Selenium 10 Years Experience Interview Questions and Answers
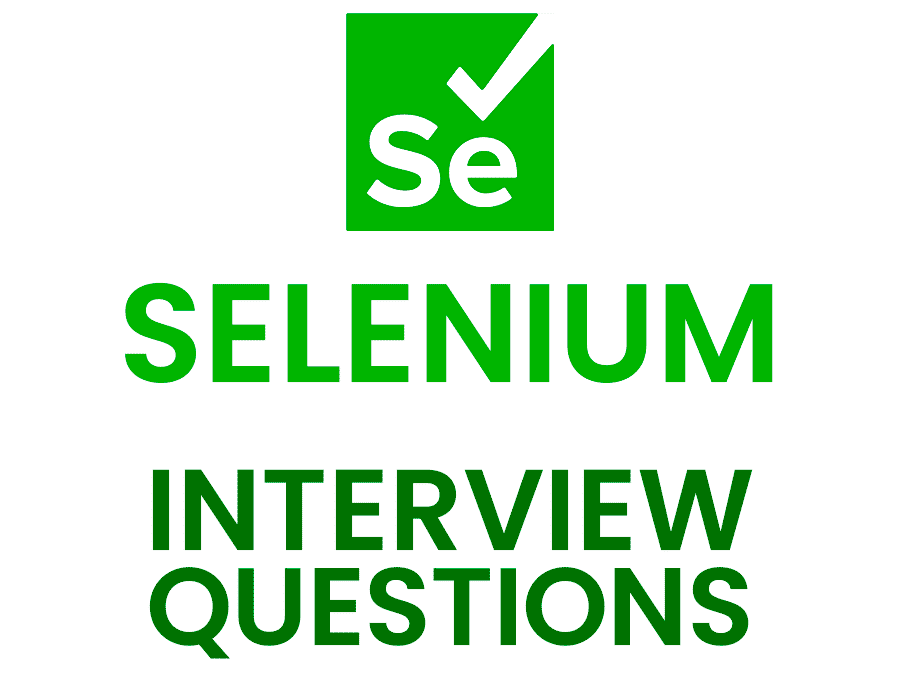
In the ever-evolving world of software development, professionals with 10 years of experience or more are often faced with challenging selenium C# interview questions to showcase their expertise. This article delves into a comprehensive list of Selenium C# interview questions for 10 years experience, designed to help experienced professionals prepare for their next job opportunity.
The questions cover various topics, including complex synchronization, the Page Object Model, Selenium Grid, custom Expected Conditions, and more. So, buckle up and get ready to dive into the world of Selenium C# and gain the knowledge you need to ace that interview.
How do you handle complex synchronization issues when working with dynamic web elements in Selenium C# to avoid race conditions?
Answer
To handle complex synchronization issues in Selenium C# and avoid race conditions, you can use various strategies like implicit waits, explicit waits, and custom waits. These waits help ensure that the test execution pauses until the desired web elements are available, avoiding errors and providing accurate test results.
- Implicit Wait: This wait tells the WebDriver to wait for a specified amount of time before throwing a NoSuchElementException if an element is not found. It applies to all elements in the script.
Loading code snippet...
- Explicit Wait: This wait is used when a specific element takes more time to load or become visible. It includes WebDriverWait and ExpectedConditions to wait for a particular condition to be met.
Loading code snippet...
- Custom Wait: If neither implicit nor explicit waits meet your requirements, you can create custom wait functions to handle complex synchronization issues. You can use a combination of loops, try-catch blocks, and sleeps to achieve the desired synchronization.
Loading code snippet...
By using these waits and synchronization techniques, you can effectively handle complex synchronization issues and avoid race conditions in your Selenium C# tests.
When using the Page Object Model pattern in Selenium C#, what’s the best way to manage multiple inheritance to maximize code reusability and maintainability?
Answer
In C#, multiple inheritance is not supported for classes. However, you can achieve code reusability and maintainability in the Page Object Model (POM) pattern by using interfaces and composition along with inheritance.
- Interfaces: Create interfaces that define the common methods and properties for similar page objects. Implement the interfaces in the respective page objects to ensure consistency.
Loading code snippet...
- Composition: Instead of inheriting from multiple classes, use composition to include instances of other classes within a page object. This allows you to reuse the functionality provided by the included classes while avoiding the limitations of multiple inheritance.
Loading code snippet...
Combining the use of interfaces and composition allows you to maximize code reusability and maintainability while adhering to the Page Object Model pattern in Selenium C#.
Can you explain how to use Selenium Grid in combination with C# to run parallel tests on different browsers and platforms for efficient cross-browser testing?
Answer
Selenium Grid allows you to run tests in parallel across different browsers and platforms, which helps in efficient cross-browser testing. To configure Selenium Grid with C# for parallel testing, follow these steps:
- Setup Selenium Grid: Download the Selenium Grid server and start the Hub and Nodes. You can configure the nodes with different browsers and platforms. Here’s an example of starting the hub and registering a node with Chrome and Firefox browsers:
Loading code snippet...
- Create Test Classes: Create test classes with test methods to be executed in parallel. Use the
[TestFixture]
attribute to define the test class,[SetUp]
attribute for setup method, and[Test]
attribute for test methods.
Loading code snippet...
- Configure Parallel Execution: Configure your test runner to execute tests in parallel. If you are using NUnit, you can use the
LevelOfParallelism
attribute to specify the number of parallel test threads. Add this attribute to your AssemblyInfo.cs file:
Loading code snippet...
- Specify Browser and Platform: Create a base class to handle driver initialization and pass the desired browser and platform information. This information can be passed through command-line arguments, configuration files, or environment variables.
Loading code snippet...
- Initialize Driver in Test Methods: Call the
Setup()
method in each test method or in a[SetUp]
method, passing the desired browser name.
Loading code snippet...
With these configurations, your Selenium C# tests will run in parallel across different browsers and platforms using Selenium Grid, improving cross-browser testing efficiency.
In Selenium C#, how do you implement custom Expected Conditions to create a more readable and maintainable codebase for handling specific web elements?
Answer
To implement custom Expected Conditions in Selenium C#, you can create your own wait methods or classes that use the WebDriverWait and Func delegate. These custom conditions make your code more readable and maintainable.
Here’s an example of creating a custom Expected Condition that waits for an element to have a specific CSS class:
Loading code snippet...
To use this custom Expected Condition, you can create a wait method that takes the locator and class name as parameters:
Loading code snippet...
Now you can call the WaitForElementToHaveClass()
method in your test code:
Loading code snippet...
By implementing custom Expected Conditions like this, you can create a more readable and maintainable codebase for handling specific web elements when using Selenium C#.
How would you optimize the performance of your Selenium C# test scripts to reduce execution time while maintaining the integrity of the tests?
Answer
Optimizing the performance of Selenium C# test scripts involves various strategies to reduce execution time while ensuring the tests remain reliable and accurate. Here are some techniques to improve your test script performance:
- Parallel Execution: Run your test cases in parallel to reduce overall execution time. Configure your test runner, such as NUnit or xUnit, to execute test cases concurrently.
- Use Appropriate Waits: Avoid using Thread.Sleep() and use implicit or explicit waits, and custom Expected Conditions when necessary. This ensures that the test execution only waits as long as needed for a specific condition to be met.
- Optimize Test Data: Minimize the amount of test data and focus on the most relevant test scenarios. Large datasets can slow down test execution.
- Optimize Locator Strategies: Use efficient locator strategies, such as ID or CSS selectors, to find web elements quickly. Avoid using XPath or locating elements by text when possible, as these can be slower.
- Reuse Browser Instances: Reuse existing browser instances whenever possible instead of opening and closing the browser for each test case. This reduces the overhead of browser initialization and teardown.
- Test Only Critical Paths: Focus on testing the most critical and frequently used paths in your application. Avoid testing every possible combination, which can lead to unnecessarily long test execution times.
- Use Headless Browsers: Headless browsers can execute tests faster than full browsers since they don’t have a graphical user interface. Use headless browsers, such as Headless Chrome or Headless Firefox, when possible.
- Continuous Integration and Distributed Testing: Use a Continuous Integration (CI) system to run tests frequently and distribute tests across multiple machines to reduce the overall execution time.
By applying these optimization techniques, you can reduce the execution time of your Selenium C# test scripts while maintaining the integrity of the tests.
As we’ve discussed various topics in Selenium C# so far, it’s essential to keep in mind that the ultimate goal is to create efficient, maintainable, and effective test automation processes.
With that in mind, let’s now explore how Selenium C# can be integrated with Continuous Integration (CI) tools and what factors should be considered to ensure a seamless and robust testing pipeline for your projects.
When working with Selenium C# and a Continuous Integration (CI) tool, what are some key considerations for setting up an effective CI pipeline for automated testing?
Answer
Setting up an effective CI pipeline for automated testing with Selenium C# involves various key considerations to ensure consistent and reliable test results. Here are some important factors to consider:
- Source Control Management: Integrate your CI tool with a source control management system, such as Git, to trigger the pipeline whenever changes are pushed to the repository.
- Build Process: Configure your CI pipeline to build your application and test projects. This includes restoring NuGet packages, compiling the application, and creating artifacts.
- Test Environment: Set up a dedicated test environment with the required browsers, platforms, and dependencies for test execution. Ensure that the test environment is isolated from development and production environments.
- Test Execution: Configure your CI pipeline to execute Selenium C# tests using a test runner, such as NUnit or xUnit. Ensure that the test runner is configured for parallel execution to minimize test execution time.
- Test Reporting: Integrate your CI tool with a test reporting system to collect and visualize test results. This helps in identifying test failures and trends.
- Notifications: Configure your CI tool to send notifications on build and test failures to relevant team members through email, Slack, or other communication channels.
- Artifact Management: Store build artifacts, such as compiled application files and test results, for a certain period to enable traceability and debugging.
- Versioning and Deployment: Implement versioning for your application to ensure that different versions can be deployed and tested independently.
- Automated Deployment: Configure your CI pipeline to deploy the application to various environments, such as staging or production, based on predefined conditions.
- Pipeline Optimization: Continuously monitor and optimize your CI pipeline to reduce execution time, improve resource utilization, and enhance the overall testing process.
By considering these factors and implementing best practices, you can set up an effective CI pipeline for automated testing with Selenium C# that ensures consistent and reliable test results while minimizing the time and effort required for test execution.
What is the best approach to handle and recover from exceptions in Selenium C# to ensure that tests continue running and provide accurate results?
Answer
To handle and recover from exceptions in Selenium C# effectively, you can follow these best practices:
- Use try-catch blocks: Encapsulate your test steps in try-catch blocks to handle exceptions. This way, if an exception occurs, the code inside the catch block can perform necessary actions to recover from the error and continue the test execution.
Loading code snippet...
- Create custom exception classes: Define custom exception classes that inherit from the base
Exception
class. This allows you to control exception handling more effectively and helps to differentiate between different types of exceptions in your test scripts.
Loading code snippet...
- Implement a centralized exception handling mechanism: Create a centralized class or method to handle exceptions throughout your test scripts. This ensures consistent handling of exceptions and reduces code duplication.
Loading code snippet...
- Use finally block: If you have any resources that need to be cleaned up or closed, use a finally block to ensure they are disposed of properly, even if an exception occurs.
Loading code snippet...
- Implement retries: For flaky tests or intermittent issues, implement a retry mechanism to rerun the failed test cases a certain number of times before marking them as failed.
By following these best practices, you can handle and recover from exceptions effectively in your Selenium C# test scripts and ensure accurate test results.
Can you explain the optimal way to manage and maintain test data for data-driven testing in Selenium C# to ensure consistent and reliable test results?
Answer
Managing and maintaining test data for data-driven testing in Selenium C# involves the following best practices:
- Externalize test data: Store test data in external files or databases instead of hardcoding it in the test scripts. This makes it easier to update the test data and maintain the tests.
- Use appropriate data sources: Select the right data source for your test data. Common data sources include CSV files, Excel files, XML files, and databases. Choose the one that fits your project requirements and is easy to maintain.
Loading code snippet...
- Utilize NUnit’s data-driven testing features: NUnit provides attributes like
[TestCase]
,[TestCaseSource]
, and[TestDataSource]
to facilitate data-driven testing. Leverage these attributes to easily parameterize your test methods and use external data sources.
Loading code snippet...
- Create a data-driven test framework: Develop a test framework that supports data-driven testing by providing utility methods and classes to read and manage test data. This ensures consistency and reusability across your test scripts.
- Maintain data consistency: Ensure that your test data is consistent and reliable by keeping it up-to-date and synchronized with the application’s data schema. Validate the test data regularly to avoid false test failures.
- Isolate test data: If possible, isolate test data for each test case to avoid conflicts between tests. This can be achieved by creating separate test data sets for each test or by using unique identifiers in the test data.
By following these best practices, you can optimally manage and maintain test data for data-driven testing in Selenium C# and ensure consistent and reliable test results.
How do you effectively implement a hybrid test automation framework in Selenium C# that combines the benefits of multiple automation approaches to meet the needs of different test scenarios?
Answer
A hybrid test automation framework combines the benefits of various automation approaches to accommodate different test scenarios. In Selenium C#, you can create a hybrid framework by incorporating elements from different frameworks, such as the Page Object Model, Keyword Driven, and Data-Driven frameworks. Here’s a high-level approach to creating a hybrid framework:
- Page Object Model (POM): Implement the POM pattern to create reusable and maintainable page objects that represent different sections or pages of your application. This enables you to separate the test logic from the UI elements and their locators.
Loading code snippet...
- Keyword Driven Approach: Create a set of keywords representing different actions or operations that can be performed on the application. These keywords will be used to create test cases in a more readable format.
Loading code snippet...
- Data-Driven Approach: Use external data sources, such as JSON, XML, or CSV files, to store test data. This helps in separating test data from the test logic, making it easier to maintain and update test cases.
Loading code snippet...
- Test Execution Engine: Create a test execution engine that reads the test cases and data from the external data source and executes the corresponding actions using the Page Object Model and Keywords.
Loading code snippet...
By combining these approaches, you can create a flexible and maintainable hybrid test automation framework in Selenium C# that can handle different test scenarios and requirements effectively.
In Selenium C#, what are some advanced techniques for locating web elements that may not be easily identifiable using standard methods, such as dynamic IDs or elements within iframes?
Answer
There are several advanced techniques for locating web elements in Selenium C# that are not easily identifiable using standard methods:
- XPath Expressions: When an element doesn’t have a unique ID or class, you can use XPath expressions to locate it based on its position in the HTML DOM, attributes, or relationship with other elements.
Loading code snippet...
- CSS Selectors: Use advanced CSS selectors to locate elements based on their attributes, relationships with other elements, or pseudo-classes.
Loading code snippet...
- Chained Locators: If an element is not directly identifiable, you can chain multiple locators to navigate through the DOM and find the desired element.
Loading code snippet...
- Handling iframes: If an element is located within an iframe, you need to switch the WebDriver’s context to the iframe before locating the element.
Loading code snippet...
- Locating Elements by JavaScript: When other methods fail, you can use JavaScript to locate elements. This can be particularly useful for elements that are generated or modified by JavaScript.
Loading code snippet...
By using these advanced techniques, you can locate web elements in Selenium C# that are not easily identifiable using standard methods, ensuring your tests are more robust and resilient to changes in the application.
Conclusion
We hope this comprehensive compilation of Selenium C# interview questions for 10 years experience has provided you with valuable insights and a deeper understanding of the subject matter. By thoroughly preparing and reviewing these questions, you can confidently approach your next interview and demonstrate your expertise in Selenium C#.
Remember, the key to acing any interview lies in being well-prepared, understanding the core concepts, and showcasing your ability to adapt to different test scenarios. With the knowledge gained from this article, you are now one step closer to landing that dream job in the world of test automation using Selenium C#.
Good luck!