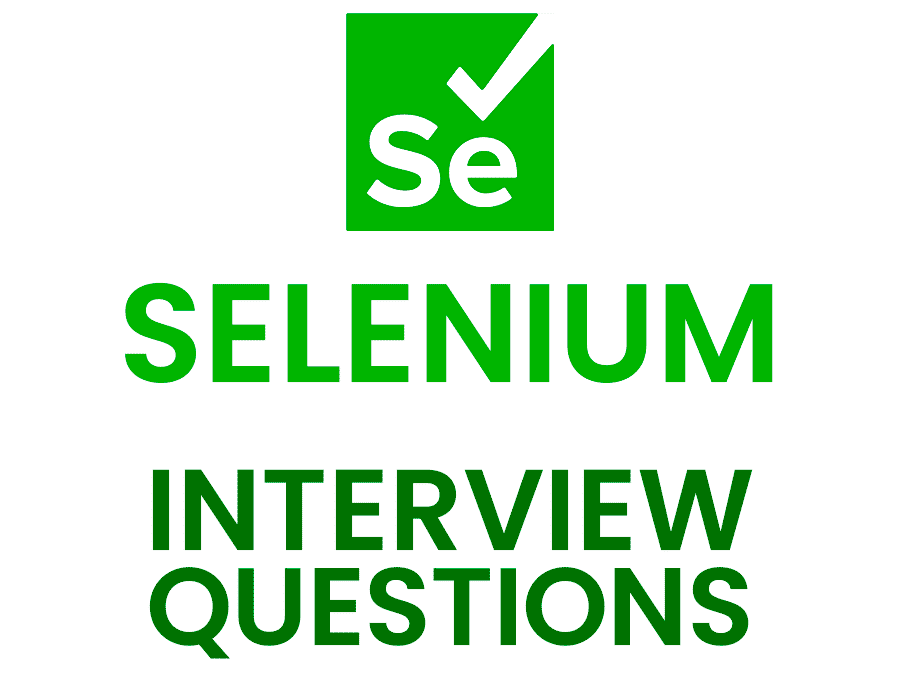
Preparing for a Selenium C# interview can be daunting, especially when you have 5 years of experience under your belt. Companies expect a higher level of expertise from experienced developers, so it’s crucial to be well-prepared for the interview.
This article covers the top Selenium C# interview questions and answers tailored for developers with 5 years of experience. We’ll discuss topics like handling dynamic web elements, Page Object Model, browser compatibility testing, synchronization, data-driven testing, and more.
By going through these questions, you’ll gain a deeper understanding of Selenium C# and be better equipped to tackle any interview challenge that comes your way.
How do you handle dynamic web elements in Selenium C#? Can you provide a brief explanation of different strategies you use?
Answer
Handling dynamic web elements in Selenium C# can be challenging as their attributes may change during runtime. Here are some strategies to handle dynamic elements:
- Use partial attributes: Use
contains
,starts-with
, orends-with
in your XPath and CSS selectors to match elements with dynamic attributes partially.
Loading code snippet...
- Use sibling or child elements: If a dynamic element has a stable sibling or child element, you can use it as an anchor to find the dynamic element.
Loading code snippet...
- Wait for elements: Sometimes, elements may not be immediately available. In such cases, use explicit waits to ensure the element is available before interacting with it.
Loading code snippet...
- Use JavaScript: When traditional methods fail, you can use JavaScript to interact with dynamic elements.
Loading code snippet...
Can you explain the concept of Page Object Model (POM) in Selenium C#? How does it help to create a more maintainable and scalable test automation framework?
Answer
Page Object Model (POM) is a design pattern in Selenium that promotes the separation of test scripts and page-specific code. It helps create a more maintainable and scalable test automation framework by providing the following benefits:
- Encapsulation: Each page in the application has a corresponding Page Object class that encapsulates all the web elements and their interactions. This way, the test script only needs to interact with the Page Object, not the web elements directly.
- Code reusability: Page Objects can be reused across multiple test scripts, reducing code duplication and making it easier to maintain the test suite.
- Readability: Test scripts become more readable and easier to understand since they use Page Object methods instead of dealing with low-level details like locators and interactions.
- Easier maintenance: When the application’s UI changes, you only need to update the corresponding Page Object, not every test script that interacts with those elements. This makes maintaining the test suite more manageable.
Here’s an example of a simple Page Object in C#:
Loading code snippet...
And the corresponding test script using this Page Object:
Loading code snippet...
In Selenium C#, how do you handle browser compatibility testing? Can you give an example of how you would set up a test suite to run on multiple browsers?
Answer
Browser compatibility testing in Selenium C# can be achieved by running your test suite on different browsers. To do this, you need to initialize the WebDriver instances for each browser you want to test and execute your test cases across all of them. Here’s an example of how to set up a test suite to run on multiple browsers:
- First, install the necessary WebDriver NuGet packages for the browsers you want to test (e.g.,
Selenium.Chrome.WebDriver
,Selenium.Firefox.WebDriver
, andSelenium.Microsoft.Edge.WebDriver
). - Create an enumeration for the browsers you want to test:
Loading code snippet...
- Add a method to initialize the WebDriver instance based on the browser:
Loading code snippet...
- Use the initialization method in your test cases for different browsers:
Loading code snippet...
By following this approach, you can run your test suite on multiple browsers, ensuring that your application is compatible across different browser environments.
How do you manage synchronization issues in Selenium C#? Can you explain the difference between implicit wait, explicit wait, and FluentWait?
Answer
Synchronization issues in Selenium C# can be managed using different types of waits. Waits ensure that the WebDriver waits for a specified condition to be met before proceeding with the next action. There are three types of waits available in Selenium C#:
- Wait: This type of wait is used to set a default waiting time for all the elements in a test script. If an element is not available immediately, the WebDriver will keep polling the DOM until the element is available or the specified timeout is reached.
Loading code snippet...
- Explicit Wait: This type of wait is used to wait for a specific condition to be met before proceeding. It is more precise than Implicit Wait and can be used for cases where you need to wait for a specific element or state.
Loading code snippet...
- FluentWait: FluentWait is a more advanced and customizable version of Explicit Wait. It allows you to specify the maximum waiting time, polling interval, and custom conditions or exceptions to ignore while waiting.
Loading code snippet...
In summary:
- Use Implicit Wait to set a default waiting time for all elements in your test script.
- Use Explicit Wait when you need to wait for a specific element or condition before proceeding.
- Use FluentWait when you require advanced customization and more control over the waiting process.
Can you describe the process of data-driven testing in Selenium C#? How do you implement data-driven tests using NUnit or xUnit?
Answer
Data-driven testing is a technique in which test data is stored separately from the test script, allowing you to execute the same test case with multiple sets of input data. This approach helps improve test coverage, reduce code duplication, and make tests more maintainable.
In Selenium C#, you can implement data-driven tests using NUnit or xUnit testing frameworks. Here’s how to do it:
Using NUnit:
- Install the
NUnit
andNUnit3TestAdapter
NuGet packages. - Use the
TestCase
orTestCaseSource
attribute to specify the input data for your test methods.
Example using TestCase
:
Loading code snippet...
Example using TestCaseSource
:
Loading code snippet...
Using xUnit:
- Install the
xunit
,xunit.runner.visualstudio
, andMicrosoft.NET.Test.Sdk
NuGet packages. - Use the
InlineData
,MemberData
, orClassData
attribute to specify the input data for your test methods.
Example using InlineData
:
Loading code snippet...
Example using MemberData
:
Loading code snippet...
By using data-driven testing techniques in Selenium C#, you can create more efficient and maintainable test cases.
Navigating through the complexities of Selenium C# can be a challenging task, but as you dive deeper into the topics, you’ll start to see the bigger picture.
As we continue with our interview questions and answers, we’ll explore advanced topics like DesiredCapabilities, ChromeOptions, handling frames and iframes, Selenium Grid, and custom exception handling.
These topics will help you build a solid foundation in Selenium C# and give you the confidence to ace your interview.
Can you explain the use of DesiredCapabilities and ChromeOptions in Selenium C#? How do you use these classes to customize browser settings and configurations?
Answer
DesiredCapabilities and ChromeOptions are classes in Selenium C# used to customize browser settings and configurations before launching the browser.
DesiredCapabilities (Deprecated)
DesiredCapabilities class is now deprecated and should be replaced with browser-specific options classes like ChromeOptions, FirefoxOptions, or EdgeOptions. This class was used to set the desired capabilities or properties for the WebDriver, such as browser settings, proxy settings, and handling SSL certificates.
ChromeOptions
ChromeOptions is a class that allows you to customize the Chrome browser settings and configurations before launching it. Some common use cases include:
- Setting browser preferences
- Adding command-line arguments
- Adding browser extensions
- Configuring proxy settings
Here’s an example of using ChromeOptions to customize browser settings:
Loading code snippet...
By using DesiredCapabilities (deprecated) and browser-specific options classes like ChromeOptions, you can customize browser settings and configurations to meet your testing requirements.
How do you handle frames and iframes in Selenium C#? What are the methods available to switch between frames and the main window?
Answer
In Selenium C#, you can handle frames and iframes by switching the WebDriver’s context to the desired frame before interacting with the elements inside it. There are several methods available to switch between frames and the main window:
- Switch to frame by index: Use the
SwitchTo().Frame()
method with an integer argument representing the frame’s index (0-based).
Loading code snippet...
- Switch to frame by name or ID: Use the
SwitchTo().Frame()
method with a string argument representing the frame’s name or ID.
Loading code snippet...
- Switch to frame using a WebElement: First, locate the frame or iframe element using any locator strategy, and then pass the WebElement as an argument to the
SwitchTo().Frame()
method.
Loading code snippet...
- Switch back to the main window: To switch back to the main window or the parent frame, use the
SwitchTo().DefaultContent()
orSwitchTo().ParentFrame()
methods, respectively.
Loading code snippet...
Here’s an example of switching between frames and the main window:
Loading code snippet...
By using these methods, you can easily switch between frames and iframes, and interact with the elements inside them.
Can you describe how you would use Selenium Grid in C# for parallel test execution? How does it help in reducing the overall test execution time?
Answer
Selenium Grid is a tool that allows you to run your Selenium tests concurrently on multiple browsers and platforms, thus reducing the overall test execution time. It consists of a central Hub and multiple Nodes where the tests are executed.
Here’s how you can use Selenium Grid in C# for parallel test execution:
- Set up the Selenium Grid: Download the Selenium Server JAR file and start the Hub and Nodes using the following commands:
Loading code snippet...
Replace <hub-ip>
with the IP address of the machine running the Hub, and x.y.z
with the version of the Selenium Server.
- Configure the RemoteWebDriver: In your test code, use the
RemoteWebDriver
class instead of the regular WebDriver (e.g.,ChromeDriver
orFirefoxDriver
). Specify the URL of the Selenium Grid Hub and the desired capabilities or browser options.
Loading code snippet...
- Run tests in parallel: Use a test runner like NUnit or xUnit to execute your tests concurrently. Configure the test runner to run tests in parallel, either by using attributes or modifying the test runner’s configuration file. Example using NUnit’s
Parallelizable
attribute:
Loading code snippet...
By using Selenium Grid and setting up your tests for parallel execution, you can significantly reduce the overall test execution time, which is especially beneficial for large test suites and cross-browser testing.
What is the role of exception handling in Selenium C# test automation? Can you provide an example of handling a custom exception in your test script?
Answer
Exception handling plays a crucial role in Selenium C# test automation, as it helps you deal with unexpected errors that may occur during test execution. Proper exception handling can:
- Provide meaningful error messages to help you identify and fix issues in your test scripts.
- Prevent your test suite from crashing due to unhandled exceptions.
- Allow you to implement custom error handling logic, such as retries, logging, or taking screenshots on failure.
Here’s an example of handling a custom exception in a Selenium C# test script:
Loading code snippet...
In this example, we catch a NoSuchElementException
and throw a custom exception with a more specific error message. This approach allows you to provide more meaningful error messages and handle exceptions specific to your test scenarios.
Can you explain how to create a custom HTML report for your Selenium C# test results? What are the key features you would include in your report for better visualization and understanding of the test results?
Answer
Creating a custom HTML report for your Selenium C# test results can help you better visualize and understand the test results, making it easier to identify issues and their root causes. To create a custom HTML report, you can use third-party libraries like ExtentReports or implement your own reporting logic. Here’s an outline of how you can create a custom HTML report using ExtentReports:
- Install the
ExtentReports
andExtentReports.Core
NuGet packages. - Initialize an instance of
ExtentReports
and create an HTML reporter.
Loading code snippet...
- Create test cases and log their results using the
ExtentReports
instance.
Loading code snippet...
By using a custom HTML report, you can include key features to improve the visualization and understanding of the test results, such as:
- Test case names and descriptions.
- Test execution status (Pass/Fail/Skip).
- Detailed step-by-step logs, including screenshots on failure.
- Test execution duration and timestamps.
- Grouping and filtering of test cases.
- Charts and graphs for a visual representation of test results.
By incorporating these features into your custom HTML report, you can gain a better understanding of your test results and make it easier to identify issues and areas for improvement.
Conclusion
In conclusion, this article has provided you with an extensive list of Selenium C# interview questions and answers for developers with 5 years of experience. By reviewing these questions and understanding the concepts behind them, you’ll be well-prepared for your upcoming interview.
Remember that practice makes perfect, so don’t hesitate to work on your own test automation projects to sharpen your skills and gain hands-on experience. Good luck with your interview, and may your journey as a Selenium C# developer be rewarding and successful!